Troubleshooting Two-Way SSL: Common Client Certificate Issues
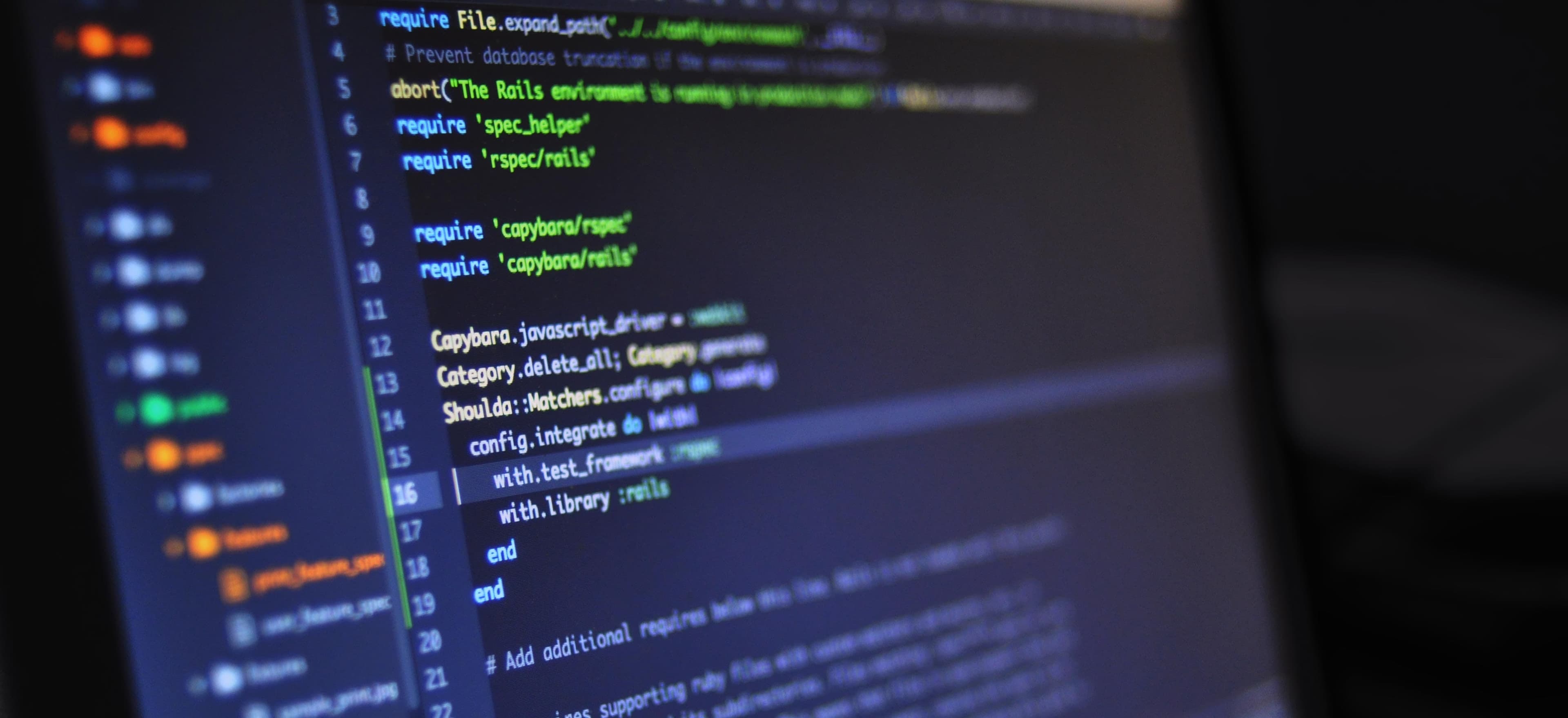
- Published on
Troubleshooting Two-Way SSL: Common Client Certificate Issues
When securing communications over the internet, two-way SSL (Secure Sockets Layer) plays a pivotal role by ensuring that both the client and the server authenticate each other. While this mechanism greatly enhances security, it can also introduce complexities, particularly related to client certificate management. In this blog post, we will explore common issues encountered with two-way SSL client certificates and provide you with troubleshooting strategies to overcome these challenges.
Understanding Two-Way SSL
Two-way SSL, also known as mutual TLS (Transport Layer Security), involves both the server and the client presenting their respective certificates during the SSL handshake. This mutual authentication process can help prevent unauthorized access and ensure that both parties involved are trustworthy.
Key Components of Two-Way SSL
- Server Certificate: Issued to the server by a trusted Certificate Authority (CA).
- Client Certificate: Issued to the client by a trusted CA; required for client authentication.
- Certificate Authority (CA): The entity that issues and manages digital certificates.
- Trust Store: A repository that contains trusted CA certificates.
Basic Workflow
- Handshake Initiation: The client connects to the server, initiating the SSL handshake.
- Server Certificate Validation: The server presents its certificate to the client for authentication.
