Android Loaders vs AsyncTask: The Performance Showdown
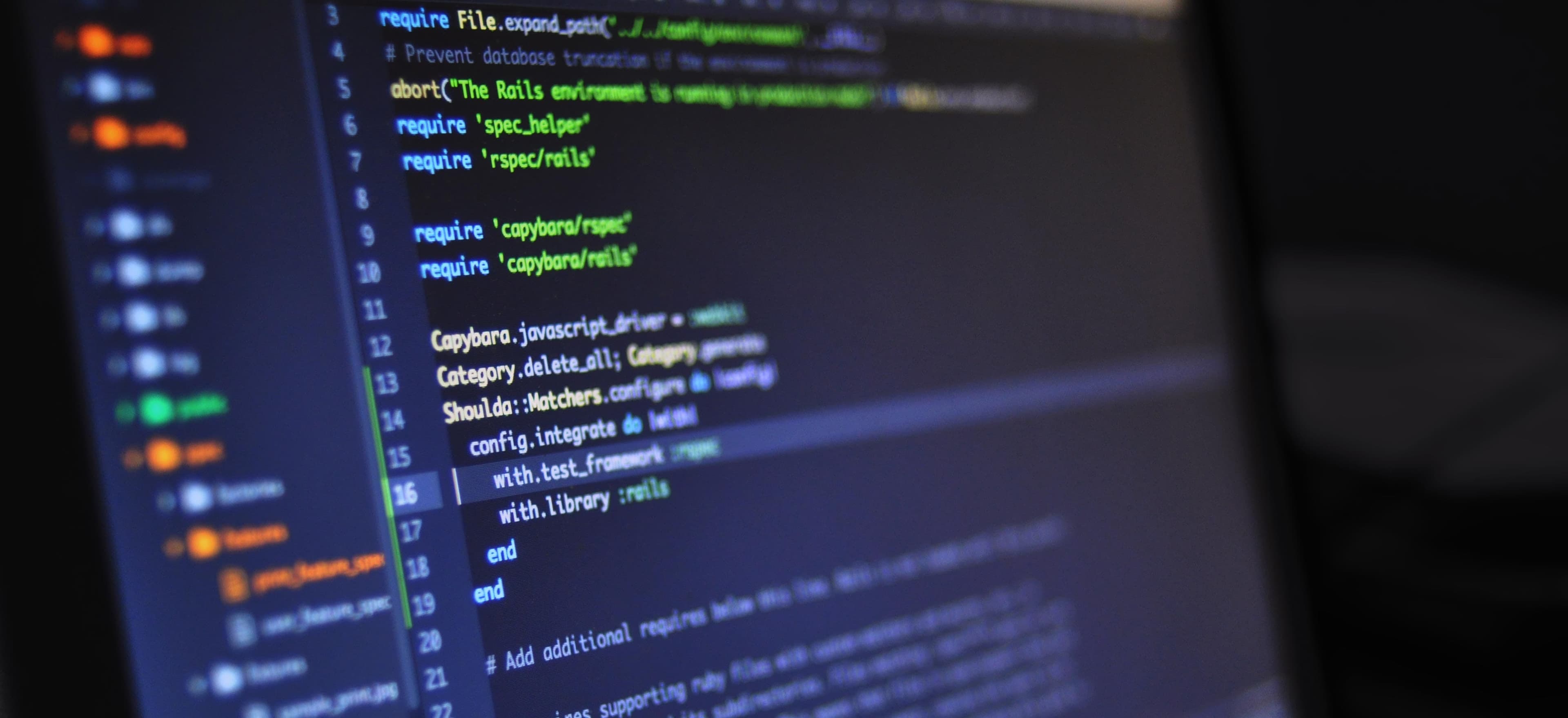
- Published on
Android Loaders vs AsyncTask: The Performance Showdown
Android development often involves handling background tasks, especially when it comes to fetching data, whether from a database or a web service. Making the right choice for performing these tasks is crucial, as it directly impacts the performance and responsiveness of your app. In this blog post, we will compare two popular methods for executing background operations in Android: Loaders and AsyncTask. We will delve deep into their performance characteristics, use cases, implementation details, and when to choose one over the other.
The Roadmap to Loaders and AsyncTask
Before diving into the performance showdown, let's clarify what Loaders and AsyncTask are and what they aim to solve.
