Overcoming Common Concurrency Pitfalls for Beginners
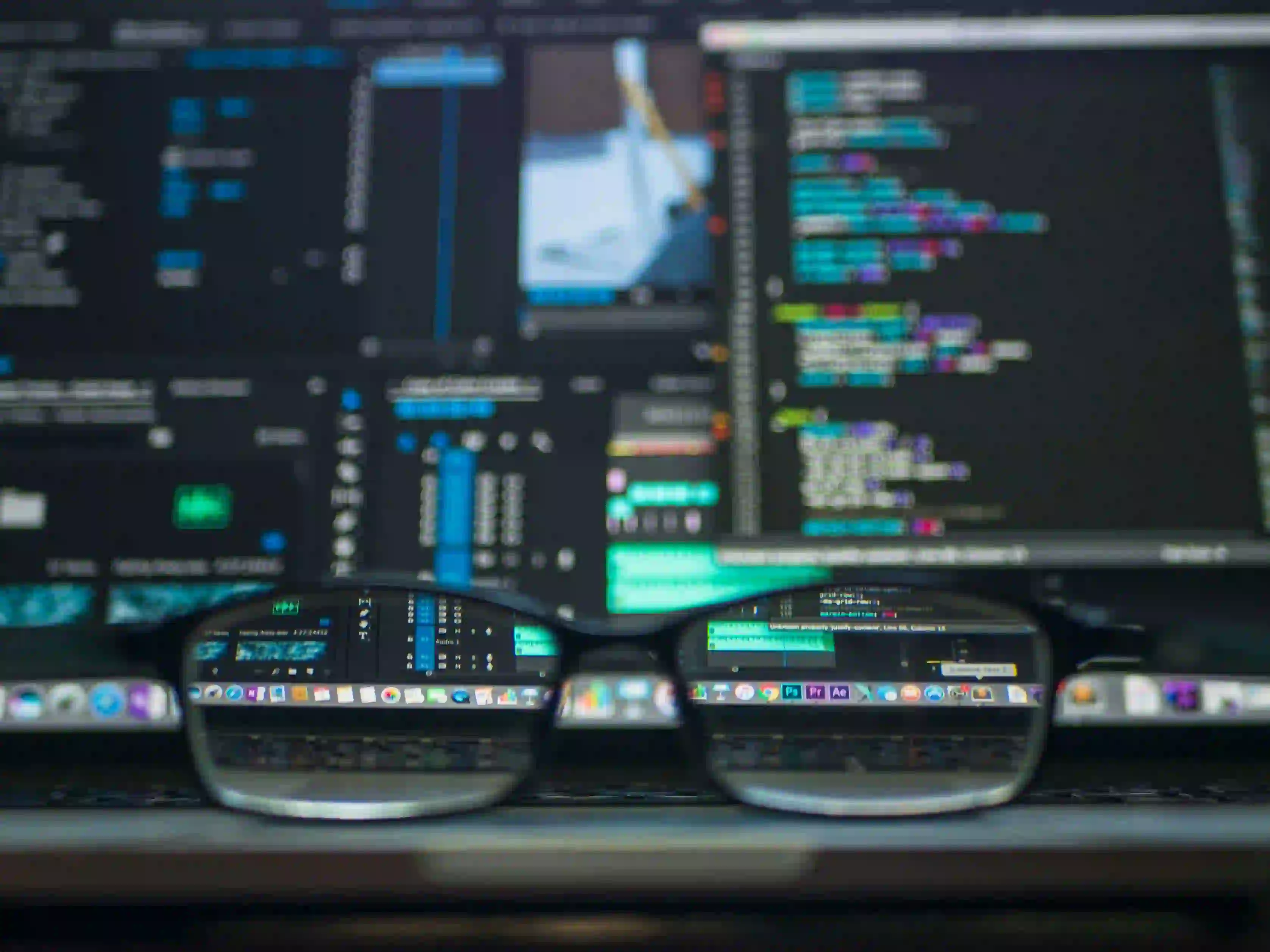
Overcoming Common Concurrency Pitfalls for Beginners in Java
Concurrency in Java is one of the most thrilling yet challenging aspects of the language. It allows your applications to perform many tasks at once, leading to more efficient use of system resources. However, with great power comes great responsibility. Beginners often encounter pitfalls that can lead to bugs, performance issues, or even crashes. In this blog post, we will delve into these common pitfalls, providing examples and solutions to help you navigate the world of concurrency with confidence.
Understanding Concurrency
Before we tackle the pitfalls, it’s essential to understand what concurrency is and why it is important. Concurrency refers to the ability of the program to execute multiple tasks simultaneously. In Java, this is commonly achieved through threads. Threads are like separate paths of execution that can work independently but share the same resources. The Java Platform provides robust libraries for managing and utilizing threads effectively.
