Troubleshooting Common JSF 2 and PrimeFaces Integration Issues
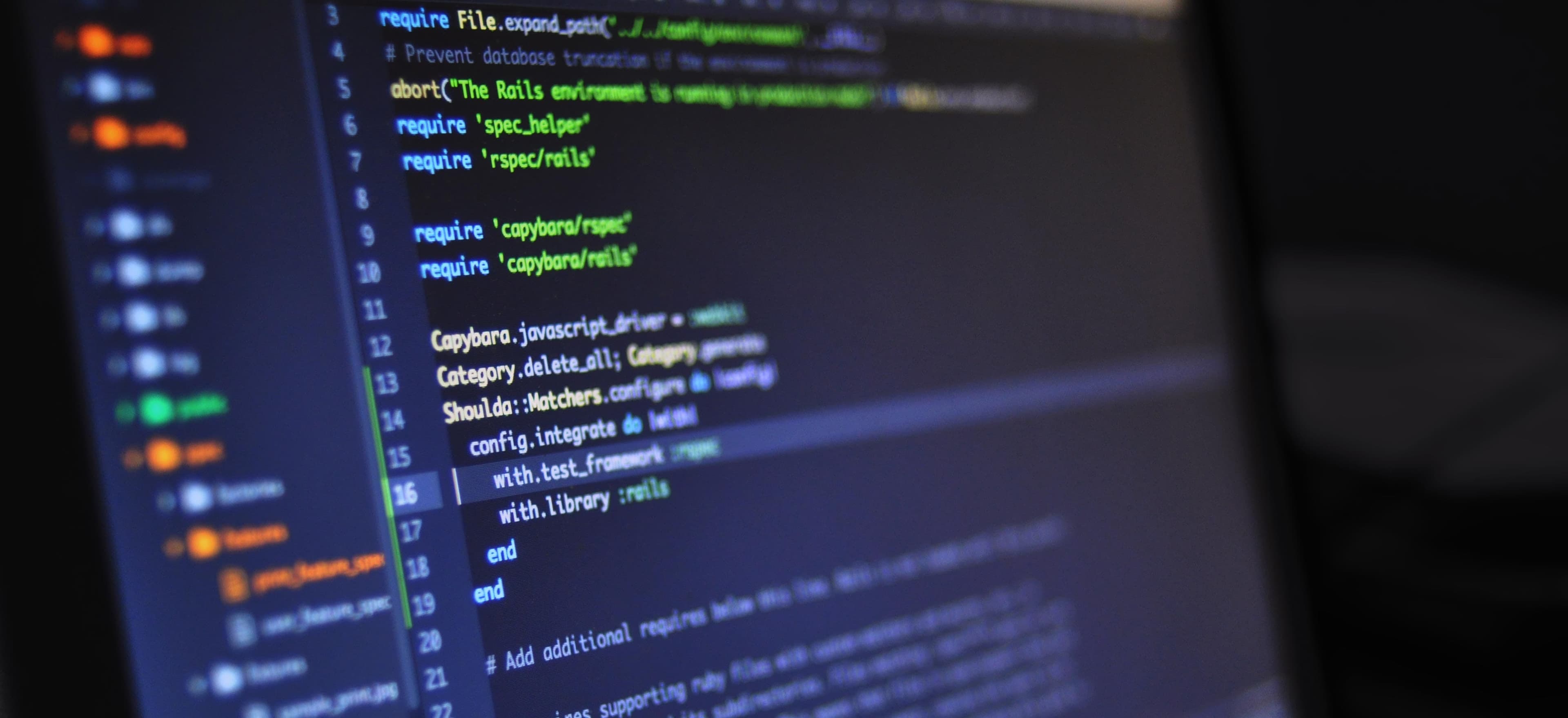
- Published on
Troubleshooting Common JSF 2 and PrimeFaces Integration Issues
JavaServer Faces (JSF) is a robust, component-based web framework for building user interfaces for Java server applications. PrimeFaces, on the other hand, provides a rich set of UI components that enhances the capabilities of JSF. While integrating JSF 2 with PrimeFaces can lead to creating dynamic and interactive applications, developers often face challenges during this process. This blog post will explore the common issues encountered during the integration of JSF 2 and PrimeFaces, along with their solutions.
