Why Your Express App Needs Rate Limiting Now
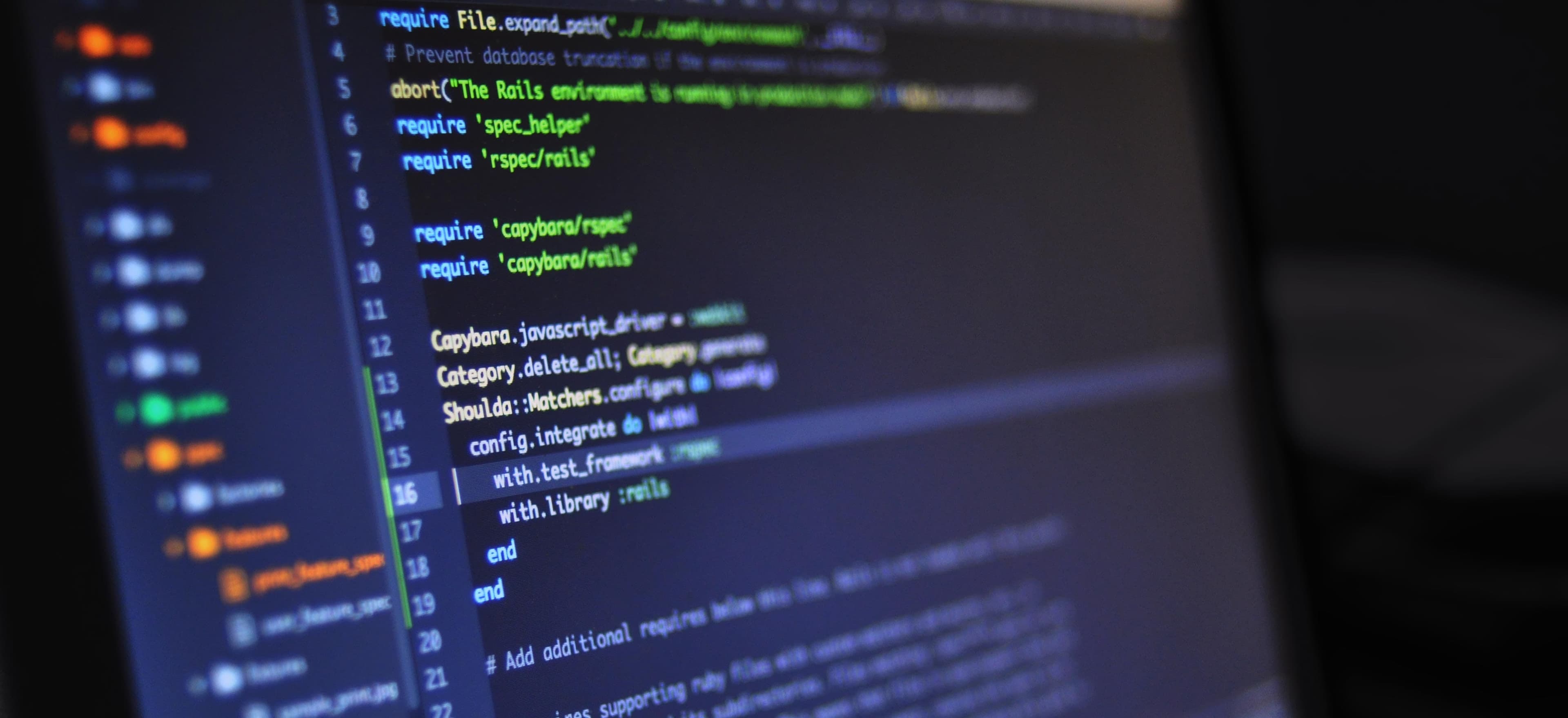
- Published on
Why Your Express App Needs Rate Limiting Now
In an era of increasing online services and endless interactions, ensuring the security of your web applications has never been more critical. One essential strategy to safeguard your Express application is rate limiting. In this blog post, we will explore what rate limiting is, why it's crucial for your application, and how to implement it effectively.
