Choosing the Right Framework: Avoiding Common Pitfalls
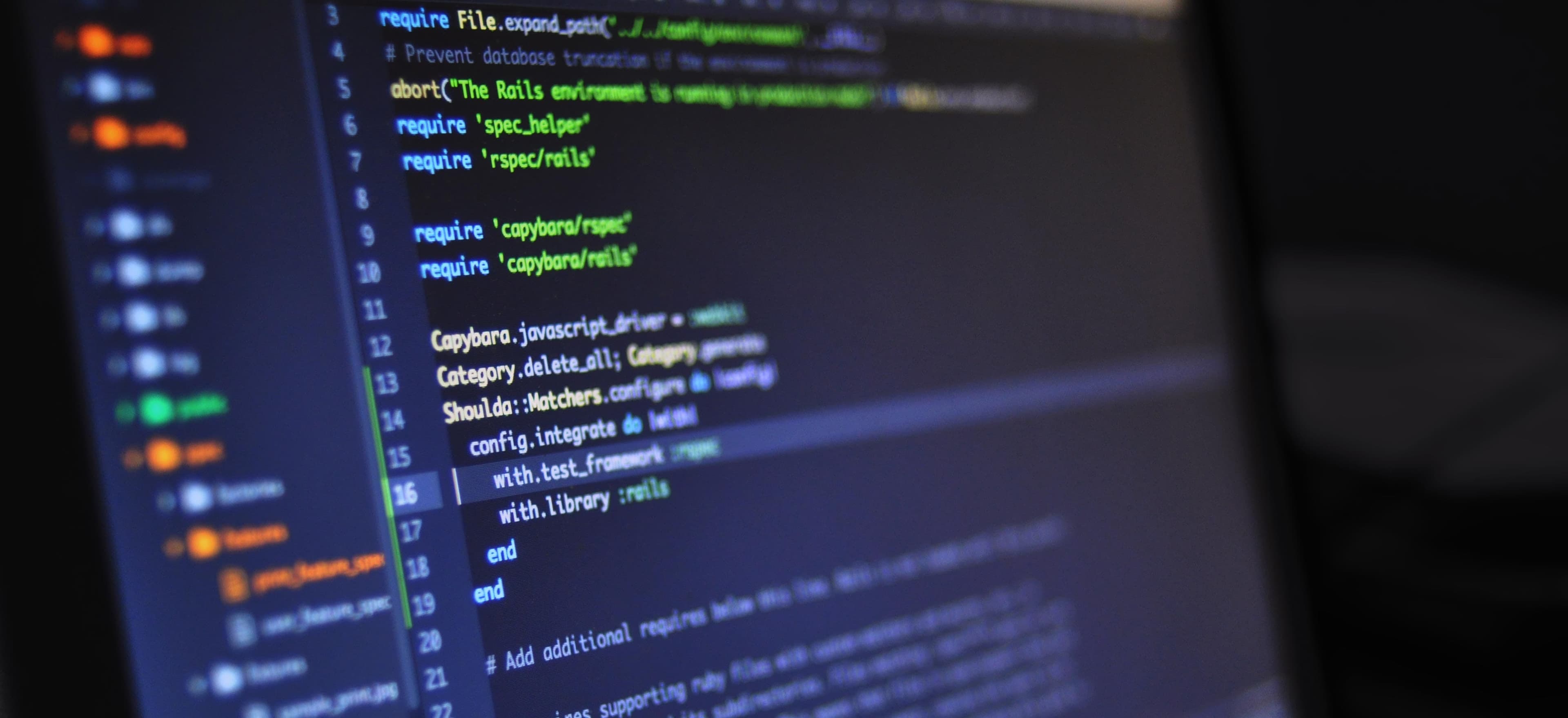
- Published on
Choosing the Right Framework: Avoiding Common Pitfalls in Java Development
In the rapidly evolving landscape of software development, choosing the right framework can be the difference between a successful project and a complete debacle. This is particularly true in Java, one of the most popular programming languages worldwide. With numerous frameworks available, each offering distinct advantages, the decision-making process can be overwhelming.
In this blog post, we'll explore the key factors to consider when selecting a Java framework, common pitfalls to avoid, and provide insights into frameworks that are well-suited for various projects.
Understanding Your Project Needs
Before diving into the framework pool, it is crucial to understand the specific requirements of your project. Are you building a web application, an enterprise application, or perhaps a microservice? Each type requires different architectural solutions and functionalities.
