Common Git Mistakes: Avoid These Version Control Pitfalls
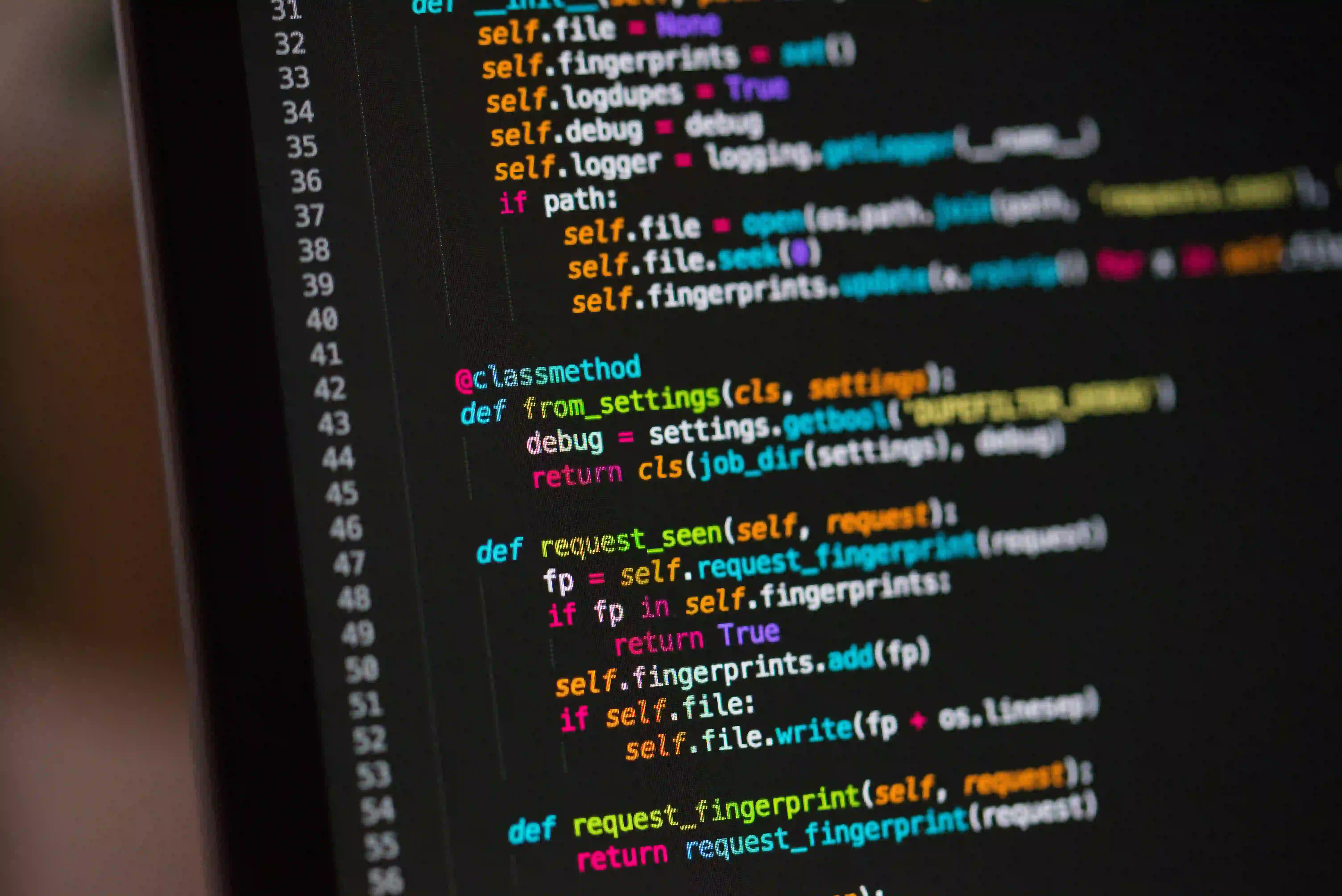
Common Git Mistakes: Avoid These Version Control Pitfalls
Version control is an essential part of modern software development, and Git is by far the most popular system in use today. While Git provides powerful tools to manage code, it can be easy to make common mistakes that derail your workflow. In this blog post, we’ll explore frequent pitfalls developers encounter while using Git and provide practical solutions to avoid them.
1. Committing Unfinished Work
One of the most common mistakes is committing code that isn't finished or adequately tested. This practice can clutter your commit history and introduce bugs into the main codebase.
Why is This a Problem?
