Decoding IBM VM Thread Dumps: Common Analysis Pitfalls
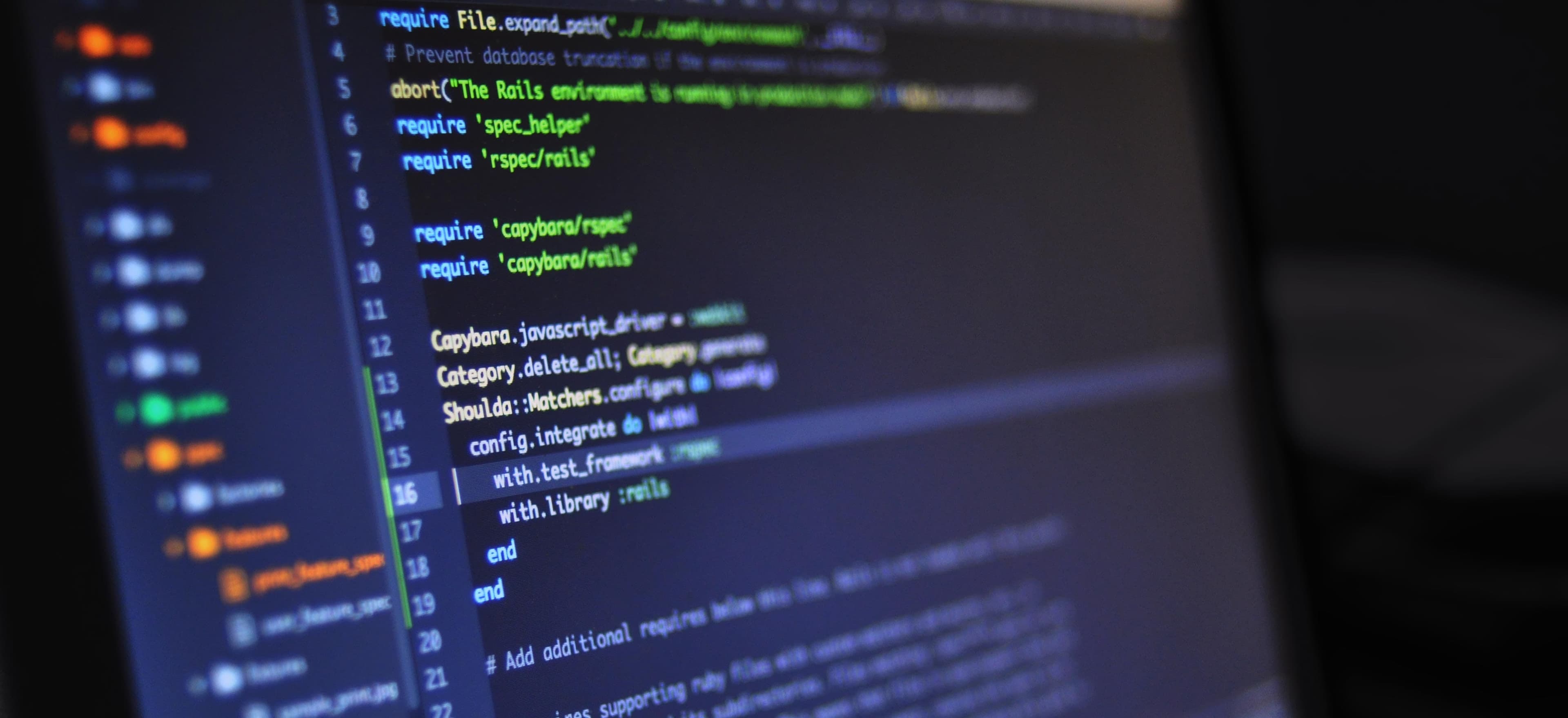
- Published on
Decoding IBM VM Thread Dumps: Common Analysis Pitfalls
Thread dumps are critical for diagnosing performance issues in multi-threaded applications. IBM Virtual Machine (VM) maintains a comprehensive method for capturing these dumps. Understanding how to interpret and analyze these dumps is essential for developers and system administrators alike.
What is a Thread Dump?
A thread dump is a snapshot of all the current threads that are executing in a virtual machine. It provides valuable insights about application performance, deadlocks, and memory usage. Each thread dump contains information about:
- Thread state (running, waiting, blocked, etc.)
- Stack traces
- Monitor locks and object status
Thread dumps can be generated on demand and are particularly useful when your application freezes or exhibits high CPU usage. IBM Documentation offers tools and commands to capture these dumps effectively.
Why Analyze Thread Dumps?
When performance issues arise in Java applications, thread dumps serve as a diagnostic tool. By analyzing thread dumps, developers can identify:
- Deadlocks: Where two or more threads wait indefinitely for one another.
