Overcoming Microservices Complexity in DevOps
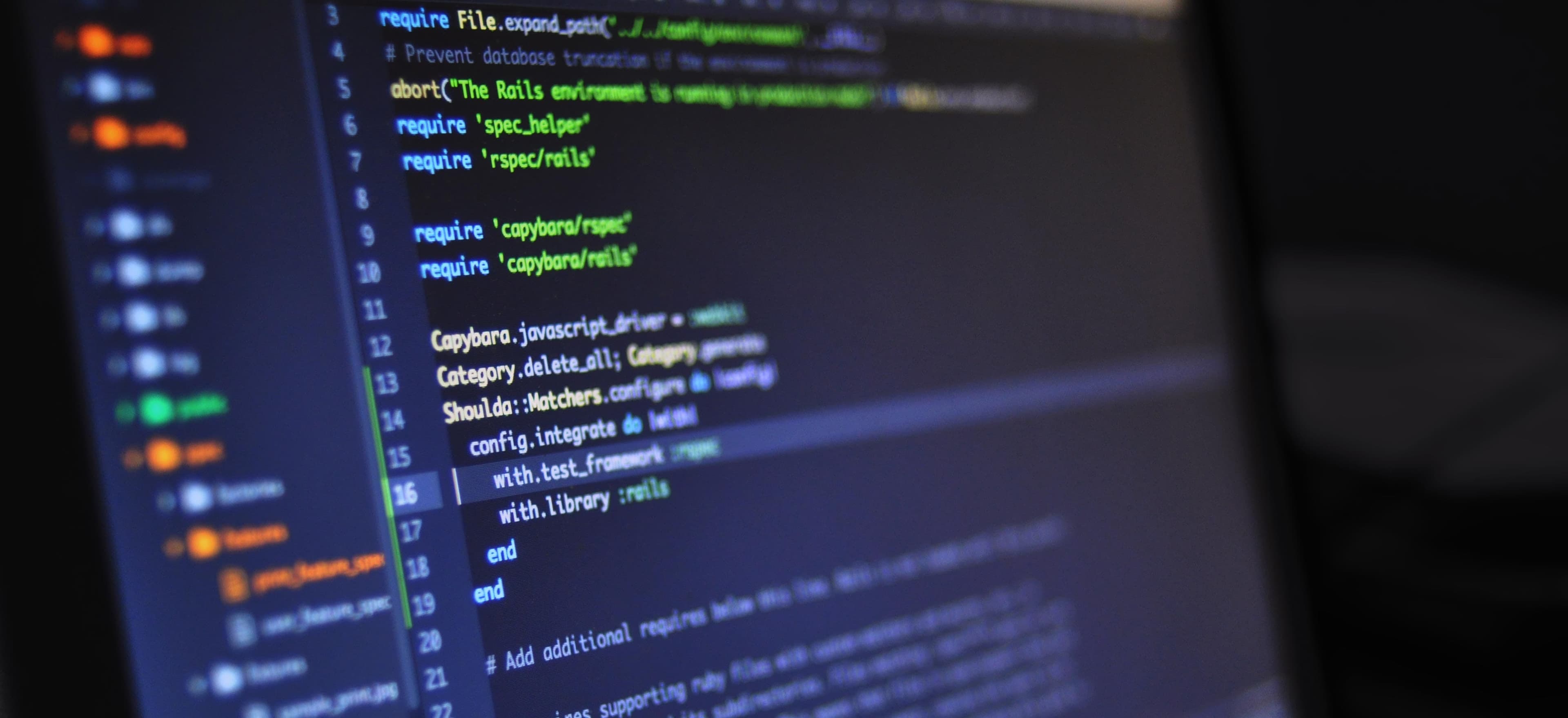
- Published on
Overcoming Microservices Complexity in DevOps
The advent of microservices architecture has fundamentally transformed the landscape of software development and deployment. This paradigm shift, while offering numerous benefits—including scalability, flexibility, and faster time to market—also introduces significant complexity. In this blog post, we will delve into the intricacies of managing microservices within a DevOps framework and discuss strategies to overcome these challenges.
Understanding Microservices
Before we dive into the challenges and solutions, let’s clarify what microservices are. Microservices architecture involves structuring an application as a collection of loosely coupled, independently deployable services. Each service is responsible for a specific business capability and can be developed, deployed, and scaled independently.
This modular approach leads to improved development cycles and allows teams to innovate faster. However, it also adds complexity, especially in a DevOps context.
The Complexity Challenge
Microservices create several challenges that can hinder productivity and efficiency in a DevOps environment:
1. Increased Deployment Overhead
With numerous microservices, deployment can become a logistical nightmare. Each service might have different deployment requirements, environments, and dependencies.