- Client Authentication: The server requests the client’s certificate to authenticate the client.
- Secure Connection: If both parties successfully validate each other, a secure connection is established.
Common Client Certificate Issues
-
Expired Client Certificates
- Problem: One of the most frequent issues is using an expired client certificate. SSL connections fail when the certificate's validity period has lapsed.
- Solution: Always ensure that client certificates are renewed before expiration. A good practice is to set up reminders based on the certificate expiration date.
// Java code to check if a certificate is expired import java.security.cert.X509Certificate; public boolean isCertificateExpired(X509Certificate cert) { try { cert.checkValidity(); // Throws an exception if expired return false; } catch (Exception e) { return true; // Certificate is expired } }
-
Incorrect Certificate Format
- Problem: Client certificates must be in the correct format, typically PEM or DER. Mismatched formats can lead to failures in SSL negotiation.
- Solution: Ensure you convert the client certificate into the required format before usage. Use libraries or commands like OpenSSL for conversion.
openssl x509 -in client.crt -outform der -out client.der
This command converts a PEM-formatted certificate into DER format if needed.
-
Untrusted Certificate Authority
- Problem: If the CA that issued the client certificate is not present in the server's trust store, the server will not trust the client's certificate.
- Solution: Install the CA's certificate into the server's trust store. Ensure that the server can correctly locate the trust store.
// Example of adding a CA certificate to a Trust Store programmatically import java.security.KeyStore; import java.io.FileInputStream; public void addCACertificate(String keystorePath, String caPath) throws Exception { KeyStore keystore = KeyStore.getInstance("JKS"); keystore.load(new FileInputStream(keystorePath), "password".toCharArray()); try (FileInputStream caInput = new FileInputStream(caPath)) { CertificateFactory cf = CertificateFactory.getInstance("X.509"); Certificate caCertificate = cf.generateCertificate(caInput); keystore.setCertificateEntry("caCertAlias", caCertificate); } try (FileOutputStream fos = new FileOutputStream(keystorePath)) { keystore.store(fos, "newPassword".toCharArray()); } }
-
Client Certificate Not Supplied
- Problem: The client fails to send its certificate during the handshake, often due to misconfiguration in the client application or incorrect SSL settings.
- Solution: Verify SSL configuration settings in the client application. Ensure that the appropriate keystore containing the client certificate is loaded correctly.
// Example of loading SSL context with client certificates import javax.net.ssl.SSLContext; import javax.net.ssl.TrustManagerFactory; import java.security.KeyStore; public SSLContext createSSLContext(String keystorePath, String password) throws Exception { KeyStore clientStore = KeyStore.getInstance("PKCS12"); try (FileInputStream clientCertStream = new FileInputStream(keystorePath)) { clientStore.load(clientCertStream, password.toCharArray()); } TrustManagerFactory tmf = TrustManagerFactory.getInstance(TrustManagerFactory.getDefaultAlgorithm()); tmf.init(clientStore); SSLContext sslContext = SSLContext.getInstance("TLS"); sslContext.init(null, tmf.getTrustManagers(), null); return sslContext; }
-
Hostname Mismatch
- Problem: The client may use a certificate for a hostname that does not match the server’s hostname, resulting in validation failures.
- Solution: Ensure that the Common Name (CN) or Subject Alternative Names (SAN) defined in the client certificate match the server's hostname.
-
Revoked Certificates
- Problem: If a client certificate is revoked by the issuing CA, the server will fail to authenticate the client.
- Solution: Use Certificate Revocation Lists (CRLs) or Online Certificate Status Protocol (OCSP) to check for revoked certificates. Implement these checks in your application logic.
// Pseudocode for checking certificate revocation status public boolean isCertificateRevoked(X509Certificate cert) { // Logic to check the certificate against CRLs or OCSP // Return true if revoked, false otherwise }
Best Practices for Managing Client Certificates
- Automate Certificate Renewal: Utilize tools or scripts to automate the certificate renewal process before expiration.
- Regular Audits: Conduct regular audits of your SSL/TLS configurations, ensuring that both the server and client configurations are up to date.
- Implement Logging and Monitoring: Log SSL/TLS handshake events, errors, and certificate validation results. Monitoring these logs can help identify patterns in certificate-related issues.
- Educate Your Team: Ensure that your development and operations teams are well-versed in SSL/TLS configurations, including two-way SSL.
Lessons Learned
Troubleshooting two-way SSL client certificate issues can be challenging. However, understanding the common pitfalls can streamline the process of diagnosing and fixing these issues. By following the best practices outlined in this blog, you can maintain a secure and efficient communication channel.
For further reading on SSL complexities, refer to SSL/TLS best practices and how certificates work for a deeper understanding.
By implementing the strategies discussed above, you not only enhance the security of your applications but also improve user trust and satisfaction. Stay secure, and happy coding!
Checkout our other articles