How to Identify and Resolve Deadlocks in Your System
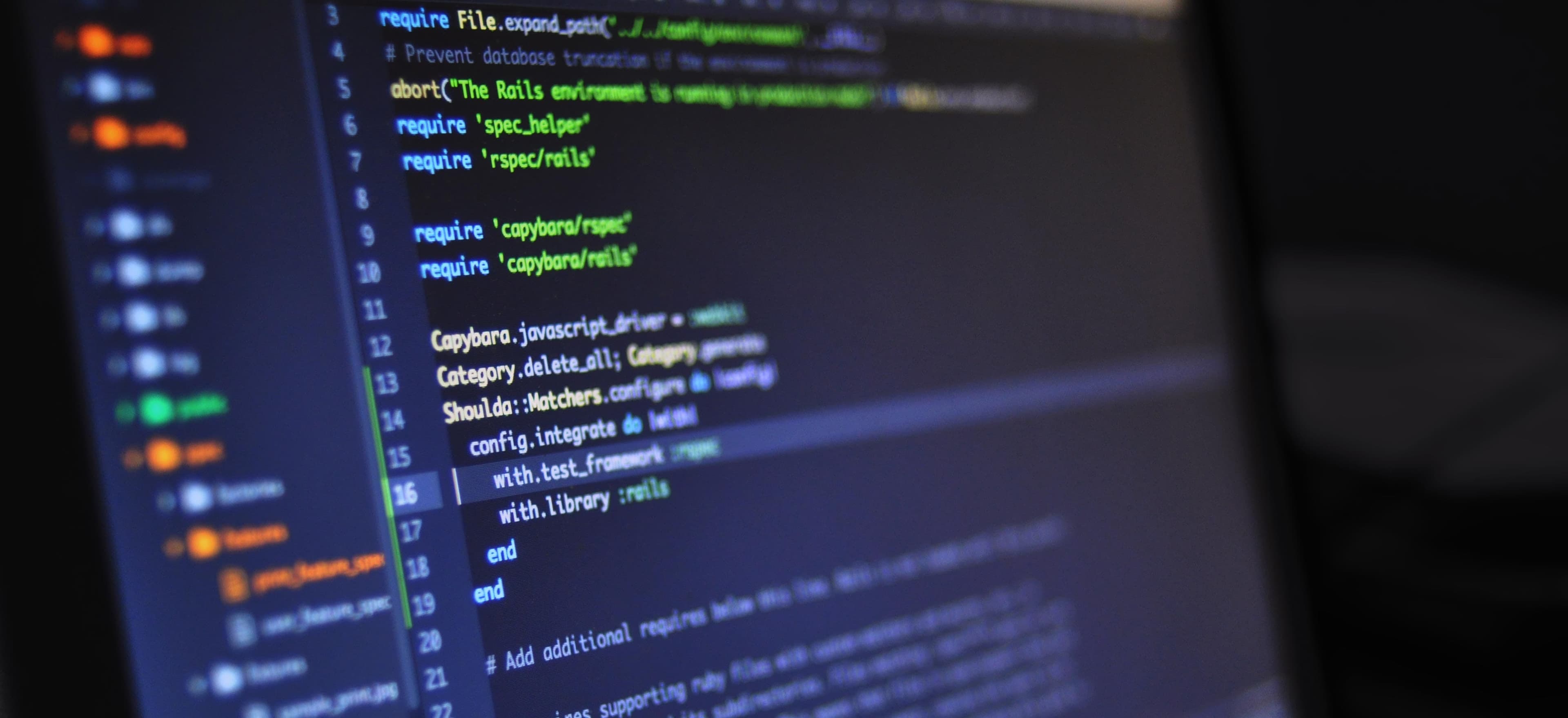
- Published on
How to Identify and Resolve Deadlocks in Your System
Deadlocks are one of the most frustrating issues any developer can face while working on concurrent programming. A deadlock occurs when two or more threads or processes are blocked forever, each waiting for the other to release a resource. Understanding deadlocks is crucial for developing robust multi-threaded applications. This blog post will guide you through the identification and resolution of deadlocks, using Java as our primary programming language.
Understanding Deadlocks
To put it simply, a deadlock is a standstill condition that arises in a multitasking system when processes are unable to proceed because each is waiting for the other to release a resource. Let’s illustrate this concept with a classic example:
Imagine two threads, Thread A and Thread B.
- Thread A holds Resource 1 and waits to acquire Resource 2.
- Thread B holds Resource 2 and waits to acquire Resource 1.
This situation creates a cycle where both threads are waiting for each other, leading to a deadlock state.
Key Conditions for Deadlocks
For a deadlock to occur, four conditions must hold true simultaneously:
- Mutual Exclusion: At least one resource must be held in a non-shareable mode.