Project Type Analysis
-
Web Applications: Popular frameworks like Spring MVC and JavaServer Faces (JSF) are designed to simplify the complexities of web development.
-
Enterprise Applications: If your focus is on enterprise solutions, frameworks such as Spring Boot and Jakarta EE (formerly Java EE) might be ideal due to their robust support for enterprise components.
-
Microservices: For microservices, consider frameworks like Spring Cloud or Quarkus, which are optimized for cloud-native applications.
By determining the type of application you are developing, you can align your framework selection with your project's primary goals.
Common Pitfalls to Avoid
1. Overengineering
One of the most common mistakes developers face is overengineering their solutions. The allure of extensive features and capabilities often leads to unnecessary complexity.
// Example of overengineering
public class UserService {
// Injecting multiple services that may not be needed for a simple task
private final NotificationService notificationService;
private final LoggingService loggingService;
private final ValidationService validationService;
public UserService(NotificationService notificationService, LoggingService loggingService, ValidationService validationService) {
this.notificationService = notificationService;
this.loggingService = loggingService;
this.validationService = validationService;
}
public void createUser(User user) {
validationService.validate(user); // Validating user
// Additional complex logic might go here
}
}
In this example, injecting unnecessary services for a simple user creation leads to complexity. Instead, keep your services lean and focused on their primary responsibilities.
2. Ignoring Community Support
Frameworks with active communities can be incredibly beneficial in the long run. Lack of support can doom a project, especially when troubleshooting unexpected issues or upgrading to newer versions.
Why Community Matters
- Documentation: Well-supported frameworks tend to have comprehensive documentation, which is invaluable for developers.
- Updates: Active communities lead to regular updates and security patches.
- Plugins and Libraries: More community support means more available third-party libraries that can save development time.
3. Neglecting Performance Considerations
Performance can be a defining factor in the user experience of your application. Some frameworks come with built-in optimizations, while others may require extensive tuning.
For instance, Spring Boot is known for its ease of use but can introduce performance issues if not configured correctly.
Example: Basic Spring Boot Application
@SpringBootApplication
public class ExampleApplication {
public static void main(String[] args) {
SpringApplication.run(ExampleApplication.class, args);
}
}
This minimal setup is excellent for starting with Spring Boot. However, to address performance, consider the following:
- Use the embedded server (Tomcat or Jetty) appropriately.
- Profile your application to find bottlenecks.
- Use caching libraries like Ehcache when necessary.
4. Lack of Testing Considerations
Testing plays a crucial role in maintaining code quality. A framework that lacks built-in testing support may hinder the quality assurance processes of your project.
Example: JUnit for Testing
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class UserServiceTest {
@Test
void testCreateUser() {
UserService userService = new UserService();
User user = new User("John Doe");
userService.createUser(user);
// Assuming there's a method to retrieve a user
assertEquals("John Doe", userService.getUser("John Doe").getName());
}
}
In this example, JUnit is being used for testing service functionality. Always integrate a testing strategy reflective of the framework’s capabilities.
5. Ignoring Scalability
When choosing a framework, consider how well it scales. A framework that works for small projects may not hold up under enterprise-level demands.
Frameworks like Spring and Jakarta EE offer built-in scalability features, providing robust solutions that can be essential for large applications.
Evaluating Popular Java Frameworks
With countless frameworks available, here's a closer look at some popular options and what they bring to the table.
Spring Framework
Strengths:
- Comprehensive ecosystem (Spring Boot, Spring Cloud).
- Rich documentation and community support.
- Flexibility and inversion of control (IoC) capabilities.
Use Cases:
Spring is perfect for web applications, microservices, and enterprise solutions.
Jakarta EE
Strengths:
- Enterprise-grade features.
- Mature and stable platform with long-term support.
- Built-in security and transaction management.
Use Cases:
Ideal for large-scale enterprise systems needing reliability and performance.
Apache Struts
Strengths:
- Established MVC framework.
- Strong integration with existing servlets.
Use Cases:
Best suited for traditional web applications but may require updates for modern practices.
The Bottom Line
Choosing the right framework in Java development is critical to your project's success. By understanding your project needs, avoiding common pitfalls, and being mindful of community support, performance, testing, and scalability, you can navigate this crucial decision wisely.
Remember, the right framework will not only align with your current needs but will also support your future scaling and development efforts. Choose wisely you will thank yourself later.
For more information on Java frameworks, you can check out Spring Framework Documentation or Jakarta EE Documentation.
By adhering to the considerations outlined in this post, you can set yourself and your team on a path to successful Java development. Happy coding!