Common Pitfalls When Integrating MongoDB with Java EE
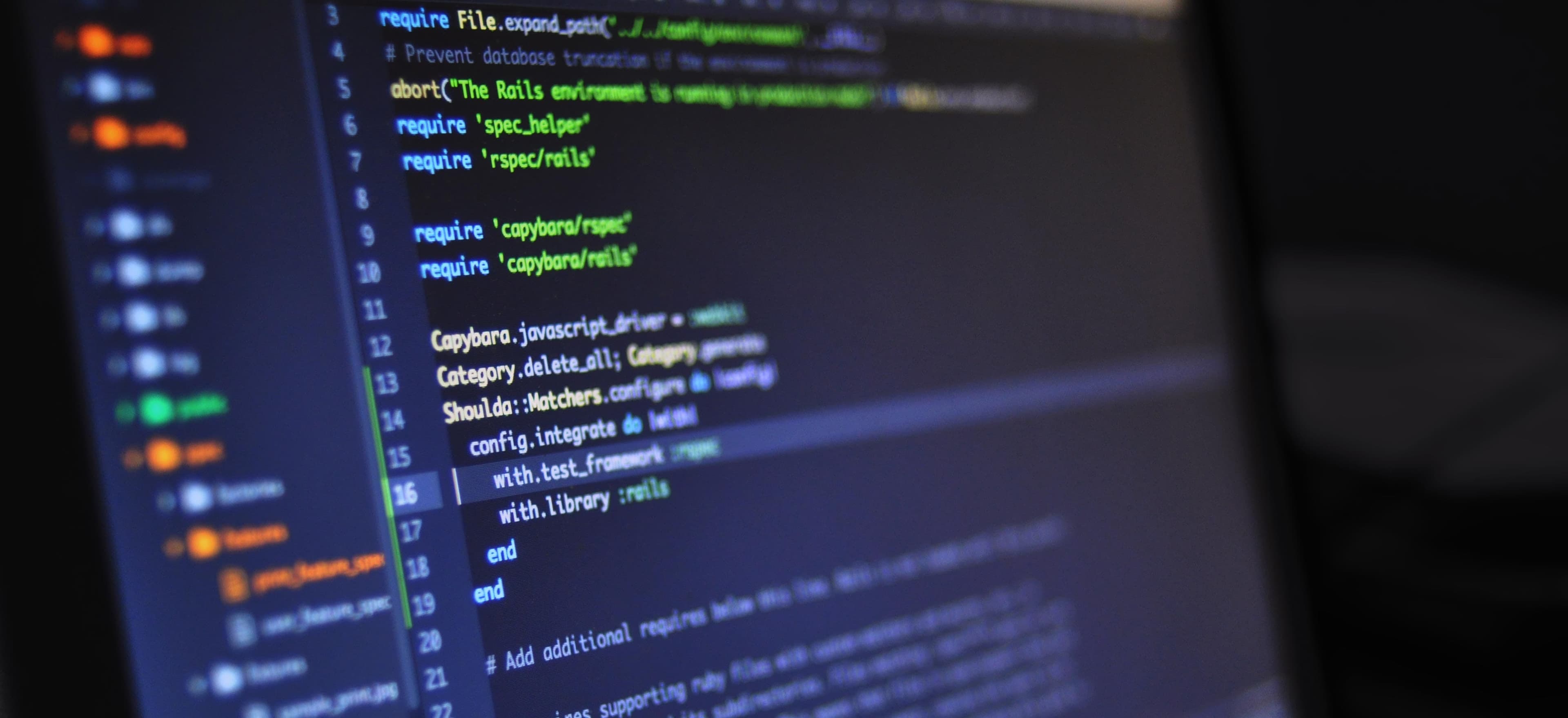
- Published on
Common Pitfalls When Integrating MongoDB with Java EE
Integrating MongoDB with Java EE can be a straightforward process, but it is not without its challenges. In this post, we will explore common pitfalls that developers encounter when working with these technologies. Our aim is to offer insights and best practices to ensure a smooth integration experience.
Understanding MongoDB and Java EE
MongoDB is a NoSQL database that uses a flexible schema to store data in a document-oriented format. On the other hand, Java EE (Enterprise Edition) is a robust platform for developing large-scale, distributed applications. The combination of these two technologies can be powerful, but it requires careful attention to detail.
Why Choose MongoDB for Java EE Applications?
- Scalability: MongoDB allows for horizontal scaling, making it suitable for applications with large amounts of data.
- Flexibility: Its schema-less nature enables rapid development, allowing developers to model data without rigid structures.
- High Performance: MongoDB excels in scenarios requiring high-speed data access, making it ideal for real-time applications.
