How to Effectively Mock gRPC Services for Unit Testing
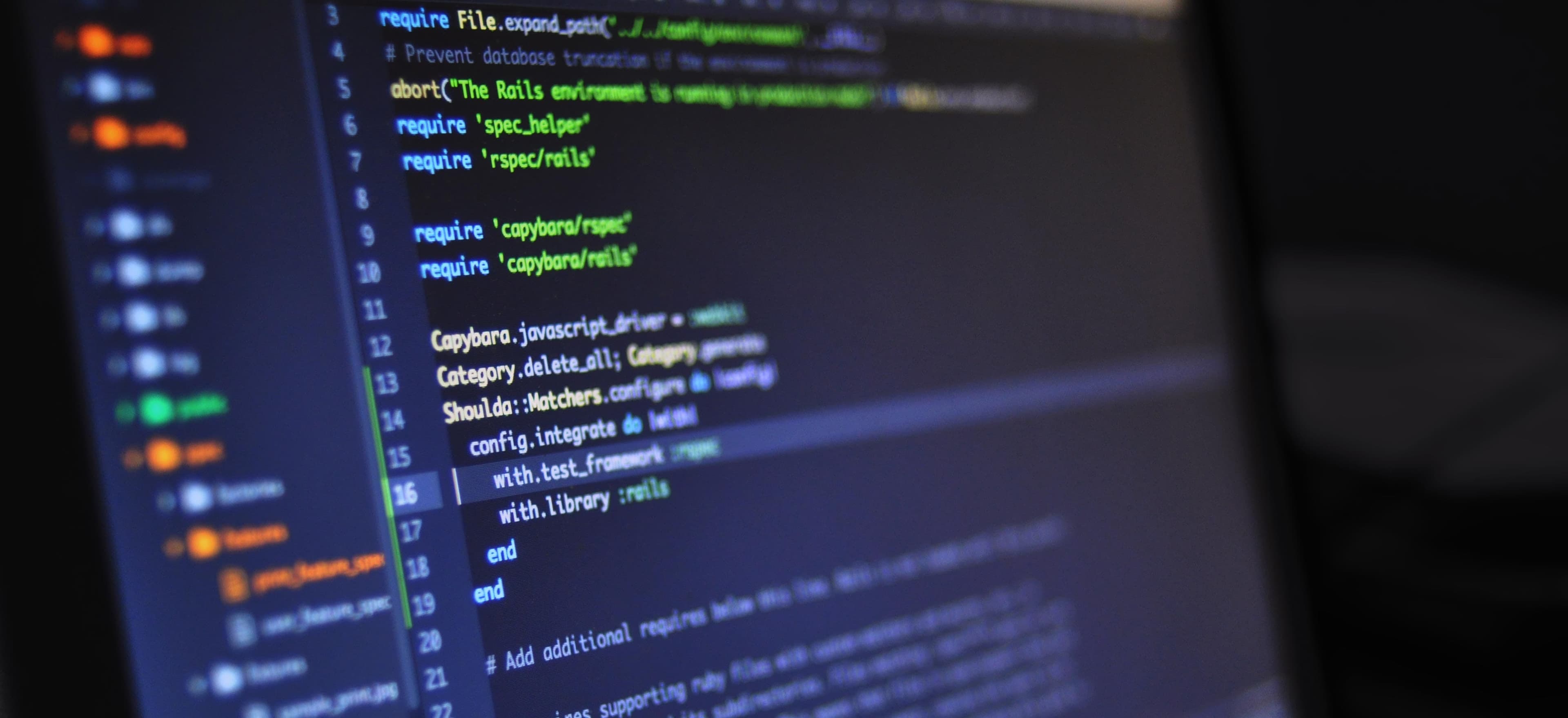
- Published on
How to Effectively Mock gRPC Services for Unit Testing
gRPC (Google Remote Procedure Call) is a modern, high-performance framework for remote procedure calls. It allows clients and servers to communicate efficiently through a defined protocol. However, when it comes to unit testing gRPC services, dealing with network calls can be cumbersome. Fortunately, mocking provides a streamlined approach to create robust unit tests for your gRPC services. In this blog post, we will explore how to effectively mock gRPC services for unit testing, providing clear code snippets and explanations.
Why Mock gRPC Services?
Unit tests are essential for ensuring the reliability and correctness of your code. When it comes to testing gRPC services, mocking offers several advantages:
- Isolation: By mocking services, you ensure that your tests focus on the unit of work and not on external dependencies.
- Speed: Mocking removes the overhead of network calls, making your tests execute faster.
- Control: Mocks provide you with the ability to simulate various scenarios, including error states.
- Deterministic Results: You can easily control the data returned by mocks, leading to predictable test outcomes.
