Overcoming Challenges in Custom Validation with Jersey 2
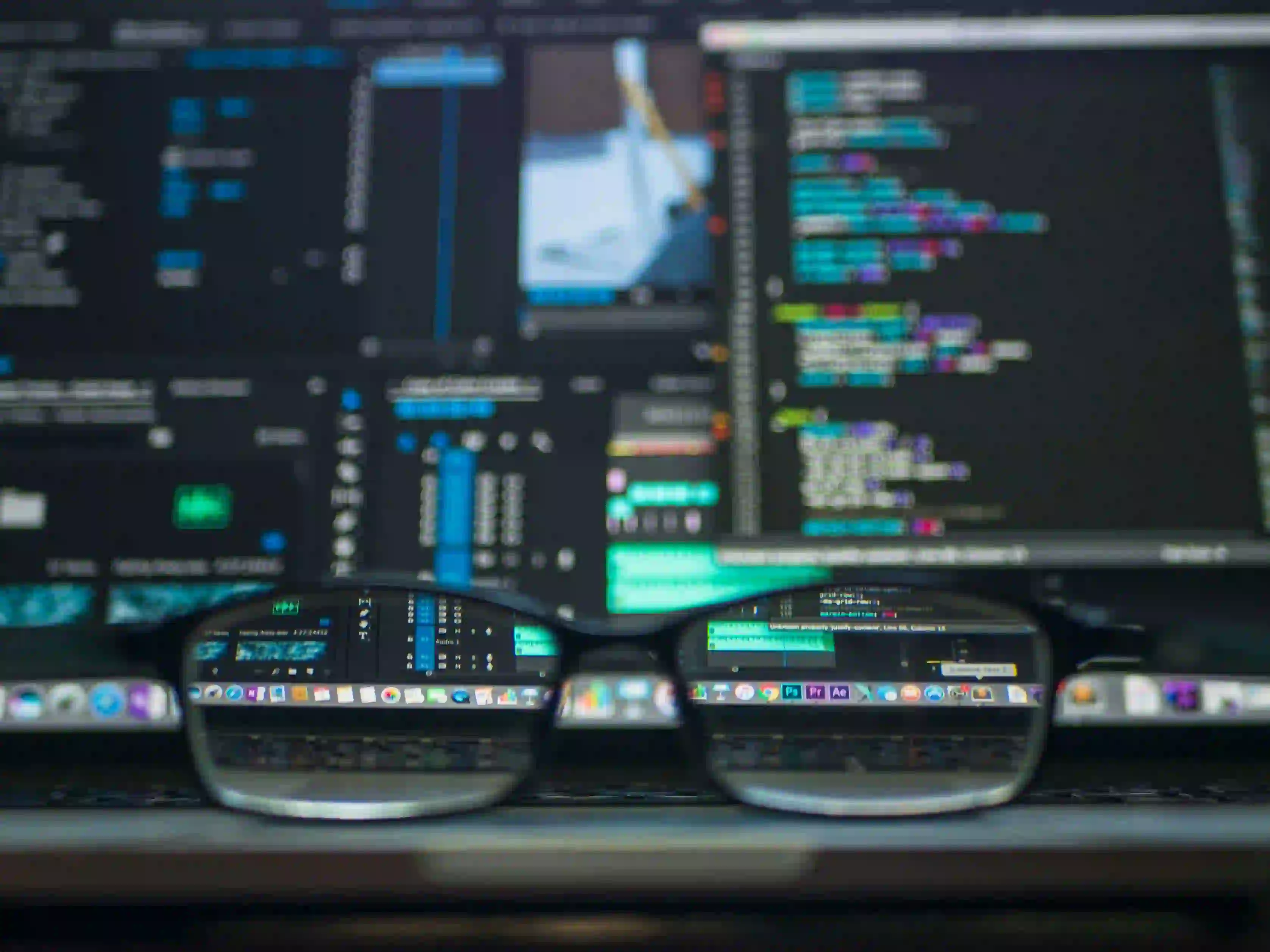
Overcoming Challenges in Custom Validation with Jersey 2
When developing RESTful web services in Java, Jersey 2 is a popular framework that provides flexibility, performance, and powerful features. One of the crucial aspects of building robust applications is ensuring that the data received from clients is valid. Custom validation is a common requirement that can help enforce business rules and improve data integrity. In this post, we'll explore the challenges associated with custom validation in Jersey 2 and provide solutions to effectively overcome them.
Understanding Jersey 2 and Validation
Jersey is an open-source framework that simplifies the creation of RESTful web services. It uses annotations to define the structure of the web service and supports various features such as context injection, content negotiation, and error handling.
Why Custom Validation Matters
Validating incoming data ensures that the application behaves correctly and efficiently. Employing custom validation means you can enforce specific rules beyond what's provided by default, such as checking the uniqueness of a field or validating complex data structures.
Challenges with Custom Validation
1. Limited Built-in Validation Capabilities
While Jersey provides basic validation annotations (like @NotNull, @Size, and @Pattern), these are often not sufficient for complex business rules. Developers frequently need to create custom validation annotations, which can introduce complexity.
