Preventing Class Conflicts with Custom Java Class Loaders
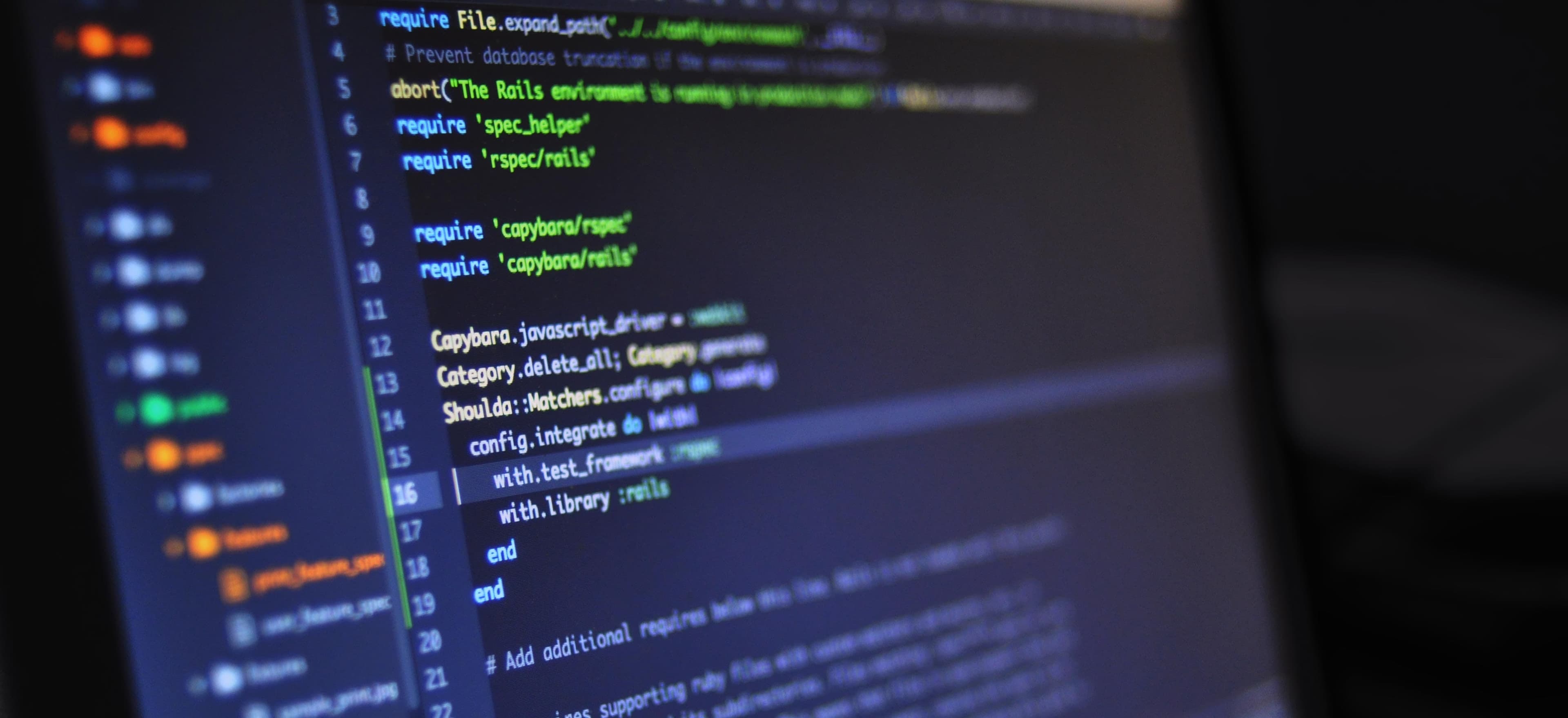
- Published on
Preventing Class Conflicts with Custom Java Class Loaders
Java, as a versatile and robust programming language, allows for powerful mechanisms to manage classes and resources. Among these mechanisms, Java Class Loaders stand out as essential tools for managing how classes are loaded into the Java Virtual Machine (JVM). In this post, we will explore the intricacies of custom Java class loaders and how they can prevent class conflicts, particularly in large applications or environments where multiple libraries can introduce overlapping class definitions.
