Uncovering Dependency Conflicts in Java Libraries
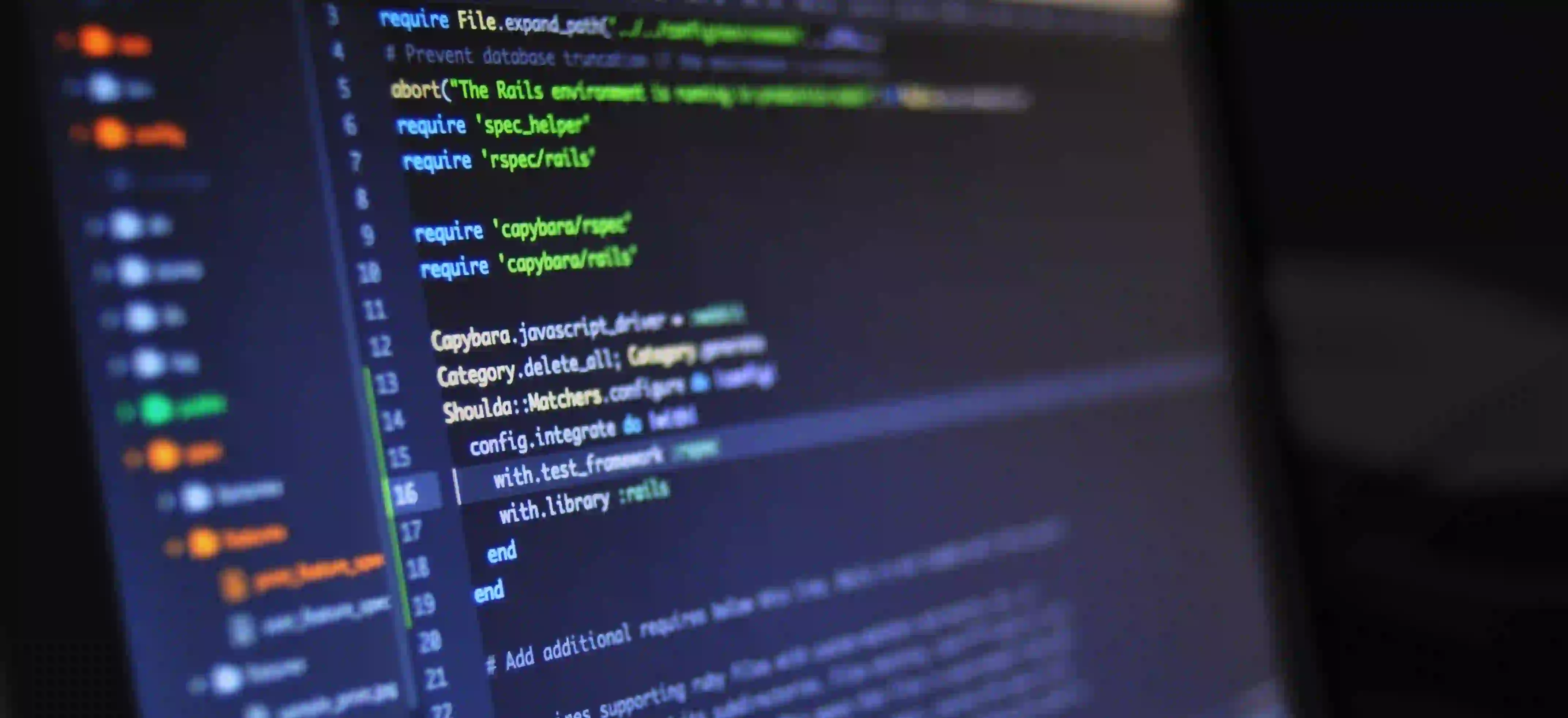
Uncovering Dependency Conflicts in Java Libraries
In today's software development landscape, managing dependencies is crucial. As project complexity increases, the likelihood of dependency conflicts also rises. This blog post will delve into dependency conflicts in Java libraries, examining their causes, impacts, and solutions, while also providing practical examples along the way.
What Are Dependency Conflicts?
Dependency conflicts occur when different libraries or modules within a project require incompatible versions of the same dependency. For instance, if Library A depends on library-X
version 1.0, and Library B depends on library-X
version 2.0, a conflict arises. This can lead to runtime errors, increased build times, or even application crashes.
