Streamlining InputStream to Stream Conversion in Java
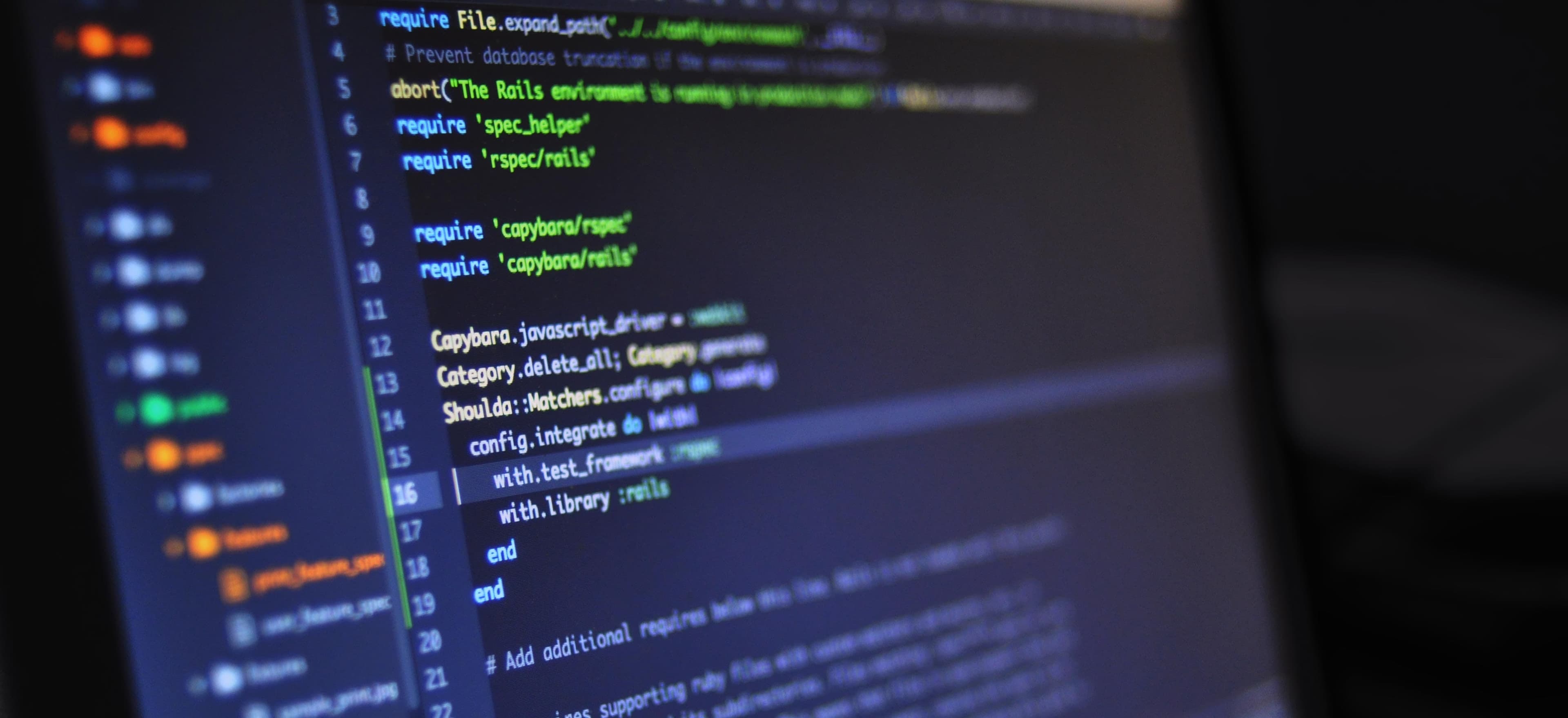
- Published on
Streamlining InputStream to Stream Conversion in Java
Handling streams in Java is a fundamental yet often overlooked aspect of data processing. Streams provide a powerful way to handle sequences of data, allowing for efficient and expressive operations. In this blog post, we will explore how to streamline the conversion between InputStream
and Java 8’s Stream
. This conversion is essential when you're dealing with I/O operations in a more functional programming style.
