Common Spring Data JPA Mistakes and How to Avoid Them
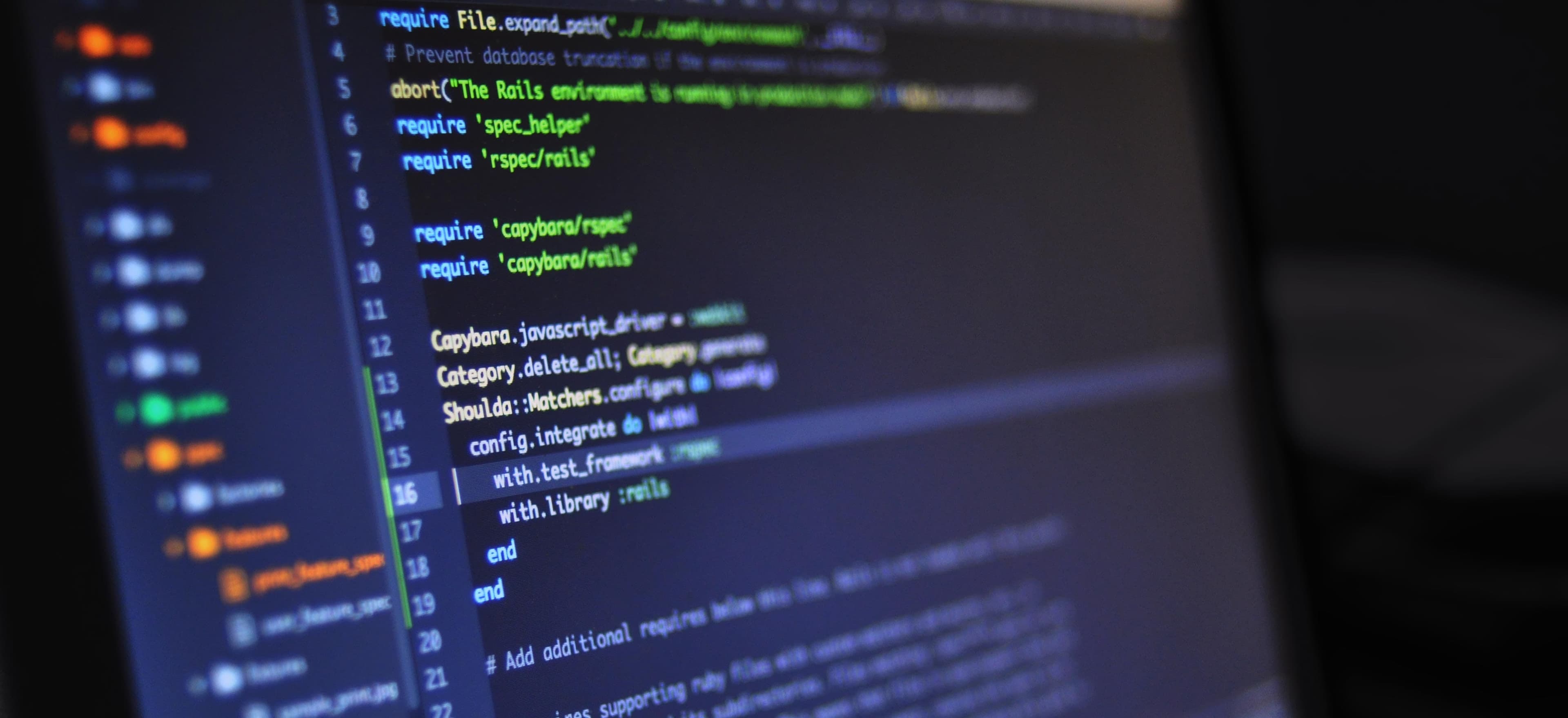
- Published on
Common Spring Data JPA Mistakes and How to Avoid Them
Spring Data JPA is a powerful tool for managing database interactions in Java applications. With its promise of simplicity and reduced boilerplate code, it's no wonder that developers often gravitate towards it. However, even seasoned developers can stumble upon pitfalls that can lead to performance degradation, inconsistent results, or even application failure.
In this post, we'll explore common mistakes made when using Spring Data JPA and how to avoid them. By following best practices, you can leverage the full potential of Spring Data JPA while mitigating risks.
1. Ignoring the Importance of Fetch Types
The Mistake
One of the most frequent issues developers face is neglecting the choice of fetch type for relationships, leading to inefficient database access patterns.
Explanation
Spring Data JPA provides two fetch strategies: FetchType.LAZY
and FetchType.EAGER
.
- FetchType.LAZY: Only fetches related entities when explicitly accessed.
- FetchType.EAGER: Fetches related entities immediately alongside the parent entity.
Choosing EAGER
fetching can lead to the infamous "N+1 Selects Problem" where, for a single parent entity, there may be a performance cost of executing multiple additional queries.
