Troubleshooting JDBC Boolean Compatibility Issues
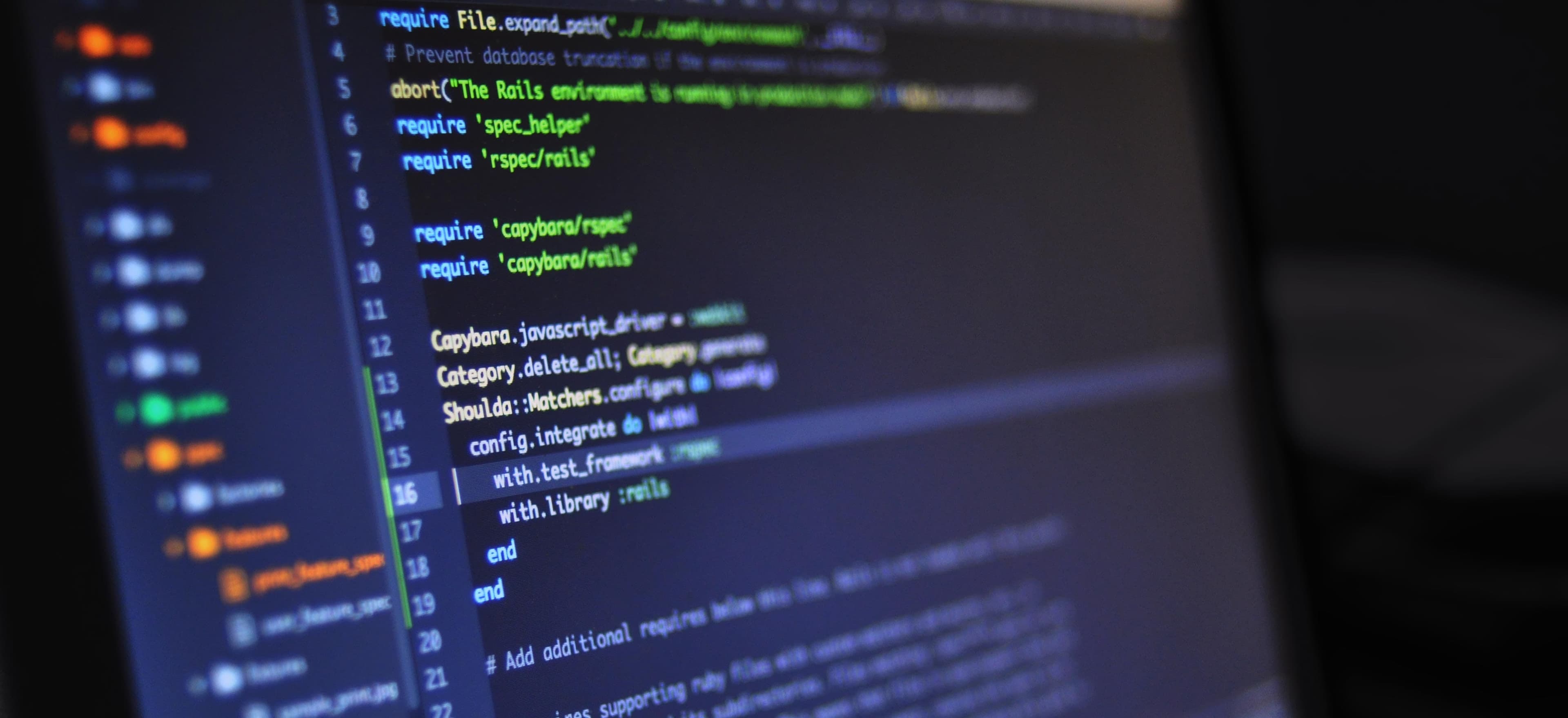
- Published on
Troubleshooting JDBC Boolean Compatibility Issues
Java Database Connectivity (JDBC) is a crucial API for connecting and executing queries against databases in Java applications. However, developers often encounter compatibility issues when dealing with Boolean values between Java and various database systems. This blog post will delve into the intricacies of JDBC Boolean compatibility, explore common pitfalls, and provide practical solutions to troubleshoot these challenges.
Understanding JDBC and Boolean Types
What is JDBC?
JDBC is an API that allows Java applications to interact with a wide range of databases. It acts as a bridge between Java applications and databases, enabling the execution of SQL statements and retrieval of results.
Boolean in Java and SQL
In Java, boolean
is a primitive data type that can hold two values: true
and false
. In SQL databases, Boolean types can vary:
- Some databases (like PostgreSQL) have a native
BOOLEAN
type. - Others (like MySQL) may represent Boolean values using numeric types (
TINYINT(1)
, where 0 is false and 1 is true). - Some databases might use strings ('Y'/'N' or 'TRUE'/'FALSE').
