Common Pitfalls in Implementing RESTful Web Services with Jersey
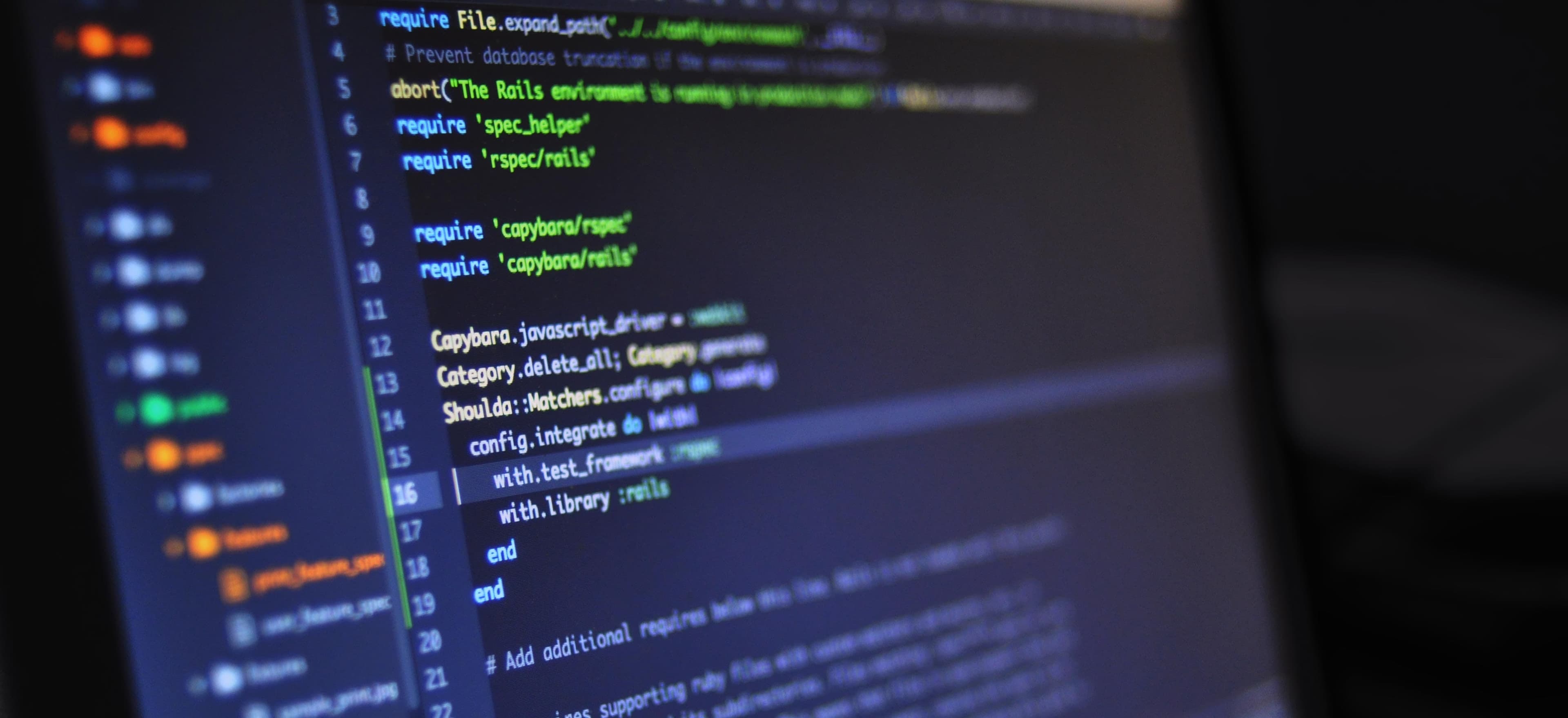
- Published on
Common Pitfalls in Implementing RESTful Web Services with Jersey
Creating RESTful web services with Jersey, a popular framework for developing REST APIs in Java, can be a rewarding experience. However, developers often encounter common pitfalls that can lead to issues such as performance degradation, poor maintainability, or security vulnerabilities. In this blog post, we'll delve deep into these challenges, share best practices to avoid them, and give you a pathway to building high-quality REST services.
What is Jersey?
Jersey is an open-source framework that simplifies the development of RESTful web services in Java. It provides a set of APIs and an implementation that enables developers to create services that conform to the REST architectural style easily. You can learn more about Jersey in the official documentation.
Common Pitfalls in Jersey
1. Not Following the REST Principles
The Issue: One of the most significant pitfalls is failing to adhere to REST principles. REST revolves around resources, and each resource should be accessible through a unique URI.
Solution: Ensure that your service is designed around resources. For example, consider a User
resource.
