Troubleshooting Common Issues with Google Cloud Function Gradle Plugin
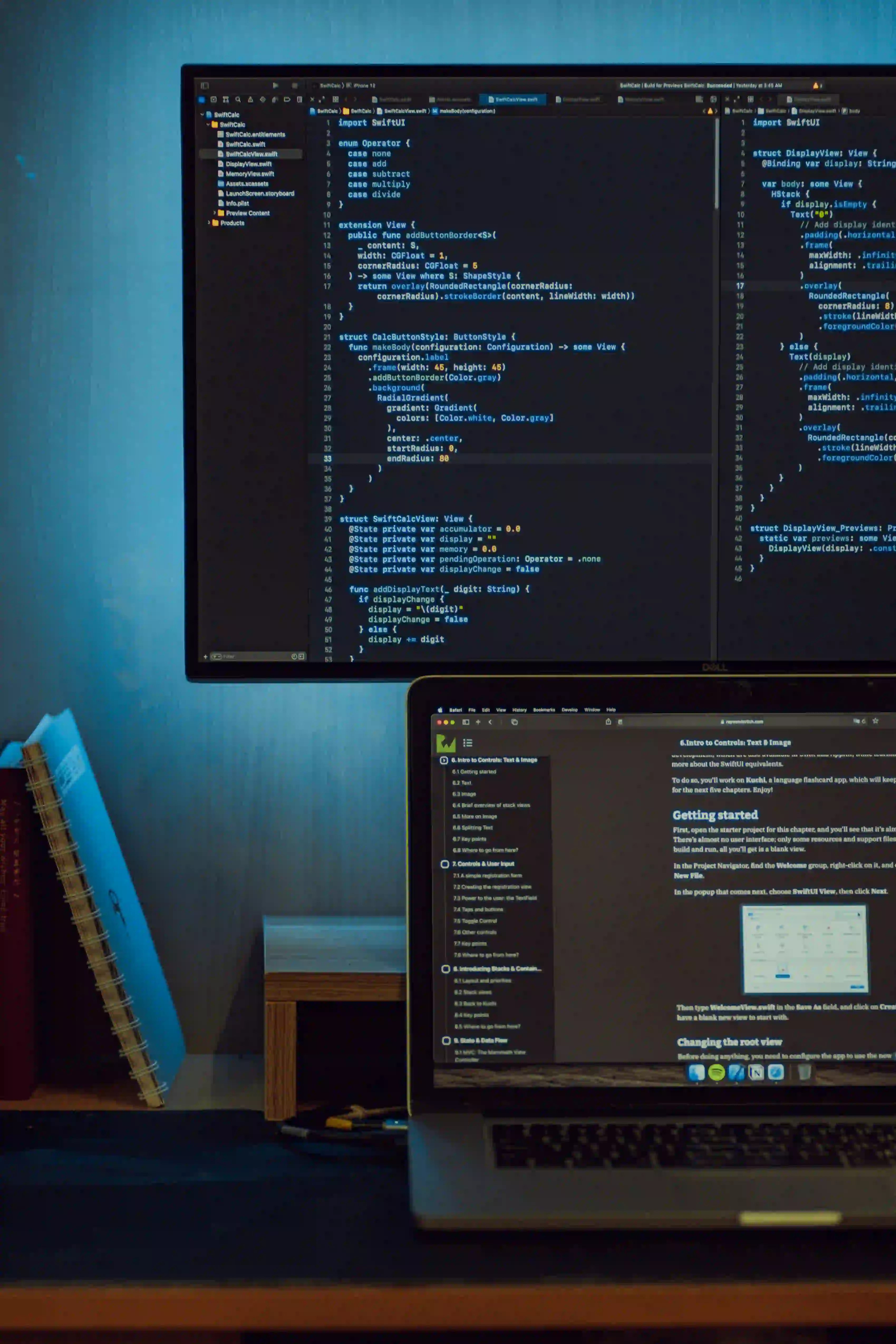
Troubleshooting Common Issues with Google Cloud Function Gradle Plugin
Google Cloud Functions provides a serverless environment that allows users to run code without provisioning or managing servers. The Google Cloud Function Gradle Plugin is a powerful tool that simplifies the deployment process for Java-based functions. This post will focus on troubleshooting common issues that developers may encounter while using this plugin. By the end, you'll have a better understanding of how to solve these problems efficiently.
