Troubleshooting WildFly Swarm Application Debugging in NetBeans
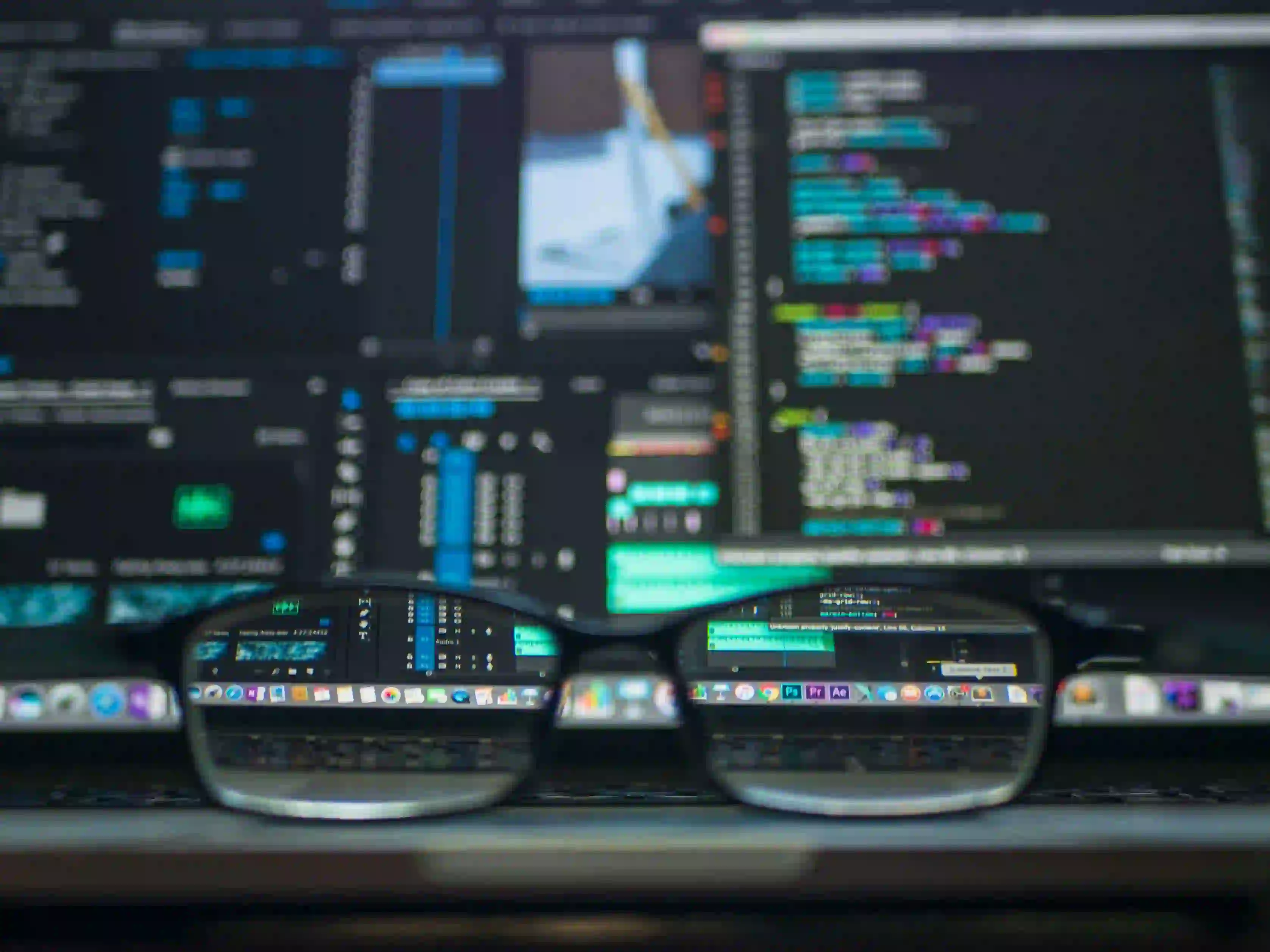
Troubleshooting WildFly Swarm Application Debugging in NetBeans
WildFly Swarm is a fascinating framework that allows developers to create microservices with the WildFly application server. One of the pain points developers often encounter when working with this platform is debugging their applications in an Integrated Development Environment (IDE) like NetBeans. In this blog post, we will explore effective strategies for troubleshooting WildFly Swarm applications in NetBeans, ensuring a smoother debugging experience.
