Java 8 Pitfall: Avoiding Memory Issues with Files.lines()
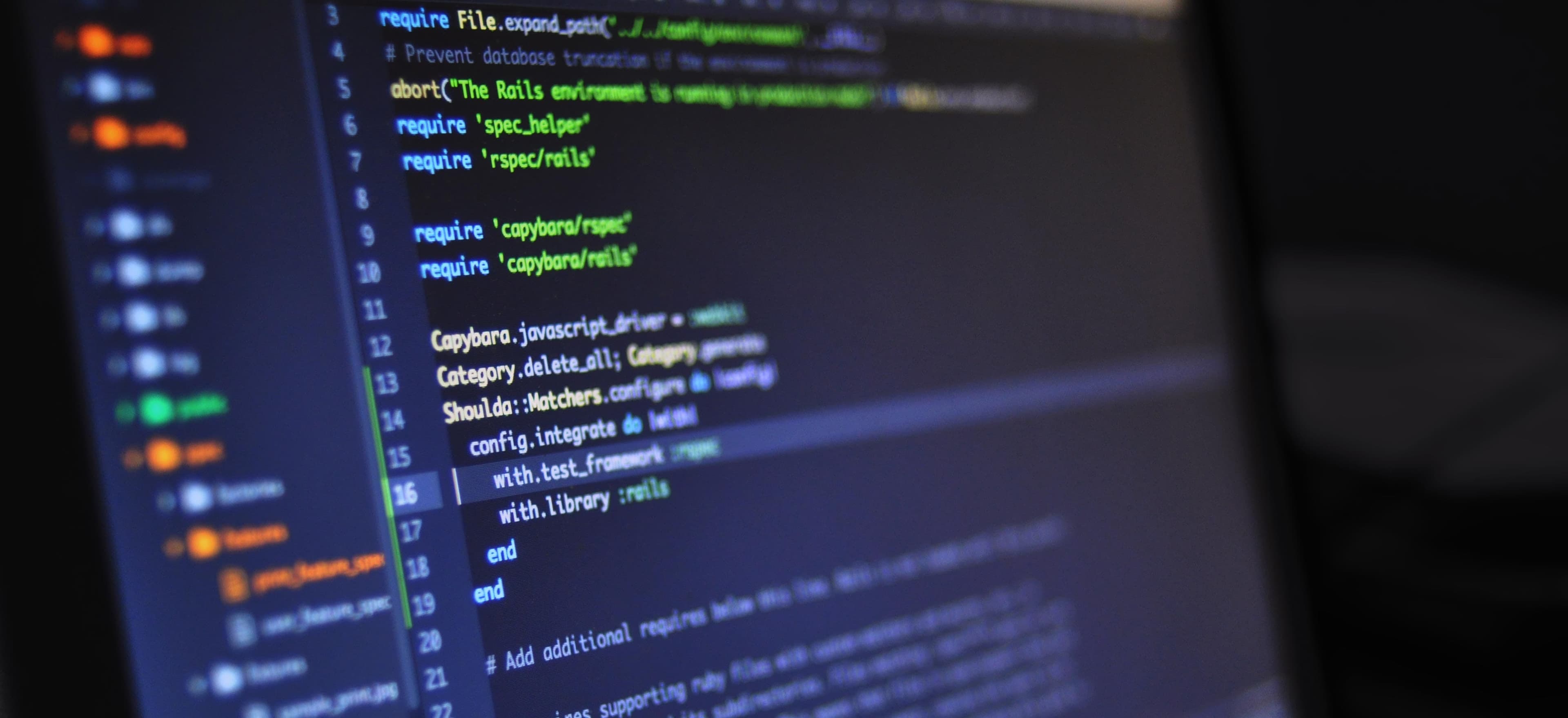
- Published on
Java 8 Pitfall: Avoiding Memory Issues with Files.lines()
Java 8 introduced a wealth of new features, among which the Files.lines()
method stands out for its ability to read lines from a file efficiently. However, it comes with some considerations that developers must understand to avoid memory-related issues.
In this blog post, we will explore the Files.lines()
method, discuss potential pitfalls, and suggest best practices to prevent memory exhaustion.
What is Files.lines()
?