Understanding Rate Limiting
Rate limiting is the practice of controlling the number of requests a user can make to your application within a specific timeframe. This mechanism helps protect your app from abuse, such as DDoS attacks, brute-force attacks, and unintended user behavior that can lead to performance degradation.
Why Is Rate Limiting Important?
-
Preventing Abuse: Rate limiting helps mitigate the risk of overloading your server. If a malicious user attempts to flood your endpoint with requests, rate limiting ensures that only a set number of requests can be processed, protecting your app's health.
-
Ensuring Fair Usage: By restricting the number of requests, you provide an equitable experience for legitimate users. This prevents any single user from monopolizing your resources.
-
Enhancing Security: It acts as an additional layer of security to thwart attacks. For instance, in a brute-force attack, rate limiting would slow down an attacker’s attempts to guess a user's password.
-
Improving Performance: Rate limiting can improve application performance by ensuring that your server is not overwhelmed with excessive requests, allowing it to operate efficiently.
How to Implement Rate Limiting in Your Express App
To implement rate limiting in an Express application, you can use middleware like express-rate-limit
. This package offers a straightforward way to manage the rate of incoming requests.
Step-by-Step Implementation
- Install express-rate-limit:
First, you need to add the package to your project. Use npm to install it:
npm install express-rate-limit
- Set Up the Rate Limiter:
Next, you can create a rate limiter for your application. Here is an example setup:
const express = require('express');
const rateLimit = require('express-rate-limit');
const app = express();
// Create a rate limiter
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // Limit each IP to 100 requests per windowMs
message: 'Too many requests from this IP, please try again later.'
});
// Apply to all requests
app.use(limiter);
app.get('/', (req, res) => {
res.send('Welcome to the homepage!');
});
app.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
Explanation of the Code
-
windowMs: This specifies the time frame (in milliseconds) for which requests are counted. In this case, each user is allowed a maximum of 100 requests every 15 minutes.
-
max: This parameter defines the maximum number of requests from a single IP in the specified time window.
-
message: This custom message is sent when a user exceeds the allowed number of requests.
By applying the limiter middleware globally using app.use(limiter)
, you ensure that all incoming requests will be subject to this rate limiting.
Customizing Rate Limiting
You may want to have various rate limits for different routes or user roles. Here's how you can customize it per route:
// Limit for login route
const loginLimiter = rateLimit({
windowMs: 5 * 60 * 1000, // 5 minutes
max: 10, // Limit each IP to 10 login requests per windowMs
message: 'Too many login attempts from this IP, please try again later.'
});
app.post('/login', loginLimiter, (req, res) => {
// Handle login
});
In this scenario, we want to limit login attempts to 10 per IP every 5 minutes. This can prevent brute-force attacks specifically on your login endpoints, enhancing security further.
Monitoring and Logging
Integrating logging with rate limiting can provide valuable insights into patterns of abuse:
const rateLimit = require('express-rate-limit');
const morgan = require('morgan');
app.use(morgan('combined')); // Logs HTTP requests
const limiter = rateLimit({
// same configuration as before
onLimitReached: (req, res) => {
console.warn(`Rate limit exceeded by IP: ${req.ip}`);
}
});
Here we utilize morgan
, a middleware for logging HTTP requests, to keep a log of all requests. Additionally, using the onLimitReached
callback, we can log an event whenever a user exceeds the rate limit.
Final Considerations
In summary, rate limiting is an essential practice for any application, especially those built with Express. It helps prevent abuse, ensure fair resource distribution, bolster security, and improve performance. By using the express-rate-limit
library, implementing rate limiting is not only easy but also essential for maintaining a robust web application.
For further reading on securing your Express applications, you may find OWASP's Top Ten valuable, and be sure to check out express-rate-limit documentation for additional customization options. Implementing these practices will put your application on the path to robust performance and security.
Now is the time to integrate rate limiting into your Express application. Make the move, and protect your digital assets!