Handling Timeout Issues with DeferredResult in Spring MVC
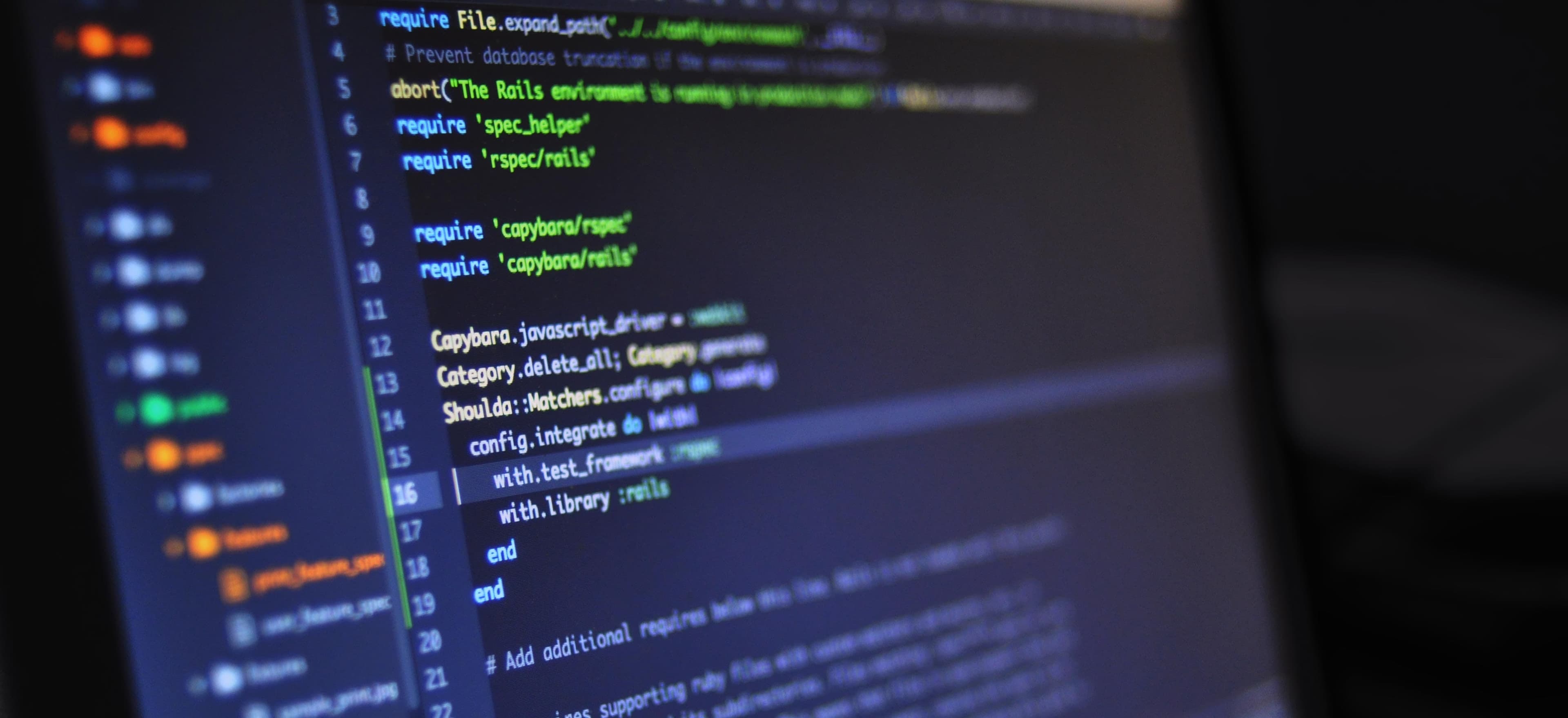
- Published on
Handling Timeout Issues with DeferredResult in Spring MVC
In today's web development landscape, creating responsive and efficient applications is paramount. Asynchronous processing is a powerful approach to achieving responsiveness, especially in applications that require long-running operations. In Spring MVC, DeferredResult
is an exceptional tool that allows developers to handle long-running tasks without blocking the request thread. However, managing timeout issues effectively is a crucial aspect many developers may overlook.
This blog post aims to provide a thorough understanding of DeferredResult
, its usage, and how to effectively handle timeout issues in a Spring MVC application.
What is DeferredResult?
