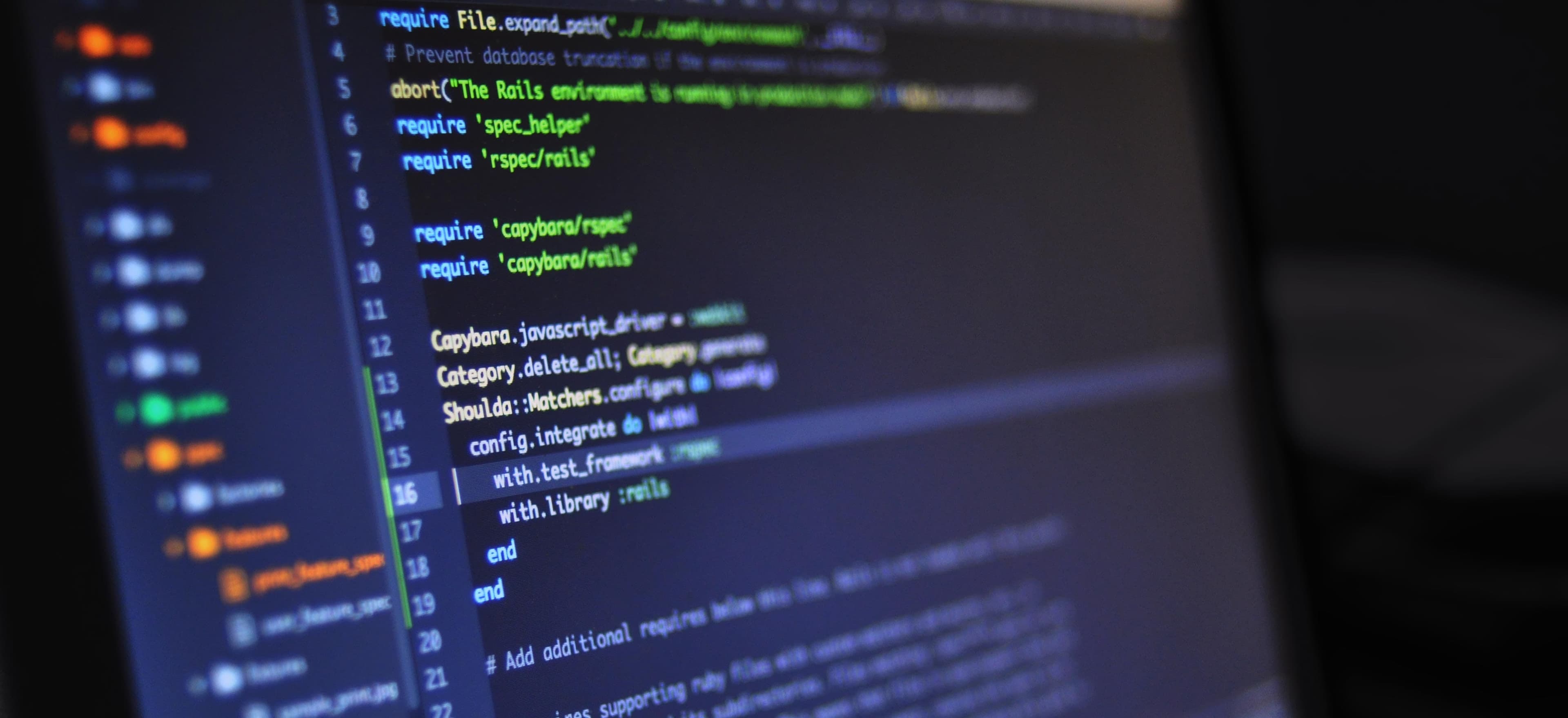
- Published on
Mastering Template Decorators in Spring Boot with Thymeleaf
In the landscape of modern web development, application frameworks streamline backend processes while ensuring a responsive user experience. One such powerful combination is Spring Boot with Thymeleaf. These tools together not only facilitate the rapid development of Java applications but also provide a meaningful way to build engaging user interfaces.
In this blog post, we will delve into Template Decorators in Thymeleaf. Template decorators allow developers to define a consistent layout for web pages while simplifying the maintenance of UI elements. Let’s explore how to effectively implement this technique within a Spring Boot application.