Setting Up the Environment
Before diving into code, make sure you have the following prerequisites:
-
gRPC Installed: Install
grpc
andgrpc-tools
in your development environment. For Go, you can use:go get google.golang.org/grpc
-
Mocking Framework: We will use
gomock
as our mocking framework. Install it with:go get github.com/golang/mock/gomock
-
Mocking Generation: You’ll need to generate mocks for your gRPC service. Below is an example of how to generate mocks for a gRPC service defined in a
.proto
file.
Generating Mocks
Let’s say you have the following service defined in a hello.proto
file:
syntax = "proto3";
package hello;
service Greeter {
rpc SayHello(HelloRequest) returns (HelloReply);
}
message HelloRequest {
string name = 1;
}
message HelloReply {
string message = 1;
}
You can generate Go mocks with:
protoc --go_out=. --go-grpc_out=. hello.proto
go generate ./...
Generating Mocks with Gomock
Next, we’ll use gomock
to generate mocks based on the gRPC service interface:
mockgen -destination=mocks/mock_greeter.go -package=mocks hello.Greeter
Now that we have our mocks set up, let's dive into mocking gRPC calls in our unit tests.
Writing Unit Tests with Mocked gRPC Services
We will create a simple example to illustrate how we can mock the Greeter
service for testing a client.
The Client Code
First, we will develop a simple gRPC client that uses the Greeter
service. Here's a simple implementation of what we want to test:
// greeter_client.go
package client
import (
"context"
"google.golang.org/grpc"
"your_project/hello"
)
type GreeterClient struct {
conn *grpc.ClientConn
greeter hello.GreeterClient
}
func NewGreeterClient(address string) (*GreeterClient, error) {
conn, err := grpc.Dial(address, grpc.WithInsecure())
if err != nil {
return nil, err
}
greeter := hello.NewGreeterClient(conn)
return &GreeterClient{conn: conn, greeter: greeter}, nil
}
func (g *GreeterClient) SayHello(ctx context.Context, name string) (string, error) {
req := &hello.HelloRequest{Name: name}
res, err := g.greeter.SayHello(ctx, req)
if err != nil {
return "", err
}
return res.Message, nil
}
func (g *GreeterClient) Close() {
g.conn.Close()
}
The Unit Test
With our client code in place, we can now proceed to write a unit test that leverages the mocked Greeter
service:
// greeter_client_test.go
package client_test
import (
"context"
"testing"
"github.com/golang/mock/gomock"
"github.com/stretchr/testify/assert"
"your_project/client"
"your_project/mocks" // import the generated mocks
"your_project/hello"
)
func TestSayHello(t *testing.T) {
ctrl := gomock.NewController(t)
defer ctrl.Finish()
mockGreeter := mocks.NewMockGreeterClient(ctrl)
// Setup the expectations for the SayHello method
mockGreeter.EXPECT().SayHello(gomock.Any(), &hello.HelloRequest{Name: "World"}).
Return(&hello.HelloReply{Message: "Hello, World!"}, nil)
greetingClient := client.GreeterClient{greeter: mockGreeter}
// Call the SayHello method
msg, err := greetingClient.SayHello(context.Background(), "World")
// Validate the result
assert.NoError(t, err)
assert.Equal(t, "Hello, World!", msg)
}
Breakdown of the Test Code
-
Setup Mock Controller: We create a new
gomock.Controller
which will manage the lifecycle of our mocks. -
Create Mock Instance: A new instance of
MockGreeterClient
is created. This instance will respond whenSayHello
is called. -
Define Expectations: We set an expectation that when
SayHello
is invoked with theHelloRequest
containing the name "World", it should return aHelloReply
with the message "Hello, World!". -
Perform the Test: We then use the mock greeter in our client and invoke the
SayHello
method, which we expect to succeed without errors and return the correct message. -
Assertions: Finally, we validate that no errors were returned and that the output matches our expectations.
Expanding Your Tests
With the basic infrastructure in place, you can easily expand your tests to cover various scenarios:
- Testing how your client behaves with different input parameters.
- Simulating error responses from the mock service to examine how your client handles failures.
- Measuring performance impacts by running your tests with mocks rather than actual gRPC calls.
Wrapping Up
Mocking gRPC services for unit testing can significantly enhance the reliability and speed of your tests. By isolating your units of work and controlling the responses, you can create a robust test suite that thoroughly verifies your client’s behavior. Utilizing tools like gomock
simplifies this process, enabling developers to focus more on writing effective tests rather than dealing with the complexities of network calls.
For more information on gRPC and mocking practices, you can explore the gRPC documentation and the gomock repository.
By following the methodologies outlined in this article, you will be well-equipped to tackle unit testing for your gRPC services effectively. Happy coding!
Checkout our other articles