Mastering String Trimming: No more Extra Spaces in Java!
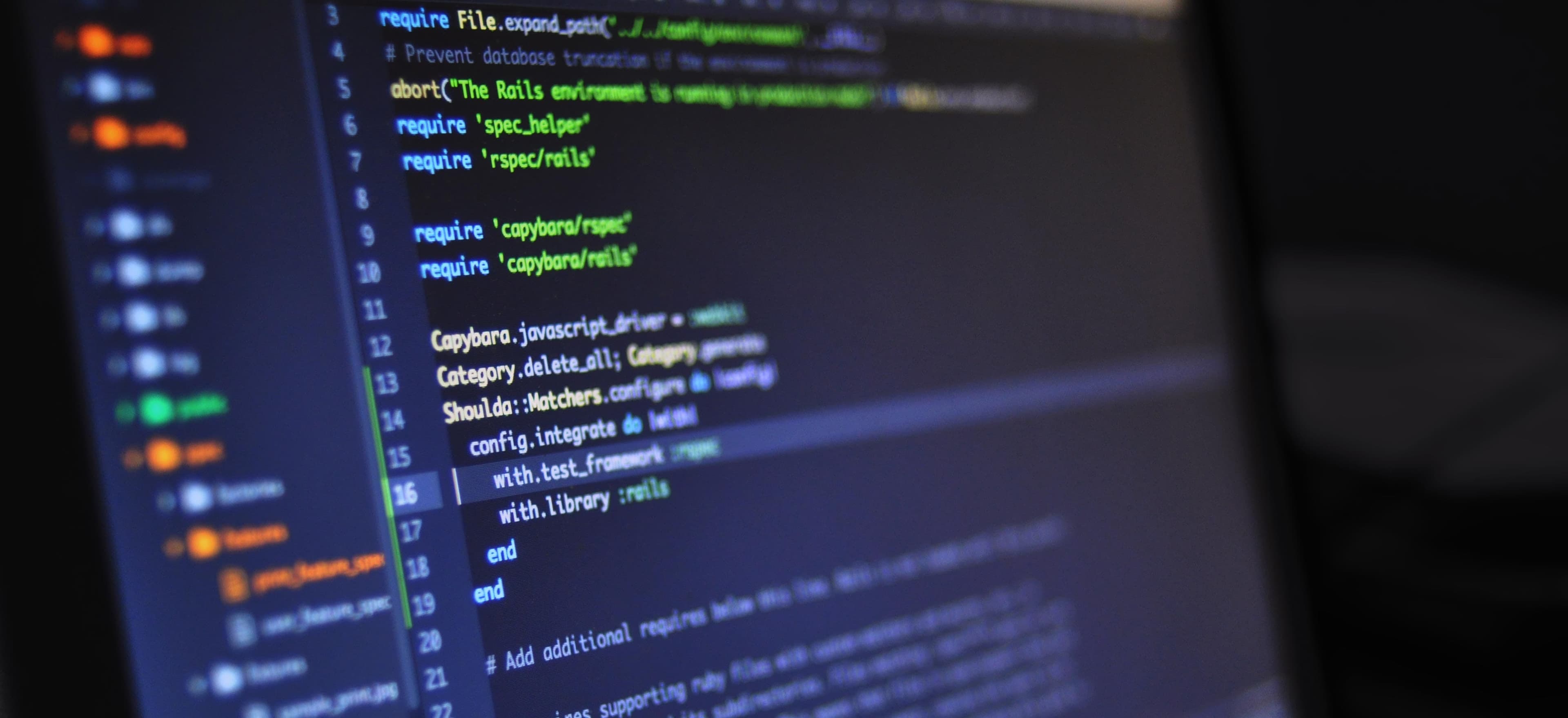
- Published on
Mastering String Trimming: No More Extra Spaces in Java!
