Simplifying Deployment Orchestration for Java Microservices
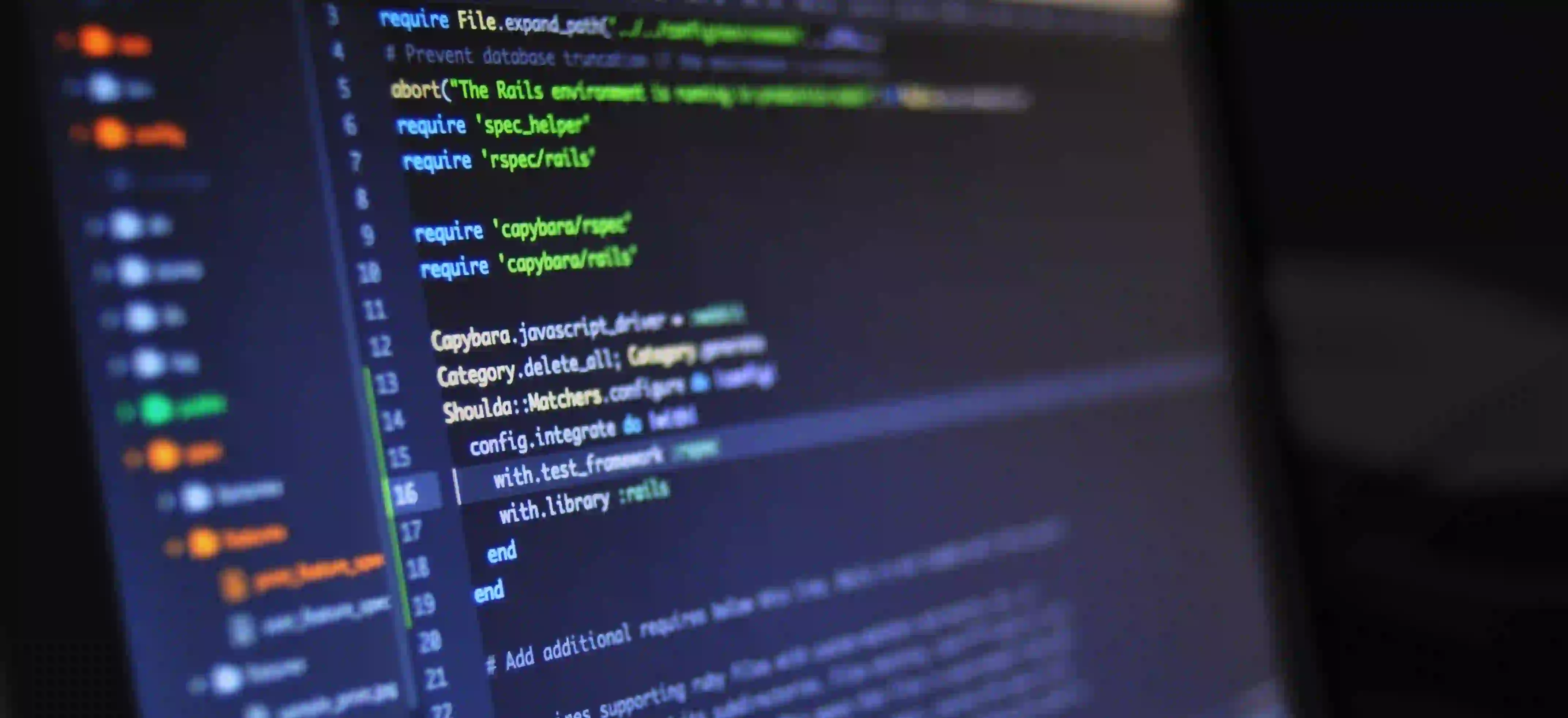
Simplifying Deployment Orchestration for Java Microservices
In today's software ecosystems, microservices have emerged as a powerful architectural style that allows organizations to build scalable and maintainable systems. One of the pivotal challenges in building a microservices architecture is deployment orchestration. This article will present methods to simplify deployment orchestration in Java microservices, enhancing both developer productivity and system reliability.
