Boosting Software Quality Through Better Object Cohesion
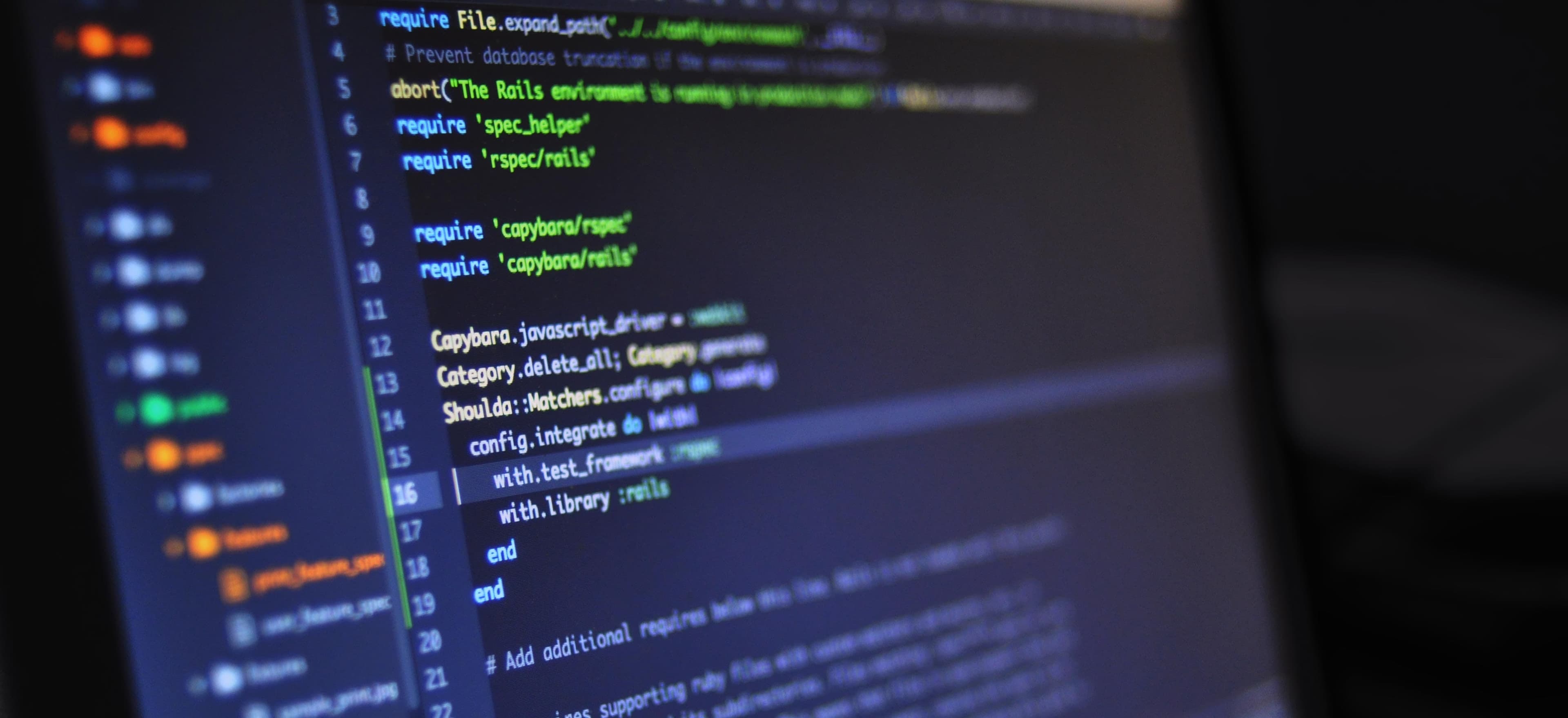
- Published on
Boosting Software Quality Through Better Object Cohesion
In the world of software development, ensuring high-quality code is not just a goal, but a necessity. One of the critical concepts that significantly affect code quality is object cohesion. But what exactly is object cohesion, and how can improving it enhance the overall quality of your software? In this post, we’ll delve deep into this concept, providing clarity on its importance, discussing strategies for improvement, and illustrating examples throughout your journey toward better software quality.
Understanding Object Cohesion
Object cohesion refers to the degree to which the elements within a class or module are related to one another. A highly cohesive class contains methods and properties that closely relate and serve a single purpose. In contrast, a low-cohesion class tends to scatter various distinct processes, reducing clarity and maintainability.
Why is Cohesion Important?
-
Maintainability: High cohesion facilitates easier updates and debugging. When related functionality is centralized, making changes requires minimal modifications elsewhere.
