Overcoming Common Pitfalls in Domain-Driven Design
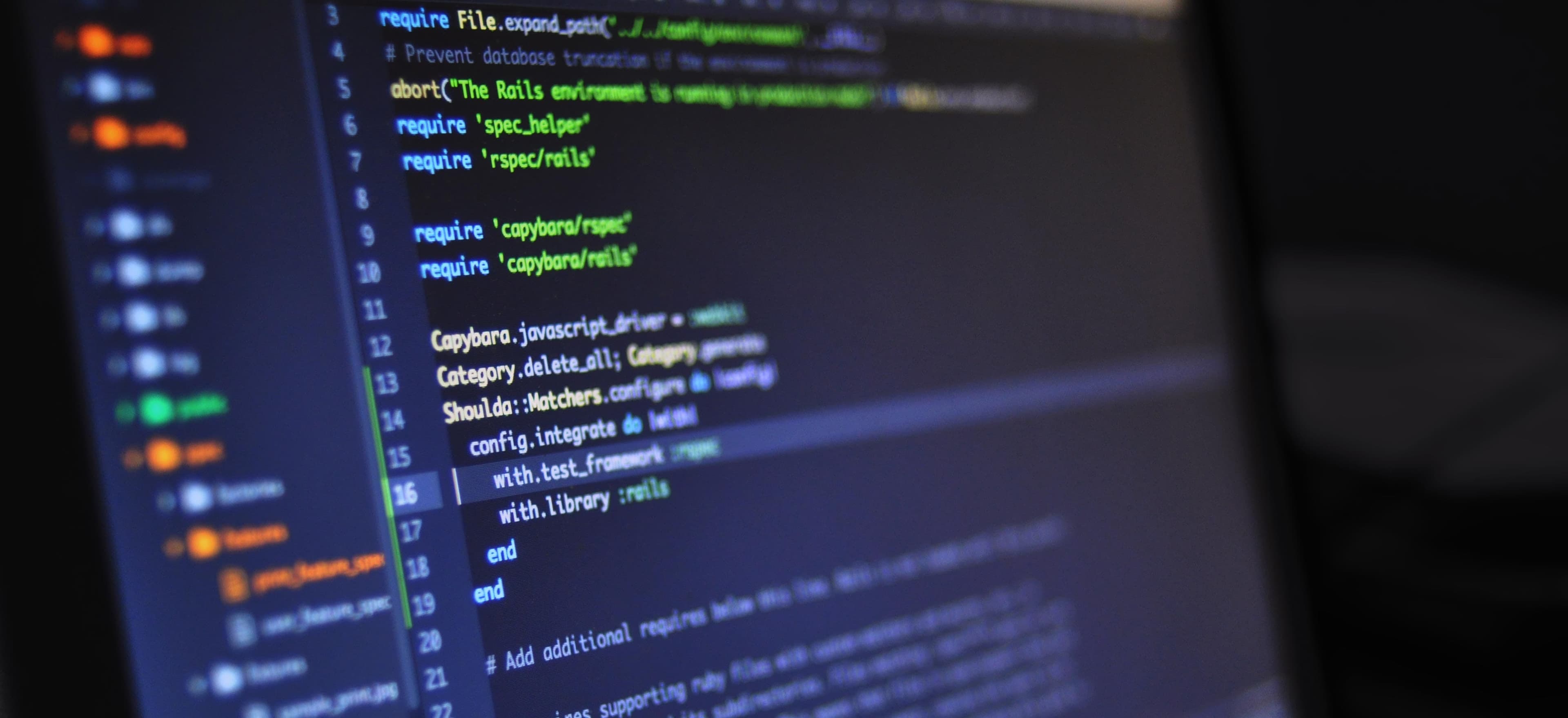
- Published on
Overcoming Common Pitfalls in Domain-Driven Design
Domain-Driven Design (DDD) is a powerful approach to software development, designed to address complex needs by aligning your codebase with the business domain. However, implementing DDD isn't without its challenges. In this article, we will explore some common pitfalls when applying DDD principles and how to avoid them.
Understanding Domain-Driven Design
Before diving into potential pitfalls, it's important to define what DDD entails. At its core, DDD focuses on creating a shared understanding of the domain among developers and stakeholders through a continuous collaboration. The primary components of DDD include:
- Domain: The problem space or the business area in which you are operating.
- Bounded Contexts: The boundaries within which a model is defined and applicable.
- Entities and Value Objects: Building blocks that comprise your domain model.
You can read more about the foundational principles of DDD in the book Domain-Driven Design: Tackling Complexity in the Heart of Software by Eric Evans.