What Are Template Decorators?
Template decorators in Thymeleaf allow you to create a reusable layout structure. Think of them as an essential blueprint for rendering common elements across multiple pages. Using template decorators ensures that you maintain a unified design. For example, the header, footer, and navigation menus can be applied across different pages without redundant code.
You achieve this through a parent-child template relationship, allowing for a clear, concise separation of common UI components and page-specific content.
Setting Up Your Spring Boot Application
-
Start a New Spring Boot Project: You can use Spring Initializr to create a new Spring Boot application. Make sure to add the Thymeleaf dependency.
-
Add Dependencies in
pom.xml
: If you're using Maven, yourpom.xml
should include the necessary Thymeleaf starter. Here’s a snippet:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
- Creating Your Application Structure: Maintain a clear layout by organizing your resources. A common structure would look like:
src/
├── main/
│ ├── java/
│ │ └── com/
│ │ └── example/
│ │ └── demo/
│ │ └── DemoApplication.java
│ └── resources/
│ ├── static/
│ ├── templates/
│ └── application.properties
Creating the Base Layout
Let's create a base layout that all pages will inherit from. This will include a header, footer, and a content block where specific pages will inject their content.
Base Layout (base.html)
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title th:text="${title} + ' - My Application'">My Application</title>
<link rel="stylesheet" type="text/css" th:href="@{/css/styles.css}">
</head>
<body>
<header>
<h1>Welcome to My Application</h1>
<nav>
<ul>
<li><a th:href="@{/}">Home</a></li>
<li><a th:href="@{/about}">About</a></li>
<li><a th:href="@{/contact}">Contact</a></li>
</ul>
</nav>
</header>
<div th:insert="~{content :: content}"></div>
<footer>
<p>© 2023 My Application - All Rights Reserved</p>
</footer>
</body>
</html>
Commentary on Base Layout
- Base Structure: This is our template that other pages will extend. The header and footer are consistent throughout the site, reducing redundancy.
- Dynamic Page Title: The title is dynamic, enabling specific page titles to be injected through
th:text
. - Content Insertion: The
th:insert
directive specifies where to render content defined in child templates.
Creating Child Templates
Now, let's create a specific page that inherits from our base template. This page will utilize the content
fragment defined in base.html
.
Home Page (home.html)
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title th:text="'Home'">Home</title>
<link rel="stylesheet" type="text/css" th:href="@{/css/styles.css}">
</head>
<body th:replace="~{base :: layout}">
<div th:fragment="content">
<h2>Home Page</h2>
<p>Welcome to the home page of My Application.</p>
</div>
</body>
</html>
Commentary on Home Page
- Inherit Layout: We use
th:replace
to load thebase
layout. This abstracts away common elements. - Fragment Specification: The content is defined in a fragment named
content
, making it reusable across other templates.
Creating a Controller to Serve the View
We need to set up a Spring Boot controller to serve our newly created templates.
package com.example.demo.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HomeController {
@GetMapping("/")
public String home(Model model) {
model.addAttribute("title", "Home");
return "home";
}
}
Commentary on the Controller
- Spring Annotations: The
@Controller
annotation defines the class as a web controller. The@GetMapping
specifies the route it handles (i.e., the root URL). - Model Interaction: The
Model
object is used for passing data (in this case, the title). - View Resolution: The return statement directs the application to render the
home.html
Thymeleaf template.
Render and Test Your Application
Run your Spring Boot application and navigate to http://localhost:8080/ to see the home page rendered with the base layout. Test additional routes, such as /about
and /contact
, by creating corresponding templates and updating the HomeController
.
Final Thoughts
Using template decorators in Thymeleaf provides a structured way to build a maintainable, clean, and responsive frontend in your Spring Boot applications. The reusable layouts reduce development time and allow for simpler updates throughout your application.
To further enhance your knowledge, refer to the Thymeleaf Documentation and explore more about Spring Boot best practices on Baeldung.
By mastering template decorators, you create a solid foundation for future projects, enabling you to focus more on functionality and less on template redundancy. Enjoy your coding journey!
Checkout our other articles