Committing unfinished work can lead to confusion among team members. If a teammate pulls in your code, they might face compilation issues or unexpected behavior in the application. Over time, a history cluttered with incomplete commits can complicate the project’s evolution.
Solution
Use Git's staging area wisely. Stage only the files you’re ready to commit using:
git add <filename>
If you find that you've staged files that are not ready to go, you can easily reset them:
git reset <filename>
This keeps your commit history cleaner. For ongoing work, consider creating a draft branch:
git checkout -b feature/draft
Once you’re ready, you can merge it into the main branch with a clean commit.
2. Making Too Many Commits
While it’s essential to make commits, making too many small commits can flood your commit history and make it difficult to track the evolution of the project.
Why is This a Problem?
Excessive commits can obscure the context of changes. When reviewing the commit history, a long list of tiny changes makes it challenging to understand the overall progress of the project.
Solution
Aim for logical, cohesive commits. Commit related changes together. For example, if you’re working on a feature that changes the user interface, make a single commit for all the related modifications.
You can combine previous commits using:
git rebase -i HEAD~<n>
This allows you to squash multiple commits into one, providing a clearer history.
3. Forgetting to Pull Before Pushing
One of the more frustrating mistakes can happen when you forget to pull the latest changes from the remote repository before pushing your own changes. This can lead to merge conflicts or even overwriting your teammate's work.
Why is This a Problem?
Not pulling can cause conflicts that require conflict resolution, making your workflow inefficient. Being unaware of upstream changes can result in losing critical code updates introduced by others.
Solution
Always pull the latest changes before pushing:
git pull origin <branch-name>
This action ensures your local branch has the most recent updates from the remote repository. If you encounter a conflict during a pull, Git will guide you through resolving it, which you can do with:
git mergetool
4. Not Using .gitignore Properly
Many developers overlook the importance of the .gitignore
file, which prevents certain files from being tracked by Git. This is vital for avoiding unnecessary clutter in your repository.
Why is This a Problem?
Ignoring files that should not be part of version control—like IDE configuration files, build outputs, or sensitive information—can lead to a messy repository and potential security issues.
Solution
Always utilize a .gitignore
file. You can create one in your project root:
touch .gitignore
Then, list files or directories that must be ignored:
# IDE files
*.iml
*.idea/
# Dependency directories
/node_modules
/build/
You can check GitHub's gitignore templates for common setups.
5. Force Pushing Changes
The git push --force
command can be handy but dangerous. It overwrites remote changes without any checks.
Why is This a Problem?
Force pushing can erase your team's work or lead to loss of important commit history. This might create friction and confusion in collaborative environments.
Solution
Instead of force pushing, consider using:
git push --force-with-lease
This command ensures you're only force pushing if you’re up-to-date with your remote. It provides a safeguard against unintentionally overwriting someone else’s work.
6. Ignoring Branching Best Practices
Using branches improperly or not utilizing them at all can lead to a chaotic development process. Many developers work directly on the main branch, which is fraught with risk.
Why is This a Problem?
Direct code changes on the main branch can introduce instability. Without branches, code reviews become challenging, and it’s hard to isolate features during development.
Solution
Always create a new branch for each feature or bug fix. Use:
git checkout -b feature/new-feature
This keeps your main branch stable. Once your feature is complete and tested, you can safely merge back into the main branch:
git checkout main
git merge feature/new-feature
7. Not Documenting Commits
Failing to write meaningful commit messages can be a hidden pitfall. Many developers will commit with generic messages like “fix stuff” or “update.”
Why is This a Problem?
Uninformative commit messages make it difficult to understand the project’s history. When trying to track down bugs or understand decision-making, the lack of context can slow you down.
Solution
Adopt a clear and structured format for commit messages. Here’s a template:
[type]: [subject]
[additional paragraphs explaining the why and how]
For example:
fix: resolve null pointer exception in UserService
This change addresses a bug that occurs when the user is not found.
Added a null check to prevent application crash and added test cases.
Lessons Learned
Mastering Git requires practice, but avoiding these common pitfalls can streamline your development process. Commit wisely, use branches effectively, document your work, and remember to pull before pushing.
By understanding these common mistakes and their solutions, you'll not only improve your own workflows but also foster a more efficient collaborative environment for your team.
By following these best practices, you ensure a smoother workflow in your projects. If you'd like to further enhance your Git skills, consider exploring the official Git documentation or check out resources on GitHub for more specialized guidance. Happy coding!