Understanding InputStream and Stream
What is InputStream?
InputStream
is an abstract class in Java representing an input stream of bytes. It provides a way to read data from different sources - it could be a file, a network socket, or another data source. Its primary method, read()
, reads the next byte of data.
Here’s a simple example:
InputStream inputStream = new FileInputStream("example.txt");
int data = inputStream.read();
while (data != -1) {
System.out.print((char) data);
data = inputStream.read();
}
inputStream.close();
This code snippet reads characters from a file until there are no more bytes to read. While convenient, this imperative approach can become cumbersome and less readable.
What is Stream?
Java’s Stream
API, introduced in Java 8, allows for functional-style operations on sequences of elements. It supports a sequence of operations that can be executed in a lazy manner.
The operations allow for powerful abstractions, such as filtering, mapping, and reducing, on the data structure. Here's a brief example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.stream()
.filter(name -> name.startsWith("A"))
.forEach(System.out::println);
This snippet filters names that start with "A" and prints them. The Stream
API provides cleaner and more expressive I/O operations. But how can we convert an InputStream
to a Stream
?
Converting InputStream to Stream
To convert an InputStream
into a Stream
, we need to read byte data and map it into elements we can work with. Below are methods to achieve efficient conversion.
Simple Method: BufferedReader and Lines
One of the most straightforward ways to convert an InputStream
to a Stream<String>
is using BufferedReader
. This method reads each line from the input stream and converts it into a stream of strings.
Here's how we can do it:
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.stream.Stream;
public Stream<String> inputStreamToStream(InputStream inputStream) {
return new BufferedReader(new InputStreamReader(inputStream)).lines();
}
Explanation:
-
BufferedReader: It reads text from a character-input stream, buffering characters for efficient reading. This is much more efficient than reading one byte at a time.
-
InputStreamReader: It bridges the byte streams to character streams, making it easier to deal with text data.
-
lines(): This method returns a stream of lines read from the buffered reader, lazily.
This stream operates lazily and only reads data when it's consumed. This is key for performance when dealing with large files.
Advanced Method: Using Files.lines()
If the source of the InputStream
is a file, we can also use Files.lines()
, which opens a file and returns a stream of its lines.
For example:
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.stream.Stream;
public Stream<String> fileToStream(String filePath) throws IOException {
Path path = Path.of(filePath);
return Files.lines(path);
}
However, it is crucial that the input stream is wrapped appropriately to ensure it can also handle more complex data sources.
Full Example: Using Both Methods
Let’s put it all together. Here’s a complete example that demonstrates how to read from an InputStream
, convert it into a stream, and perform some operations.
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.stream.Stream;
public class StreamConversion {
public Stream<String> inputStreamToStream(InputStream inputStream) {
// Convert InputStream to Stream<String>
return new BufferedReader(new InputStreamReader(inputStream)).lines();
}
public void processInputStream(InputStream inputStream) {
// Use the conversion to process data
try (Stream<String> stream = inputStreamToStream(inputStream)) {
stream
.filter(line -> line.contains("Java")) // Filter lines containing "Java"
.forEach(System.out::println); // Print each matching line
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why Stream Conversion Matters
By converting InputStream
into a Stream
, you're not just simplifying your code; you're also benefiting from Stream's features—the lazy evaluation, parallel processing, and functional programming constructs. This integration leads to more maintainable, readable, and efficient code.
Tips for Efficient Stream Handling
- Use try-with-resources: Like in our example, always manage resources properly to avoid memory leaks.
- Leverage parallel streams: If you're processing large datasets, consider using parallel streams for enhanced performance.
- Limit data processed: Use
limit(int maxSize)
for large streams to control memory usage. - Avoid side-effects: When working with lambda expressions, make sure functions are stateless without side effects, which helps in predictable behavior.
Final Considerations
Converting InputStream
to a Stream
not only increases code efficiency but also enables a more elegant approach to handling data. By embracing Java 8’s Stream API, developers can tackle even the most complex data processing tasks with simplicity and precision.
For additional insights on Java streams, you might want to check out the official Java documentation on streams. Happy coding!