How to Choose the Right Cryptographic Algorithm for Your Needs
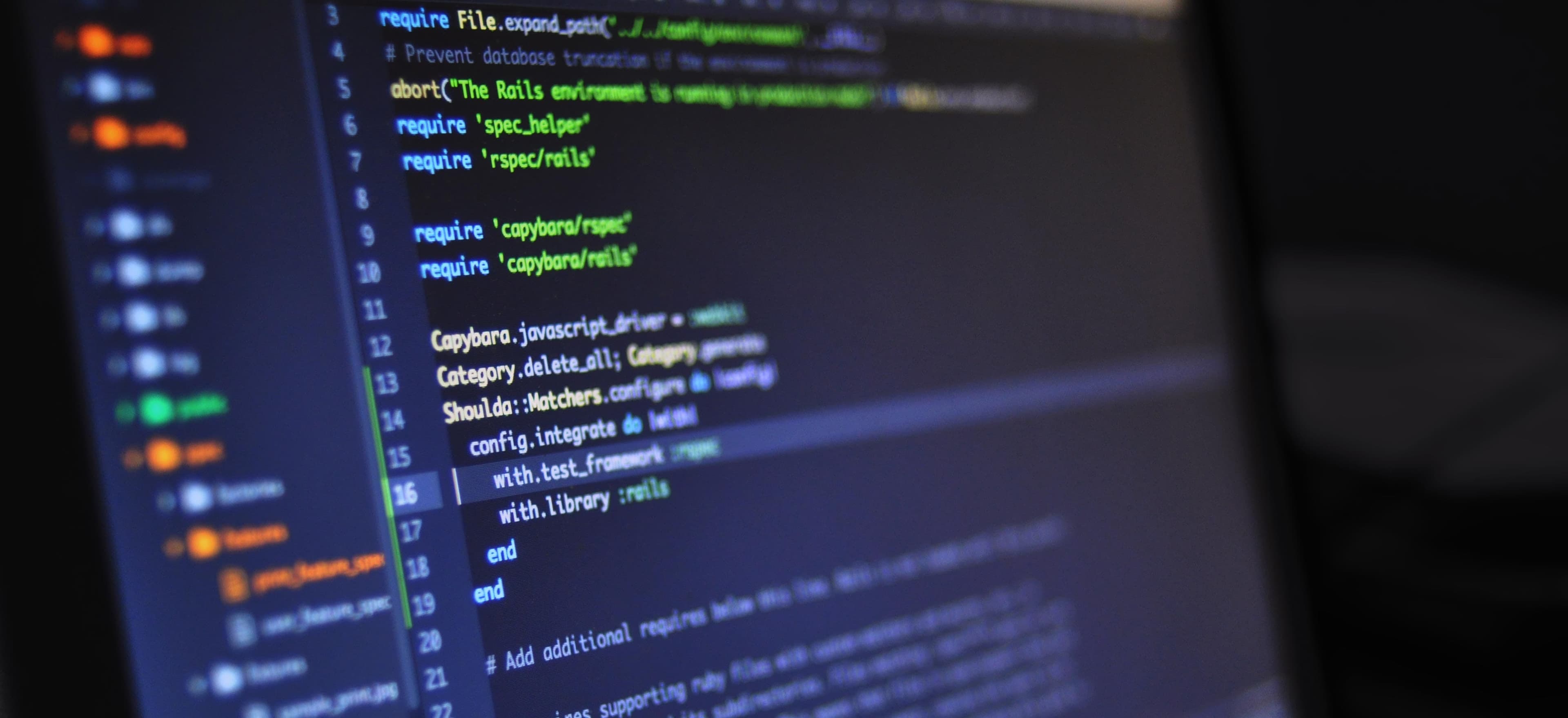
- Published on
How to Choose the Right Cryptographic Algorithm for Your Needs
In today's digital landscape, securing sensitive data is more important than ever. One vital component of this security is cryptography, a technique that ensures data confidentiality, integrity, and authenticity. With numerous cryptographic algorithms available, selecting the right one for your application can be bewildering. This blog post will guide you through the process of choosing the appropriate cryptographic algorithm by elucidating the core principles, common algorithms, and essential factors to consider.
Understanding Cryptography
Cryptography is the practice of securing communication and data through encoding techniques. It involves processes like encryption (converting plain text into ciphertext) and decryption (converting ciphertext back into plain text). There are two main types of cryptographic algorithms:
-
Symmetric Key Algorithms: Both encryption and decryption use the same key. This type of algorithm is generally faster and suited for encrypting large volumes of data. Common symmetric algorithms include AES (Advanced Encryption Standard) and DES (Data Encryption Standard).
