Handling SQS Delays: Boosting Spring Boot with Project Reactor
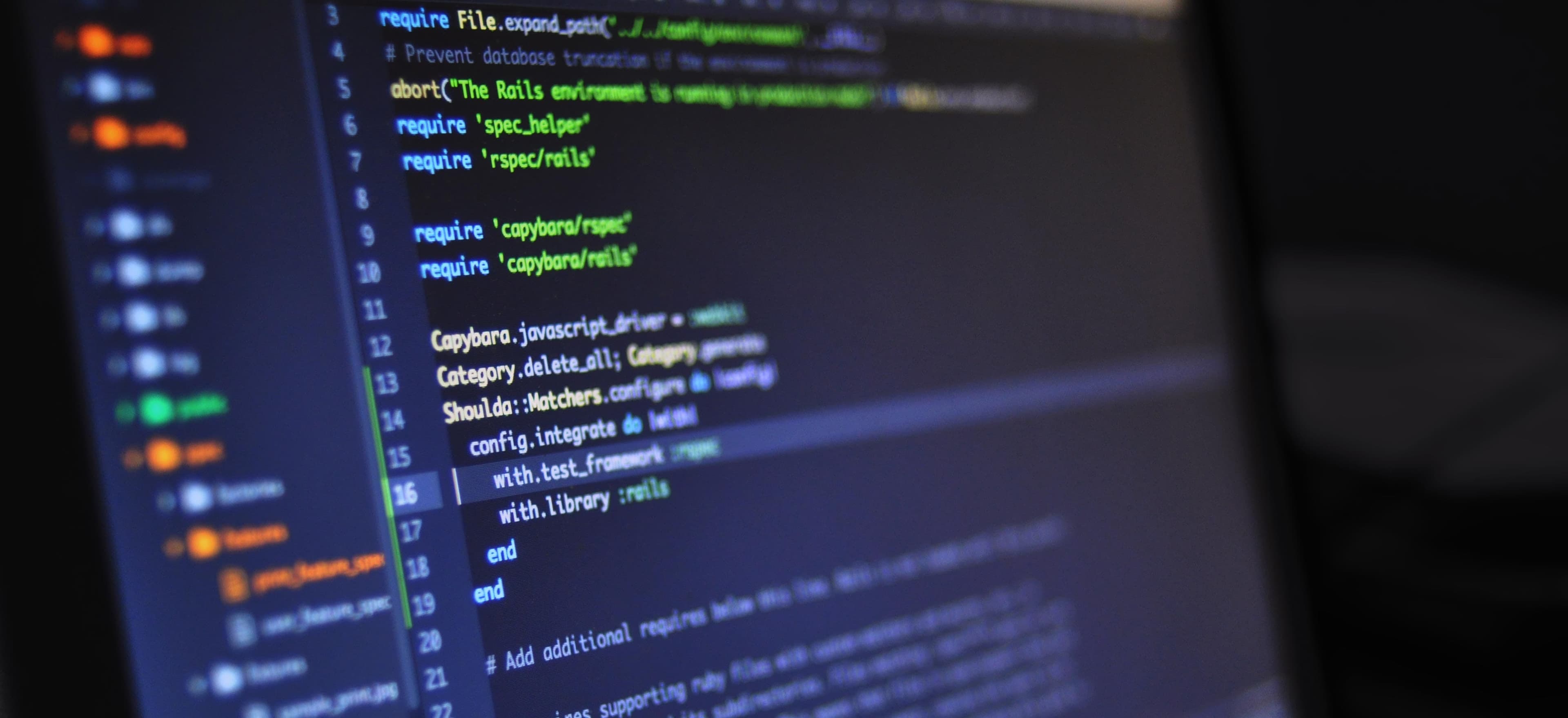
- Published on
Handling SQS Delays: Boosting Spring Boot with Project Reactor
In the modern world of software development, message queues play a crucial role in system architecture, enabling decoupling of services and ensuring reliable communication. Amazon Simple Queue Service (SQS) is one such solution that provides a scalable way to handle asynchronous message processing. However, managing SQS, especially when it comes to delays, poses its challenges. In this blog post, we discuss how to effectively handle SQS delays in a Spring Boot application using Project Reactor.
Table of Contents
- Understanding SQS and Delays
- Spring Boot and Project Reactor Overview
- Setting Up Your Spring Boot Application
- Integrating SQS with Spring Boot
- Handling Delays with Project Reactor
- Example Code
- Best Practices
- Conclusion
Understanding SQS and Delays
Amazon SQS is a fully managed message queuing service that provides reliable, secure, and scalable communication channels between distributed application components. A key feature of SQS is the ability to set delays on messages, which can be invaluable for handling scenarios like time-sensitive operations, rate limiting, and managing state transitions.