Why Compatibility Issues Arise
The differences in how Boolean values are represented can lead to issues in JDBC operations. When a Java boolean
is set into a SQL query, it might not map correctly if the destination database treats Boolean values differently.
Common JDBC Boolean Compatibility Issues
Issue 1: Mapping Differences
Mapping between Java boolean and SQL Boolean can cause problems. For example, inserting a Java boolean
into a MySQL TINYINT
field may not function as expected because JDBC interprets boolean
as different underlying types.
Issue 2: SQL Dialect Differences
Different SQL dialects handle Boolean types in a unique way. If you are utilizing multiple databases or migrating from one to another, these differences can cause unexpected behavior during runtime.
Issue 3: ResultSet Retrieval
Fetching Boolean values can lead to mistakes, especially when using methods that do not explicitly specify expected types. For instance, fetching a BOOLEAN
value using getInt()
in a context expecting a Boolean could return an incompatible type leading to exceptions.
Troubleshooting JDBC Boolean Compatibility Issues
To effectively troubleshoot these issues, we need to adhere to best practices. Below are some strategies:
Use Appropriate Methods for Setting PreparedStatement Parameters
When setting boolean values, always use the appropriate JDBC method. Consider the following example:
String sqlInsert = "INSERT INTO your_table (is_active) VALUES (?)";
try (PreparedStatement pstmt = connection.prepareStatement(sqlInsert)) {
boolean isActive = true;
pstmt.setBoolean(1, isActive); // Correct way to set boolean
pstmt.executeUpdate();
}
Why?: Using setBoolean()
ensures that JDBC correctly interprets the Java boolean value, converting it to the corresponding SQL representation.
Handle ResultSet Retrieval Carefully
When retrieving Boolean values from the database, ensure you’re using the right method:
String sqlSelect = "SELECT is_active FROM your_table WHERE id = ?";
try (PreparedStatement pstmt = connection.prepareStatement(sqlSelect)) {
pstmt.setInt(1, 1);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
boolean isActive = rs.getBoolean("is_active"); // Correct method for retrieving boolean
System.out.println("Active status: " + isActive);
}
}
Why?: Using getBoolean()
straightforwardly retrieves the value as a boolean, reducing the risk of misinterpretation.
Ensure Database Compatibility
Make sure your database schema accurately reflects your intended use of boolean types. If you're using MySQL and meant to create a boolean field, your table definition should look something like this:
CREATE TABLE your_table (
id INT PRIMARY KEY,
is_active TINYINT(1) NOT NULL
);
Why?: Specifying the correct data types in your database helps to prevent confusion and ensures smoother interoperability with JDBC.
Utilize Dialect-Specific Libraries when Needed
If you are working with various databases or facing significant compatibility issues, consider using dialect-specific libraries or ORM tools like Hibernate. These libraries abstract much of the complexity associated with database interaction.
Example Code Illustrating Troubleshooting Steps
Here’s a complete Java code snippet incorporating our best practices:
import java.sql.*;
public class BooleanTest {
private static final String DB_URL = "jdbc:mysql://localhost:3306/your_db";
private static final String USER = "root";
private static final String PASS = "password";
public static void main(String[] args) {
try (Connection connection = DriverManager.getConnection(DB_URL, USER, PASS)) {
// Insert example
insertActiveStatus(connection, true);
// Fetching example
fetchActiveStatus(connection, 1);
} catch (SQLException e) {
e.printStackTrace();
}
}
private static void insertActiveStatus(Connection connection, boolean isActive) throws SQLException {
String sqlInsert = "INSERT INTO your_table (is_active) VALUES (?)";
try (PreparedStatement pstmt = connection.prepareStatement(sqlInsert)) {
pstmt.setBoolean(1, isActive);
pstmt.executeUpdate();
System.out.println("Inserted active status: " + isActive);
}
}
private static void fetchActiveStatus(Connection connection, int id) throws SQLException {
String sqlSelect = "SELECT is_active FROM your_table WHERE id = ?";
try (PreparedStatement pstmt = connection.prepareStatement(sqlSelect)) {
pstmt.setInt(1, id);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
boolean isActive = rs.getBoolean("is_active");
System.out.println("Active status fetched: " + isActive);
}
}
}
}
Why this approach?: This code illustrates reading from and writing to the database using the correct JDBC methods for boolean values, minimizing compatibility issues.
A Final Look
Troubleshooting JDBC Boolean compatibility issues requires a keen understanding of data types and their representations across Java and your chosen database. By following best practices—utilizing the correct JDBC methods for setting and retrieving Boolean values, ensuring your database schema's integrity, and considering ORM solutions for complex mappings—you can significantly mitigate potential issues.
For further reading on JDBC and database interoperability, refer to Oracle's JDBC Documentation or check out frameworks like Hibernate.
By honing your approach to Boolean handling in JDBC, you pave the way for smoother database operations in your Java applications. Happy coding!