Common Pitfalls When Integrating MongoDB with Java EE
1. Lack of Proper Connection Management
One of the first challenges developers face is managing connections effectively. MongoDB provides a client driver that can be reused across your application to avoid performance hits caused by frequent connections.
Here's a typical connection setup:
import com.mongodb.MongoClient;
import com.mongodb.MongoClientURI;
public class MongoDBConnection {
private static final String URI = "mongodb://localhost:27017";
private static MongoClient mongoClient;
public static void connect() {
if (mongoClient == null) {
MongoClientURI clientURI = new MongoClientURI(URI);
mongoClient = new MongoClient(clientURI);
}
}
public static MongoClient getClient() {
return mongoClient;
}
}
Why This Matters: By implementing a single instance of MongoClient
, you minimize the overhead of establishing multiple connections, making your application more scalable.
2. Ignoring the Benefits of Document Structure
Many developers transitioning from relational databases fall into the trap of expecting data to be organized in tables. MongoDB allows you to store related data within a single document.
Poor practice example:
// Storing user information in separate collections
userCollection.insert(user);
addressCollection.insert(address);
Preferred practice example:
import org.bson.Document;
Document userDocument = new Document("name", "John Doe")
.append("email", "john.doe@example.com")
.append("address", new Document("city", "New York")
.append("zipcode", "10001"));
userCollection.insertOne(userDocument);
Why This Matters: By embedding related data in a single document, you reduce the number of queries, optimize read performance, and make it easier to retrieve related information.
3. Not Using Java EE Contextual Resources
Java EE provides Context and Dependency Injection (CDI) to manage database connections. Relying on traditional JNDI (Java Naming and Directory Interface) can lead to issues with resource pooling and management.
import javax.annotation.Resource;
import javax.enterprise.context.RequestScoped;
import com.mongodb.MongoClient;
@RequestScoped
public class UserService {
@Resource(lookup = "java:global/mongoClient")
private MongoClient mongoClient;
public void performDatabaseOperations() {
// Use mongoClient here
}
}
Why This Matters: Leveraging CDI simplifies resource management, enhances the maintainability of your application, and aligns with Java EE's standards.
4. Misunderstanding Transaction Support
MongoDB has its own way of handling transactions, especially with its support for multi-document ACID transactions starting from version 4.0. Failing to understand how this works can lead to data inconsistencies.
An example of a multi-document transaction:
import com.mongodb.client.ClientSession;
import com.mongodb.client.MongoDatabase;
try (ClientSession session = mongoClient.startSession()) {
session.startTransaction();
try {
// Perform multiple operations
userCollection.withSession(session).insertOne(newUser);
orderCollection.withSession(session).insertOne(newOrder);
session.commitTransaction();
} catch (Exception e) {
session.abortTransaction();
// Handle error
}
}
Why This Matters: Utilizing transactions properly ensures data integrity, especially in scenarios that involve multiple operations across different collections.
5. Forgetting to Handle Indexes
MongoDB is a powerful database, but if you do not leverage indexes effectively, you will encounter slow query performance. Not defining indexes can lead to full collection scans, resulting in unacceptable response times.
Create an index:
userCollection.createIndex(Indexes.ascending("email"));
Why This Matters: Indexes help optimize queries, speed up search operations, and reduce resource consumption during data retrieval.
6. Not Validating User Input
Security is paramount, especially when dealing with web applications. Neglecting to validate user input can expose your application to NoSQL injection attacks. Always sanitize and validate data before writing it to the database.
Input validation example:
public void insertUser(String userInputEmail) {
if(isValidEmail(userInputEmail)) {
Document userDocument = new Document("email", userInputEmail);
userCollection.insertOne(userDocument);
} else {
throw new IllegalArgumentException("Invalid Email Address");
}
}
private boolean isValidEmail(String email) {
// Regex for validating email
return email.matches("^[\\w-\\.]+@([\\w-]+\\.)+[\\w-]{2,4}$");
}
Why This Matters: Validating input helps prevent critical security vulnerabilities, protecting both your application and users' data.
Additional Considerations
While we've covered some of the common pitfalls, there are additional factors to consider:
- Choosing the Right Driver: Ensure you are using the appropriate MongoDB Java driver based on your version of Java EE.
- Error Handling: Implement robust error handling for database operations to ensure your application can recover gracefully from unexpected issues.
To Wrap Things Up
Integrating MongoDB with Java EE can yield substantial advantages when done correctly. By avoiding common pitfalls such as improper connection management, ignoring document structures, leveraging Java EE resources, and ensuring proper security practices, developers can significantly enhance application performance and maintainability.
If you're looking to dive deeper into MongoDB and its integration with Java EE, consider checking out the official MongoDB Java Driver Documentation and Java EE Specifications.
By understanding these common challenges and addressing them proactively, you can elevate your application development experience and harness the full potential of both MongoDB and Java EE. Happy coding!