Mastering the Red-Green Test Cycle for Better Code Quality
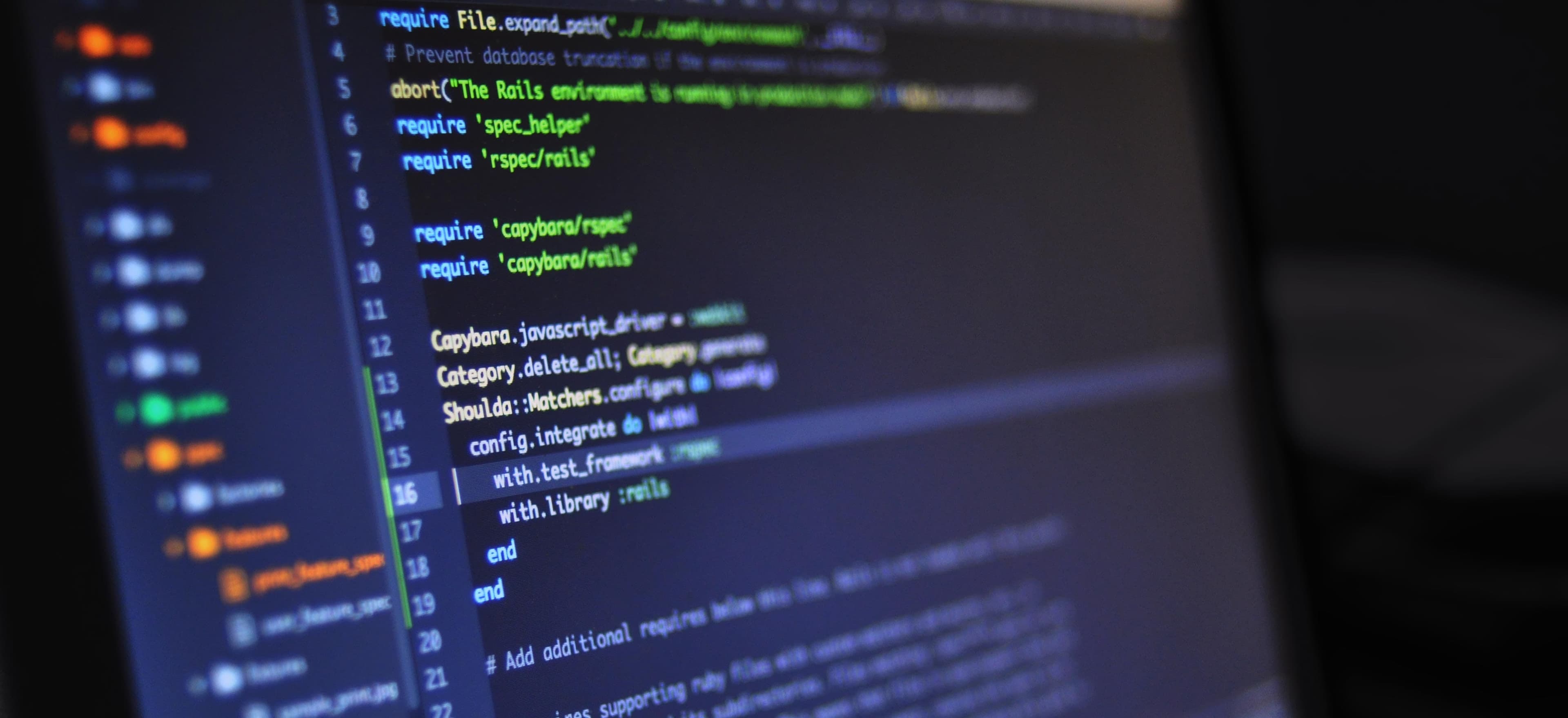
- Published on
Mastering the Red-Green Test Cycle for Better Code Quality
In the evolving landscape of software development, achieving high code quality is paramount. One robust methodology that stands out in this regard is the Red-Green Test Cycle, which lies at the heart of Test-Driven Development (TDD). TDD not only encourages the creation of clean, maintainable code but also fosters collaboration among teams. In this blog post, we will delve into the Red-Green Test Cycle, unravel its benefits, explore an example with Java code, and highlight best practices to get the most out of this methodology.