@Path("/users")
public class UserService {
@GET
@Produces(MediaType.APPLICATION_JSON)
public List<User> getAllUsers() {
return userService.findAll();
}
@GET
@Path("{id}")
@Produces(MediaType.APPLICATION_JSON)
public User getUserById(@PathParam("id") Long id) {
return userService.findById(id);
}
}
Why?: This clarity in your API design promotes better usability and enhances the chances of successful integration with client applications. Each URI corresponds to a specific resource, making it easier for consumers to navigate your API.
2. Ignoring Proper HTTP Method Usage
The Issue: Confusing HTTP methods is a common pitfall. Each method (GET, POST, PUT, DELETE) is intended for specific operations.
Solution: Always map HTTP methods to their appropriate actions. For instance, use POST for creating resources and DELETE for removing them.
@POST
@Consumes(MediaType.APPLICATION_JSON)
public Response createUser(User user) {
userService.save(user);
return Response.status(Response.Status.CREATED).entity(user).build();
}
@DELETE
@Path("{id}")
public Response deleteUser(@PathParam("id") Long id) {
userService.delete(id);
return Response.noContent().build();
}
Why?: Using the correct HTTP method enhances the predictability of your API. Clients can reliably understand the operations they can perform based on the given HTTP methods.
3. Skipping Error Handling
The Issue: Omitting error handling can lead to ungraceful API failures, leaving clients in the dark about what went wrong.
Solution: Implement exception handling to return meaningful HTTP responses.
@Provider
public class MyExceptionMapper implements ExceptionMapper<MyCustomException> {
@Override
public Response toResponse(MyCustomException exception) {
return Response.status(Response.Status.INTERNAL_SERVER_ERROR)
.entity("Error: " + exception.getMessage())
.build();
}
}
Why?: Proper error handling aids clients in troubleshooting issues, enhancing their experience with your API. Clear messages improve the debuggability of client applications.
4. Overexposing Information
The Issue: Exposing too much information in your API responses (like internal application structure details) can be a security risk.
Solution: Limit the information shared in error messages, and consider employing a logging framework to log detailed errors internally.
@GET
@Path("{id}")
@Produces(MediaType.APPLICATION_JSON)
public User getUserById(@PathParam("id") Long id) {
try {
return userService.findById(id);
} catch (Exception e) {
// Log the exception for analysis
logger.error("Error fetching user with ID: " + id, e);
throw new MyCustomException("User not found.");
}
}
Why?: Keeping internal workings private minimizes security vulnerabilities and prevents potential attackers from gaining insights into your application.
5. Not Utilizing Content Negotiation
The Issue: Ignoring content negotiation can lead to unexpected behaviors when clients request different content types.
Solution: Implement content negotiation based on the Accept
header to allow clients to decide their preferred media type.
@GET
@Path("{id}")
@Produces({MediaType.APPLICATION_JSON, MediaType.APPLICATION_XML})
public User getUser(@PathParam("id") Long id, @HeaderParam("Accept") String accept) {
return userService.findById(id);
}
Why?: Content negotiation enhances the flexibility of your API, allowing it to serve a wider array of clients with varying preferences.
6. Hardcoding URLs
The Issue: Hardcoding URLs in responses can lead to issues, especially if your application is restructured or deployed in different environments.
Solution: Use URI building strategies to dynamically create links.
@GET
@Path("{id}")
@Produces(MediaType.APPLICATION_JSON)
public Response getUser(@PathParam("id") Long id, @Context UriInfo uriInfo) {
User user = userService.findById(id);
URI userUri = uriInfo.getAbsolutePathBuilder().build();
return Response.ok(user).location(userUri).build();
}
Why?: Dynamically created URLs help maintain flexibility in different environments and configurations, thus enhancing maintainability.
7. Overly Complicated Endpoints
The Issue: Creating overly complex endpoints with many query parameters can lead to confusion and potential misuse.
Solution: Simplify your API by minimizing the number of parameters and using clear naming conventions.
@GET
@Path("/search")
public List<User> searchUsers(@QueryParam("name") String name) {
return userService.findByName(name);
}
Why?: Simplicity in API design fosters better usability, encouraging developers to adopt your API without being overwhelmed by complexity.
To Wrap Things Up
Designing RESTful services with Jersey is not without challenges. Avoiding these common pitfalls will pave the way for more robust, secure, and user-friendly APIs. Remember to adhere to REST principles, utilize correct HTTP methods, implement error handling, limit exposed information, and maintain simplicity.
By adhering to the best practices outlined in this post, you'll not only enhance the quality of your services but also make life easier for the developers who will interact with them. For a deeper dive into Jersey and REST principles, consider exploring resources like RESTful Web Services by Leonard Richardson and Sam Ruby.
Take your REST API development to the next level by being mindful of these common pitfalls and solutions. Happy coding!
Checkout our other articles