2. Integration with JSR 380 (Bean Validation 2.0)
Jersey integrates with JSR 380, but setting this up can be tricky, particularly when you want to create your own validation logic. Many developers struggle with getting their custom annotations recognized and executed during the validation lifecycle.
3. Error Handling
Providing meaningful error responses is critical for a good API design. However, managing different types of error messages can be cumbersome when dealing with custom validation logic.
Implementing Custom Validation in Jersey 2
Let’s walk through the steps of creating a custom validation in Jersey 2, addressing these common challenges.
Step 1: Create a Custom Annotation
First, we will create a custom annotation that we can apply to a field in our data model. For this example, we will create a @ValidAge
annotation to validate that the age is within a specific range.
import javax.validation.Constraint;
import javax.validation.Payload;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Constraint(validatedBy = AgeValidator.class)
@Target({ ElementType.METHOD, ElementType.FIELD, ElementType.ANNOTATION_TYPE, ElementType.PARAMETER })
@Retention(RetentionPolicy.RUNTIME)
public @interface ValidAge {
String message() default "Invalid age.";
Class<?>[] groups() default {};
Class<? extends Payload>[] payload() default {};
}
Why This Annotation?
- We set the
@Constraint
annotation to link our custom validator,AgeValidator
. - Retention policy is set to
RUNTIME
so that the annotation is available during the running of the application. - This design allows you to easily extend the validation rules if required.
Step 2: Create the Validator
Next, implement the logic for your custom validation. Here's a simple validator that checks if the age is between 0 and 120.
import javax.validation.ConstraintValidator;
import javax.validation.ConstraintValidatorContext;
public class AgeValidator implements ConstraintValidator<ValidAge, Integer> {
@Override
public void initialize(ValidAge constraintAnnotation) {
// Initialization code if needed
}
@Override
public boolean isValid(Integer age, ConstraintValidatorContext context) {
return age != null && age >= 0 && age <= 120;
}
}
Why This Validator?
- It implements
ConstraintValidator
, which is the interface required for custom validation logic. - The
isValid
method uses straightforward logic, enhancing readability and maintainability.
Step 3: Applying the Custom Annotation
Now that we have our custom validation set up, let's apply it to a model class:
import javax.validation.constraints.NotNull;
public class User {
@NotNull(message = "Name is required.")
private String name;
@ValidAge
private Integer age;
// Getters and Setters
}
Step 4: Configuring Jersey to Use Bean Validation
To ensure that our custom validations are recognized, we must configure Jersey correctly. This can often be a stumbling block.
Add this to your Jersey configuration class:
import org.glassfish.jersey.server.ResourceConfig;
public class MyApplication extends ResourceConfig {
public MyApplication() {
packages("com.example"); // Adjust this package name as necessary
register(org.glassfish.jersey.server.validation.ValidationFeature.class);
}
}
Why Configure Jersey This Way?
- The
ResourceConfig
class is how we instruct Jersey on which packages to scan for resources and providers, ensuring that it hooks into the Bean Validation feature.
Step 5: Handling Validation Errors
When validation fails, you should provide meaningful feedback to the client. You can achieve this by creating an ExceptionMapper
.
import javax.validation.ConstraintViolationException;
import javax.ws.rs.core.Response;
import javax.ws.rs.ext.ExceptionMapper;
import javax.ws.rs.ext.Provider;
@Provider
public class ConstraintViolationExceptionMapper implements ExceptionMapper<ConstraintViolationException> {
@Override
public Response toResponse(ConstraintViolationException exception) {
// Build a response with specific error messages
return Response.status(Response.Status.BAD_REQUEST)
.entity(exception.getConstraintViolations())
.build();
}
}
Why This ExceptionMapper?
- It creates a structured response for validation errors, making it easier for clients to identify issues in their requests.
Putting It All Together
Now that we have custom validation implemented, let's see how all this fits into a complete RESTful endpoint:
import javax.ws.rs.*;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
@Path("/users")
public class UserResource {
@POST
@Consumes(MediaType.APPLICATION_JSON)
@Produces(MediaType.APPLICATION_JSON)
public Response createUser(User user) {
// Process user creation logic
// Return successful response
return Response.status(Response.Status.CREATED).entity(user).build();
}
}
In Conclusion, Here is What Matters
By following the steps outlined, you can effectively implement custom validation in Jersey 2. By creating your own annotations, implementing validation logic, and configuring Jersey appropriately, you can tackle common challenges such as limited built-in capabilities and error handling.
Creating a smooth validation experience ultimately leads to more robust applications. Whether it's validation for user data or any other input, it’s key to building RESTful web services that are both reliable and secure.
For further reading on Java validation, consider checking out the Java Bean Validation official documentation. If you're interested in exploring more about Jersey 2, check the Jersey documentation.
Additional Resources
- Understanding Bean Validation
- Creating REST APIs with Jersey
Leave a comment below if you have any questions or encounters any challenges while working with custom validations in Jersey 2!