What is the Google Cloud Function Gradle Plugin?
Before we dive into troubleshooting, let's briefly outline what the Google Cloud Function Gradle Plugin is and why it is widely used.
The plugin allows developers to build and deploy Google Cloud Functions directly from a Gradle build script. It streamlines the deployment process and integrates seamlessly with existing Gradle-based Java projects.
You can learn more about the plugin here.
Setting Up Your Environment
First, make sure you have your environment set up correctly. Your build.gradle
file should contain the necessary dependencies. Here's an exemplary code snippet showcasing how to configure the plugin:
plugins {
id 'java'
id 'com.google.cloud.functions' version '1.0.0'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'com.google.cloud.functions:functions-framework-api:1.0.0'
}
functions {
function("myFunction") {
runtime = 'java11'
entryPoint = 'com.example.MyFunction'
sourceArchive = file('build/libs/myfunction-1.0.0.jar')
}
}
Explanation of Key Components
- id 'com.google.cloud.functions': This applies the Google Cloud Functions plugin.
- entryPoint: This defines the fully qualified name of your cloud function class.
- sourceArchive: This points to the JAR file that contains your function code.
Common Issues and How to Troubleshoot
Here are some common issues that developers face when using the Google Cloud Function Gradle Plugin, along with strategies for troubleshooting them.
1. Plugin Compatibility Issues
Problem: The version of the Gradle plugin may not be compatible with your project.
Solution: Always ensure that your Gradle version is compatible with the plugin version you are using. Check the Gradle release notes to see if there are any compatibility changes.
If your Gradle version is outdated, you can upgrade it using:
./gradlew wrapper --gradle-version <latest-version>
2. HTTP Status Code Errors
Problem: When you deploy your function, you might encounter HTTP status code errors such as 400 or 404.
Solution:
-
400 Bad Request: This often occurs if the function's entry point is incorrectly specified. Double-check your entry point in the
build.gradle
, ensuring that it matches the fully qualified class name. -
404 Not Found: This error often indicates that the deployment did not succeed or the function does not exist. Use the command below to list your deployed functions:
gcloud functions list
3. Dependency Issues
Problem: Missing or conflicting dependencies in your project can lead to deployment failures.
Solution: Ensure that all your dependencies are correctly listed in the dependencies
block of your build.gradle
file. Consider using the ./gradlew dependencies
command to visualize the dependency tree and identify any conflicts.
./gradlew dependencies
Example of Resolving Dependency Issues
If you find that your application needs an additional library, you can add it like this:
dependencies {
implementation 'org.slf4j:slf4j-api:1.7.30'
implementation 'com.google.cloud:google-cloud-storage:1.113.15'
}
4. Authentication Issues
Problem: Authentication failures when deploying or invoking a function.
Solution: Make sure that your Google Cloud SDK is authenticated. Run the following command:
gcloud auth login
Also, ensure that your project is set correctly with:
gcloud config set project <your-project-id>
5. Execution Timeouts
Problem: Your function may timeout due to insufficient memory or timeout settings.
Solution: You can extend the timeout and memory in your build.gradle
as follows:
functions {
function("myFunction") {
runtime = 'java11'
entryPoint = 'com.example.MyFunction'
timeout = '60s' // Set timeout to 60 seconds
availableMemoryMb = 256 // Set memory to 256 MB
}
}
6. Debugging Logs
Problem: You want to see logs for debugging purposes, but donβt know where to find them.
Solution: Google Cloud functions logs can be viewed in the Google Cloud Console under Logging. You can also view logs from the command line using:
gcloud functions logs read myFunction
7. Deployment Errors
Problem: Sometimes, deployment may fail with messages that are not very clear.
Solution: Use the verbose flag during deployment to get more insight:
./gradlew deploy -Pverbose=true
This will provide detailed output which can help in identifying the root cause of the issue.
Closing the Chapter
Troubleshooting issues with the Google Cloud Function Gradle Plugin can be challenging. However, knowing the common issues and their solutions can save you a lot of time and effort.
Further Reading
By following the strategies outlined in this post, you should be well-equipped to tackle the majority of issues that arise when using the Google Cloud Function Gradle Plugin. Happy coding!