Common Set-Up Issues for Android Wear SDK: Fixing Hello World
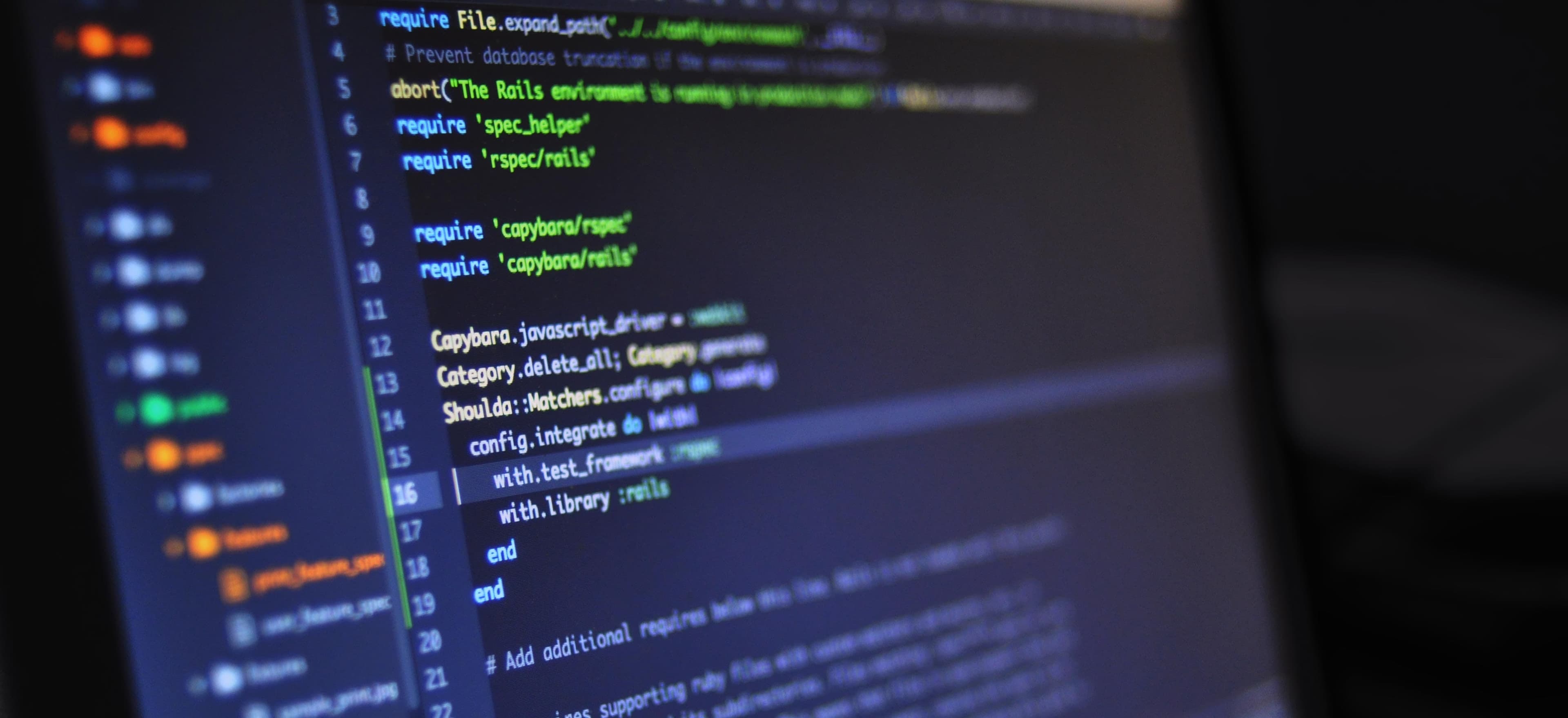
- Published on
Common Set-Up Issues for Android Wear SDK: Fixing Hello World
Creating your first Android Wear app can be an exciting yet frustrating experience. The Android Wear SDK simplifies the process of developing apps for wearable devices, but as any developer will tell you, set-up issues can easily derail your project. This post will guide you through some common set-up issues when creating your own Android Wear “Hello World” app, and how to fix them in a straightforward manner.
