Efficient File Parsing Using Java 8 Stream API
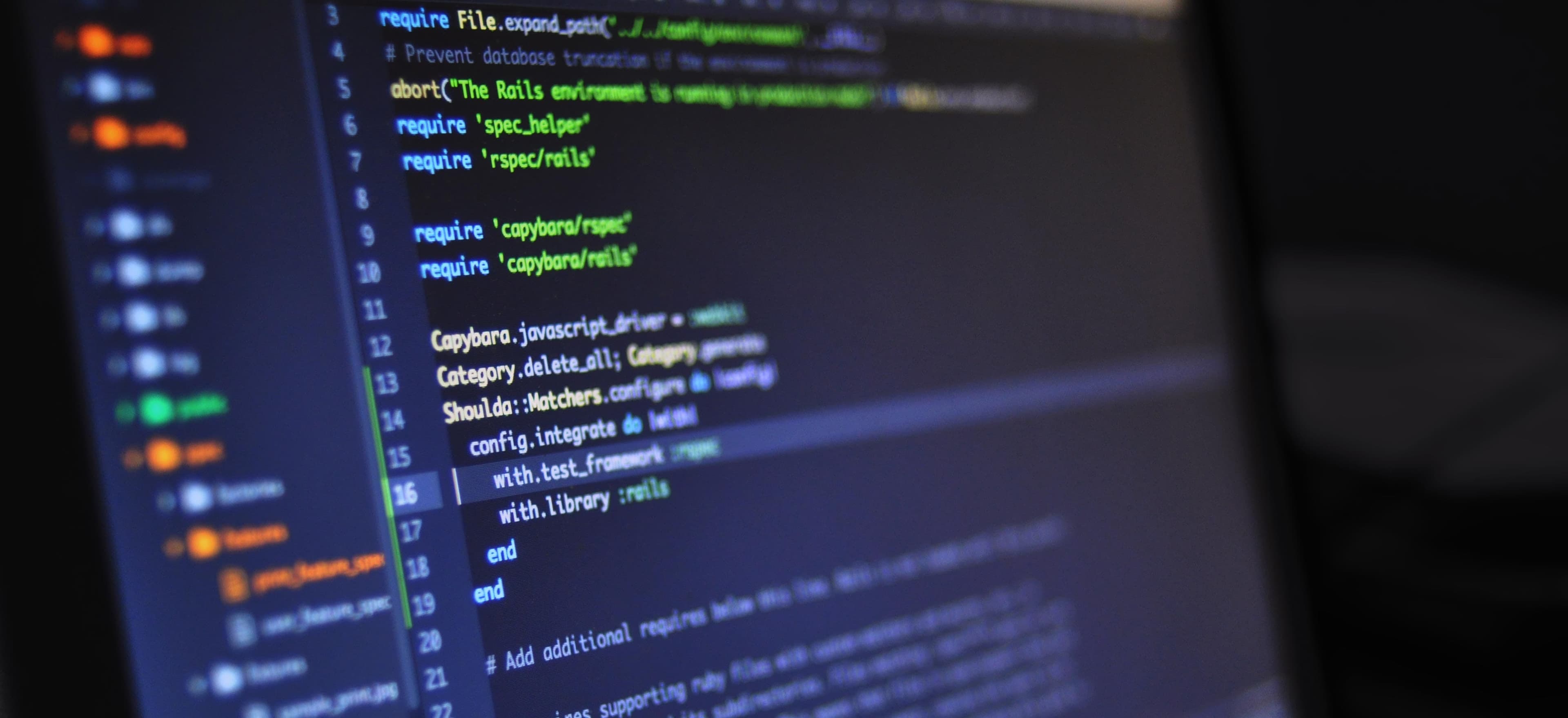
- Published on
Efficient File Parsing Using Java 8 Stream API
In the world of Java programming, file parsing is a common task. Whether you are dealing with log files, CSV files, or any other type of structured data, parsing files efficiently is crucial for the performance of your application. In this article, we will explore how to utilize the Java 8 Stream API to parse files in a more efficient and concise manner.
Understanding the Java 8 Stream API
The Stream API in Java 8 provides a powerful and expressive way to process data in a functional style. It allows developers to perform operations on a sequence of elements, such as filtering, mapping, and reducing, using high-level abstractions.
One of the key advantages of using the Stream API for file parsing is its ability to process data in a lazy and efficient manner. Instead of loading the entire file into memory at once, which can be memory-intensive for large files, the Stream API allows for processing data in a pipelined fashion, only pulling in as much data as needed for each operation.
This makes it an ideal choice for parsing large files while keeping memory consumption to a minimum.
Parsing a CSV File Using Java 8 Stream API
Let's consider a scenario where we have a large CSV file containing sales data, and we want to parse this file to calculate the total sales amount. Traditionally, this would involve reading the file line by line, splitting each line by the comma, and then processing the individual fields. However, with the Stream API, we can achieve this in a more elegant and efficient way.
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
public class CsvFileParser {
public static void main(String[] args) {
String fileName = "sales.csv";
try {
double totalSales = Files.lines(Paths.get(fileName))
.skip(1) // Skip the header
.mapToDouble(line -> Double.parseDouble(line.split(",")[1]))
.sum();
System.out.println("Total sales: " + totalSales);
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this example, we use the Files.lines
method to create a stream of lines from the CSV file. We then use the skip
method to ignore the header line, mapToDouble
to extract the sales amount from each line, and finally sum
to calculate the total sales amount.
This approach is not only concise but also efficient, as it processes the file line by line without loading the entire file into memory.
Benefits of Using Java 8 Stream API for File Parsing
- Efficiency: By processing data lazily, the Stream API reduces memory overhead, making it suitable for parsing large files.
- Conciseness: With its fluent and expressive syntax, the Stream API allows for more readable and maintainable code compared to traditional looping constructs.
- Parallelism: The Stream API seamlessly supports parallel processing, enabling concurrent execution of operations on large data sets.
Applying Stream Operations for Advanced File Parsing
Beyond basic operations like mapping and filtering, the Stream API offers a wide range of intermediate and terminal operations that can be leveraged for advanced file parsing tasks.
For instance, let's consider a scenario where we have a log file containing various events, and we want to extract and count the occurrences of a specific event type.
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.Map;
import java.util.stream.Collectors;
public class LogFileParser {
public static void main(String[] args) {
String fileName = "events.log";
String eventType = "ERROR";
try {
Map<String, Long> eventCountByType = Files.lines(Paths.get(fileName))
.filter(line -> line.contains(eventType))
.collect(Collectors.groupingBy(e -> eventType, Collectors.counting()));
System.out.println("Occurrences of " + eventType + ": " + eventCountByType.get(eventType));
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this example, we use the filter
method to only retain lines containing the specified event type, and then use the collect
method along with groupingBy
and counting
collectors to aggregate and count the occurrences of the event type.
Key Takeaways
The Java 8 Stream API revolutionized the way data processing is performed in Java. When it comes to file parsing, leveraging the Stream API can bring about significant improvements in terms of efficiency, readability, and maintainability.
By embracing the functional programming paradigm and taking advantage of lazy evaluation, parallelism, and a rich set of stream operations, developers can parse files more effectively and make their applications more robust and performant.
In this article, we explored the benefits of using the Java 8 Stream API for file parsing and demonstrated how stream operations can be applied to parse CSV and log files in a concise and efficient manner.
To delve deeper into file parsing and the Stream API, check out the official Java Stream API documentation and experiment with various stream operations to enhance your file parsing skills.