Unlocking Java: Overcoming Common Beginner Challenges
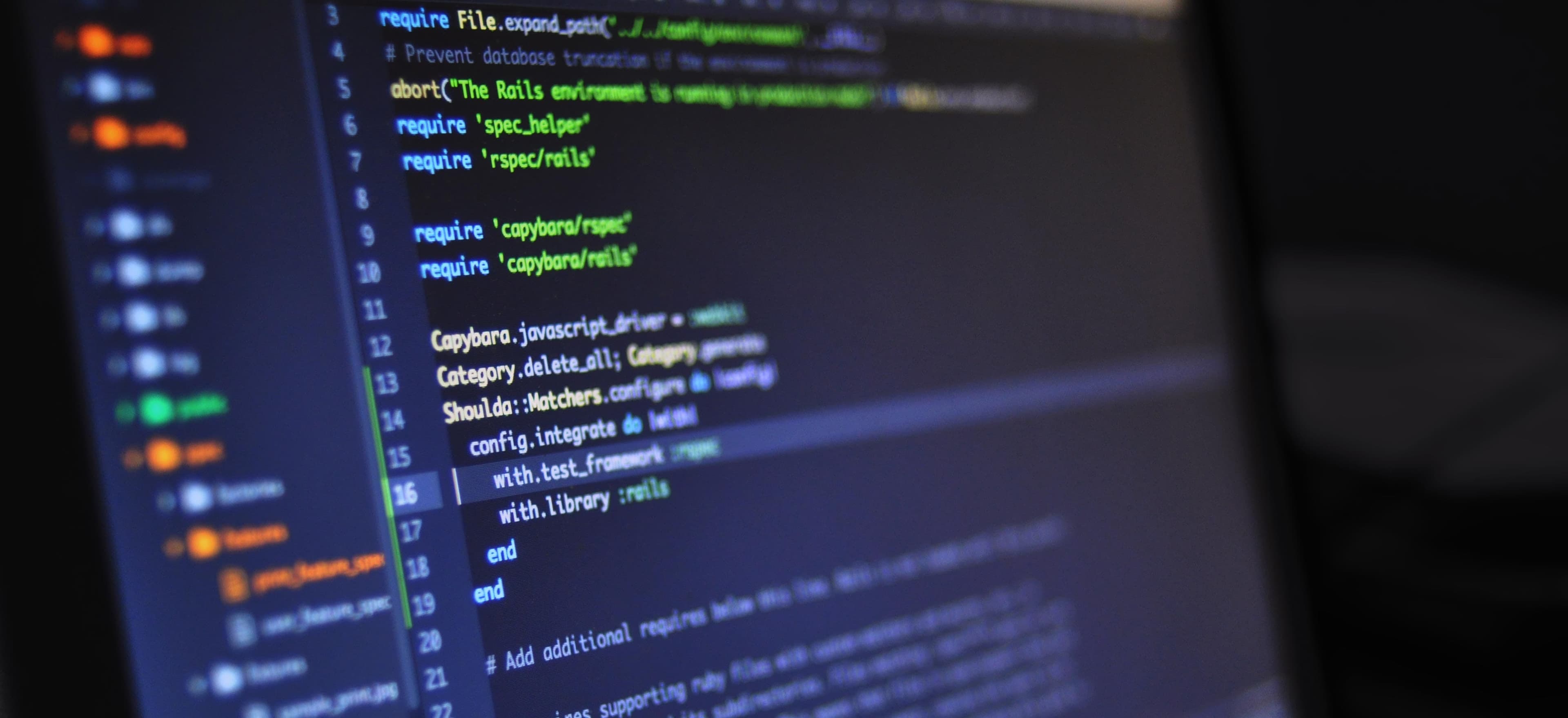
- Published on
Unlocking Java: Overcoming Common Beginner Challenges
Java is one of the most popular programming languages in the world. Known for its versatility and portability, it is the go-to language for many applications, from mobile apps to large-scale enterprise systems. However, beginners often encounter several challenges when starting their journey in Java. This blog post will help you unlock the potential of Java by addressing common beginner challenges and providing solutions, tips, and code snippets to overcome them.
Understanding Java's Syntax
Challenge: Complex Syntax
Java's syntax can appear intimidating for newcomers. It is important to recognize that while Java is stricter than some languages, this is often a benefit, ensuring that your code is more predictable and less prone to errors.