- Hold and Wait: There must be a process holding at least one resource and waiting to acquire additional resources.
- No Preemption: A resource can be released only voluntarily by the process holding it, after the process has completed its task.
- Circular Wait: There must be a circular chain of processes where each process is waiting for a resource held by the next process in the chain.
Identifying Deadlocks
Detecting deadlocks typically involves monitoring your application or using tools specifically designed for deadlock detection. Here are a few ways to identify deadlocks in Java:
1. Using Thread Dumps
A thread dump provides a snapshot of all the threads that are currently running in the Java Virtual Machine (JVM). It often contains useful information regarding the thread state, including those that are blocked.
To generate a thread dump, you can use tools like jstack
:
jstack <pid>
You will need to replace <pid>
with the process ID of your Java application. The output will show you the state of all threads. Look for threads in the BLOCKED
state. The dump contains stack traces that indicate which resource each thread is waiting for.
2. Java Monitoring Tools
Several Java monitoring tools can point out deadlocks in your application, such as:
- VisualVM: A tool that monitors, troubleshoots, and optimizes Java applications in a variety of environments.
- Java Mission Control: Provided with the Oracle JDK, this tool helps profile and monitor Java applications.
Using these application performance monitoring tools can help visualize threads and their states in real-time, thereby making deadlock diagnosis easier.
3. Programmatic Detection
You can include programmatic detection of deadlocks using the ThreadMXBean
class from the java.lang.management
package:
import java.lang.management.ManagementFactory;
import java.lang.management.ThreadInfo;
import java.lang.management.ThreadMXBean;
public class DeadlockDetector {
public static void main(String[] args) {
ThreadMXBean threadMXBean = ManagementFactory.getThreadMXBean();
// Get thread IDs of threads that are deadlocked
long[] deadlockedThreads = threadMXBean.findDeadlockedThreads();
if (deadlockedThreads != null) {
System.out.println("Deadlocked threads detected:");
for (long threadId : deadlockedThreads) {
ThreadInfo threadInfo = threadMXBean.getThreadInfo(threadId);
System.out.println(threadInfo);
}
} else {
System.out.println("No deadlocks detected.");
}
}
}
In this code snippet:
- We use the
ThreadMXBean
to retrieve any deadlocked threads. - If deadlocked threads are found, we print their information.
Resolving Deadlocks
Once you’ve identified a deadlock, resolving it is the next step. Here are several strategies to consider:
1. Resource Ordering
One of the most effective methods for preventing deadlocks is to enforce an ordering on resource acquisition. Ensure that all threads acquire resources in a predefined order.
public class Resource {
private final Object lockA = new Object();
private final Object lockB = new Object();
public void process() {
synchronized (lockA) {
System.out.println("Acquired Lock A");
synchronized (lockB) {
System.out.println("Acquired Lock B");
}
}
}
}
In this example, if all threads acquire lockA
before lockB
, you will eliminate the possibility of a deadlock situation arising.
2. Timeout for Resource Acquisition
Another strategy involves implementing a timeout mechanism. When a thread attempts to acquire a resource, it waits for a specific period before giving up. Here’s how you can implement it:
import java.util.concurrent.TimeUnit;
public void safeLock(Object lock1, Object lock2) {
boolean gotLock1 = false;
boolean gotLock2 = false;
try {
gotLock1 = tryLock(lock1);
gotLock2 = tryLock(lock2);
} finally {
if (gotLock1 && !gotLock2) {
unlock(lock1);
} else if (gotLock2 && !gotLock1) {
unlock(lock2);
}
}
}
private boolean tryLock(Object lock) {
try {
return lock.tryLock(5, TimeUnit.SECONDS);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
return false;
}
}
In the above code:
- We check if we can acquire locks on
lock1
andlock2
within a timeout. - If we fail to acquire both, we back off and release the locks to avoid deadlocks.
3. Deadlock Detection and Recovery
If you cannot prevent deadlocks, you can implement a recovery mechanism. When a deadlock is detected, one of the involved threads can be terminated (a kill strategy) or rolled back to a previous state (rollback). Recovery strategies can vary depending on your application.
A Final Look
Deadlocks can be a nightmare for developers working with multi-threaded applications. However, with proper identification techniques and resolution strategies, you can mitigate deadlocks effectively.
- Always monitor your Java application, either through thread dumps or monitoring tools.
- Adopt best practices such as resource ordering and timeout management to prevent deadlocks upfront.
- Implement detection and recovery mechanisms to handle deadlocks that arise despite your best precautions.
For further reading on topics related to multi-threading and concurrency in Java, consider exploring Java Concurrency in Practice or check out the Oracle's Java Tutorial on Threads.
By rigorously applying these techniques, you can retain full control over your Java applications, ensuring a smooth user experience free from deadlock issues. Happy coding!