Understanding Common Java 8 Date API Pitfalls
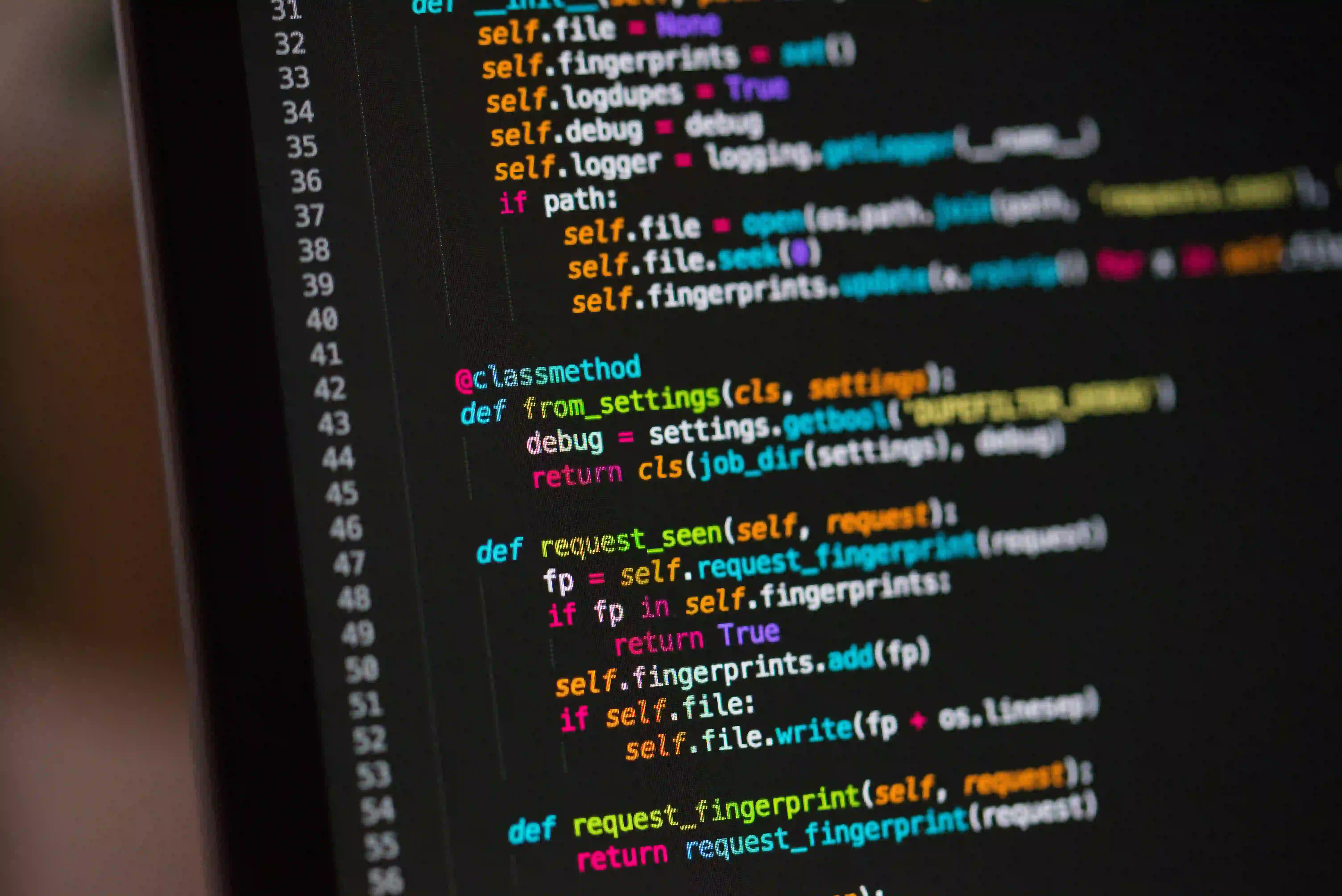
Understanding Common Java 8 Date API Pitfalls
Java 8 introduced a new Date and Time API for handling dates and times more effectively. While this API aimed to overcome the limitations of the older java.util.Date
and java.util.Calendar
classes, there are still common pitfalls that developers need to be aware of when working with it. In this post, we will explore these pitfalls in depth and provide code snippets to illustrate the challenges and solutions.
Why the New Date API?
Before diving into the common pitfalls, let's understand why Java 8 introduced a new Date API. The motivation behind this change was to provide:
- Immutability: The new classes are immutable, leading to safer code.
- Thread Safety: The immutability guarantees that they are inherently thread-safe.
- Fluent API: The new API provides a more fluent and easy-to-read API with natural language constructs.
