Overcoming Common Akka Pitfalls for Beginners
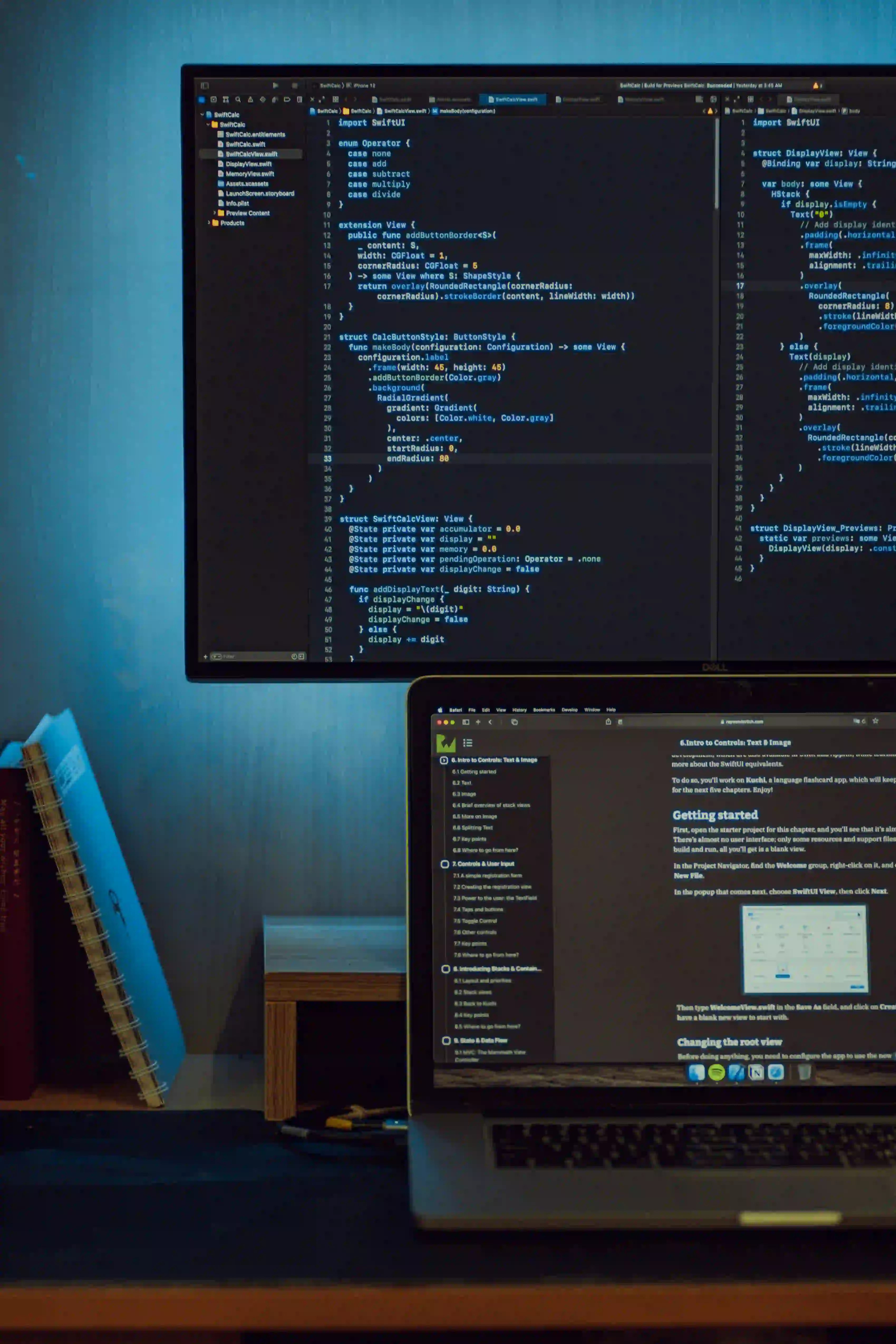
Overcoming Common Akka Pitfalls for Beginners
Akka, the powerful toolkit for building concurrent and distributed applications in Java, is a game-changer for many developers. However, as with any new technology, beginners often encounter challenges that can hinder their progress. In this blog post, we will discuss common pitfalls that beginners face when working with Akka and provide effective strategies to overcome them.
Table of Contents
- Understanding the Actor Model
- Configuration Mistakes
- Actor Lifecycle Mismanagement
- Actor Message Handling
- Testing Strategies
- Conclusion
1. Understanding the Actor Model
Before diving into coding, it's crucial to grasp the Actor Model, which is the cornerstone of Akka. The Actor Model simplifies concurrent programming by decoupling the way data is processed and how it is communicated.
