Overcoming Challenges in Kafka's Exactly Once Semantics
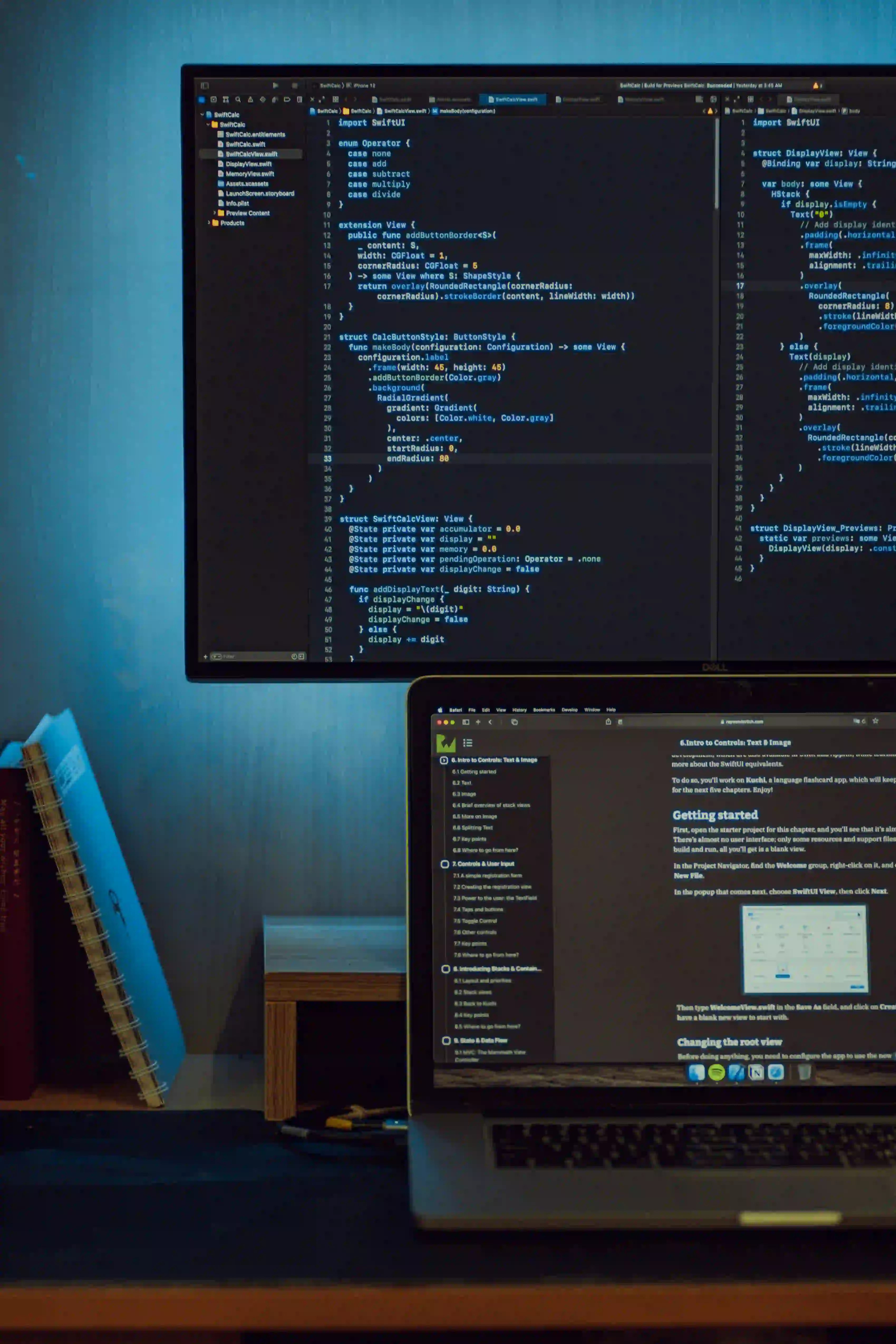
Overcoming Challenges in Kafka's Exactly Once Semantics
Apache Kafka is a powerful distributed streaming platform, and one of its most impactful features is its capability to support "Exactly Once Semantics" (EOS). This feature is crucial for applications where accuracy and consistency of message processing is paramount. While the promise of EOS is enticing, developers often face significant challenges in implementing it correctly. This blog post addresses these challenges and provides guidance on how to effectively leverage Kafka's Exactly Once Semantics, complete with code examples and detailed explanations.
Understanding Exactly Once Semantics in Kafka
What Are Exactly Once Semantics?
