Mastering JPA Criteria Queries: Common Hibernate Pitfalls
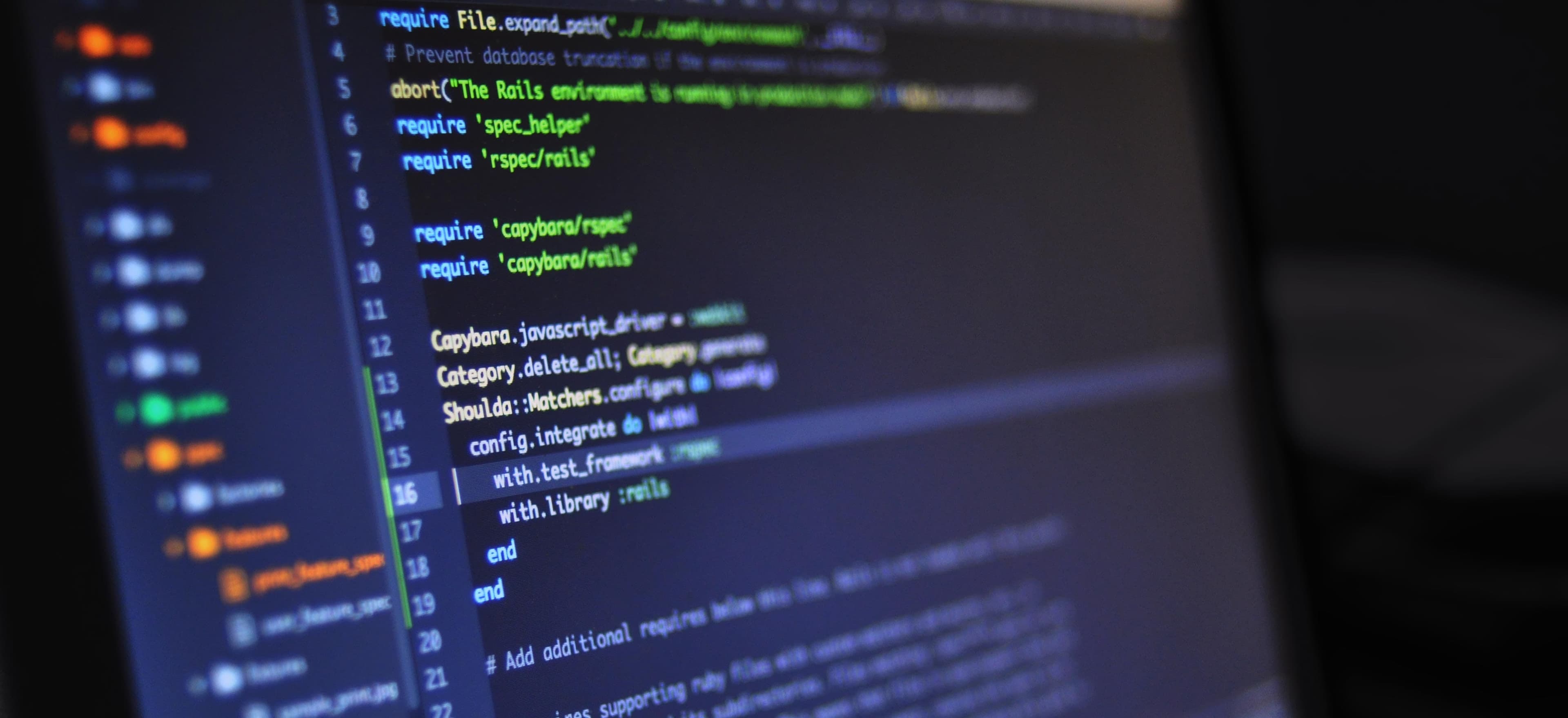
- Published on
Mastering JPA Criteria Queries: Common Hibernate Pitfalls
When working with Java Persistence API (JPA) and Hibernate ORM, executing queries can often be a source of confusion and frustration. While criteria queries offer a type-safe and flexible way to build queries programmatically, they can also introduce common pitfalls that developers must navigate. This blog post aims to dive deep into JPA criteria queries, highlighting common mistakes and how to avoid them while providing best practices.
What Are JPA Criteria Queries?
JPA criteria queries are a powerful feature that allows developers to create dynamic queries, reducing the risk of SQL Injection and making the queries easier to maintain. Unlike JPQL (Java Persistence Query Language), which is string-based, the Criteria API provides a programmatic way to compose queries using Java objects.
Basic Structure of a Criteria Query
Here’s a simple illustration of how to use JPA criteria queries:
import javax.persistence.criteria.CriteriaBuilder;
import javax.persistence.criteria.CriteriaQuery;
import javax.persistence.criteria.Root;
public List<Employee> getEmployees(EntityManager entityManager) {
CriteriaBuilder criteriaBuilder = entityManager.getCriteriaBuilder();
CriteriaQuery<Employee> criteriaQuery = criteriaBuilder.createQuery(Employee.class);
Root<Employee> employeeRoot = criteriaQuery.from(Employee.class);
criteriaQuery.select(employeeRoot);
return entityManager.createQuery(criteriaQuery).getResultList();
}
In this snippet:
- We first obtain a
CriteriaBuilder
instance, which is used to construct the criteria query. - We create a
CriteriaQuery
object, specifying the type of the result (in this case,Employee
). - We define the root entity from which we want to retrieve data.