Why Dependency Conflicts Matter
- Runtime Failures: Applications may fail to start or behave unpredictably if the right version of a dependency is not loaded.
- Hard-to-Debug Issues: Conflicts can lead to subtle bugs that might be challenging to trace back to the library version inconsistency.
- Increased Maintenance Costs: Managing dependencies takes time, and inefficient handling can add to the overall time taken to maintain a project.
Common Causes of Dependency Conflicts
-
Transitive Dependencies: When a library you use pulls in its own dependencies, these may conflict with other libraries in your project. Tools like Maven or Gradle manage this but can sometimes overlook conflicts.
-
Outdated Libraries: Using outdated versions of libraries might lead to conflicts with newer libraries attempting to utilize newer features or APIs.
-
Forked Libraries: Often, developers might fork libraries and modify them for their specific needs. If the original library updates, it might introduce conflicts when merging these changes back.
-
Multiple Module Projects: In multi-module projects, different modules might have dependencies on different versions of the same library, leading to clashes.
Detecting Dependency Conflicts
The first step in solving dependency conflicts is detecting them. Here's how you can identify dependency conflicts in Java projects.
Using Maven
If you're using Maven, you can execute the following command:
mvn dependency:tree
This command provides a detailed visualization of your project's dependencies, including their versions. Any conflicts will be highlighted here.
Using Gradle
For Gradle projects, you can run:
./gradlew dependencies
This will print out the dependency tree, making it easy to spot any version conflicts.
Example Code: Using Maven to Detect Conflicts
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>dependency-demo</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.10</version>
</dependency>
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>30.0-jre</version>
</dependency>
</dependencies>
</project>
The above Maven configuration includes dependencies on Commons Lang3 and Guava. Running the mvn dependency:tree
command would reveal any conflicts.
Resolving Dependency Conflicts
Once you identify the conflicts, it is time to resolve them. Here are several strategies:
1. Exclude Transitive Dependencies
You can exclude the conflicting versions of transitive dependencies directly from your pom.xml
(Maven) or build.gradle
(Gradle) file.
Maven Example
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>5.3.8</version>
<exclusions>
<exclusion>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
</exclusion>
</exclusions>
</dependency>
In this snippet, we are excluding commons-lang3
, which might have caused conflicts.
Gradle Example
implementation('org.springframework:spring-web:5.3.8') {
exclude group: 'org.apache.commons', module: 'commons-lang3'
}
2. Use Dependency Management
Maven provides a way to manage dependencies' versions globally using the <dependencyManagement>
section of your pom.xml
.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
</dependencies>
</dependencyManagement>
This approach centralizes the version management, reducing conflicts.
3. Upgrade or Downgrade Dependencies
Sometimes, the easiest way to resolve a conflict is to either upgrade or downgrade one of the conflicting dependencies. Regularly updating your libraries can help mitigate conflicts.
4. Utilize Dependency Resolution Strategies
In Gradle, you can define resolution strategies to force a particular version for dependencies.
configurations.all {
resolutionStrategy {
force 'org.apache.commons:commons-lang3:3.12.0'
}
}
Example: Handling Conflicts in a Real-world Scenario
Suppose you have a project with the following libraries: Library A and Library B.
Library A relies on commons-lang3:3.10
, and Library B relies on commons-lang3:3.12
.
If running mvn dependency:tree
shows a dependency conflict, you should:
- Identify the correct version you want to use.
- Apply the dependency exclusion as shown earlier.
- Execute tests to ensure no functionality is broken.
In Conclusion, Here is What Matters
Identifying and resolving dependency conflicts in Java libraries is a crucial skill for developers. Understanding how dependencies interact and affect each other can lead to more robust and maintainable code.
By employing the methods discussed in this blog post, you can minimize the impact of dependency conflicts and ensure that your Java projects run smoothly. Remember, tools like Maven and Gradle have powerful capabilities to aid in managing dependencies, so make the most of them.
For further reading on dependency management in Java Maven, check Spring's Official Documentation and for Gradle, consider visiting Gradle's Getting Started Guide.
By being proactive about dependency management, you can save yourself and your team a significant amount of time and frustration in your Java projects. Happy coding!