However, working with delayed messages can also complicate processing. The main challenge lies in ensuring that messages are:
- Properly Delayed: Avoid unnecessary delays that may lead to consumer timeouts or performance issues.
- Efficiently Consumed: Processing should happen as soon as the delay is lifted.
Understanding these aspects will pave the way for an efficient implementation using Spring Boot and Project Reactor.
Spring Boot and Project Reactor Overview
Spring Boot is a popular framework for building Java applications that simplifies the development process. It provides a range of features like dependency injection, embedded servers, and configuration management that allow developers to focus on business logic rather than boilerplate code.
Project Reactor is a reactive library for building non-blocking applications on the JVM. Its core components, Mono
and Flux
, enable developers to handle asynchronous data processing cleanly. By combining Spring Boot with Project Reactor, we can create a highly responsive application that can manage delays effectively.
Setting Up Your Spring Boot Application
To get started, create a new Spring Boot project. You can use Spring Initializr or any IDE that supports Maven or Gradle. Make sure to include the following dependencies:
- Spring Web
- Spring Cloud AWS Messaging
- Project Reactor
For example, using Maven, your pom.xml
might look like this:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-aws-messaging</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>io.projectreactor</groupId>
<artifactId>reactor-spring</artifactId>
</dependency>
With these dependencies in place, you are ready to set up your application to send and receive messages from SQS.
Integrating SQS with Spring Boot
First, configure your Spring Boot application to utilize Amazon SQS. Add the following properties to your application.properties
(or application.yml
):
cloud.aws.region.static=us-west-2
cloud.aws.credentials.access-key=YOUR_ACCESS_KEY
cloud.aws.credentials.secret-key=YOUR_SECRET_KEY
cloud.aws.sqs.endpoint=YOUR_SQS_ENDPOINT
Ensure you replace placeholders with your actual AWS credentials and endpoint.
Next, create a simple message producer using the AmazonSQSAsync
client to send messages to the SQS queue.
Handling Delays with Project Reactor
Now that we’ve integrated SQS with Spring Boot, let’s dive into how we can handle delays effectively using Project Reactor. When messages are sent to SQS with a delay, they will not be immediately available to consumers. We can manage this using Mono
or Flux
to schedule message sending and deferred processing.
Here’s a simple example to demonstrate leveraging Mono
and Flux
:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cloud.aws.messaging.core.QueueMessagingTemplate;
import org.springframework.stereotype.Service;
import reactor.core.publisher.Mono;
@Service
public class SqsService {
@Autowired
private QueueMessagingTemplate queueMessagingTemplate;
public Mono<Void> sendMessage(String message, int delay) {
return Mono.fromRunnable(() -> {
// Sending the message to the SQS queue with delay
queueMessagingTemplate.send("your-queue-name", message);
})
.delayElement(Duration.ofSeconds(delay))
.then();
}
}
Why This Code Matters
- Reactive Programming: By using
Mono.fromRunnable()
, we encapsulate sending the message into a mono that can be transformed. - Delay Handling:
delayElement()
allows us to defer message sending until a specified time, directly addressing the challenge of timing. - Asynchronous Processing: The
then()
operator ensures that we can chain further processing without blocking the thread.
Example Code
Let’s build upon the preceding code and demonstrate a complete workflow, including receiving messages.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cloud.aws.messaging.listener.annotation.SqsListener;
import org.springframework.stereotype.Service;
import reactor.core.publisher.Mono;
@Service
public class SqsReceiver {
@SqsListener("your-queue-name")
public void listen(String message) {
processMessage(message)
.subscribe();
}
private Mono<Void> processMessage(String message) {
return Mono.just(message)
.doOnNext(msg -> System.out.println("Received message: " + msg))
.then();
}
}
Best Practices
- Error Handling: Ensure that your reactive flow includes error handling mechanisms (like
.onErrorResume()
) to gracefully manage failures. - Monitoring: Use tools to monitor your message processing to keep track of delays and performance metrics.
- Backoff Strategy: Implement a strategy for exponential backoff for delayed message retries, reducing the load on your SQS queue.
- Testing: Create unit tests to validate message processing logic. Spring provides excellent support for testing SQS integration.
The Bottom Line
Handling SQS delays in a Spring Boot application using Project Reactor can significantly enhance your application's responsiveness and efficiency. By utilizing reactive programming principles, you can better manage asynchronous processing and ensure effective communication between your services.
If you're looking to dive deeper into Spring Boot and SQS, consider exploring the Spring Cloud AWS documentation for advanced functionalities and examples.
By following the practices and principles outlined in this article, you can build resilient and efficient applications that thrive in a messaging-centric architecture.
Happy coding!