Pitfall: Many newcomers misunderstand the concept of actors — thinking of them as threads rather than lightweight, concurrent entities.
Solution: Remember that each actor in Akka is designed to be isolated. They don’t share state and communicate exclusively through messages.
import akka.actor.AbstractActor;
import akka.actor.ActorRef;
import akka.actor.ActorSystem;
import akka.actor.Props;
public class SimpleActor extends AbstractActor {
@Override
public Receive createReceive() {
return receiveBuilder()
.match(String.class, this::onReceiveMessage)
.build();
}
private void onReceiveMessage(String message) {
System.out.println("Received message: " + message);
}
public static void main(String[] args) {
ActorSystem system = ActorSystem.create("MyActorSystem");
ActorRef actorRef = system.actorOf(Props.create(SimpleActor.class), "simpleActor");
actorRef.tell("Hello, Akka!", ActorRef.noSender());
}
}
In this code, we create a simple actor that prints received messages. Notice how the actor handles messages in its createReceive()
method. This separation helps manage state effectively.
2. Configuration Mistakes
Another common issue arises from Akka's configuration. Akka applications require a detailed configuration file, typically application.conf
, to function optimally.
Pitfall: Beginners often overlook critical settings, leading to performance issues or unexpected behavior.
Solution: Always start with a basic configuration that specifies the actor system name, dispatcher configuration, and log levels. Here's a minimal example:
akka {
actor {
provider = "akka.actor.LocalActorRefProvider"
}
loglevel = "DEBUG"
}
This configuration sets the log level to DEBUG, allowing you to see detailed logs that can aid in debugging. For more configuration options, you can check the Akka Documentation.
3. Actor Lifecycle Mismanagement
Actors have a lifecycle, and managing it incorrectly can lead to resource leaks or unexpected behaviors.
Pitfall: Beginners may not handle actor pre-start and post-stop events properly.
Solution: Implement preStart()
and postStop()
methods to manage resources effectively. For example:
@Override
public void preStart() {
System.out.println("Actor is starting!");
}
@Override
public void postStop() {
System.out.println("Actor has stopped!");
}
Finding the right balance in handling the lifecycle can lead to smoother applications. Failure to utilize these methods is a common misstep that results in complications later in development.
4. Actor Message Handling
Message handling is at the core of the Actor Model.
Pitfall: Newcomers often use the wrong message types or neglect to define message objects properly, leading to runtime errors.
Solution: Use dedicated message classes to encapsulate your data.
public class Greeting {
public final String message;
public Greeting(String message) {
this.message = message;
}
}
private void onReceiveMessage(Greeting greeting) {
System.out.println("Greeting received: " + greeting.message);
}
Using a dedicated message class makes the code more readable and maintainable. It also ensures that you are working with type-safety throughout your application.
5. Testing Strategies
Testing in asynchronous environments can be tricky, especially with actors.
Pitfall: Beginners may try to test actors in a synchronous manner, leading to unreliable tests.
Solution: Utilize Akka TestKit, which provides tools to interact with actors and validate their behaviors.
import akka.actor.ActorSystem;
import akka.actor.Props;
import akka.testkit.javadsl.TestKit;
import org.junit.AfterClass;
import org.junit.BeforeClass;
import org.junit.Test;
public class SimpleActorTest {
static ActorSystem system;
@BeforeClass
public static void setup() {
system = ActorSystem.create("TestSystem");
}
@AfterClass
public static void teardown() {
TestKit.shutdownActorSystem(system);
}
@Test
public void testGreeting() {
new TestKit(system) {{
final Props props = Props.create(SimpleActor.class);
final var actorRef = system.actorOf(props);
actorRef.tell(new Greeting("Hello, Test!"), getRef());
expectMsg("Greeting received: Hello, Test!");
}};
}
}
This test checks if the actor processes a greeting message correctly. Note how we use TestKit
to create an actor system for testing and validate the expected message. For more on testing with Akka, see the Akka Testing Documentation.
Closing the Chapter
Navigating the Akka landscape can be daunting, especially for beginners. By understanding the Actor Model deeply, maintaining proper configuration, managing the actor lifecycle, handling messages appropriately, and implementing robust testing strategies, you can avoid many of the common pitfalls associated with Akka development.
Take the time to familiarize yourself with the tools at your disposal and don't hesitate to refer to the official Akka documentation for more advanced topics. Remember, each step you take toward mastery not only enhances your skills but also contributes to the creation of more efficient and scalable applications. Happy coding!