Solution: Implement Continuous Integration/Continuous Deployment (CI/CD) pipelines meticulously tailored for microservices. Tools like Jenkins, GitLab CI, and CircleCI can automate testing and deployment processes.
Here’s an exemplary configuration for a Jenkins pipeline that can streamline CI/CD for microservices:
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
echo 'Building microservice...'
sh './gradlew build' // Building with Gradle
}
}
}
stage('Test') {
steps {
script {
echo 'Running tests...'
sh './gradlew test' // Running unit tests
}
}
}
stage('Deploy') {
steps {
script {
echo 'Deploying microservice...'
sh 'kubectl apply -f k8s/deployment.yaml' // Deploy to Kubernetes
}
}
}
}
}
Why: The pipeline automates the build, test, and deployment process for your microservices. It saves time and reduces human error, enabling teams to deliver updates faster.
2. Service Communication Difficulties
Microservices need to communicate with one another, often leading to increased complexity in how they are integrated. Different services may utilize various protocols and message formats, resulting in potential compatibility issues.
Solution: Adopt service mesh technologies like Istio or Linkerd. These solutions manage service-to-service communication and provide features like traffic management, security, and observability.
Code Example with Service Communication
Consider a microservice architecture where a User Service communicates with an Order Service:
// UserService.java
@RestController
@RequestMapping("/users")
public class UserService {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/{id}/orders")
public ResponseEntity<List<Order>> getUserOrders(@PathVariable String id) {
String orderServiceUrl = "http://order-service/orders/user/" + id;
ResponseEntity<List<Order>> orders = restTemplate.exchange(
orderServiceUrl,
HttpMethod.GET,
null,
new ParameterizedTypeReference<List<Order>>() {}
);
return orders;
}
}
Why: This code snippet illustrates how the User Service retrieves orders from the Order Service. Using RestTemplate
simplifies outbound HTTP requests, providing high-level abstractions for integrating with other microservices.
3. Data Management Challenges
Microservices usually adopt a decentralized data management approach, where each service may maintain its own database. This can lead to data consistency issues and complex transactions.
Solution: Implement event sourcing and CQRS (Command Query Responsibility Segregation) patterns. These strategies allow services to communicate changes through events, increasing decoupling and reducing synchronization overhead.
Code Example: Event Sourcing with Kafka
Let's look at how you can use Kafka for event sourcing:
// ProducerService.java
@Service
public class ProducerService {
@Autowired
private KafkaTemplate<String, Order> kafkaTemplate;
public void sendOrder(Order order) {
kafkaTemplate.send("orders-topic", order);
}
}
// OrderConsumer.java
@Service
public class OrderConsumer {
@KafkaListener(topics = "orders-topic", groupId = "order_group")
public void consume(Order order) {
// Process the order event...
System.out.println("Order received: " + order);
}
}
Why: This example shows how to publish and consume events using Kafka. It enhances decoupling between services and ensures reliability in communication.
Monitoring and Security
4. Observability and Monitoring
Microservices can become a 'black box' if not monitored properly. Invisible errors may arise from inter-service communication, making debugging challenging.
Solution: Utilize centralized logging solutions like ELK Stack (Elasticsearch, Logstash, Kibana) or monitoring services like Prometheus and Grafana. These tools provide insights into microservices' health and performance.
Best Practices:
- Implement appropriate logging levels.
- Ensure logs contain contextual information regarding service interactions.
- Set up alerts for anomalies in service behavior.
5. Security Concerns
Securing microservices is inherently complex due to their distributed nature. Service interactions via APIs can expose vulnerabilities.
Solution: Follow the principle of least privilege and secure the microservices using OAuth and JWT for service authentication and authorization.
Code Example - JWT Authentication:
// JWTProvider.java
@Component
public class JWTProvider {
private String secretKey = "mySecretKey";
public String generateToken(String username) {
return Jwts.builder()
.setSubject(username)
.setExpiration(new Date(System.currentTimeMillis() + 3600000)) // 1 hour expiration
.signWith(SignatureAlgorithm.HS256, secretKey)
.compact();
}
}
Why: This snippet showcases the generation of a JWT token for authentication. Using tokens elevates the security of inter-service communications by verifying the identity of users before granting access.
My Closing Thoughts on the Matter
Navigating the complexities of microservices in a DevOps setting can be daunting. However, employing strategies like CI/CD automation, service meshes, event sourcing, centralized logging, and robust security mechanisms can significantly streamline operations.
As we continue to embrace microservices, it is essential to invest in educating teams on best practices and tools. By doing so, organizations can fully harness the potential of microservices while minimizing the challenges that accompany them.
For more insights into the world of microservices and DevOps, feel free to check out the following resources:
By understanding and addressing these complexities, teams can navigate their microservices journey with much greater confidence and agility. Happy coding!
Checkout our other articles