Common Pitfalls in Java 8 Date API
1. Confusion Between LocalDate, LocalTime, and LocalDateTime
Many developers struggle with the distinction between LocalDate
, LocalTime
, and LocalDateTime
. Each serves a unique purpose:
LocalDate
: Represents a date (year-month-day) without a time zone.LocalTime
: Represents a time (hour-minute-second) without a date or time zone.LocalDateTime
: CombinesLocalDate
andLocalTime
, but still does not contain timezone information.
Code Snippet:
import java.time.LocalDate;
import java.time.LocalTime;
import java.time.LocalDateTime;
public class DateExample {
public static void main(String[] args) {
LocalDate date = LocalDate.now(); // Today's date
LocalTime time = LocalTime.now(); // Current time
LocalDateTime dateTime = LocalDateTime.now(); // Current date and time
System.out.println("Date: " + date);
System.out.println("Time: " + time);
System.out.println("Date and Time: " + dateTime);
}
}
Why This Matters: Using the appropriate class is critical. Misusing them can lead to errors in date calculations and formatting, which can impact your application logic.
2. Forgetting Time Zones
One of the major pitfalls is not considering the time zone when using date-time objects. Java 8 introduced classes like ZonedDateTime
and OffsetDateTime
, which incorporate time zones. Using LocalDateTime
can lead to confusion, especially when working with users in different regions.
Code Snippet:
import java.time.ZonedDateTime;
import java.time.ZoneId;
public class TimeZoneExample {
public static void main(String[] args) {
ZonedDateTime zonedDateTime = ZonedDateTime.now(ZoneId.of("America/New_York"));
System.out.println("Current Time in New York: " + zonedDateTime);
}
}
Why This Matters: If you don't account for time zones, you risk misaligning data across different regions. This can result in unexpected behaviors and errors.
3. Misusing Formatting and Parsing
Formatting and parsing dates might seem straightforward, but beginners often misinterpret how to use the DateTimeFormatter
. Incorrect patterns or assumptions could lead to runtime exceptions.
Code Snippet:
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class FormattingExample {
public static void main(String[] args) {
LocalDate date = LocalDate.of(2023, 10, 11);
// Using ISO_DATE formatter
String formattedDate = date.format(DateTimeFormatter.ISO_DATE);
System.out.println("Formatted Date: " + formattedDate);
// Custom formatting
DateTimeFormatter customFormatter = DateTimeFormatter.ofPattern("dd-MM-yyyy");
String customFormattedDate = date.format(customFormatter);
System.out.println("Custom Formatted Date: " + customFormattedDate);
// Parsing a date string
LocalDate parsedDate = LocalDate.parse("11-10-2023", customFormatter);
System.out.println("Parsed Date: " + parsedDate);
}
}
Why This Matters: Misformatted dates are a common source of bugs. Always ensure that the format you are parsing matches the string representation.
4. Creating Dates from Invalid Values
It’s vital to validate the values used to create date instances. For example, using February 30th, or asking for the 25th day of a month that only has 30 days will lead to runtime exceptions.
Code Snippet:
import java.time.LocalDate;
public class InvalidDateExample {
public static void main(String[] args) {
// This will throw an exception
try {
LocalDate invalidDate = LocalDate.of(2023, 2, 30);
} catch (Exception e) {
System.out.println("Caught exception: " + e.getMessage());
}
}
}
Why This Matters: Creating invalid dates can lead to crashes or erratic behavior in applications. Always handle potential exceptions properly.
5. Not Leveraging Temporal Adjusters
Java 8 provides a powerful concept known as Temporal Adjusters, which allow you to create complex date calculations with ease. However, many developers overlook this feature, opting instead for manual calculations.
Code Snippet:
import java.time.LocalDate;
import java.time.temporal.TemporalAdjusters;
public class TemporalAdjusterExample {
public static void main(String[] args) {
LocalDate today = LocalDate.now();
LocalDate nextMonday = today.with(TemporalAdjusters.next(java.time.DayOfWeek.MONDAY));
System.out.println("Today: " + today);
System.out.println("Next Monday: " + nextMonday);
}
}
Why This Matters: Leveraging Temporal Adjusters not only increases the readability of your code but also ensures that complex date calculations are handled correctly and efficiently.
Final Considerations
Java 8's Date and Time API fundamentally changes how developers manage dates and times in Java applications. However, pitfalls such as confusion between classes, neglecting time zones, misusing formatting, invalid date values, and underutilizing Temporal Adjusters can undermine its effectiveness.
To strengthen your understanding and usage of the Java Date API, consider reading Oracle's Java Documentation or exploring innovative Java programming techniques on Baeldung.
Embrace the new API and remember to stay vigilant against these common pitfalls to write reliable and cleaner Java date manipulation code. Happy coding!