Pitfall 1: Neglecting the Ubiquitous Language
One of the cornerstones of DDD is the concept of creating a Ubiquitous Language. This is a shared vocabulary used by both developers and non-technical stakeholders, ensuring clarity in communication.
Why It Matters
Failing to adopt a Ubiquitous Language can lead to misunderstandings and rework, causing business requirements to become muddled.
How to Overcome It
To solidify the Ubiquitous Language:
- Engage with Domain Experts: Schedule regular sessions with domain experts to refine the model.
- Document Terminology: Create and maintain a glossary of terms that everyone can refer to.
// Example: Defining an Entity using Ubiquitous Language
public class Customer {
private String name;
private String email;
// Constructor and Getters
public Customer(String name, String email) {
this.name = name;
this.email = email;
}
public String getName() {
return name;
}
public String getEmail() {
return email;
}
}
// In meetings, use "Customer" instead of terms like "user" or "client."
Pitfall 2: Misunderstanding Bounded Contexts
The idea of Bounded Contexts is fundamental in DDD. It's about establishing clear boundaries within which a particular domain model applies.
Why It Matters
When Bounded Contexts are not well-defined, it can lead to models that become bloated and tightly coupled, affecting maintainability and scalability.
How to Overcome It
- Explicitly Define Boundaries: Conduct workshops to identify distinct areas of your business logic.
- Allow for Context Mapping: Utilize context maps to show relationships between different Bounded Contexts.
// Example: Two Bounded Contexts represented as separate classes
public class Order {
private String orderId;
public Order(String orderId) {
this.orderId = orderId;
}
// Create order functionality here
}
public class Payment {
private String paymentId;
public Payment(String paymentId) {
this.paymentId = paymentId;
}
// Process payment functionality here
}
// Clearly distinguish between Order and Payment domains.
Pitfall 3: Ignoring Domain Events
Domain Events are crucial in DDD, as they provide a means to communicate changes within the domain. Ignoring them can lead to synchronization issues and tight coupling between components.
Why It Matters
Failing to use Domain Events can make your system inflexible. Changes in one part of your application may not be communicated effectively to others.
How to Overcome It
Implement an event-based architecture where relevant actions can emit events.
// Example: Emitting a Domain Event when an Order is placed
public class OrderService {
public void placeOrder(Order order) {
// Logic to place order
emitOrderPlacedEvent(order);
}
private void emitOrderPlacedEvent(Order order) {
// Logic for emitting an event
System.out.println("Order placed: " + order.getOrderId());
}
}
// Use the event to trigger workflows in other services.
Pitfall 4: Over-Engineering the Model
When developers dive deeply into DDD, they may fall into the trap of over-engineering, creating complex models that add little value.
Why It Matters
Over-engineered solutions lead to unnecessary complexity and increased technical debt.
How to Overcome It
- Start Simple: Begin with a basic model that captures essential business needs.
- Iterate and Refine: Continuously refine the model, making it more complex only when necessary.
// A simple Customer model without unnecessary complexities
public class SimpleCustomer {
private String name;
public SimpleCustomer(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
// Avoid adding features or abstractions that are not currently needed.
Pitfall 5: Disregarding Testing
Tests play a crucial role in DDD as they ensure the domain model behaves as expected. Neglecting testing is a common mistake that can lead to bugs and inconsistencies.
Why It Matters
Without proper tests, there's no assurance that the model reflects business rules accurately, leading to potential issues down the line.
How to Overcome It
- Adopt Test-Driven Development (TDD): Write tests first to clarify requirements and design.
- Accept Refactoring: Regularly refactor based on test results to enhance your domain model.
// Example: A simple JUnit test case for the Customer entity
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CustomerTest {
@Test
public void testCustomerCreation() {
Customer customer = new Customer("Alice", "alice@example.com");
assertEquals("Alice", customer.getName());
}
}
// Test-driven development ensures your model meets business requirements.
The Closing Argument
Domain-Driven Design offers a robust framework to tackle complex software projects. However, it's critical to be aware of its common pitfalls. By nurturing a Ubiquitous Language, defining clear Bounded Contexts, utilizing Domain Events, avoiding over-engineering, and maintaining a rigorous testing approach, you can harness the power of DDD effectively.
For further reading on DDD, consider exploring Eric Evans’ “Domain-Driven Design” and Vaughn Vernon’s “Implementing Domain-Driven Design.”
By understanding and overcoming these pitfalls, orgs can better realize the full potential of Domain-Driven Design, leading to more maintainable, flexible, and effective software solutions. Happy coding!