Troubleshooting Spring Integration XML-Free Configuration Issues
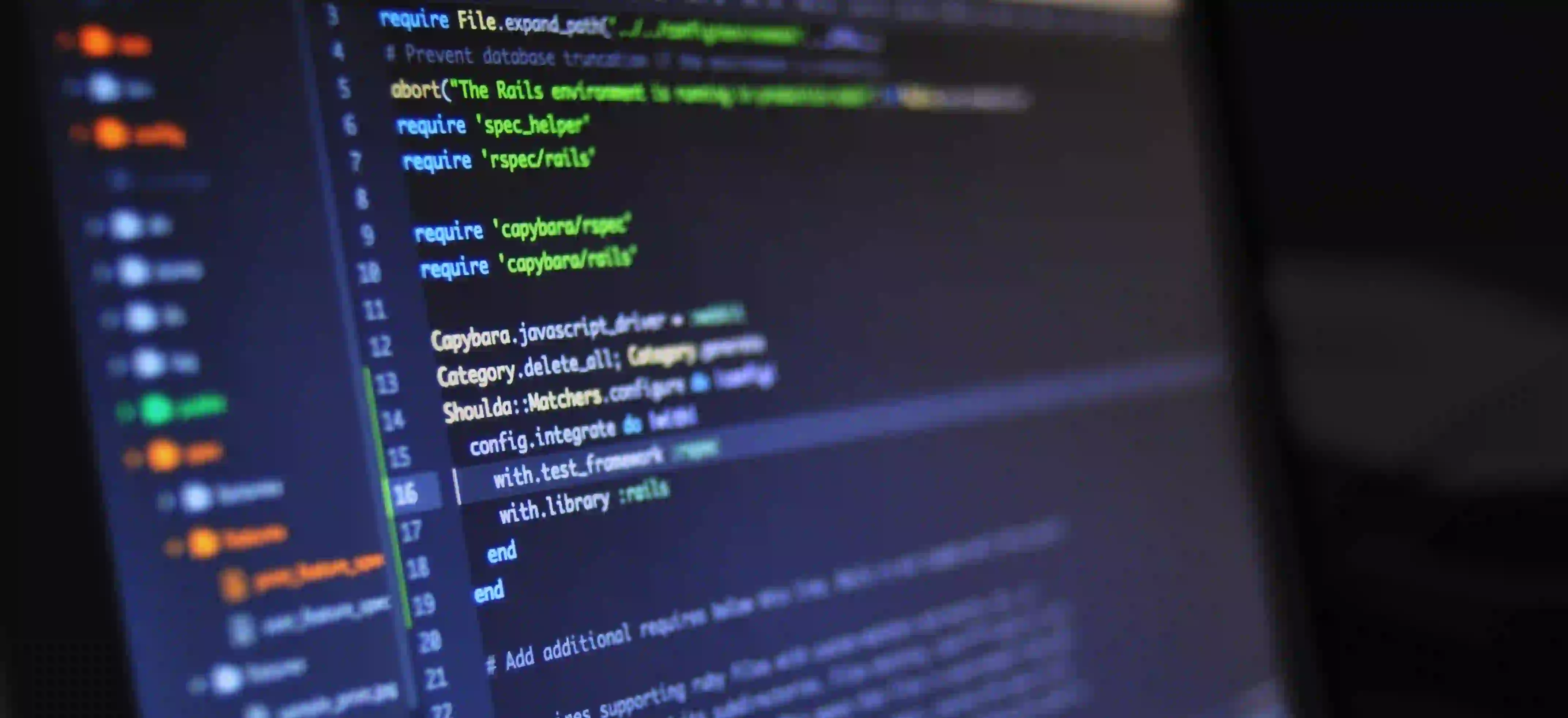
Troubleshooting Spring Integration XML-Free Configuration Issues
Spring Integration offers a powerful framework for building event-driven architectures through messaging. While XML configuration has historically been a common method for setting up applications, many developers now prefer the flexibility and clarity that Java-based configuration provides. However, transitioning to XML-free (Java Config) setups can introduce its own set of challenges. This article aims to guide you through common issues developers face when configuring Spring Integration without XML, and how to effectively troubleshoot these problems.
Benefits of XML-Free Configuration
Before diving into troubleshooting, let’s briefly discuss the benefits of using XML-Free configuration:
- Type Safety: Java configuration provides compile-time type checking, helping catch errors early in the development process.