Table of Contents
- Understanding JSF and PrimeFaces
- Common Integration Issues
- 2.1 Dependencies and Configuration Issues
- 2.2 View Handling Problems
- 2.3 AJAX Issues
- 2.4 Component Rendering Errors
- Debugging Techniques
- Conclusion
1. Understanding JSF and PrimeFaces
JSF is a component-based web application framework, similar to MVC (Model-View-Controller) architecture, which allows developers to create user interfaces using reusable UI components. PrimeFaces adds to this framework by providing a suite of advanced UI components, rich user interface features such as charts, dialog boxes, and forms.
Before we delve into troubleshooting, it's essential to ensure that the basic configuration is correct. A basic Maven dependency for JSF and PrimeFaces would look like this:
<dependency>
<groupId>org.glassfish</groupId>
<artifactId>javax.faces</artifactId>
<version>2.3.9</version>
</dependency>
<dependency>
<groupId>org.primefaces</groupId>
<artifactId>primefaces</artifactId>
<version>10.0.0</version>
</dependency>
Make sure you use compatible versions. Refer to the PrimeFaces documentation for detailed version compatibility information.
2. Common Integration Issues
2.1 Dependencies and Configuration Issues
Issue: Upon starting the application, you receive ClassNotFound exceptions for JSF or PrimeFaces classes.
Solution:
Check your pom.xml
for the correct dependencies and version compatibility. Ensure that you also have the necessary JSF implementation, such as Mojarra or MyFaces. Here is an example of including Mojarra:
<dependency>
<groupId>com.sun.faces</groupId>
<artifactId>jsf-api</artifactId>
<version>2.3.9</version>
</dependency>
In your web.xml
, ensure that the servlet mappings are defined correctly:
<servlet>
<servlet-name>Faces Servlet</servlet-name>
<servlet-class>javax.faces.webapp.FacesServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>Faces Servlet</servlet-name>
<url-pattern>*.xhtml</url-pattern>
</servlet-mapping>
2.2 View Handling Problems
Issue: FacesContext cannot find the view or throws a 404 error on trying to load an xhtml page.
Solution:
Verify that your .xhtml
files are located in the correct directory and accessible by the web server. The standard locations are typically /src/main/webapp/
for Maven projects. Ensure that the facelet view handler is configured in web.xml
:
<context-param>
<param-name>javax.faces.FACELETS_APPLICATION_MAPS</param-name>
<param-value>faces</param-value>
</context-param>
2.3 AJAX Issues
Issue: AJAX calls are not functioning as expected, and components do not update on the page.
Solution:
Make sure that the update
attribute in your AJAX call is targeting the correct components. For example:
<p:commandButton value="Submit" action="#{bean.submit}" update="messages" />
<p:messages id="messages" />
If the component with the specified ID does not exist, the framework will not update it. Additionally, enable partialSubmit
if you want to submit only some values from the form.
2.4 Component Rendering Errors
Issue: Some PrimeFaces components render incorrectly or do not display at all.
Solution:
Ensure that you have included the necessary CSS and JavaScript files in your view. For example, if you are using a PrimeFaces component, your <h:head>
section should resemble:
<h:head>
<title>My Application</title>
<h:outputStylesheet name="primefaces.css" />
<h:outputScript name="primefaces.js" />
</h:head>
Additionally, check for any console errors in the web browser. JavaScript errors may disrupt the rendering of components.
3. Debugging Techniques
Debugging is crucial when troubleshooting issues in JSF and PrimeFaces applications. Here are some techniques:
-
Logging: Utilize a logging framework like SLF4J with Logback to capture logs. Set the logging level to DEBUG to track down any specific issues.
-
Developer Tools: Use the browser's developer tools (usually accessible through F12). Check the console for errors and the network tab to verify AJAX request/response.
-
JSF Lifecycle: Familiarize yourself with the JSF lifecycle phases (Restore View, Apply Request Values, Process Events, etc.). This understanding can help you identify where the issue may be occurring.
4. Conclusion
Integrating JSF 2 with PrimeFaces can significantly enhance your Java web applications. However, like any technology stack, challenges can arise. By understanding common issues related to dependencies, configuration, AJAX functionality, and component rendering, you can resolve these problems efficiently.
Don't forget to follow best practices for dependency management and ensure you are using the right versions of each library. For more advanced topics, consider exploring Java EE 8 and keeping your project updated.
By following this guide, you'll be well-equipped to troubleshoot common JSF and PrimeFaces integration issues and ensure a smoother development experience. Happy coding!