-
Asymmetric Key Algorithms: This approach uses a pair of keys—public and private. The public key encrypts the data, while the private key decrypts it. This method is more secure for key distribution, as the private key does not need to be shared. RSA (Rivest-Shamir-Adleman) is a prominent asymmetric algorithm.
Factors to Consider When Choosing a Cryptographic Algorithm
1. Security Level Required
The primary objective of any cryptographic approach is to ensure that your data remains confidential and secure. Different algorithms offer varying security levels. For example:
- AES is widely recognized for its strength, offering key sizes of 128, 192, and 256 bits.
- RSA can provide strong security, but the key size typically ranges from 2048 to 4096 bits.
When selecting an algorithm, analyze how much security is needed based on the type of data you are protecting. Government agencies and financial institutions typically require higher security standards than personal or non-sensitive information.
2. Performance and Speed
Performance is a crucial factor, particularly if you’re working with large data sets or require real-time encryption. Symmetric algorithms generally outperform asymmetric ones. For instance, AES is far more efficient than RSA when encrypting substantial amounts of data.
Here's a sample code snippet illustrating AES encryption in Java:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class AESEncryptionExample {
public static void main(String[] args) throws Exception {
// Generating a new AES key
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(256); // You can also use 128 or 192 bits
SecretKey secretKey = keyGen.generateKey();
// Cipher instance for AES
Cipher cipher = Cipher.getInstance("AES");
String plainText = "Hello World";
// Encrypting the data
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] cipherText = cipher.doFinal(plainText.getBytes());
System.out.println("Encrypted Text: " + new String(cipherText));
}
}
Why use AES? It balances speed and security, making it a reliable choice for various applications.
3. Regulatory Requirements
Before selecting a cryptographic algorithm, be aware of legal and regulatory requirements. Specific sectors (such as finance and healthcare) may mandate particular standards or algorithms to ensure compliance. For example, the Financial Industry Regulatory Authority (FINRA) may require institutions to use AES for data protection.
4. Implementation Complexity
Complex algorithms may provide heightened security but can also introduce implementation challenges, leading to potential vulnerabilities. For many use cases, simpler algorithms are preferable. When implementing a cryptographic algorithm, choosing one that is well-documented and supported by existing libraries can ease the process.
Consider libraries such as Bouncy Castle or Java Cryptography Architecture (JCA). They provide a robust foundation for building applications without reinventing the wheel.
5. Community and Peer Support
Cryptography is a continually evolving field, meaning some algorithms can become outdated or suffer vulnerabilities over time. Therefore, consider the community support behind an algorithm. Frequently updated libraries and active user groups can provide insights and updates, ensuring you're using the best practices and the most secure algorithms.
Overview of Popular Cryptographic Algorithms
To give you a better understanding, here's a brief overview of some common cryptographic algorithms:
-
AES
- Type: Symmetric
- Key Size: 128, 192, 256 bits
- Use Cases: Securing data in storage and transmission.
-
RSA
- Type: Asymmetric
- Key Size: 2048 bits (minimum recommended)
- Use Cases: Secure data transmission, digital signatures.
-
SHA (Secure Hash Algorithm)
- Type: Hash Function
- Key Size: Variable (SHA-256, SHA-512)
- Use Cases: Digital signatures, integrity checks.
-
ECC (Elliptic Curve Cryptography)
- Type: Asymmetric
- Key Size: Smaller compared to RSA for equivalent security
- Use Cases: Mobile and embedded devices; SSL/TLS certificates.
Example: Implementing RSA in Java
Now, let's look at an example of generating RSA keys and encrypting data.
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PublicKey;
import javax.crypto.Cipher;
public class RSAExample {
public static void main(String[] args) throws Exception {
// Generating RSA Key Pair
KeyPairGenerator keyGen = KeyPairGenerator.getInstance("RSA");
keyGen.initialize(2048);
KeyPair keyPair = keyGen.generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
// Encrypting data
String plainText = "Hello RSA";
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] cipherText = cipher.doFinal(plainText.getBytes());
System.out.println("Encrypted Text: " + new String(cipherText));
}
}
Why is RSA significant? It allows secure data transmission without requiring a shared secret key, simplifying key exchange issues.
The Closing Argument
Choosing the right cryptographic algorithm is a foundational skill in any developer's toolkit. It requires a thorough understanding of various algorithms, their strengths, weaknesses, and the context in which they are utilized. Always consider the security level, performance, regulatory requirements, complexity, and community support.
For further reading on cryptography and Java implementations, check out:
By following these guidelines, you can ensure your applications are built on robust security practices, ultimately protecting your data and maintaining user trust.