Overcoming Data Consistency Challenges in Serverless Microservices
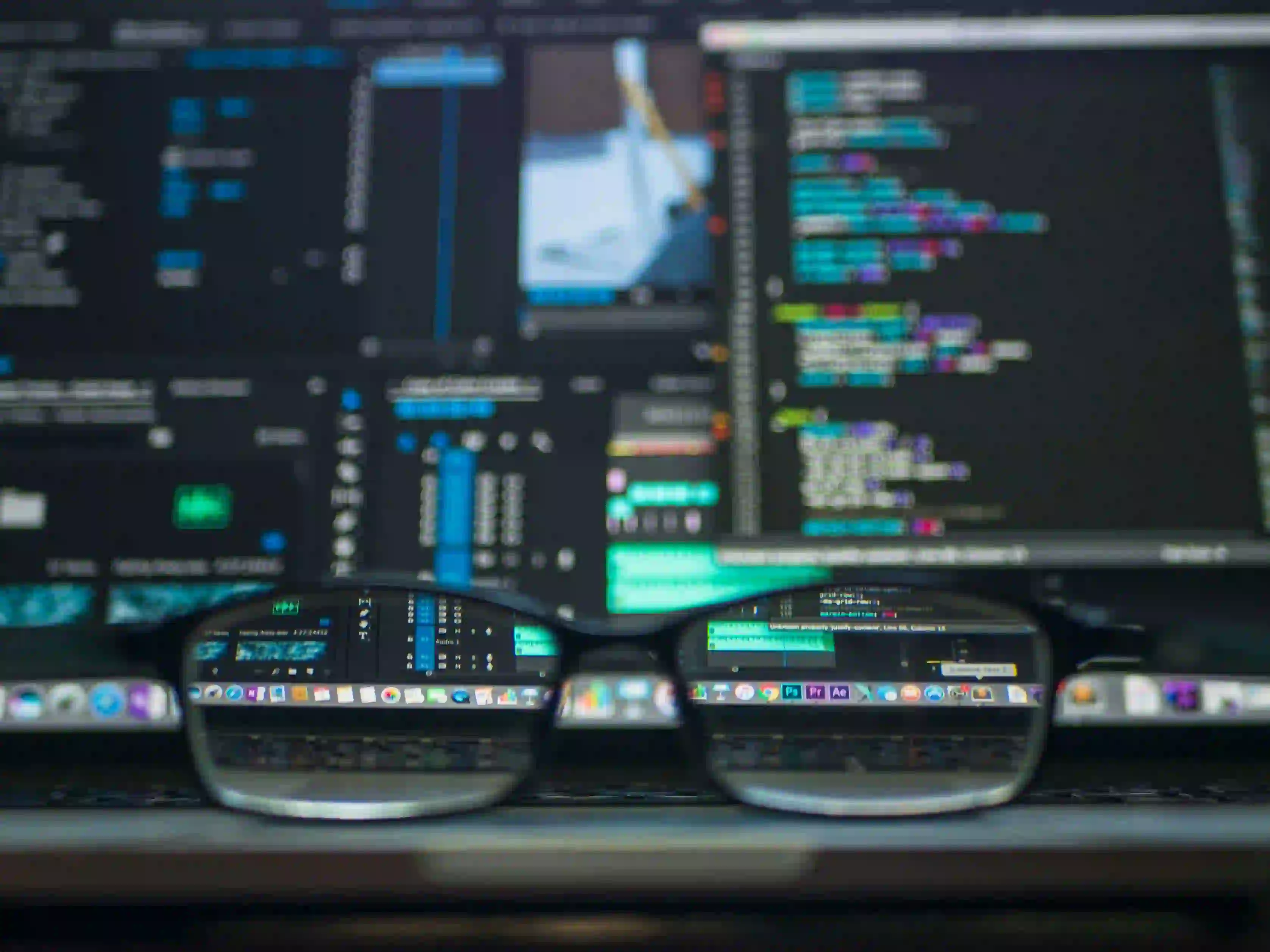
Overcoming Data Consistency Challenges in Serverless Microservices
In the modern software architecture landscape, serverless microservices have emerged as a powerful paradigm. They enable developers to focus on writing code without managing infrastructure, which brings efficiency, scalability, and resilience. However, with this architectural style comes a particular set of challenges—chief among them being data consistency. This post aims to explore the complexities of ensuring data consistency in serverless microservices and present strategies to overcome these challenges.
Understanding Data Consistency in Microservices
What is Data Consistency?
