Fixing Common Cobertura & Maven Code Coverage Issues
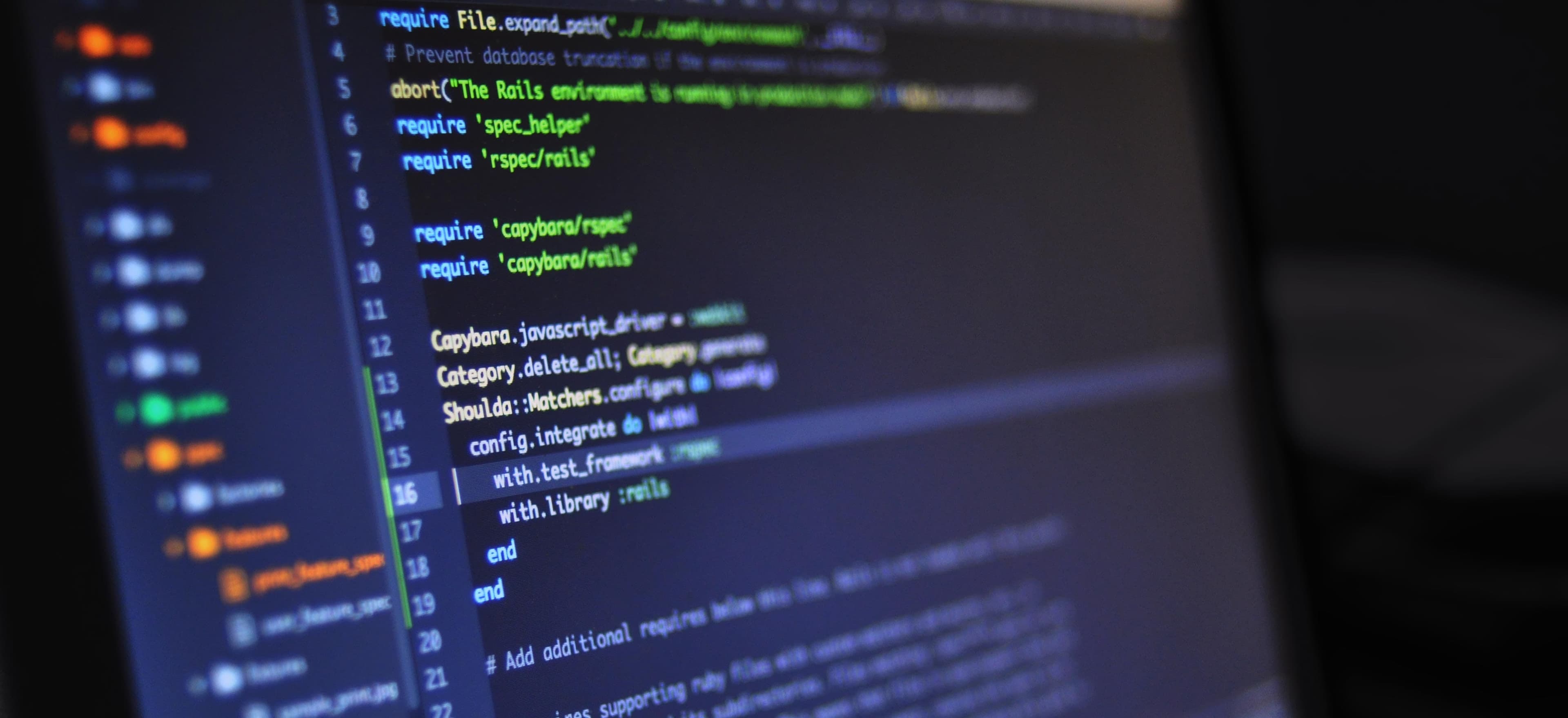
- Published on
Fixing Common Cobertura & Maven Code Coverage Issues
Code coverage is a critical aspect of software development. It ensures that your tests effectively verify your code's performance, reliability, and functionality. In Java, developers often utilize tools like Cobertura to measure code coverage. When using Cobertura with Maven, various issues may arise that can halt your productivity. This blog post explores common Cobertura and Maven code coverage issues and how to fix them.
