Navigating Team Dynamics as an Advanced Java Lead
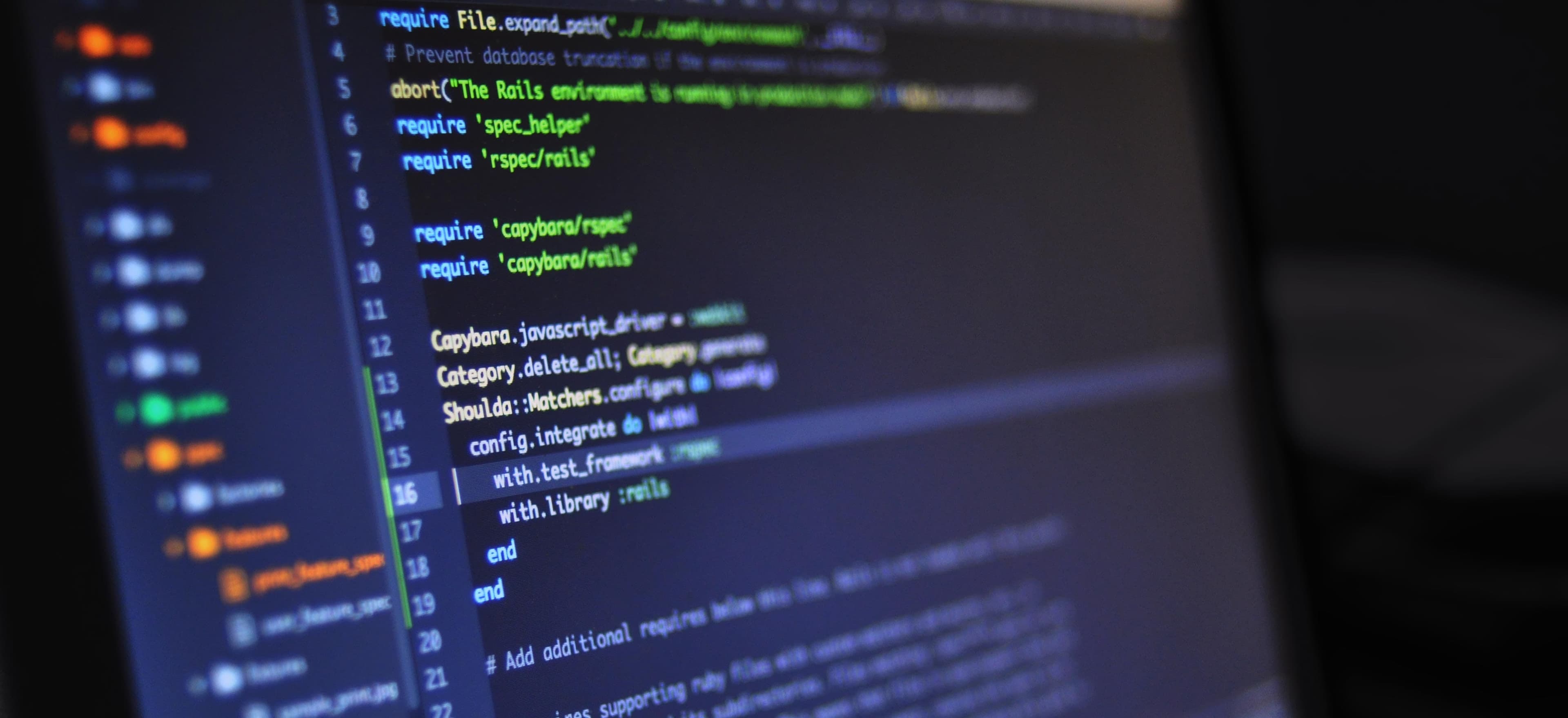
- Published on
Navigating Team Dynamics as an Advanced Java Lead
In the ever-evolving landscape of software development, leading a team, especially as an Advanced Java Lead, is both a challenge and an opportunity. Java is not just a programming language; it’s a robust ecosystem of frameworks and libraries, making effective leadership crucial for success. This guide aims to equip you with the knowledge and strategies to navigate team dynamics, fostering collaboration, improving productivity, and driving project successes.
Understanding Team Dynamics
Before delving into specific strategies, it's important to understand what team dynamics entails. Team dynamics refer to the behaviors, interactions, and relationships that shape how a team functions. They can be influenced by:
- Team Composition: Skills, personalities, and experiences that team members bring.
- Communication Styles: How information is shared and received.
- Leadership Approach: Your style impacts motivation and direction.
Navigating these dynamics effectively is essential for an Advanced Java Lead, as it directly contributes to the team’s performance and the quality of the deliverables.
The Role of an Advanced Java Lead
As an Advanced Java Lead, your responsibilities extend beyond technical guidance. Here are some key areas of focus:
- Technical Expertise: Mastery of Java SE, EE, Spring Framework, and associated technologies.