Files.lines()
is a method from the java.nio.file.Files
class that allows you to read all lines from a file using a Stream. This makes it easy to work with files in a functional style, enabling operations like filtering, mapping, and summarizing.
Basic Usage
Here’s a straightforward example of how to use Files.lines()
to read from a file and print all lines to the console:
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
public class ReadFileExample {
public static void main(String[] args) {
String filePath = "example.txt"; // The file to read
try (Stream<String> lines = Files.lines(Paths.get(filePath))) {
lines.forEach(System.out::println);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why Use Files.lines()
?
-
Efficiency: It utilizes Java's Stream API, allowing for more efficient processing of the file contents, especially when combined with lambda expressions.
-
Lazy Evaluation:
Files.lines()
reads the file lazily, meaning it processes lines one at a time, reducing memory footprint compared to loading the entire file into memory. -
Functional Style: It makes code cleaner and more readable by allowing functional operations on file contents.
Understanding the Pitfall
While the Files.lines()
method does offer a host of benefits, it has one notable pitfall: the Stream must be closed. Failing to close the Stream can lead to memory issues, especially when dealing with large files.
Memory Issues Explained
When you create a Stream with Files.lines()
, it opens a connection to the file. If this connection is not closed, it may result in:
- Increased memory consumption.
- Resource leaks.
- Inability to reread the file until the Stream is closed, which can lead to application hang or crash.
Example of Memory Issue
Consider the following example that neglects to close the Stream properly:
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.stream.Stream;
public class MemoryIssueExample {
public static void main(String[] args) {
String filePath = "largefile.txt"; // A large file
Stream<String> lines = Files.lines(Paths.get(filePath));
// Processing lines without closing the stream
lines.filter(line -> line.contains("error"))
.forEach(System.out::println);
// Memory could potentially run out if this block is re-executed
}
}
In this case, if the method is called multiple times, the connection stays open indefinitely leading to memory problems.
Best Practices for Avoiding Memory Issues
Now that we know the potential issues, here are best practices to avoid running into memory problems with Files.lines()
:
1. Always Use Try-with-Resources
A sure way to manage resources effectively is to use the try-with-resources statement. This automatically closes resources when the block exits:
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.stream.Stream;
public class BestPracticeExample {
public static void main(String[] args) {
String filePath = "largefile.txt"; // A large file
try (Stream<String> lines = Files.lines(Paths.get(filePath))) {
lines.filter(line -> line.contains("error"))
.forEach(System.out::println);
} catch (IOException e) {
e.printStackTrace();
}
}
}
2. Process Lines in Batches
If you're working with a large file and doing multiple operations, consider breaking the processing down into smaller batches. This keeps memory usage in check:
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.stream.Stream;
public class BatchProcessingExample {
public static void main(String[] args) {
String filePath = "hugefile.txt";
try (Stream<String> lines = Files.lines(Paths.get(filePath))) {
lines.forEach(line -> {
// Process each line individually
processLine(line);
});
} catch (IOException e) {
e.printStackTrace();
}
}
private static void processLine(String line) {
// Dummy processing
if (line.contains("error")) {
System.out.println(line);
}
}
}
3. Choose Appropriate Data Structures
Using the correct data structure is crucial. For example, if you are collecting lines that match a condition, use a structure that limits growth, like a List
with a maximum size:
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.List;
import java.util.ArrayList;
import java.util.stream.Stream;
public class LimitedStorageExample {
public static void main(String[] args) {
String filePath = "log.txt";
List<String> errors = new ArrayList<>(100); // limit the size
try (Stream<String> lines = Files.lines(Paths.get(filePath))) {
lines.filter(line -> line.contains("error"))
.forEach(line -> {
if (errors.size() < 100) { // size limit
errors.add(line);
}
});
} catch (IOException e) {
e.printStackTrace();
}
errors.forEach(System.out::println); // Printing errors
}
}
The Closing Argument
While Java 8's Files.lines()
method introduces a powerful way to read files, developers need to address its memory management implications. By following best practices like using try-with-resources and processing data in batches, you can harness the benefits of this method without falling prey to common pitfalls.
For further reading on Java's File I/O, you can check the official documentation Java NIO Tutorial.
By applying the guidelines discussed in this post, you can efficiently use Files.lines()
while ensuring that your Java applications remain robust and performant. Stay tuned for more insights into Java development!
Checkout our other articles