Solution
To avoid this issue, consider the most appropriate fetch type for your use case. Below is an example of how to define fetch type in your entity:
@Entity
public class Order {
@OneToMany(mappedBy = "order", fetch = FetchType.LAZY)
private List<OrderItem> items;
// Other fields and methods
}
Use FetchType.LAZY
for collections to avoid loading unnecessary data and to optimize performance.
2. Not Using Paging and Sorting
The Mistake
Another common oversight is bypassing the built-in support for paging and sorting, resulting in potentially overwhelming data loads that hinder user experience.
Explanation
When querying large datasets, it's essential to limit the amount of data retrieved to avoid long loading times and excessive memory usage. Spring Data JPA provides PagingAndSortingRepository
for this purpose.
Solution
Make use of built-in pagination capabilities. Here's an example of how to implement it:
public interface OrderRepository extends PagingAndSortingRepository<Order, Long> {
Page<Order> findAll(Pageable pageable);
}
To use it, you simply call:
Page<Order> page = orderRepository.findAll(PageRequest.of(0, 10));
List<Order> orders = page.getContent();
In this example, only 10 orders are fetched at a time, greatly improving efficiency.
3. Overusing @Transactional
The Mistake
Misusing the @Transactional
annotation can lead to hidden bugs or performance degradation.
Explanation
The @Transactional
annotation is a powerful feature that can manage transaction boundaries for data operations. However, incorrectly placing this annotation can result in unintended behavior such as multiple database calls, especially when applied at the wrong layer in your application.
Solution
Apply @Transactional
at the service layer instead of the repository layer. This way, you contain transaction scope without leaking into lower layers. An example might look like this:
@Service
public class OrderService {
@Autowired
private OrderRepository orderRepository;
@Transactional
public void placeOrder(Order order) {
orderRepository.save(order);
// Additional logic that may benefit from a transaction
}
}
By managing transactions at the service layer, you avoid potential pitfalls in your data access logic.
4. Not Leveraging Query Methods
The Mistake
Some developers overuse @Query
and miss out on the capabilities provided by Spring Data JPA's query generation from method names.
Explanation
Spring Data JPA allows you to create queries just by naming your methods correctly, which can lead to cleaner, more readable code without the need for a lot of boilerplate.
Solution
Instead of using @Query
for simple queries, leverage the naming convention. Here's an example:
public interface OrderRepository extends JpaRepository<Order, Long> {
List<Order> findByCustomerName(String customerName);
}
By naming the method appropriately, Spring automatically generates the required SQL query based on the method name, saving you from manual effort.
5. Forgetting to Handle Concurrency
The Mistake
Failing to implement proper concurrency controls can lead to lost updates or inconsistent data.
Explanation
In applications where multiple users are modifying data concurrently, it's critical to manage potential conflicts. Spring Data JPA provides optimistic locking to handle such scenarios.
Solution
Use @Version
to implement optimistic locking:
@Entity
public class Order {
@Id
private Long id;
@Version
private Long version; // for optimistic locking
// Other fields and methods
}
With this setup, Spring will automatically check the version when trying to update the entity. If the version does not match, an OptimisticLockException
will be thrown, indicating the data has changed since it was loaded.
6. Not Evaluating the N+1 Select Problem
The Mistake
Developers often overlook the N+1 select problem, which can severely impact performance.
Explanation
This occurs when the application executes N additional queries to retrieve child entities of a parent entity after initially fetching it. This can drastically slow down your application when working with large datasets.
Solution
Using EntityGraph
or JOIN FETCH
in your queries can help optimize data retrieval:
@Entity
public class Order {
@OneToMany(mappedBy = "order")
private List<OrderItem> items;
}
public interface OrderRepository extends JpaRepository<Order, Long> {
@Query("SELECT o FROM Order o JOIN FETCH o.items")
List<Order> findAllWithItems();
}
In this example, the JOIN FETCH
directive retrieves both orders and their related items in a single query, thus avoiding the N+1 issue.
Final Considerations
Incorporating Spring Data JPA into your Java application can drastically enhance productivity and simplify data management. However, as we've discussed, overlooking common pitfalls can lead to inefficient and buggy applications.
By being aware of these common mistakes and implementing the recommended practices, you can effectively harness the power of Spring Data JPA while ensuring performance and reliability.
For more information about Spring Data JPA, feel free to explore the official Spring Data documentation.
Key Takeaways
- Select appropriate fetch types.
- Utilize pagination and sorting.
- Manage transactions at the service layer.
- Leverage Spring's method naming for queries.
- Implement optimistic locking for concurrency.
- Be aware of and address the N+1 select problem.
By following these guidelines, you will be well on your way to building efficient and maintainable data-access layers using Spring Data JPA. Happy coding!
Checkout our other articles