Data consistency refers to the correctness and uniformity of data across different systems and applications. In a microservices architecture, where each service can maintain its own data store, ensuring data is consistent can be quite challenging. This challenge is often amplified in serverless environments where instances of services can spin up and down dynamically, complicating the traditional mechanisms for enforcing consistency.
Types of Data Consistency Models
- Strong Consistency: All clients see the same data at the same time. It's difficult to achieve in distributed systems but beneficial for scenarios needing immediate accuracy.
- Eventual Consistency: The system guarantees that, given enough time without new updates, all replicas will converge to the same state. Considered more practical in microservice environments.
- Weak Consistency: No guarantees are made about when updates will be visible. This can be useful in distributed applications where latency is a primary concern.
For serverless microservices, eventual consistency is often the model of choice due to the ephemeral nature of functions and data storage.
Challenges of Data Consistency in Serverless Architectures
1. Distributed Data Storage
In a serverless architecture, each microservice typically has its own data store. This diversity can lead to a lack of a unified view of the data, complicating updates and introducing the risk of outdated information.
Example: Let's say you have a user service and an order service. Each service manages its own database, which results in multiple data states. If the user updates their profile information, the order service will not immediately reflect this change unless you implement a robust syncing mechanism.
2. Asynchronous Communication
Microservices often communicate asynchronously using event-driven architecture. While this improves performance and decouples services, it can also lead to delays in data visibility, exacerbating consistency issues.
3. Failure and Retries
In serverless environments, functions are stateless and can experience failures and timeouts. Operations may be retried without clear visibility into previous successes or failures, leading to potential data duplication or conflicting updates.
Strategies for Achieving Data Consistency
1. Use of Event Sourcing
Event sourcing is a design pattern that captures all changes to an application's state as a sequence of events. Instead of storing just the current state, every change is logged. This can simplify tracking changes across services, enabling easier reconstruction of the system state.
Code Example: Basic Event Sourcing Pattern
public class UserEvent {
private String userId;
private String eventType;
private LocalDateTime timestamp;
// Constructor, getters and setters
}
public class UserService {
private final List<UserEvent> eventStore = new ArrayList<>();
public void updateUser(User user) {
// Update user logic
eventStore.add(new UserEvent(user.getId(), "USER_UPDATED", LocalDateTime.now()));
}
}
// In another service, retrieve and apply events
public void applyEvents(List<UserEvent> events) {
// Logic to apply each event to update the current state
}
Why: Event sourcing allows for replaying events which can help synchronize state across microservices effectively.
2. Implementation of Saga Pattern
The Saga pattern manages distributed transactions by allowing each service to handle its own updates while maintaining overall consistency through a series of compensating actions.
Code Example: Saga Implementation
public class OrderSaga {
public void createOrder(Order order) {
try {
userService.reserveItems(order.getItems());
paymentService.processPayment(order.getPaymentDetails());
} catch(Exception e) {
// Compensating actions
userService.releaseItems(order.getItems());
}
}
}
Why: The Saga pattern helps manage distributed transactions without requiring strong consistency, providing flexibility and fault tolerance.
3. Leverage Managed Database Services
Using managed database services like Amazon DynamoDB or Google Cloud Firestore can abstract away some challenges of data consistency. These services often provide built-in mechanisms like conditional writes, atomic transactions, and multi-region replication that help to maintain data integrity.
4. Implement a Data Access Layer (DAL)
Creating a Data Access Layer simplifies the complexity of managing data consistency. It abstracts data access for different services, allowing them to interact with data in a consistent framework.
Code Example: Implementing a DAL
public class UserDAL {
public User getUser(String userId) {
// Database call to fetch user
}
public void updateUser(User user) {
// Update user in the database with proper error handling
}
}
Why: A DAL ensures that all data access code is centralized, reducing redundancy and inconsistencies across microservices.
5. Strong Monitoring and Logging
Always remember: visibility is key. To effectively manage data consistency, you need robust logging and monitoring frameworks. Tools like AWS CloudWatch or Google Stackdriver can help you gain insights into data flow across your microservices.
Final Thoughts
Serverless microservices undeniably streamline development, but they also require a thoughtful approach to data management. Embracing patterns such as event sourcing and sagas, leveraging managed database services, and centralizing your data access can go a long way toward ensuring data consistency in your application.
For further reading, you can check out these resources: Microservices Data Management and Event Sourcing - Martin Fowler.
By understanding the pitfalls and strategies for overcoming data consistency challenges, developers can harness the full potential of serverless microservices architecture while providing a seamless experience for end users.