In this blog post, we will explore a fundamental aspect of string manipulation in Java: trimming extra spaces. Understanding how to effectively handle strings is a key skill for any Java developer. Whether you're developing a simple application or a complex enterprise-level system, managing extra spaces can help improve data processing and enhance user experience.
Table of Contents
- What is String Trimming?
- The Importance of Trimming Strings
- Java String Trimming Methods
- Code Examples
- Conclusion
1. What is String Trimming?
String trimming refers to the process of removing unnecessary spaces from both the beginning and the end of a string. For example, the string " Hello, World! " can be trimmed to "Hello, World!" This is a common requirement in applications where input may come from users, databases, or external sources.
Why Trim Strings?
- Data Integrity: Extra spaces can lead to unexpected behavior, especially in comparisons.
- Presentation: Clean strings enhance UI elements.
- Storage Efficiency: Removing unnecessary spaces can save memory in large-scale applications.
2. The Importance of Trimming Strings
Imagine receiving user input, such as usernames or email addresses, that include extra spaces. These spaces can trigger issues such as mismatched values in databases, leading to failed logins or data inconsistency.
Consider the following scenarios:
- Username: " user123 " should match "user123".
- Email: " example@test.com " should be treated as "example@test.com".
By trimming strings, you avoid these pitfalls and ensure smooth data processing.
3. Java String Trimming Methods
Java offers several built-in methods for trimming strings:
String.trim()
: The most straightforward method for removing leading and trailing whitespace.String.replaceAll()
: A more flexible option to remove internal spaces or patterns using regular expressions.- Java 11's
String.strip()
: This method allows for Unicode space removal and is more comprehensive.
Use Cases
-
String.trim()
:- Suitable for basic use cases.
- Removes spaces based on ASCII values.
-
String.replaceAll()
:- Ideal for more complex patterns.
- Allows for extensive manipulation beyond simple spaces.
-
String.strip()
:- Perfect for modern applications needing Unicode compatibility.
4. Code Examples
Example 1: Basic Trimming
public class StringTrimExample {
public static void main(String[] args) {
String original = " Hello, World! ";
String trimmed = original.trim();
System.out.println("Original: '" + original + "'");
System.out.println("Trimmed: '" + trimmed + "'");
}
}
Commentary:
In this basic example, we are using the trim()
method to remove leading and trailing spaces. This method is quick and easy to implement for common string inputs.
Example 2: Removing All Spaces
public class StringReplaceExample {
public static void main(String[] args) {
String original = " Hello, World! ";
String noSpaces = original.replaceAll("\\s+", " ").trim();
System.out.println("Original: '" + original + "'");
System.out.println("No spaces: '" + noSpaces + "'");
}
}
Commentary:
In this example, replaceAll("\\s+", " ")
replaces multiple consecutive spaces with a single space. The trim()
method is again used to clean the edges. This is particularly useful for user-generated inputs where multiple spaces might be a common error.
Example 3: Using Java 11's String.strip()
public class StringStripExample {
public static void main(String[] args) {
String unicodeString = " \u2003Hello, World!\u2003 ";
String stripped = unicodeString.strip();
System.out.println("Unicode Original: '" + unicodeString + "'");
System.out.println("Stripped: '" + stripped + "'");
}
}
Commentary:
Java 11 introduces the strip()
method, which handles Unicode whitespace characters. In modern applications, supporting internationalization is critical, making this method a must-know for developers.
5. Conclusion
String trimming might seem trivial, but it plays a crucial role in data management and user input handling. The ability to effectively trim and clean strings can lead to more robust, error-free applications.
Key Takeaways
- Always trim strings before processing user input.
- Explore
replaceAll()
for more complex space handling. - Leverage
strip()
for modern applications needing Unicode support.
By understanding and mastering string trimming in Java, you can enhance the reliability and user-friendliness of your applications. For more information on string manipulation techniques, check out the Oracle Java Documentation.
Now that you've learned the ins and outs of string trimming, start incorporating these methods into your day-to-day programming tasks. You will notice a significant improvement in your application's performance and user experience. Happy coding!