AsyncTask
AsyncTask
is a built-in Android class in the android.os
package designed for simplifying the process of performing background operations. It allows you to execute tasks in the background and publish results on the UI thread without having to manage threads and handlers manually.
Loaders
Loaders
, on the other hand, are part of the Android framework that provides a way to perform asynchronous loading of data, specifically designed for the activity and fragment lifecycle. Loaders manage the lifecycle of your operations and automatically reconnect to the right data source when the activity or fragment is recreated.
Performance Comparison
Now that we have a basic understanding of both, let's compare their performance considering key factors like lifecycle management, ease of use, and efficiency.
1. Lifecycle Management
One of the standout features of Loaders is their built-in lifecycle management. Loaders are tied to the activity or fragment lifecycle, so they automatically manage the data they fetch. When an activity is stopped, and then later recreated (e.g., during configuration changes), the Loader retains the data, preventing unnecessary reloads.
public class MyLoader extends AsyncTaskLoader<List<MyData>> {
public MyLoader(Context context) {
super(context);
}
@Override
protected void onStartLoading() {
forceLoad();
}
@Override
public List<MyData> loadInBackground() {
// Perform long-running operation here
// For example, fetching data from the network
return fetchDataFromNetwork();
}
}
In contrast, AsyncTask
lacks this automatic lifecycle management. You need to handle screen rotation and other lifecycle events manually, which can lead to memory leaks or data loss.
2. Efficiency
When it comes to efficiency, Loaders stand out, especially in situations where data needs to be retained. Loaders can serve data even when the UI is destroyed and recreated without reloading it.
On the other hand, AsyncTask can be less efficient for data loading tasks. In applications where you frequently switch between activities or fragments, AsyncTask may result in repeated and costly data loads.
Consider the following example of an AsyncTask:
private class FetchDataTask extends AsyncTask<Void, Void, List<MyData>> {
@Override
protected List<MyData> doInBackground(Void... voids) {
// Perform a long-running operation to fetch data
return fetchDataFromNetwork();
}
@Override
protected void onPostExecute(List<MyData> result) {
// Update UI with the data
updateUIWithData(result);
}
}
Here, when the user's orientation changes, a new instance of FetchDataTask
is often triggered, leading to unnecessary network calls.
3. Memory Management
Memory management is a critical concern in Android development. Loaders help combat memory leaks better than AsyncTasks. When an activity is destroyed, a Loader can effectively drop any references it holds to the activity context.
With AsyncTask, it is common to hold a reference to an activity, which can lead to memory leaks if the task is still ongoing when the activity is destroyed. Thus, you must always be cautious about memory leaks with AsyncTask.
private class SafeAsyncTask extends AsyncTask<Void, Void, List<MyData>> {
private WeakReference<MainActivity> activityReference;
SafeAsyncTask(MainActivity context) {
activityReference = new WeakReference<>(context);
}
@Override
protected List<MyData> doInBackground(Void... voids) {
// Perform long-running operation
return fetchDataFromNetwork();
}
@Override
protected void onPostExecute(List<MyData> result) {
MainActivity activity = activityReference.get();
if (activity != null && !activity.isFinishing()) {
activity.updateUIWithData(result);
}
}
}
This pattern of using a WeakReference
helps prevent memory leaks, but it introduces extra code complexity.
Final Considerations: When to Use Each Approach
Choosing between Loaders and AsyncTask depends on your app's specific needs:
-
Use Loaders when:
- You need data to survive activity and fragment recreation.
- You want to simplify lifecycle management without worrying about leaks.
- Your application deals with databases or content providers which are already optimized for Loaders.
-
Use AsyncTask when:
- You have small tasks that can be executed relatively quickly and don't involve managing UI state after the lifecycle.
- You are working within scenarios where Loaders might add unnecessary complexity.
For more detailed guides on improving performance while developing Android applications, refer to Android Developer Guidelines and Improve App Performance.
Summary
In this performance showdown between Android Loaders and AsyncTask, we see that both have their unique advantages and disadvantages. While AsyncTask
provides a quick remedy for simple background tasks, Loaders
offer better lifecycle management, efficiency, and memory handling.
Deciding which to use comes down to the specific requirements of your application. By understanding how each works and their respective use cases, you can ensure a smoother user experience in your Android apps.
By choosing the right tool for your background operations, you lay the groundwork for an efficient, responsive, and user-friendly Android application. Happy coding!
Checkout our other articles