Solving Dependency Injection Issues in Spring Configurations
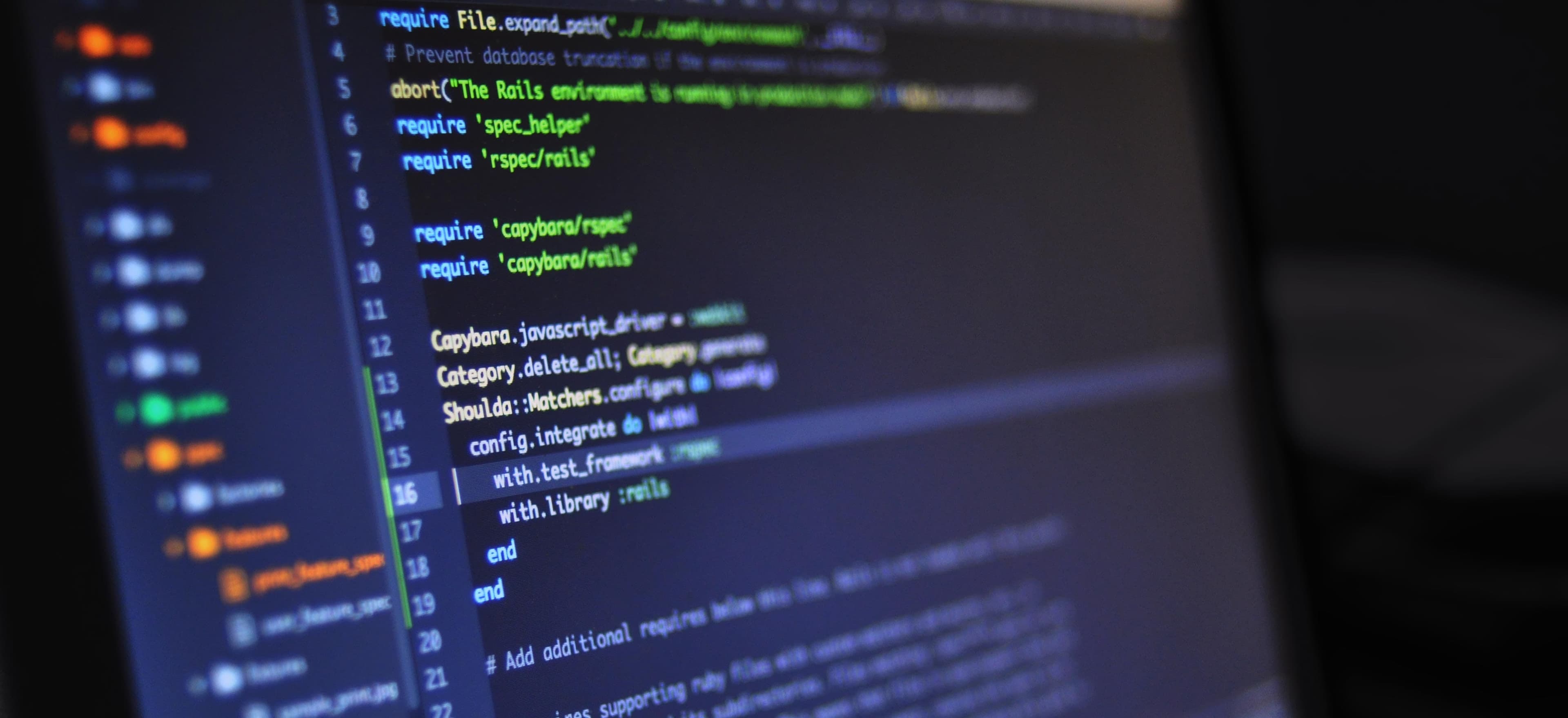
- Published on
Solving Dependency Injection Issues in Spring Configurations
Dependency Injection (DI) is a fundamental concept in Spring Framework that promotes loose coupling between components and enhances testability. However, various issues can arise during the configuration of DI, potentially leading to runtime errors and unexpected behaviors. In this blog post, we will explore common DI issues in Spring applications and provide strategies for resolving them effectively.