Understanding Deployment Orchestration
Deployment orchestration is the automated configuration, management, and coordination of complex software deployments. In the context of Java microservices, orchestration ensures that various service components interact seamlessly while adhering to the desired state and performance metrics.
Why is Orchestration Important?
- Scalability: Microservices are built to be scaled independently. Orchestration helps you automate scaling processes based on demand.
- Resilience: Automatic failover and recovery mechanisms can be implemented to reduce downtime.
- Isolation: Changes to individual services do not affect the entire system, aiding in minimizing risks during rollouts.
Common Tools for Orchestration
Before diving into simplification techniques, it's essential to know some common orchestration tools for Java microservices:
- Kubernetes: An industry-leading platform for automating deployment, scaling, and operation of application containers.
- Docker Swarm: Native clustering for Docker, allowing you to manage a cluster of Docker Engines.
- Spring Cloud: Offers tools for developers to quickly build some of the common patterns in distributed systems (e.g., configuration management, service discovery).
Simplifying Orchestration with These Techniques
1. Use a Unified Configuration Management System
In the world of microservices, managing configurations for each service can become convoluted. To address this, consider utilizing a unified configuration management system.
import org.springframework.cloud.context.config.annotation.RefreshScope;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RefreshScope
public class ConfigController {
@Value("${message:Default message}")
private String message;
@GetMapping("/message")
public String getMessage() {
return message;
}
}
In the above example, the @Value
annotation dynamically pulls configuration values, and the RefreshScope
annotation allows for runtime refresh of the configurations.
Why this matters: It simplifies configuration management and offers a consolidated approach for configuration across multiple microservices.
2. Automate Service Discovery
In a microservices architecture, various services need to communicate with each other. This is where service discovery comes in. Utilize tools like Spring Cloud Netflix Eureka for automating service registration and discovery.
@EnableEurekaServer
@SpringBootApplication
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
This code snippet demonstrates setting up a simple Eureka server.
Why this is effective: It reduces the overhead of hardcoding service endpoints, allowing services to discover their peers dynamically.
3. Leverage Containerization
Containerization via Docker allows you to encapsulate your Java microservices along with their dependencies, leading to a consistent deployment environment.
FROM openjdk:11-jre-slim
COPY target/myapp.jar /app/myapp.jar
ENTRYPOINT ["java", "-jar", "/app/myapp.jar"]
In this Dockerfile, we define a simple image for our Java application.
Why this practice is vital: It ensures that microservices behave consistently across different environments (local development, testing, and production).
4. CI/CD Pipeline Integration
Implementing a Continuous Integration and Continuous Deployment (CI/CD) pipeline significantly simplifies the deployment process. Tools like Jenkins or GitHub Actions can automate build, test, and deployment processes.
An example of a basic Jenkinsfile might look like this:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Deploy') {
steps {
sh 'docker build -t myapp .'
sh 'docker run -d -p 8080:8080 myapp'
}
}
}
}
Why CI/CD matters: Automation reduces human errors and allows for frequent deployments, enabling a more Agile development approach.
5. Implement Circuit Breakers
When microservices call each other, they can become susceptible to failures. By implementing circuit breakers, you prevent cascading failures and improve the resilience of your system. The following code shows how to implement a circuit breaker using Spring Cloud Circuit Breaker:
@RestController
public class MyServiceController {
@Autowired
private MyClient myClient;
@CircuitBreaker
@GetMapping("/fallback")
public String getData() {
return myClient.callService();
}
}
Why this is essential: Circuit breakers help in maintaining the stability of your application by preventing calls to failing services, allowing for graceful degradation of features.
Final Thoughts
In summary, simplifying deployment orchestration for Java microservices is not just about tools and frameworks; it also involves adopting best practices that foster productivity and resilience. By embracing unified configuration management, automating service discovery, containerization, CI/CD practices, and implementing circuit breakers, developers can create a more manageable microservices architecture.
For further reading on microservices and orchestration, you might find the following resources helpful:
By applying these principles, you will not only streamline your deployment orchestration process but also contribute to the overall reliability and efficiency of your microservices architecture. Happy coding!