Unlocking Java: The Hidden Power of Advanced Synchronizers
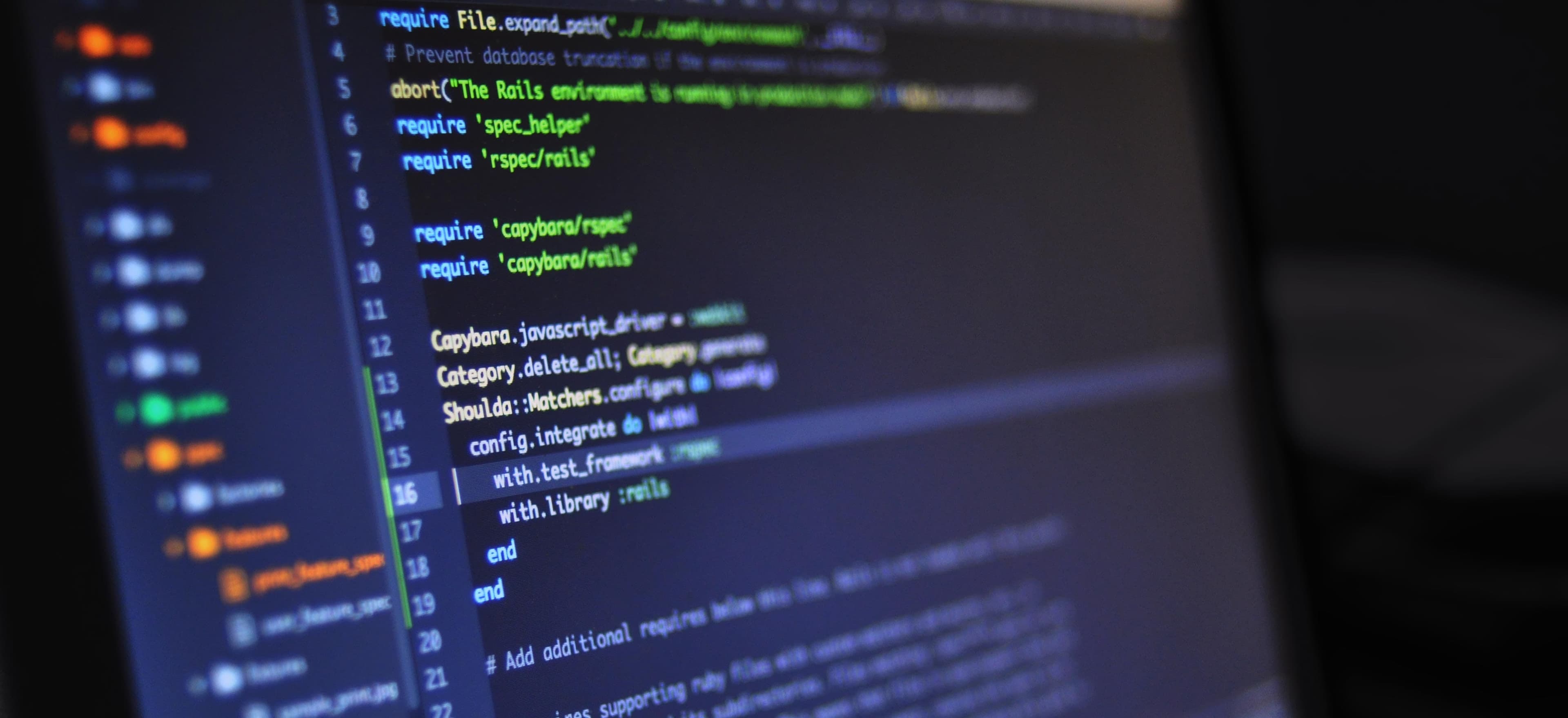
- Published on
Unlocking Java: The Hidden Power of Advanced Synchronizers
Java is a powerful programming language, known for its versatility and strong concurrency support. While many developers are familiar with basic synchronization concepts, Java's advanced synchronization constructs offer tools that can significantly simplify thread management in multi-threaded applications. In this blog post, we'll explore these advanced synchronizers, including ReentrantLock
, Semaphore
, CountDownLatch
, and CyclicBarrier
. By the end, you'll have a solid understanding of these tools and how to leverage their power in your Java applications.
Why Advanced Synchronizers?
Java provides several built-in mechanisms to handle synchronization, such as the synchronized
keyword and the volatile
modifier. However, these constructs have their limitations, particularly in complex situations involving multiple threads and intricate interactions. Advanced synchronizers help overcome these limitations, providing greater flexibility, improved performance, and enhanced readability.
Key Concepts
Before we delve into the code, let's discuss some key concepts that underpin Java's advanced synchronizers:
- Mutual Exclusion: Ensuring that only one thread can access a resource at a time.
- Blocking: Making threads wait when a resource is unavailable.
- Signaling: Allowing threads to communicate about state changes.
Now, let’s examine each advanced synchronizer in detail.