- Mentorship: Provide support and guidance to junior developers to foster their growth.
- Project Management: Oversee the project lifecycle, ensuring timelines and quality standards are met.
- Stakeholder Communication: Act as a bridge between team members and higher management.
Building a Cohesive Team
Assess Skill Sets
To effectively navigate team dynamics, start with an assessment of your team’s skill sets. This ensures you understand individual strengths and areas for improvement.
public class SkillSetAnalysis {
private Map<String, String> teamMembersSkills;
public SkillSetAnalysis() {
teamMembersSkills = new HashMap<>();
}
public void addMemberSkill(String memberName, String skill) {
teamMembersSkills.put(memberName, skill);
}
public void displaySkills() {
for (Map.Entry<String, String> entry : teamMembersSkills.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
Commentary: The SkillSetAnalysis
class represents the team’s skills. By analyzing the skills available, you can effectively allocate tasks and promote collaboration based on strengths.
Foster Open Communication
Encourage a culture of open communication and feedback. Regular check-ins, brainstorming sessions, and code reviews can significantly enhance team dynamics.
public class CommunicationChannel {
public void openChannel(String message) {
System.out.println("Communication Message: " + message);
}
public void receiveFeedback(String feedback) {
System.out.println("Feedback Received: " + feedback);
}
}
Commentary: The CommunicationChannel
class represents a simple model for sharing messages and receiving feedback. As an Advanced Java Lead, your role is to facilitate these interactions.
Conflict Resolution
Conflicts are inevitable in any team. Your approach to conflict resolution can shape team dynamics.
Identify Conflict Types
Recognize that conflicts can arise from various sources:
- Task-related Conflicts: Disagreements on methods or project requirements.
- Interpersonal Conflicts: Personality clashes and communication issues.
Mediate Effectively
Establish a neutral ground for discussion and encourage team members to express their viewpoints. Use the following structure to mediate effectively:
- Listen Actively: Ensure everyone feels heard.
- Facilitate Discussion: Use probing questions to clarify issues.
- Seek Common Ground: Identify shared goals to foster collaboration.
Empowering Team Members
Empowerment is key to enhancing team dynamics and productivity. Here’s how you can empower your Java development team:
Delegate Responsibilities
Effective delegation not only encourages ownership but also builds trust.
public class DelegateTask {
public void assignTask(String member, String task) {
System.out.println("Task: " + task + " assigned to " + member);
}
}
Commentary: The DelegateTask
class exemplifies how to communicate task assignments effectively. This approach highlights the importance of trust and autonomy in team dynamics.
Encourage Learning and Growth
Invest in your team's ongoing education. Encourage participation in workshops, online courses, and conferences. Provide resources like Java Tutorials by Oracle to deepen their understanding.
Embracing Agile Methodologies
As an Advanced Java Lead, embracing Agile methodologies can enhance team dynamics. Agile promotes collaboration, flexibility, and continuous improvement.
Implement Scrum
Scrum is an Agile framework that can help streamline your team’s workflow:
- Daily Standups: Quick check-ins to discuss progress and roadblocks.
- Sprint Planning: Collaborative sessions to outline upcoming tasks.
- Retrospectives: Reflect on what went well and areas for improvement.
Utilize Tools
Adopt tools that facilitate Agile practices. For example, tools like Jira or Trello can help track work and manage tasks effectively.
Maintaining Quality Assurance
As a Java Lead, you have the responsibility to ensure that your team’s output meets the highest quality standards. Integrating continuous integration and testing practices can significantly enhance quality.
Implement CI/CD Workflows
Continuous Integration (CI) and Continuous Deployment (CD) are crucial for maintaining product quality. Utilize tools like Jenkins to automate the testing and deployment process.
public class ContinuousIntegration {
public void runTests() {
System.out.println("Running automated tests...");
// Simulate test results
boolean testsPassed = true;
if (testsPassed) {
System.out.println("All tests passed successfully!");
} else {
System.out.println("Some tests failed. Please check the logs.");
}
}
}
Commentary: The ContinuousIntegration
class simulates automated testing. You can ensure that all features function correctly and maintain a high quality when working collaboratively on larger projects.
A Final Look
Navigating team dynamics as an Advanced Java Lead is an intricate blend of technical leadership and interpersonal skills. By understanding your team, fostering open communication, empowering individuals, and embracing Agile methodologies, you can create a collaborative environment that drives success.
In the words of Peter Drucker, "The most important thing in communication is hearing what isn't said." As a Java Lead, cultivate a culture where team members feel valued, understood, and motivated to contribute their best work.
Additional Resources
By investing time and effort into team dynamics, you not only improve project outcomes but also build a legacy of success that will resonate throughout your organization. Happy coding!
Checkout our other articles