Mastering Circuit Breakers: Avoiding Hystrix Pitfalls
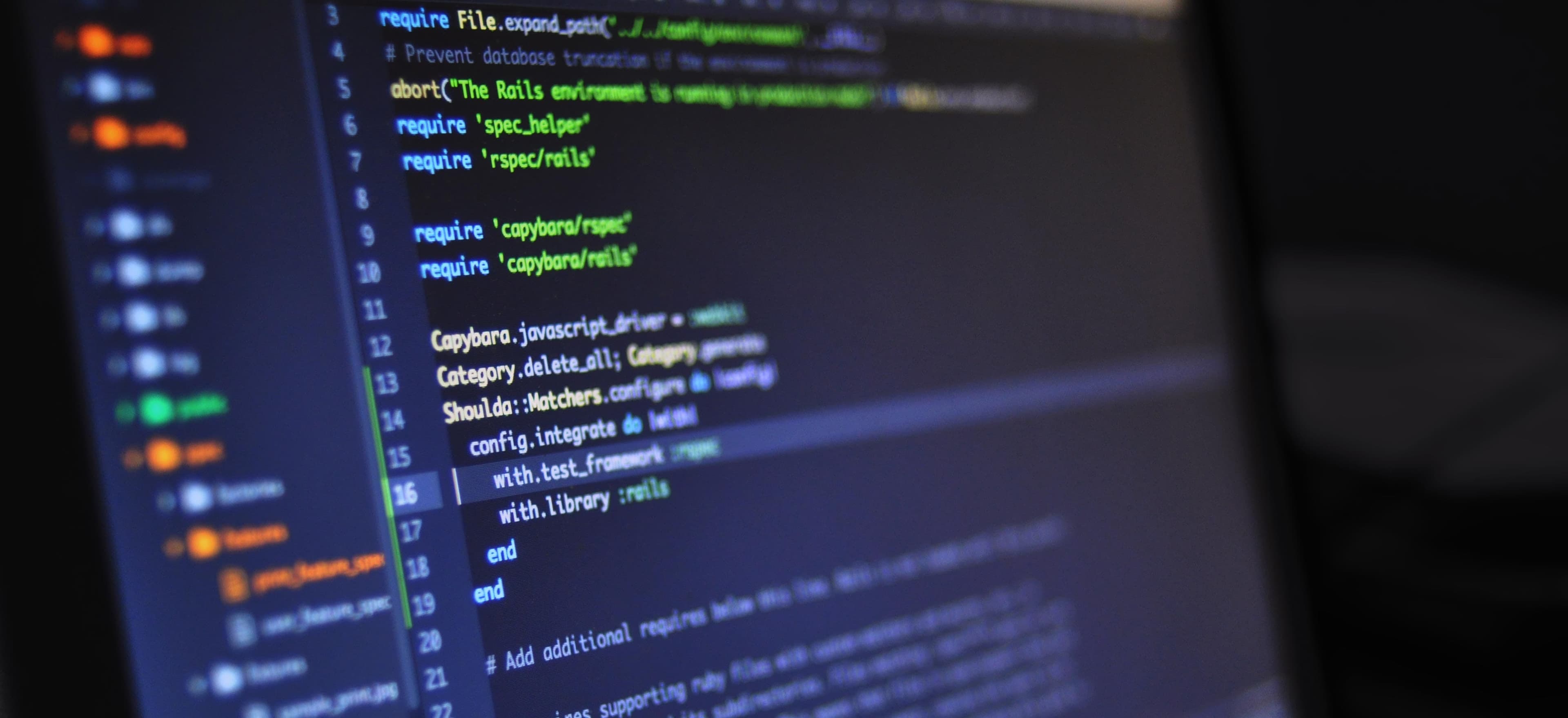
- Published on
Mastering Circuit Breakers: Avoiding Hystrix Pitfalls
In the world of microservices, resilience is key. Applications must handle failures gracefully to maintain a smooth user experience. This is where circuit breakers come into play. They’re a vital pattern for managing system resources and dealing with potential service failures. In this blog post, we’ll explore the concept of circuit breakers, shine a spotlight on Hystrix, and discuss common pitfalls and best practices.
What Is a Circuit Breaker?
A circuit breaker is a design pattern used to detect failures and encapsulate the logic of preventing a cascade failure. When a certain threshold of failures is reached, the circuit breaker trips to prevent further attempts to perform the action until a specified period elapses. This allows the system to rest and recover before testing the service again.
!Circuit Breaker Pattern
How Does a Circuit Breaker Work?