DeferredResult
is a Spring MVC class that provides an asynchronous mechanism for handling requests. Using DeferredResult
, you can return a result from a background thread while keeping the requesting thread free and ready to handle another incoming request.
Here’s a simple overview of how it works:
- The controller method returns a
DeferredResult
. - The actual processing happens in a background thread, allowing the request thread to return immediately.
- Once the processing is complete, the result can be set, which will trigger the response to the client.
Example of DeferredResult
Here is a simple example of how you can use DeferredResult
in a REST controller:
import org.springframework.web.bind.annotation.*;
import org.springframework.web.context.request.async.DeferredResult;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
@RestController
@RequestMapping("/api")
public class AsyncController {
private final ScheduledExecutorService executorService = Executors.newScheduledThreadPool(5);
@GetMapping("/process")
public DeferredResult<String> processRequest() {
DeferredResult<String> deferredResult = new DeferredResult<>(5000L); // 5000 ms timeout
deferredResult.onTimeout(() -> {
System.out.println("Request timed out");
deferredResult.setErrorResult("Request timed out - please try again later");
});
executorService.submit(() -> {
try {
// Simulating a long-running task
TimeUnit.SECONDS.sleep(3);
deferredResult.setResult("Processing complete");
} catch (InterruptedException e) {
deferredResult.setErrorResult("Processing interrupted");
}
});
return deferredResult;
}
}
Explanation of the Code
- Timeout Handling: In this code, a
DeferredResult
is instantiated with a timeout of 5000 milliseconds. TheonTimeout
callback is registered to handle the scenario where the processing takes longer than anticipated. - Background Processing: The task is executed in a separate thread using the
ExecutorService
. Here, we simulate a long-running process withTimeUnit.SECONDS.sleep(3);
.
Handling Timeout Issues
Why Handle Timeouts?
Timeout issues can directly affect user experience. When a user initiates a request, waiting indefinitely can lead to frustration, ultimately resulting in a lack of engagement with your application. Implementing effective timeout handling ensures that users receive timely feedback and can take further action.
Setting a Timeout Value
Choosing the right timeout value is essential. Setting it too low may result in premature timeouts, while too high can lead to long waits for users. Consider real-world scenarios in your application to establish a reasonable timeout.
Customizing the Timeout Response
The DeferredResult
allows you to customize the response in case of a timeout. You can set an error message, redirect the user, or log the incident for debugging purposes.
deferredResult.onTimeout(() -> {
System.out.println("Request timed out");
deferredResult.setErrorResult("Request timed out - please try again later");
});
In the above snippet, we log the timeout and provide feedback to the user.
Monitoring and Debugging
Implementing proper logging mechanisms can help in monitoring timeout occurrences and debugging any related issues. Utilize tools like Spring’s built-in logging or AOP (Aspect-Oriented Programming) to keep track of request processing times.
Using Spring Boot’s @Async annotation
In conjunction with DeferredResult
, Spring Boot offers the @Async
annotation, which allows methods to be executed asynchronously. Here is how you can effectively incorporate this in your application:
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
@Service
public class AsyncService {
@Async
public String longRunningTask() {
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
return "Processing interrupted";
}
return "Task complete";
}
}
The @Async
annotation creates a proxy that runs the method in a separate thread. Combine this service with our DeferredResult
example for enhanced processing.
Error Handling with DeferredResult
Handling errors gracefully is another critical aspect of using DeferredResult
. You can utilize the onError
callback to manage unexpected exceptions:
deferredResult.onError(throwable -> {
System.err.println("Error occurred: " + throwable.getMessage());
deferredResult.setErrorResult("An error occurred while processing your request.");
});
Final Thoughts
DeferredResult
in Spring MVC allows for efficient asynchronous processing, but it comes with its challenges, especially regarding timeouts and error handling. Adopting best practices for these aspects can significantly enhance the responsiveness and user experience of your application.
Whether you implement simple timeout handling or delve deeper into asynchronous service integration, understanding DeferredResult
gives you a profound advantage in web application development.
For more information on using @Async
in Spring, check out the Spring Documentation.
If you're interested in learning more about asynchronous processing with Spring, consider exploring Spring WebFlux, which offers a non-blocking reactive programming model.
By following the guidelines and examples provided in this post, you should now be well-equipped to handle timeout issues effectively within your Spring MVC applications. Happy coding!