- CPU issues: Hot spots indicating which threads are consuming excessive resources.
- Stuck threads: Threads that are blocked and fail to progress.
However, interpreting thread dumps can lead to confusion and mistakes due to their complexity. In this blog post, we will explore common pitfalls to avoid when analyzing IBM VM thread dumps.
Common Analysis Pitfalls
1. Ignoring Thread States
The Pitfall: One common mistake is to overlook the various thread states. A thread might appear to be blocked or waiting but is this really an issue?
The Solution: Examine each thread's state to understand its activity. Thread states can be one of several forms:
- RUNNABLE: Actively executing.
- BLOCKED: Waiting for a monitor lock.
- WAITING: Waiting indefinitely for another thread to perform a particular action.
- TIMED_WAITING: Waiting for another thread to perform an action for a specified period.
To clearly delineate these states, use a tool like IBM Thread and Monitor Dump Analyzer to visualize the state differences.
Example Code
public class MyThreadExample extends Thread {
@Override
public void run() {
synchronized (this) {
try {
Thread.sleep(1000); // Simulating work
System.out.println("Thread is running.");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
In this code, we can see that if multiple threads were trying to run this run()
method, they could enter a BLOCKED state due to the synchronized keyword.
2. Failing to Check for Deadlocks
The Pitfall: Another common mistake is not recognizing deadlocks in thread dumps. Deadlocks can cause your application to freeze.
The Solution:
When examining a thread dump, look for threads that are waiting on locks held by each other. This pattern indicates a potential deadlock. Use the jstack
command to get a clearer picture of the locking structures in place.
Example Code
public class DeadlockExample {
static final Object lock1 = new Object();
static final Object lock2 = new Object();
public static void main(String[] args) {
Thread thread1 = new Thread(() -> {
synchronized (lock1) {
synchronized (lock2) {
System.out.println("Thread 1 got both locks");
}
}
});
Thread thread2 = new Thread(() -> {
synchronized (lock2) {
synchronized (lock1) {
System.out.println("Thread 2 got both locks");
}
}
});
thread1.start();
thread2.start();
}
}
In this code, thread1
and thread2
can cause a deadlock. When analyzing the thread dump, you would expect to see both threads waiting for locks that the other thread currently owns.
3. Overanalyzing the Dump
The Pitfall: Over-analyzing or misinterpreting what seems like critical information can lead to misguided troubleshooting.
The Solution: Focus on the thread dumps that immediately pertain to the issue at hand. Investigate only the threads that are reported to be slow or failing.
4. Lack of Context
The Pitfall: Failing to consider the state of the application when the dump was created can be misleading.
The Solution: Always gather information about the application's state at the time of the dump. What requests were being processed? What resources were available? Additionally, consider using tools like IBM APM for context.
5. Neglecting System Metrics
The Pitfall: Ignoring overall system health metrics while analyzing thread dumps can lead to incomplete conclusions.
The Solution: Collect and review system metrics such as CPU and memory usage alongside thread dumps. Code can often give a false impression of issues without understanding the broader context of resource consumption.
Tools for Thread Dump Analysis
IBM Thread Dump Analyzer
This tool automatically analyzes thread dumps for you, spotting potential issues like deadlocks and bottlenecks. It provides a user-friendly interface for examining thread states and transitions.
jstack
Included with the Java Development Kit (JDK), jstack
can take thread dumps from a running application. You can use it to observe the system state without installing additional software.
VisualVM
VisualVM is a visual tool for monitoring and troubleshooting Java applications. It allows for real-time monitoring of thread states, memory usage, and CPU profiling.
Best Practices for Analysis
-
Regular Dumping: Generate thread dumps regularly for active applications. This will help establish a baseline for normal behavior.
-
Document Findings: When you analyze a thread dump, document your findings. This history can become invaluable for future troubleshooting.
-
Automate Analysis: Consider automating the analysis with scripts or software tools to minimize human error.
-
Engage the Team: Collaborate with your team members to review and discuss findings, as different perspectives can illuminate overlooked details.
My Closing Thoughts on the Matter
Decoding IBM VM thread dumps does not have to be a daunting task. By avoiding common analysis pitfalls, incorporating the right tools, and adopting best practices, developers can gain insightful knowledge about their applications' performance. The next time you encounter performance issues, remember to analyze the thread dump thoroughly, keeping in mind the pitfalls discussed here.
For more information on analyzing Java performance, check out the IBM Developer portal or explore Java Performance Tuning. Happy coding!
Feel free to comment with any questions or additional insights on thread dump analysis. The journey to understanding system performance is ongoing, and every shared experience broadens our collective knowledge.