Typically, a circuit breaker includes three states:
-
Closed: The circuit breaker is operational, and requests can pass through. If failures exceed a predefined threshold, it transitions to the Open state.
-
Open: The circuit breaker is open, and requests are not allowed to pass through. Instead, a predefined fallback method is executed. After a specific timeout, the circuit breaker transitions to the Half-Open state.
-
Half-Open: In this state, it allows a limited number of requests to pass through. If they succeed, the circuit breaker transitions back to Closed. If they fail, it returns to Open.
Implementing Circuit Breakers in Java with Hystrix
Hystrix, developed by Netflix, is a popular library for implementing the circuit breaker pattern in Java applications. It provides an easy way to define fallback logic and manage timeouts in your applications, enhancing the robustness of microservices.
Getting Started with Hystrix
To use Hystrix, add its dependencies to your Maven project:
<dependency>
<groupId>com.netflix.hystrix</groupId>
<artifactId>hystrix-core</artifactId>
<version>1.5.18</version>
</dependency>
<dependency>
<groupId>com.netflix.hystrix</groupId>
<artifactId>hystrix-metrics-event-stream</artifactId>
<version>1.5.18</version>
</dependency>
A Simple Hystrix Command
Let's implement a simple service to demonstrate how to use Hystrix. Suppose we have a service that interacts with an external API which may be prone to failures:
import com.netflix.hystrix.HystrixCommand;
import com.netflix.hystrix.HystrixCommandGroupKey;
public class ApiService extends HystrixCommand<String> {
private final String apiUrl;
public ApiService(String apiUrl) {
super(HystrixCommandGroupKey.Factory.asKey("ApiServiceGroup"));
this.apiUrl = apiUrl;
}
@Override
protected String run() throws Exception {
// Simulate a call to an external API
if (Math.random() > 0.5) {
throw new RuntimeException("Failed to fetch data from API");
}
return "Success: Data from " + apiUrl;
}
@Override
protected String getFallback() {
return "Fallback: Unable to fetch data, please try again later.";
}
}
Why Hystrix?
- Robustness: By providing a fallback mechanism, your application can still work even if the external API fails.
- Monitoring: Hystrix provides metrics, allowing you to track service degradation over time.
- Isolation: By using Hystrix, you can ensure one service's failure doesn't cause the entire application to collapse.
Testing the Circuit Breaker
Let’s see how we can test our ApiService
:
public class Main {
public static void main(String[] args) {
ApiService apiService = new ApiService("http://example.com/api");
for (int i = 0; i < 10; i++) {
System.out.println(apiService.execute());
}
}
}
Pitfalls and Best Practices
While Hystrix is powerful, it comes with its own unique challenges and pitfalls. Understanding these can help you avoid common missteps.
1. Overusing Circuit Breakers
While it can be tempting to place circuit breakers around every external call, overusing them can lead to complexities that may hinder your system rather than help it. Use circuit breakers judently where the cost of failure warrants their implementation.
2. Improper Configuration
Many developers underestimate the importance of tuning configuration settings such as timeout and fallback logic. An improperly configured circuit breaker can either trip too early (leading to unnecessary failures) or too late (compromising system availability).
Here’s how you can configure timeouts in Hystrix:
@Override
protected String run() throws Exception {
// Set a timeout of 1 second
HystrixCommand.Setter.withGroupKey(HystrixCommandGroupKey.Factory.asKey("ApiServiceGroup"))
.andCommandPropertiesDefaults(HystrixCommandProperties.Setter()
.withExecutionTimeoutInMilliseconds(1000));
// Simulated long-running external API call
}
3. Ignoring Metrics
Hystrix comes with powerful metrics that should not be ignored. Failing to monitor your circuit breakers means ignoring the health of your application. Use Hystrix Dashboard or integrate with tools like Prometheus to keep an eye on highlights such as failure rates or latency.
4. Complex Fallbacks
Fallback methods should be simple and quick to execute. Avoid placing business logic inside your fallback methods. This ensures a smoother recovery and leaves the fallback as a last resort.
The Last Word
Circuit breakers, especially with the assistance of Hystrix, are crucial for building resilient microservice architectures. Understanding the functionality and the pitfalls of Hystrix is essential to leverage its full potential. As you implement circuit breakers, keep an eye on configuration, the necessity of usage, metrics, and simple fallback strategies.
For further insight on building resilient microservices, check out these resources:
Learn from these pitfalls, be strategic in your implementation, and master circuit breakers to create robust, reliable systems! Happy coding!
Checkout our other articles