What is the Red-Green Test Cycle?
The Red-Green Test Cycle is a simple cycle that consists of three primary phases:
- Red Phase: Write a test for a new feature or function that currently does not exist. As no implementation exists, this test should fail.
- Green Phase: Write the simplest code to make the test pass. This does not mean you should implement the entire feature but rather enough to satisfy the test.
- Refactor Phase: Improve the code while ensuring that all tests still pass. This improves code quality without altering external behavior.
The Benefits of the Red-Green Test Cycle
Utilizing the Red-Green cycle provides several advantages:
- Enhanced Code Quality: It naturally leads to better-designed code. By writing tests first, developers think through the application’s requirements more thoroughly.
- Immediate Feedback: It minimizes the feedback loop. You know right away whether the implemented functionality is correct.
- Simplified Refactoring: With a robust suite of tests, refactoring becomes safer and easier, allowing for continuous improvement without fear of breaking existing functionality.
For a structured overview, consider the relationship between the phases:
- New features ==> Write a test (Red)
- Implement Minimal Code ==> Make it pass (Green)
- Improve the Implementation ==> Enhance quality (Refactor)
Implementing the Red-Green Test Cycle in Java
Let’s walk through an example of how to implement the Red-Green Test Cycle in Java. Suppose we are building a simple application that needs to calculate the area of a rectangle.
Step 1: Red Phase
First, write the test case for the Rectangle
class that calculates the area.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class RectangleTest {
@Test
public void testCalculateArea() {
Rectangle rectangle = new Rectangle(5, 10);
assertEquals(50, rectangle.calculateArea(), "The area should be 50");
}
}
Commentary
At this point, running this test will lead to a failure, as the Rectangle
class and its method calculateArea()
do not exist yet. The red color signifies that our implementation is incomplete.
Step 2: Green Phase
Next, implement the minimal code necessary to make the test pass.
public class Rectangle {
private final int width;
private final int height;
public Rectangle(int width, int height) {
this.width = width;
this.height = height;
}
public int calculateArea() {
return width * height;
}
}
Commentary
Here, we created the Rectangle
class with a constructor for width and height, and a method to calculate the area. Since we now have the requisite code in place, running the test should result in a successful pass.
Step 3: Refactor Phase
After attaining a passing test, it’s time to optimize our code, although in this case, the implementation is pretty straightforward. However, let's say we want to ensure that no negative dimensions are allowed.
public class Rectangle {
private final int width;
private final int height;
public Rectangle(int width, int height) {
if (width < 0 || height < 0) {
throw new IllegalArgumentException("Width and height must be non-negative");
}
this.width = width;
this.height = height;
}
public int calculateArea() {
return width * height;
}
}
Commentary
In this version, we added validation to the constructor. We throw an IllegalArgumentException
if negative dimensions are passed to ensure robust integrity of our Rectangle class.
Continuation of the Cycle
After refactoring, you can add more tests that check for edge cases or additional features, perpetuating the cycle. For extensive learning on TDD, you may refer to The Pragmatic Programmer for insights.
Best Practices for the Red-Green Test Cycle
- Keep Tests Small: Each test should ideally cover a single behavior. This helps isolate bugs efficiently.
- Write Descriptive Test Names: Always use clear and descriptive names for your tests, as it eases understanding and maintenance.
- Stay Consistent: Consistently follow the cycle for all features, ensuring you build a robust suite of tests over time.
- Prioritize Readability: Always write code that is easy to read, as this aids maintenance and collaboration amongst team members.
Lessons Learned
Mastering the Red-Green Test Cycle can significantly improve code quality in your Java applications. The process encourages disciplined coding practices and leads to cleaner, more maintainable code. Remember, the goal is not just to write tests but to write meaningful tests that drive your code's architecture.
As you incorporate this methodology in your workflows, you’ll notice more reliable applications and, ultimately, happier users. To further enhance your knowledge, explore resources on TDD and join communities that discuss these practices. Happy coding!