- Easier Refactoring: IDEs can offer better support for code navigation and refactoring in Java compared to XML.
- Enhanced Readability: Straightforward Java syntax can be more readable than complex XML structures.
For more on Spring Integration and its features, you can refer to the Spring Integration documentation.
Key Troubleshooting Steps
Here are some common issues that may arise during XML-free configuration, along with guidance on how to resolve them.
1. Missing Bean Definitions
Issue: One of the most frequent issues when transitioning from XML to Java configuration is the accidental omission of bean definitions.
Solution: Ensure that all necessary beans are explicitly declared in your configuration class. For example:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.integration.dsl.IntegrationFlow;
import org.springframework.integration.dsl.IntegrationFlows;
@Configuration
public class IntegrationConfig {
@Bean
public IntegrationFlow myFlow() {
return IntegrationFlows.from("inputChannel")
.handle("myService", "process")
.get();
}
}
2. Channel Mismatch
Issue: Channels are fundamental in Spring Integration. Mismatched channel definitions can lead to failures when messages fail to route properly.
Solution: Double-check the channels you are using and ensure they are correctly mapped. For example, if you have defined a channel as follows:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.integration.channel.DirectChannel;
import org.springframework.messaging.MessageChannel;
@Configuration
public class ChannelConfig {
@Bean
public MessageChannel inputChannel() {
return new DirectChannel();
}
}
Make sure that the inputChannel
is referred to correctly wherever it is being utilized. Using a property placeholder, for instance, can lead to quiet errors.
3. Incorrect Annotations
Issue: If annotations are not appropriately placed or wrong ones are used, this can hinder the Spring context from loading the configuration.
Solution: Confirm that your configuration class is annotated with @Configuration
. Also, ensure you are utilizing the appropriate @EnableIntegration
annotation:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.integration.annotation.EnableIntegration;
@Configuration
@EnableIntegration
public class ApplicationConfig {
// Your beans here
}
4. Component Scanning Issues
Issue: When using Java configuration, classes may not be auto-detected if component scanning is not configured correctly.
Solution: Ensure that your component scan includes your integration configuration class:
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
@Configuration
@ComponentScan(basePackages = "com.example")
public class AppConfig {
}
5. Message Flow Errors
Issue: Incorrect message flows can result in lost messages or exceptions during processing.
Solution: Use debugging tools or logging to trace the flow of messages. For instance, configuring a logging channel adapter can be exceedingly helpful:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.integration.dsl.IntegrationFlow;
import org.springframework.integration.dsl.IntegrationFlows;
import org.springframework.integration.dsl.Transformers;
@Configuration
public class LoggingFlow {
@Bean
public IntegrationFlow loggingFlow() {
return IntegrationFlows.from("inputChannel")
.transform(Transformers.toString())
.handle(message -> System.out.println("Received: " + message))
.get();
}
}
6. Spring Context Misconfiguration
Issue: Sometimes, the Spring Context may not be setup correctly, causing beans to not be initialized.
Solution: Ensure that the application is running in a Spring context. The context can be created and initialized using:
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class Main {
public static void main(String[] args) {
AnnotationConfigApplicationContext context =
new AnnotationConfigApplicationContext(AppConfig.class);
// Your logic here
context.close();
}
}
7. Dependency Injection Failures
Issue: Failing to correctly inject dependencies can lead to NullPointerExceptions
or context initialization failures.
Solution: Make sure you verify that all dependencies are marked with @Autowired
and are being properly scanned.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class MyService {
private final MyRepository repo;
@Autowired
public MyService(MyRepository repo) {
this.repo = repo;
}
public void process() {
// Logic here
}
}
8. Testing Your Configuration
After making changes, ensure to test your configuration thoroughly. Spring provides an excellent testing module that allows you to mock the Spring context.
import org.junit.jupiter.api.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import static org.junit.jupiter.api.Assertions.*;
public class MyServiceTest {
@Test
public void testMyService() {
ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
MyService service = context.getBean(MyService.class);
assertNotNull(service);
// Further assertions
}
}
The Last Word
Integrating your application using Spring without XML can undoubtedly improve maintainability and readability. However, as outlined above, transitioning to XML-free configuration requires diligence. By closely following the best practices outlined here, you can troubleshoot and resolve common issues effectively.
For further reading on specific aspects of Spring Integration, check out these resources:
- Spring Integration Tutorials
- Java Configuration in Spring
With a well-structured approach to XML-free configuration, you're equipped to build a robust and efficient messaging solution. Happy coding!