In distributed systems, message delivery guarantees can often fall into one of three categories: at-most-once, at-least-once, and exactly-once. The distinction is vital in systems like Kafka, where message processing could lead to duplications or data loss if not handled correctly.
With exactly-once semantics, every message is processed one and only one time, thus ensuring data consistency even in the face of failures. This guarantees that no duplicate messages are seen by downstream consumers, which is crucial for data integrity.
How Does Kafka Achieve Exactly Once Semantics?
Kafka achieves EOS through a combination of idempotent producers, transactions, and consumer offsets management.
-
Idempotent Producers: An idempotent producer ensures that messages with the same key and sequence number are only written once. This eliminates duplicate messages caused by retries.
-
Transactions: Kafka transactions allow you to group multiple produce and consume operations so they either all succeed or all fail—ensuring atomicity across various operations.
-
Consumer Offsets Management: By committing offsets at the end of a transaction, consumers can always read messages in a consistent state.
Despite Kafka’s inherent capabilities, implementing EOS is not without challenges.
Common Challenges in Implementing Exactly Once Semantics
1. Network Partitions
Network partitions can lead to situations where a producer sends a message, but the acknowledgment is not received. In such cases, due to retry mechanisms, duplicate messages can be sent.
Solution:
Enable idempotence on your producer. You can set the producer properties to make the producer idempotent.
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("enable.idempotence", "true");
props.put("acks", "all");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
KafkaProducer<String, String> producer = new KafkaProducer<>(props);
Why Idempotent Producers?
Enabling idempotence ensures that even if a message is sent multiple times due to network failures or retries, it will only be recorded once in the Kafka topic. This is essential for achieving EOS without manual checks for duplicates.
2. Transactional Writing
Using transactions is key for guaranteeing that either all messages are sent or none are. However, managing transactions can introduce complexities, particularly in ensuring that the transaction is opened and committed appropriately.
Solution:
Use Kafka’s Transaction API effectively. Here’s a snippet of how to begin a transaction:
producer.initTransactions();
try {
producer.beginTransaction();
// Produce messages
producer.send(new ProducerRecord<>("topicName", "key", "value"));
producer.commitTransaction();
} catch (ProducerFencedException | OutOfOrderSequenceException | AuthorizationException e) {
producer.abortTransaction();
}
Why Use Transactions?
Transactions enable atomic message processing. If an error occurs mid-process, the transaction can be aborted, preventing partial data from being committed. This is critical for maintaining consistent states in your application.
3. Handling Consumer Offsets
When using transactions, you need to ensure that your consumer offset is committed within the same transaction as the messages. Failure to do this can lead to processing the same message more than once.
Solution:
Use the KafkaTemplate
in Spring Kafka or handle offsets manually. In transactions, you can commit offsets like so:
try {
producer.beginTransaction();
producer.send(new ProducerRecord<>("topicName", "key", "value"));
// Commit the offset only after successfully producing the message
consumer.commitSync();
producer.commitTransaction();
} catch (Exception e) {
producer.abortTransaction();
}
Why Commit Offsets in Transactions?
By committing consumer offsets within the same transaction, you ensure that the consumer does not process messages that might be lost or in an inconsistent state. This is crucial for maintaining the integrity of the application under failure conditions.
Best Practices for Implementing Exactly Once Semantics
-
Always Enable Idempotence: Regardless of whether you’re using transactions or not, enabling idempotence is a safety net against duplicate messages.
-
Understand Your Application’s Needs: Evaluate whether EOS is necessary for your use case. Sometimes, at-least-once semantics may suffice.
-
Implement Robust Error Handling: Ensure your error handling strategies involve graceful retries and fallback mechanisms.
-
Test Extensively: Simulate network failures and transactions to understand how your application behaves under different scenarios.
Wrapping Up
Implementing exactly once semantics in Kafka poses several challenges, mainly around idempotence, transaction management, and consumer offset management. However, with the right strategies and code implementations, these challenges can be overcome to ensure robust and reliable message processing.
For developers venturing into the world of distributed systems, understanding the intricacies of Kafka's exactly once semantics is vital. As applications increasingly rely on data accuracy and integrity, EOS serves as a foundation upon which dependable streaming solutions can be built.
Further Reading and Resources
- Kafka Documentation on Transactions
- Spring Kafka – Transactional Messaging
- Apache Kafka: The Definitive Guide
Stay tuned for more articles diving deep into the world of distributed systems and message streaming! Happy coding!