-
Reusability: Cohesive modules are easier to reuse in other projects or components, efficiently promoting best practices in software design.
-
Readability: Code structures that exhibit high cohesion promote understanding. Developers can grasp a cohesive class's purpose more intuitively than a disjointed one.
-
Testability: Cohesive classes are significantly easier to test. When functionalities are grouped logically, writing automated tests becomes more straightforward.
Types of Cohesion
Understanding the different types of cohesion can assist you in evaluating and improving your code’s structure. Here’s an overview:
-
Functional Cohesion: Every element is essential for a single function. This is the highest level of cohesion and should be the goal.
-
Sequential Cohesion: Elements are related such that one triggers the execution of another.
-
Communicational Cohesion: Elements share the same data or information.
-
Procedural Cohesion: Elements are grouped because they always follow a specific sequence of execution.
-
Temporal Cohesion: Elements are related by being executed at the same time.
-
Logical Cohesion: Elements are grouped because they are related logically, but the interaction is weak.
-
Coincidental Cohesion: This is the worst level where elements are grouped arbitrarily, with no clear relationship.
Example of Cohesion in Code
Let’s consider a basic example in Java to illustrate cohesive and non-cohesive classes.
// Low Cohesion Example
public class Utility {
public void printReport() {
// Code to print reports
}
public void connectToDatabase() {
// Code to connect to a database
}
public void sendEmail() {
// Code to send an email
}
}
In the above example, the Utility
class has low cohesion. It performs unrelated tasks: printing reports, connecting to a database, and sending emails.
Now, let's refactor this into a more cohesive structure:
public class ReportPrinter {
public void printReport(Report report) {
// Code to print the report
}
}
public class DatabaseConnector {
public void connect() {
// Code to connect to a database
}
}
public class EmailSender {
public void sendEmail(String emailAddress, Report report) {
// Code to send the report via email
}
}
Here, we have segmented the functionalities into separate classes. Each class has a single responsibility, leading to high cohesion within each class.
Strategies for Improving Cohesion
As developers, we can adopt various strategies to enhance object cohesion in our software design:
1. Follow the Single Responsibility Principle (SRP)
SRP stipulates that a class should have one and only one reason to change. By adhering to this principle, you naturally align the cohesion of your classes. Each class should encapsulate a single purpose, reducing the risk of unintended side effects during changes.
2. Use Meaningful Names
Names are powerful. Class and method names should genuinely reflect their functionality. This clarity encourages logical grouping and improves cohesion.
3. Refactor Regularly
Refactoring is essential to maintaining code quality. Regularly examine your codebase for low-cohesion classes and refactor them into cohesive units.
4. Modularize Your Functions
Break down complex methods into smaller, focused functions. This practice will ensure that each function does only one task and does it well, enhancing overall cohesion.
5. Leverage Design Patterns
Design patterns can help create standards and structures that encourage cohesive designs. Patterns like the Strategy, Composite, or Observer can aid in effective object grouping.
6. Code Reviews
Engage in code reviews with your peers. They can provide insights into cohesion that might not be apparent to you, offering time to discuss and improve your design choices.
7. Automated Testing
Implement unit tests to ensure that each class behaves as it should. This practice will help maintain high cohesion, as it requires each part of the code to fulfill a specific role effectively.
// Example of Unit Test for Cohesive Class
import org.junit.Test;
import static org.junit.Assert.*;
public class ReportPrinterTest {
@Test
public void testPrintReport() {
Report report = new Report("Test Title", "Some content");
ReportPrinter printer = new ReportPrinter();
String result = printer.printReport(report);
assertEquals("Expected output message", result);
}
}
In this test, we are ensuring that our ReportPrinter
class works as expected. This forms a solid verification that each class is cohesive and achieves its intended functionality.
To Wrap Things Up
Improving object cohesion is an essential step in boosting software quality. By establishing cohesive classes, you enhance maintainability, testability, and readability. This leads to more robust designs and ultimately better software products.
Incorporating strategies like following the Single Responsibility Principle, modularizing functions, and leveraging design patterns will set you on a path toward creating high-quality software. Take the time to refactor regularly and engage with your peers in meaningful discussions about design decisions.
For more insights into software design principles, you might find these resources helpful:
- Martin Fowler's Refactoring
- Clean Code by Robert C. Martin
By understanding and applying the concept of cohesion, you can unlock a powerful tool for improving your development process and the software you produce. Start today by analyzing your existing code for cohesion, and see how it can transform the quality of your projects. Happy coding!