Common Pitfalls in JPA Criteria Queries
While JPA criteria queries are powerful, they are not without their issues. Below are some common pitfalls developers may encounter along with solutions to address them.
1. Performance Issues Due to Multiple Queries
One frequent mistake is executing multiple queries instead of a single query which can drastically affect performance. For instance, in a one-to-many relationship, fetching associated entities with separate queries can slow down an application.
Solution: Use JOIN
queries to fetch related entities in a single go.
import javax.persistence.criteria.Join;
import javax.persistence.criteria.Predicate;
public List<Department> getDepartmentsWithEmployees(EntityManager entityManager) {
CriteriaBuilder criteriaBuilder = entityManager.getCriteriaBuilder();
CriteriaQuery<Department> criteriaQuery = criteriaBuilder.createQuery(Department.class);
Root<Department> departmentRoot = criteriaQuery.from(Department.class);
// Using JOIN
Join<Department, Employee> employeeJoin = departmentRoot.join("employees");
criteriaQuery.select(departmentRoot).distinct(true);
criteriaQuery.where(criteriaBuilder.equal(employeeJoin.get("status"), "ACTIVE"));
return entityManager.createQuery(criteriaQuery).getResultList();
}
Why? This example uses a join to get departments with active employees in a single query, thus optimizing performance.
2. Failing to Handle NULL Values
Criteria queries can return unexpected results if NULL values in your database are not handled correctly. If you're not careful, you might inadvertently filter out critical data.
Solution: Make explicit conditions to handle NULL values.
public List<Employee> getActiveEmployees(EntityManager entityManager) {
CriteriaBuilder criteriaBuilder = entityManager.getCriteriaBuilder();
CriteriaQuery<Employee> criteriaQuery = criteriaBuilder.createQuery(Employee.class);
Root<Employee> employeeRoot = criteriaQuery.from(Employee.class);
// Check for NULL values
Predicate statusPredicate = criteriaBuilder.and(
criteriaBuilder.equal(employeeRoot.get("status"), "ACTIVE"),
criteriaBuilder.isNotNull(employeeRoot.get("department"))
);
criteriaQuery.where(statusPredicate);
return entityManager.createQuery(criteriaQuery).getResultList();
}
Why? By adding a condition that checks for NULL values, we ensure that only active employees with assigned departments are returned.
3. Inefficient Use of Expressions
Creating overly complex expressions can lead to decreased readability and performance. Deeply nested predicates make the code harder to maintain and could result in less efficient SQL queries.
Solution: Keep your expressions straightforward and readable.
public List<Employee> getFilteredEmployees(EntityManager entityManager, String department, Integer minSalary) {
CriteriaBuilder criteriaBuilder = entityManager.getCriteriaBuilder();
CriteriaQuery<Employee> criteriaQuery = criteriaBuilder.createQuery(Employee.class);
Root<Employee> employeeRoot = criteriaQuery.from(Employee.class);
Predicate departmentPredicate = criteriaBuilder.equal(employeeRoot.get("department").get("name"), department);
Predicate salaryPredicate = criteriaBuilder.greaterThan(employeeRoot.get("salary"), minSalary);
criteriaQuery.where(criteriaBuilder.and(departmentPredicate, salaryPredicate));
return entityManager.createQuery(criteriaQuery).getResultList();
}
Why? This example illustrates how to keep conditions direct, improving maintainability. Additionally, readability aids in preventing future errors, especially in large projects.
4. Not Using TypedQuery
Failing to use TypedQuery
can lead to unchecked conversions which may cause runtime exceptions. While this isn’t strictly an issue with the Criteria API itself, it’s generally a best practice to adopt the type-safe mechanism.
Solution: Always utilize TypedQuery
.
TypedQuery<Employee> query = entityManager.createQuery(criteriaQuery);
return query.getResultList();
Why? Using TypedQuery
enhances type-safety, reducing the risk of ClassCastException at runtime. It ensures that the returned list always matches the expected type.
5. Ignoring Transaction Management
When performing multiple operations with JPA, ensuring transaction management is vital. Neglecting to do so might result in inconsistent states.
Solution: Always wrap your database transactions properly.
EntityTransaction transaction = entityManager.getTransaction();
transaction.begin();
try {
List<Employee> activeEmployees = getActiveEmployees(entityManager);
// Perform other operations...
transaction.commit();
} catch (Exception e) {
transaction.rollback();
throw e;
}
Why? Employing transaction management is crucial for data integrity. If an error occurs, rolling back ensures that the previous state remains unaffected.
The Closing Argument
Mastering JPA Criteria Queries and being mindful of their common pitfalls is essential for effective data retrieval and manipulation in Java applications. By adhering to best practices and avoiding these common mistakes, you can write cleaner, more efficient code.
For additional resources, consider visiting Baeldung's guide on JPA for a comprehensive understanding of JPA queries.
Feel free to explore, experiment, and, above all, keep learning. Happy coding!