Understanding WildFly Swarm
Before diving into troubleshooting, let’s briefly discuss what WildFly Swarm is. It is a lightweight variant of the WildFly application server designed for containerized microservices. Combining the robust capabilities of WildFly with frameworks like JAX-RS and CDI, WildFly Swarm provides a unique environment for developing enterprise applications.
Setting Up Your Environment
Proper setup of your development environment is crucial for effective debugging. Below are the steps to ensure that your NetBeans IDE is correctly configured for WildFly Swarm:
Prerequisites
- Java JDK: Ensure that you have the Java Development Kit (JDK) installed.
- NetBeans IDE: Download and install the latest version of NetBeans that supports your JDK.
- WildFly Swarm: Add WildFly Swarm dependencies to your project using Maven or Gradle.
Example Maven Configuration
If you are using Maven, include the following dependencies in your pom.xml
:
<dependency>
<groupId>org.wildfly.swarm</groupId>
<artifactId>swarm-core</artifactId>
<version>latest.version</version>
</dependency>
Configuring WildFly Swarm in NetBeans
After ensuring that all prerequisites are in place, set up WildFly Swarm:
- Create or Open a Project: In NetBeans, create a new Maven project or open your existing WildFly Swarm project.
- Add the WildFly Swarm Plugin:
Add the plugin to your
pom.xml
to configure the built-in JBoss CLI commands:
<plugin>
<groupId>org.wildfly.swarm</groupId>
<artifactId>wildfly-swarm-maven-plugin</artifactId>
<version>latest.version</version>
<configuration>
<exec>
<executable>run</executable>
</exec>
</configuration>
</plugin>
This plugin will help you create an executable JAR that can be launched with JVM arguments necessary for debugging.
Debugging WildFly Swarm
Starting Your Application in Debug Mode
One of the essential steps for debugging is to run your application in debug mode. You can do this using the command line or directly within NetBeans.
To start your application in debug mode via the command line, use:
mvn swarm:run -Dswarm.debug=true
Setting Up Remote Debugging in NetBeans
Once your WildFly Swarm application is running in debug mode, follow these steps to set up remote debugging:
-
Configure Breakpoints: Open your Java classes in NetBeans and set breakpoints where you want the execution to stop.
-
Create a Debug Configuration:
- Go to the "Run" menu and select "Set Project Configuration".
- Choose "Customize". In the "Debugger" tab, add a remote server.
- Use
localhost
and the debug port you specified in your JVM arguments (e.g.,8787
).
-
Attach to Debugger: With your project configured, go to the "Run" menu and select "Attach Debugger." Your NetBeans IDE should connect to the application running on
localhost
.
Common Troubleshooting Issues
Despite the correct setup, issues can still arise during debugging. Here are some common problems and their solutions:
1. No Breakpoints Hit
If your breakpoints are not being hit, ensure:
- The debugger is attached before the application code is executed.
- Code changes have been compiled, and the latest version is running.
2. Application Crashes
In case your application crashes, check the following:
- Review the console and stack trace logs for error messages. Understanding exceptions will guide you in fixing issues.
- Ensure that your application dependencies are correctly defined in
pom.xml
.
3. Port Conflicts
When running multiple applications or servers, port conflicts may occur:
- Verify that the debug port (
8787
by default) is not being used by another application. - If conflicts are found, adjust the ports in your
standalone.xml
configuration.
4. Maven Dependency Issues
Sometimes, dependencies can cause classpath issues resulting in ClassNotFoundException
:
- Use
mvn dependency:tree
to visualize your dependency hierarchy. - Ensure that conflicting versions of libraries are resolved.
Leveraging Logging for Debugging
Debugging is not only about breakpoints; logging plays a crucial role. WildFly Swarm allows you to use various logging frameworks like Log4j, SLF4J, etc. Configure logging to track application behavior.
Example Logger Configuration
To use Log4j, add the dependency:
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>latest.version</version>
</dependency>
Create a simple logger in your Java class:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class MyService {
private static final Logger logger = LogManager.getLogger(MyService.class);
public void performAction() {
logger.info("Action is being performed");
// Your business logic here
}
}
Using logging not only helps you track application flow but also aids in troubleshooting. Make sure to adjust the logging level according to your needs.
Final Thoughts
Debugging WildFly Swarm applications in NetBeans can present challenges, but with the right setup, common troubleshooting techniques, and effective logging, it can be a straightforward process. Remember to keep your environment updated and refer to the official WildFly Swarm documentation for the latest updates and best practices.
Whether you are a seasoned developer or just starting with microservices, mastering the debugging process will significantly enhance your productivity and the quality of your applications. Embrace the challenge, and happy coding!