ReentrantLock
Overview
ReentrantLock
is a part of the java.util.concurrent.locks
package and provides a more powerful and flexible locking mechanism than the traditional synchronized
keyword. It allows greater control over thread synchronization, including the ability to acquire and release locks explicitly.
Example
import java.util.concurrent.locks.ReentrantLock;
public class ReentrantLockExample {
private final ReentrantLock lock = new ReentrantLock();
private int counter = 0;
public void increment() {
lock.lock(); // Acquire the lock
try {
counter++; // Critical section
} finally {
lock.unlock(); // Release the lock
}
}
public int getCounter() {
return counter;
}
}
Commentary
This example demonstrates a simple counter implementation using ReentrantLock
. Here’s why this code is effective:
- Granular Control: The explicit lock acquisition (
lock.lock()
) and release (lock.unlock()
) allow us to define precisely when a thread can enter the critical section, providing more control than asynchronized
method. - try-finally Block: Wrapping the critical section in a
try
block ensures that the lock is always released, preventing deadlock scenarios.
If you want to dive deeper into locks, refer to the official Lock Interface Documentation.
Semaphore
Overview
A Semaphore
maintains a set of permits. Threads can acquire permits before proceeding and release them when they've finished their work. This is useful for controlling access to a resource pool.
Example
import java.util.concurrent.Semaphore;
public class SemaphoreExample {
private final Semaphore semaphore = new Semaphore(2); // At most 2 permits
public void accessResource() {
try {
semaphore.acquire(); // Acquire a permit
System.out.println(Thread.currentThread().getName() + " accessing resource");
Thread.sleep(2000); // Simulate resource access time
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
} finally {
semaphore.release(); // Release the permit
}
}
}
Commentary
In this example, we limit access to a shared resource using a Semaphore
:
- Permit Management: The semaphore is initialized with a maximum of 2 permits, meaning only 2 threads can access the resource concurrently.
- Acquire and Release: Each thread acquires a permit before accessing the resource, and it releases the permit afterward, ensuring controlled access.
For a comprehensive understanding of semaphores, check out the Semaphore Documentation.
CountDownLatch
Overview
CountDownLatch
enables one or more threads to wait until a set of operations in other threads completes. It's particularly useful for implementing waiting strategies in multithreaded applications.
Example
import java.util.concurrent.CountDownLatch;
public class CountDownLatchExample {
private final CountDownLatch latch = new CountDownLatch(3); // Wait for 3 tasks
public void task() {
try {
Thread.sleep(1000); // Simulate task
System.out.println(Thread.currentThread().getName() + " completed task");
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
} finally {
latch.countDown(); // Decrement the count
}
}
public void waitForCompletion() {
try {
latch.await(); // Wait until counter reaches zero
System.out.println("All tasks completed!");
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
Commentary
This example shows how to use CountDownLatch
to wait for multiple tasks to complete:
- Task Completion Tracking: Each task decrements the latch count when it completes, allowing the main thread to wait for all tasks.
- Simplicity: The
await
method blocks the main thread until the countdown reaches zero, making implementation straightforward.
For more details, please visit the CountDownLatch Documentation.
CyclicBarrier
Overview
CyclicBarrier
is another synchronization aid that allows a set of threads to all wait for one another to reach a common barrier point, facilitating the coordinated execution of threads.
Example
import java.util.concurrent.CyclicBarrier;
public class CyclicBarrierExample {
private final CyclicBarrier barrier = new CyclicBarrier(3, () -> System.out.println("All threads are ready!"));
public void task() {
try {
System.out.println(Thread.currentThread().getName() + " is preparing");
Thread.sleep(1000); // Simulate work
barrier.await(); // Wait at the barrier
System.out.println(Thread.currentThread().getName() + " started execution");
} catch (Exception e) {
Thread.currentThread().interrupt();
}
}
}
Commentary
In this example, we utilize a CyclicBarrier
to synchronize multiple threads:
- Barrier Action: When all threads reach the barrier, a specified action (a runnable) is executed, providing a great way to perform a follow-up action once all threads are ready.
- Reusability: Once the barrier is tripped, it can be reused, allowing new sets of threads to synchronize repeatedly.
For further knowledge, check the CyclicBarrier Documentation.
Closing the Chapter
Java's advanced synchronizers are powerful tools that significantly enhance your ability to manage complex concurrency scenarios. By understanding and effectively using constructs like ReentrantLock
, Semaphore
, CountDownLatch
, and CyclicBarrier
, developers can write cleaner, safer, and more efficient multithreaded applications.
If you're looking to optimize your Java applications, consider integrating these advanced synchronizers into your code. Remember, effective synchronization is key in multi-threaded programming, allowing you to prevent issues like race conditions and deadlocks.
For more information about concurrency in Java, check the Java Concurrency Tutorial.
Ready to harness the power of advanced synchronizers? Dive in, experiment, and unlock the hidden potential of Java concurrency today!