Solution: Getting Familiar with Java Syntax
Take some time to understand Java's basic structure. Every Java program starts with a class definition. Here's a simple example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Why this works:
public class HelloWorld
defines a class calledHelloWorld
.public static void main(String[] args)
is the main method where the program begins execution.System.out.println("Hello, World!");
prints the string to the console.
Understanding this foundational structure is essential for further learning.
Managing Data Types
Challenge: Understanding Data Types
Choosing the right data type is crucial in Java as it directly impacts memory usage and performance. Beginners often struggle with the different primitive data types and reference types.
Solution: Study Data Types and Their Usage
Java has eight primitive data types: byte
, short
, int
, long
, float
, double
, char
, and boolean
. Here’s a simple breakdown:
int age = 30; // Integer type
double salary = 55000.50; // Floating point type
char grade = 'A'; // Character type
boolean isEmployed = true; // Boolean type
Why it matters:
- Choosing the right data type can optimize memory consumption.
- Java’s strict typing reduces errors during compile-time, helping avoid type mismatch errors at runtime.
Deep Dive on Data Types
To understand further, consider using Oracle's Java Documentation. It provides comprehensive details about each data type's properties and use cases.
Control Structures: Loops and Conditionals
Challenge: Mastering Control Structures
Control structures dictate the flow of the program. Beginners often find loops and conditionals puzzling.
Solution: Practice with Examples
Here’s an example of a simple if-else conditional and a loop:
int number = 10;
// If-Else Conditional
if (number > 0) {
System.out.println("Positive Number");
} else {
System.out.println("Non-Positive Number");
}
// For Loop
for (int i = 1; i <= 5; i++) {
System.out.println("Iteration: " + i);
}
Why it works:
- The if-else structure lets your program take different paths based on conditions, enhancing its functionality.
- The for-loop allows you to repeat actions, essential for tasks that require iteration.
Understanding Scope and Lifetime
Understanding variable scope is key when using loops and conditionals. Each block of code can create its own scope, affecting variable visibility. Here’s a brief demonstration:
for (int i = 0; i < 5; i++) {
int temp = i * 2; // temp is scoped only within this loop
System.out.println(temp);
}
// System.out.println(temp); // This will throw an error
Why it matters:
- Knowing the scope helps prevent unintended errors and ensures your variables are accessible where needed.
Object-Oriented Programming (OOP)
Challenge: Grasping OOP Concepts
OOP is a core principle of Java, but beginners can be overwhelmed by concepts like inheritance, encapsulation, and polymorphism.
Solution: Start Simple with Classes and Objects
The first step to understand OOP is to get comfortable creating classes and objects. Here's a basic example:
class Car {
String color;
void displayColor() {
System.out.println("Color: " + color);
}
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car();
myCar.color = "Red";
myCar.displayColor(); // Output: Color: Red
}
}
Why this approach is effective:
- By defining a class (
Car
) and creating an object (myCar
), you take a fundamental step into OOP. - Methods like
displayColor()
encapsulate behavior, showcasing the principles of encapsulation and allowing code reuse.
Best Practices for OOP
For further reading on best practices and how OOP can be utilized effectively, check out Java’s OOP Principles. This resource covers in-depth details on class design, inheritance, and more.
Debugging: Finding and Fixing Errors
Challenge: Debugging Code
Beginners often find themselves stuck when their code throws errors or does not behave as expected.
Solution: Use Built-in Tools and Systematic Debugging
Start by using a simple debugging strategy: read error messages carefully, and take advantage of IDE debugging tools.
public class DebuggingExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3};
System.out.println(numbers[3]); // This will cause an ArrayIndexOutOfBoundsException
}
}
Why this intervention is critical:
- The above code will throw an error because array indexing starts from 0. It would help to validate array length before accessing elements.
Closing Remarks: Embrace the Learning Curve
Embarking on a journey to learn Java can be challenging, but each hurdle you encounter is an opportunity for growth. Familiarizing yourself with syntax, understanding data types, mastering control structures, embracing OOP principles, and becoming adept at debugging are all milestones on your road to proficiency.
As you dive deeper, consider joining communities like Stack Overflow or local Java user groups. They offer invaluable support and resources, making learning more interactive and enjoyable.
Remember, programming is an art that takes time to refine. Stay curious, be patient with yourself, and don’t hesitate to seek help when needed. Happy coding!
Checkout our other articles