Understanding Class Loaders
In Java, a class loader is a part of the Java Runtime Environment that dynamically loads Java classes into the JVM. The class loader works by searching for class definitions in a particular order, starting with the bootstrap class loader, followed by the extension class loader, and lastly the application class loader.
The Importance of Class Loaders
Class loaders help isolate classes by loading them into different namespaces. This can prevent conflicts when the same class name exists in different libraries. Here’s a fundamental structure of how class loading works:
- Bootstrap Class Loader: Loads core Java libraries located in
${java.home}/lib
. - Extension Class Loader: Loads classes from the extension directories (e.g.,
${java.home}/lib/ext
). - System/Application Class Loader: Loads classes from the application’s classpath.
This hierarchy is crucial for maintaining order and avoiding conflicts, but issues arise when different libraries use the same class names.
Class Conflicts: The Enemy of Modular Design
Consider a scenario in a large enterprise application where multiple JAR files might have conflicting class names. If two libraries implement a class User
, the JVM might load only one, leading to unexpected behavior or ClassNotFoundException
. To mitigate these issues, developers turn to custom class loaders.
Creating a Custom Class Loader
Custom class loaders extend the built-in ClassLoader
class. This allows developers active control over the class loading process. Below is a simple implementation of a custom class loader:
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.nio.file.Files;
public class CustomClassLoader extends ClassLoader {
private String path;
public CustomClassLoader(String path) {
this.path = path;
}
@Override
protected Class<?> findClass(String name) throws ClassNotFoundException {
byte[] b = loadClassData(name);
if (b == null) {
throw new ClassNotFoundException();
}
return defineClass(name, b, 0, b.length);
}
private byte[] loadClassData(String name) {
try {
String filePath = path + File.separator + name.replace('.', File.separatorChar) + ".class";
return Files.readAllBytes(new File(filePath).toPath());
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
}
Commentary on Code
- Extending ClassLoader: By extending
ClassLoader
, we can create an isolated class loading behavior. - Overriding findClass: This method is essential in defining how our custom class loader will locate and load classes.
- loadClassData: This method handles the raw bytecode loading from the filesystem, allowing for greater flexibility in where classes are stored.
This custom loader loads classes from a specific directory, ensuring there is no overlap with classes loaded by the standard class loader.
Leveraging Custom Class Loaders for Isolation
When employing custom class loaders, it becomes easier to isolate different versions of libraries or classes within the same application. Here’s how it can effectively prevent class conflicts:
Use Case Example: Plugin Architecture
Consider a plugin architecture where independent modules can be added or removed without affecting the core application. Each plugin can use its own custom class loader.
public class PluginManager {
private Map<String, CustomClassLoader> plugins = new HashMap<>();
public void loadPlugin(String pluginName, String path) throws Exception {
CustomClassLoader classLoader = new CustomClassLoader(path);
Class<?> pluginClass = classLoader.loadClass(pluginName);
plugins.put(pluginName, classLoader);
// Instantiate and use the plugin as needed.
}
}
In this example, PluginManager
loads plugins using their own CustomClassLoader
, ensuring that conflicts with core application classes are avoided. Each plugin can load its classes in isolation, which allows for flexibility and modularity.
Best Practices in Using Custom Class Loaders
While custom class loaders are powerful tools for avoiding class conflicts, they also come with their own complexities. Here are some best practices to keep in mind:
- Limit the Scope of Class Loaders: Use custom class loaders for modular components of the application to maintain clarity.
- Clean Up Resources: When done using a custom class loader, explicitly clean up resources and references to avoid memory leaks.
- Monitor and Log: Implement logging in your class loading mechanism to trace issues effectively.
Bringing It All Together
Custom Java class loaders are a valuable mechanism to handle class loading in complex applications. They facilitate modularity and prevent class conflicts, making them an indispensable tool in a developer's arsenal. As you embark on designing your Java applications, consider the architectural implications of class loaders and structure your code to leverage their capabilities effectively.
For further reading and deeper insights on Java class loaders, you can check out:
- Java Class Loading Mechanism - A formal specification in the Java Language.
- Understanding Class Loaders in Java - A comprehensive tutorial for beginners.
By understanding and implementing custom class loaders strategically, you can navigate around class conflicts and build a robust, modular application architecture. Happy coding!
Checkout our other articles