Understanding Code Coverage
Before diving into solutions, let’s briefly discuss what code coverage is and why it matters. Code coverage measures how much of your source code is executed when your tests run. It helps identify untested parts of your application, ensuring that potential bugs don’t go unnoticed. Developers can use various tools, including Jacoco and Cobertura, to achieve this.
With Cobertura, Maven users can generate detailed reports in various formats, including HTML, XML, and text, providing insights into coverage percentages. However, settings sometimes need adjustments or explanations to enable seamless integration.
Common Issues with Cobertura and Maven
1. Installation and Configuration Issues
Often, developers encounter issues with the installation of Cobertura through Maven. Misconfiguring your pom.xml
can lead to conflicting versions or missing dependencies.
Solution: Basic Setup
You need to include the Cobertura Plugin in your Maven project as follows:
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>cobertura-maven-plugin</artifactId>
<version>2.7</version>
<configuration>
<format>html</format>
</configuration>
<executions>
<execution>
<goals>
<goal>cobertura</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Why This Works:
- This configuration ensures that the Cobertura plugin is appropriately set up.
- The
<configuration>
tag allows you to specify the report format you want.
2. Filters Not Working
Sometimes, the filters configured to exclude certain classes might not work as expected. This can occur due to incorrect regex patterns.
Solution: Correct Empty Filters
When excluding classes, use proper naming conventions and check for case sensitivity:
<configuration>
<excludes>
<exclude>your/package/to/exclude/*</exclude>
</excludes>
</configuration>
Why This Works:
- The
exclude
syntax allows you to pinpoint the classes suffering from code coverage problems. Ensuring the correct package path prevents clutter and provides clearer reports.
3. Aggregated Reports Not Displaying
In multi-module Maven projects, you might notice that the coverage report does not aggregate correctly across modules.
Solution: Generate Reports at the Parent Level
Make sure your parent pom.xml
has the following configurations:
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>cobertura-maven-plugin</artifactId>
<version>2.7</version>
<executions>
<execution>
<goals>
<goal>aggregate</goal>
</goals>
</execution>
</executions>
</plugin>
Why This Works:
- This ensures you get a consolidated report for your entire project, improving visibility of the overall coverage beyond individual modules.
4. No Coverage Data Generated
Many times, you may run the Maven command, but the reports lack data or show 0% coverage
.
Solution: Ensure Tests are Running
To solve the problem, validate that your tests are executing before the Cobertura goals. You can achieve this by altering the default phase of your build lifecycle:
<executions>
<execution>
<id>cobertura</id>
<phase>test</phase>
<goals>
<goal>cobertura</goal>
</goals>
</execution>
</executions>
Why This Works:
- This configuration ensures that coverage data is generated immediately after running tests, leading to an accurate report.
Advanced Configuration Options
Once you’ve resolved basic issues, you might need to explore advanced configurations for better control over your reports.
1. Output Directory Customization
Sometimes, you may want the reports to reside in a specific directory apart from the default target directory.
Solution: Custom Report Directory
<reporting>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>cobertura-maven-plugin</artifactId>
<version>2.7</version>
<configuration>
<outputDirectory>${project.build.directory}/custom-reports</outputDirectory>
</configuration>
</plugin>
</plugins>
</reporting>
Why This Works:
- This helps organize your reports, making it easier to locate them when analyzing code coverage.
2. Integration with CI/CD
For projects utilizing Continuous Integration/Continuous Deployment (CI/CD) pipelines, integrating Cobertura with tools like Jenkins is a must-check.
Solution: CI Integration Example
In a Jenkins pipeline, include a build step to execute:
mvn clean test cobertura:cobertura
Why This Works:
- This ensures that code coverage is evaluated every time your code is pushed or merged, maintaining code quality standards.
Lessons Learned
In summary, while Cobertura offers robust functionalities for measuring code coverage in Java applications, common issues can disrupt your workflow. By understanding and addressing these problems systematically—through proper configurations, command executions, and advanced setups—you can improve the efficacy of your tests and ultimately enhance your application’s quality.
For more insights on test coverage and configuration management, you can refer to the official Cobertura documentation and explore Maven's documentation for effective project management techniques.
As you implement these solutions, keep in mind the importance of regularly revisiting your coverage standards—continuous improvements lead to superior software. Happy coding!