Understanding Dependency Injection in Spring
At its core, Dependency Injection is a design pattern in which an object's dependencies are provided externally, rather than being hardcoded within the object. Spring uses Inversion of Control (IoC) to manage dependencies, allowing you to define how components interact with each other.
Types of Dependency Injection
- Constructor Injection: Dependencies are provided through a class constructor.
- Setter Injection: Dependencies are set via setter methods.
- Field Injection: Dependencies are injected directly into class fields.
Example of Constructor Injection
@Component
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// Business logic methods
}
In the example above, UserService
depends on UserRepository
. By using constructor injection, we ensure that UserService
cannot be created without a UserRepository
, enforcing clear dependencies.
Common Dependency Injection Issues
Understanding how to configure DI effectively can prevent several common problems:
1. Circular Dependencies
Circular dependencies occur when two or more beans are dependent on each other, leading to a BeanCurrentlyInCreationException
.
Example of Circular Dependency
@Component
public class A {
@Autowired
private B b;
}
@Component
public class B {
@Autowired
private A a;
}
Solution: Refactor your code to eliminate circular dependencies, sometimes using interfaces or introducing a third class can resolve the issue. Alternatively, you can use setter injection instead of constructor injection.
Adjusted Example
@Component
public class A {
private B b;
@Autowired
public void setB(B b) {
this.b = b;
}
}
@Component
public class B {
private A a;
@Autowired
public void setA(A a) {
this.a = a;
}
}
2. Beans Not Found or NoUniqueBeanDefinitionException
If Spring cannot find a bean or multiple beans match a single dependency, you may encounter NoSuchBeanDefinitionException
or NoUniqueBeanDefinitionException
.
Resolving Bean Not Found
Ensure that your beans are correctly annotated with configuration annotations like @Component
, @Service
, or @Repository
.
Resolving No Unique Bean Definition
When multiple beans satisfy a dependency, you can use @Qualifier
to specify which bean to inject.
@Component
public class NotificationService {
private final MessagingService messagingService;
@Autowired
public NotificationService(@Qualifier("emailService") MessagingService messagingService) {
this.messagingService = messagingService;
}
}
3. Application Context Issues
Sometimes the application context may not load as expected, leading to ApplicationContextException
. This is commonly due to improper configuration of component scanning.
Solution
Make sure your package structure is consistent and update the @ComponentScan
annotation if needed:
@Configuration
@ComponentScan(basePackages = "com.example.myapp")
public class AppConfig {
}
4. Using the Wrong Scope
Beans in Spring can have different scopes: singleton, prototype, request, session, etc. Misusing these scopes can cause unexpected behavior.
For instance, a prototype-scoped bean should not be injected directly into a singleton-scoped bean:
@Component
public class SingletonBean {
@Autowired
private PrototypeBean prototypeBean; // This can lead to issues
}
Solution: Use @Lookup
method injection to resolve this problem.
@Component
public abstract class SingletonBean {
public void someMethod() {
PrototypeBean prototypeBean = getPrototypeBean();
prototypeBean.doSomething();
}
@Lookup
protected abstract PrototypeBean getPrototypeBean();
}
Best Practices for Dependency Injection in Spring
- Favor Constructor Injection: It ensures that dependencies are immutable and promotes easier testing.
- Decouple Beans: Keep your beans independent, using interfaces whenever possible.
- Utilize Profiles: Use Spring Profiles to manage environment-specific configurations.
- Keep the Application Context Lightweight: Only load what you need to minimize startup time.
- Understand Bean Scopes: Choose the appropriate bean scope based on usage.
My Closing Thoughts on the Matter
Dependency Injection significantly enhances the flexibility and maintainability of your Spring applications. By understanding common DI issues and applying best practices, you can avoid pitfalls and build robust applications.
Don’t forget to check out the official Spring Documentation for more in-depth guidelines on Dependency Injection.
Further Reading
- Spring Framework: Inversion of Control
- Understanding Bean Scopes
- Dependency Injection in Spring
By following these principles, you can tackle dependency injection issues effectively and create well-structured code that is easier to manage and scale. Happy coding!