Mastering Non-Blocking Data in Java: Key Challenges Explained
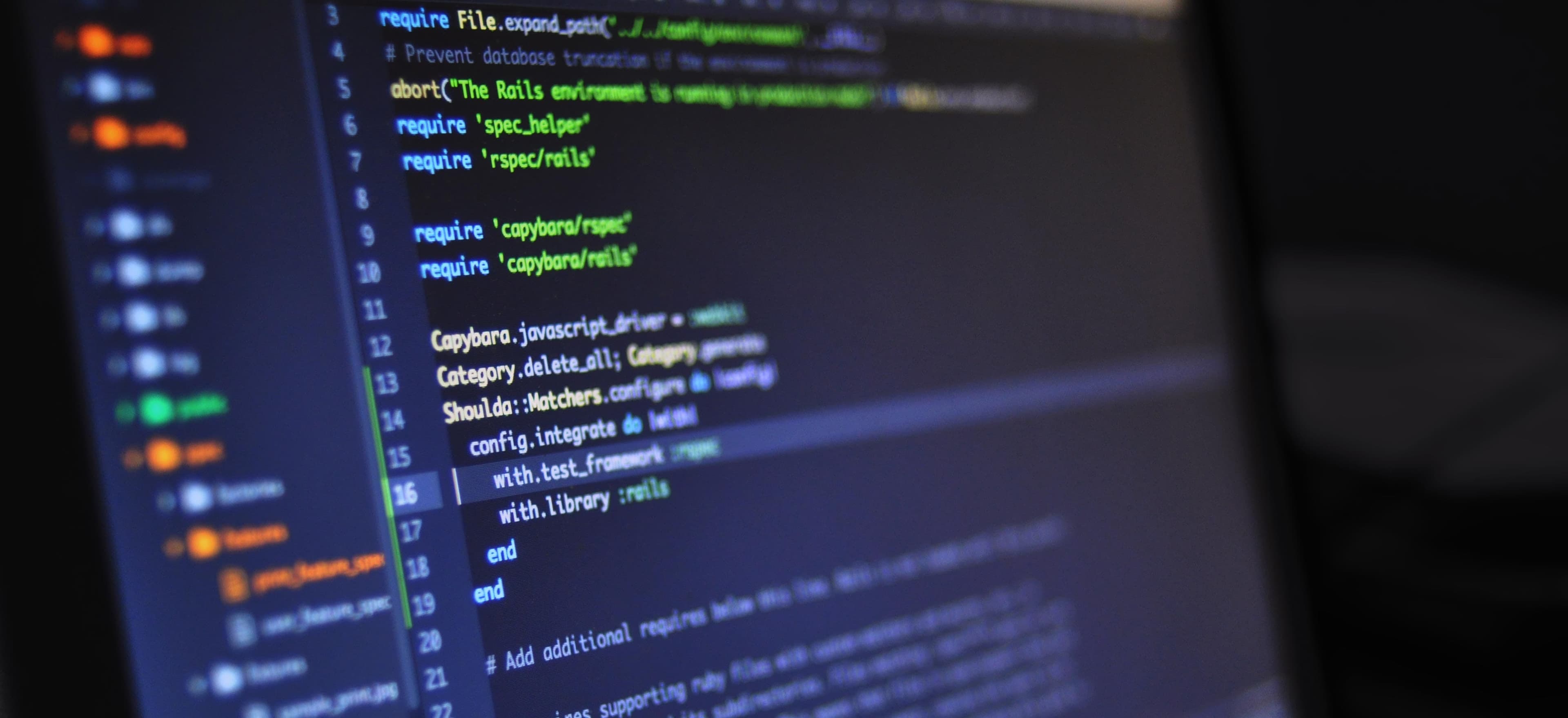
- Published on
Mastering Non-Blocking Data in Java: Key Challenges Explained
In the realm of concurrent programming, non-blocking data structures have emerged as a powerful technique for designing highly efficient applications. Java offers various built-in classes and libraries that facilitate non-blocking practices, but mastering them presents its own unique challenges. This post will delve into the concept of non-blocking data structures in Java, examine their advantages, and discuss the key hurdles developers encounter when implementing them.
What Are Non-Blocking Data Structures?
Non-blocking data structures allow multiple threads to access and modify shared data without traditional locks, minimizing thread contention and "stopping" issues. This is primarily achieved through atomic operations and advanced concurrency controls. These structures can be particularly beneficial in multi-threaded environments where performance and responsiveness are crucial.