Understanding the Android Wear SDK
Before diving into the issues, let’s briefly outline what the Android Wear SDK is. It is a set of libraries and tools that facilitate the development of applications for wearable devices running on the Android OS. It extends Android's features to make them compatible with small and touch-based interfaces of wearables.
The first step after installing Android Studio is to begin creating your first app. However, many developers encounter issues during this process.
Common Issues and Fixes
1. Incorrect SDK Setup
If your Android Studio can’t find the required SDK tools, you may face issues during your app creation.
Solution: Install the Required SDK Tools
- Open Android Studio, go to File -> Settings -> Appearance & Behavior -> System Settings -> Android SDK.
- Ensure that you have installed the latest version of the Android SDK and the Android SDK Build-Tools.
- Make sure to install the Wear OS by Google app option to ensure that you have all necessary components.
2. Emulator Challenges
Often, the emulator fails to start, or it doesn't display your app correctly.
Solution: Configure the Emulator Properly
- Create a new Virtual Device (AVD) with Wear OS specifications. Go to Tools -> AVD Manager and click on Create Virtual Device.
- Select a Wear OS device profile compatible with your app target.
- Ensure you have enough memory allocated for the emulator and that HAXM is installed for better performance.
3. Gradle Build Issues
One of the most frequent setbacks can stem from Gradle sync problems. These can arise from outdated dependencies or misconfiguration.
Solution: Update Dependencies
Ensure that your build.gradle
files are properly configured. Here is an example of what your module build.gradle
file could look like:
apply plugin: 'com.android.application'
android {
compileSdkVersion 30
defaultConfig {
applicationId "com.example.hworld"
minSdkVersion 23
targetSdkVersion 30
versionCode 1
versionName "1.0"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
}
dependencies {
implementation 'com.google.android.gms:play-services-wearable:17.0.0'
implementation 'androidx.wear.widget:wear:1.1.0'
}
This code snippet ensures that you are using the latest libraries compatible with wearables. Update the versions as needed based on what you find in the Android Developer documentation.
4. Manifest Configuration
Sometimes the AndroidManifest.xml file is not configured correctly, leading to application crashes or unexpected behavior.
Solution: Adjust Your Manifest File
Make sure your AndroidManifest.xml file contains the correct permissions and settings for wearables:
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.hworld">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
This file defines the main activity, and it’s crucial for app visibility on the device. Furthermore, ensure your app has the necessary permissions for sensors, internet, and other features you intend to use.
5. Device Sync Issues
If you're using a physical device, apps may not sync correctly from Android Studio to your wearable.
Solution: Check Connectivity
Make sure that your wearable and mobile device are connected via Bluetooth. You may need to close and reopen your mobile app to re-establish the connection, allowing Android Studio to deploy your app correctly.
6. Testing Your App
Finally, after resolving the above issues, you may still encounter difficulties running your "Hello World" application.
Solution: Use Logcat for Debugging
Use Android's Logcat tool to view real-time logs from your device which can provide insight into any issues during execution.
You can start testing by adding Log statements within your MainActivity.java
like so:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Log.d("HelloWorld", "App is up and running!");
}
This piece of code allows you to debug whether the onCreate method is being executed correctly.
Key Takeaways
Setting up the Android Wear SDK may seem daunting at first, but understanding common issues can save you considerable time and effort. From SDK installation and emulator configuration to Gradle and Manifest setups, each step is critical to your app’s successful launch.
If you want to delve deeper into the Android Wear SDK, check the official resources from the Android Developers site for additional tutorials and documentation.
Don’t let initial roadblocks deter you from creating innovative wearable applications. Address these set-up issues with confidence, and you are one step closer to your first Android Wear app. Happy coding!
Checkout our other articles