Common Concurrency Pitfalls
1. Race Conditions
A race condition occurs when two or more threads try to modify shared data simultaneously. When this happens, the final outcome depends on the timing of how the threads are scheduled, leading to unpredictable results.
Example:
public class Counter {
private int count = 0;
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
In the example above, if two threads call increment()
simultaneously, you may not get the expected count. The increment operation is not atomic, which means it can be interrupted.
Solution:
Use the synchronized
keyword to ensure that only one thread can execute the method at a time.
public synchronized void increment() {
count++;
}
By synchronizing the method, you enforce a lock that prevents other threads from accessing it while one thread is executing.
2. Deadlocks
Deadlocks occur when two or more threads are blocked forever while waiting for each other to release resources. This situation can freeze your application entirely.
Example:
public class DeadlockExample {
private final Object lock1 = new Object();
private final Object lock2 = new Object();
public void method1() {
synchronized (lock1) {
synchronized (lock2) {
// Perform operations
}
}
}
public void method2() {
synchronized (lock2) {
synchronized (lock1) {
// Perform operations
}
}
}
}
In this example, if method1()
locks lock1
and then waits for lock2
, while another thread runs method2()
and locks lock2
first, both methods will wait indefinitely.
Solution:
Design your synchronization hierarchy so that all threads acquire locks in a consistent order. Alternatively, you can use java.util.concurrent
package features like ReentrantLock
with timeouts to detect deadlocks.
3. Starvation
Starvation occurs when one or more threads are perpetually denied access to resources they need for execution while other threads are continually given preference. This can happen, for example, when using unfair synchronization policies.
Example:
public class StarvationExample {
public void methodWithLock() {
synchronized (this) {
// Perform operations
}
}
}
If there are multiple threads and one thread continuously acquires the lock, others may be unable to proceed, leading to starvation.
Solution:
Using fair locks can help eliminate starvation. This can be accomplished using the ReentrantLock
with a true
parameter for fairness.
ReentrantLock lock = new ReentrantLock(true);
4. Not Shutting Down Executors Properly
When using ExecutorService, failing to shut down correctly can lead to memory leaks or resources not being released. If you don't shut down the executor, it holds onto resources even when they are no longer needed.
Example:
ExecutorService executor = Executors.newFixedThreadPool(5);
// Submit tasks to executor
// No shutdown
Solution:
Always ensure proper shutdown of the executor service, especially in production environments.
executor.shutdown();
try {
if (!executor.awaitTermination(60, TimeUnit.SECONDS)) {
executor.shutdownNow();
}
} catch (InterruptedException e) {
executor.shutdownNow();
}
5. Ignoring Thread Safety in Collections
Java provides various collections, but not all of them are thread-safe. For instance, ArrayList
is not synchronized, meaning it cannot be used safely by multiple threads.
Example:
List<Integer> list = new ArrayList<>();
// Multiple threads modifying the list
Solution:
Use thread-safe collections such as CopyOnWriteArrayList
, or encapsulate your ArrayList
within Collections.synchronizedList
.
List<Integer> safeList = Collections.synchronizedList(new ArrayList<>());
6. Overusing Synchronization
While synchronization is essential for maintaining data integrity, overusing it can lead to performance bottlenecks. If every operation on shared resources is synchronized, you may end up with a significant delay due to waiting threads.
Example:
public synchronized void updateData() {
// Update logic
}
Solution:
Analyze your code to determine if certain operations can be executed without synchronization or if finer-grained locks are more efficient.
Best Practices for Concurrency in Java
1. Prefer High-Level Concurrency Constructs
Java’s java.util.concurrent
package provides high-level constructs like Executors
, CountDownLatch
, and Semaphore
. These can help manage concurrency more effectively than dealing with threads directly.
2. Use Immutable Objects
Where possible, use immutable objects. Since immutable objects cannot be changed after they are created, they are inherently thread-safe.
3. Keep Critical Sections Short
Minimize the amount of code inside synchronized blocks to reduce waits for other threads. Keeping critical sections short makes it less likely that other threads will be blocked.
4. Test Concurrency
Testing concurrent programs can be challenging. Tools like JUnit
can help in writing tests, but consider using specialized frameworks like ConcurrencyTest
or Java Concurrency in Practice
to validate the behavior under concurrent scenarios.
For comprehensive Java concurrency documentation, visit Oracle's Java Concurrency Tutorial.
Closing Remarks
In summary, concurrency is a double-edged sword in Java. Understanding these common pitfalls is crucial for building robust, efficient applications. By applying the solutions discussed, you’ll be better equipped to handle concurrency in Java. For more resources and deeper insights, consider exploring Java Concurrency in Practice, a definitive guide on the subject.
Keep learning, keep coding, and with practice, you will become proficient in managing concurrency in Java!