Key Benefits of Non-Blocking Data Structures
-
Increased Performance: Non-blocking algorithms often outperform their blocking counterparts under high contention because they avoid the overhead of context switching and locking.
-
Better Responsiveness: Applications leveraging non-blocking data structures can maintain a high level of responsiveness, especially in user-facing applications.
-
Avoidance of Deadlocks: Because there are no locks to manage, the risk of deadlocks is effectively eliminated.
-
Scalability: Non-blocking structures can handle more threads concurrently, making them highly suitable for modern multi-core processors.
Types of Non-Blocking Data Structures in Java
Java provides a plethora of built-in non-blocking data structures. Some of the most commonly used include:
- Atomic Variables: Classes such as
AtomicInteger
,AtomicBoolean
, andAtomicReference
. - Concurrent Collections: Such as
ConcurrentHashMap
,CopyOnWriteArrayList
, andConcurrentLinkedQueue
.
Each of these structures and classes utilizes atomic operations under the hood to achieve non-blocking behavior.
Example of Atomic Variable Usage
Here's a basic example using AtomicInteger
to illustrate how non-blocking operations work in Java:
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicExample {
public static void main(String[] args) {
AtomicInteger atomicCounter = new AtomicInteger(0);
// Simulate multiple threads incrementing the counter
for (int i = 0; i < 5; i++) {
new Thread(() -> {
for (int j = 0; j < 1000; j++) {
atomicCounter.getAndIncrement();
}
}).start();
}
// Wait for threads to complete
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
System.out.println("Final counter value: " + atomicCounter.get());
}
}
Code Commentary
- AtomicInteger: The
AtomicInteger
class provides methods that ensure atomic operations, so even when multiple threads read and write to the counter simultaneously, there won't be any race condition. - getAndIncrement(): This method returns the current value and then increments it atomically. Thus, you ensure that your increments happen without interference from other threads.
Challenges in Non-Blocking Data Structures
While non-blocking algorithms offer significant advantages, implementing them is fraught with challenges. Let's explore some of these challenges.
1. Complexity of Algorithms
Non-blocking algorithms can be much harder to understand and implement than their blocking counterparts. For instance, compare the simplicity of a synchronized method to the intricacies involved in implementing a non-blocking stack.
2. Debugging Difficulty
Debugging multi-threaded applications is notoriously challenging. When using non-blocking data structures, the complexity escalates. Race conditions can occur without clear indications of issues, often leading to unpredictable behavior.
3. Performance Tuning
Non-blocking algorithms may not always outperform blocking algorithms. Under low contention scenarios, the overhead introduced by atomic operations can yield worse performance. Developers must consider the context in which they deploy these structures to determine the optimal design choice.
4. Limited Use Cases
Some problems are inherently blocking in nature. For instance, certain data acquisition protocols may demand a locking mechanism, thus making non-blocking data structures less useful.
5. Learning Curve
Non-blocking data structures often rely on concepts such as the Compare-And-Swap (CAS) operation. Familiarizing yourself with these concepts requires time and effort, particularly for those who are used to imperative locking mechanisms.
Best Practices for Using Non-Blocking Data Structures
-
Choose the Right Structure: Understand the expected operational load and choose between blocking and non-blocking structures accordingly.
-
Keep It Simple: Do not over-engineer your solution. If a simple blocking approach suffices, it may reduce complexity and debugging effort.
-
Profile and Measure: Use profiling tools to measure the performance of your application. This data can guide you in making informed choices about non-blocking versus blocking structures.
-
Test Extensively: Create unit and integration tests to verify thread safety and correctness in concurrent scenarios.
The Bottom Line
Mastering non-blocking data structures in Java can significantly enhance the performance and scalability of your applications. However, navigating the challenges associated with these structures requires a clear understanding of their underpinnings and potential pitfalls.
By pursuing best practices and leveraging the built-in atomic classes and concurrent collections provided by the Java standard library, developers can construct efficient and responsive applications. As always, consider your specific use case carefully when deciding between non-blocking and traditional locking mechanisms.
For further reading on concurrent programming in Java, consider exploring:
- Java Concurrency in Practice
- The Java™ Tutorials - Concurrency
By cultivating a deep understanding of non-blocking paradigms, you can effectively harness the power of Java's concurrency utilities to deliver top-notch, high-performance applications. Happy coding!
Checkout our other articles