Constructor vs Setter: Which is Best for Your Code?
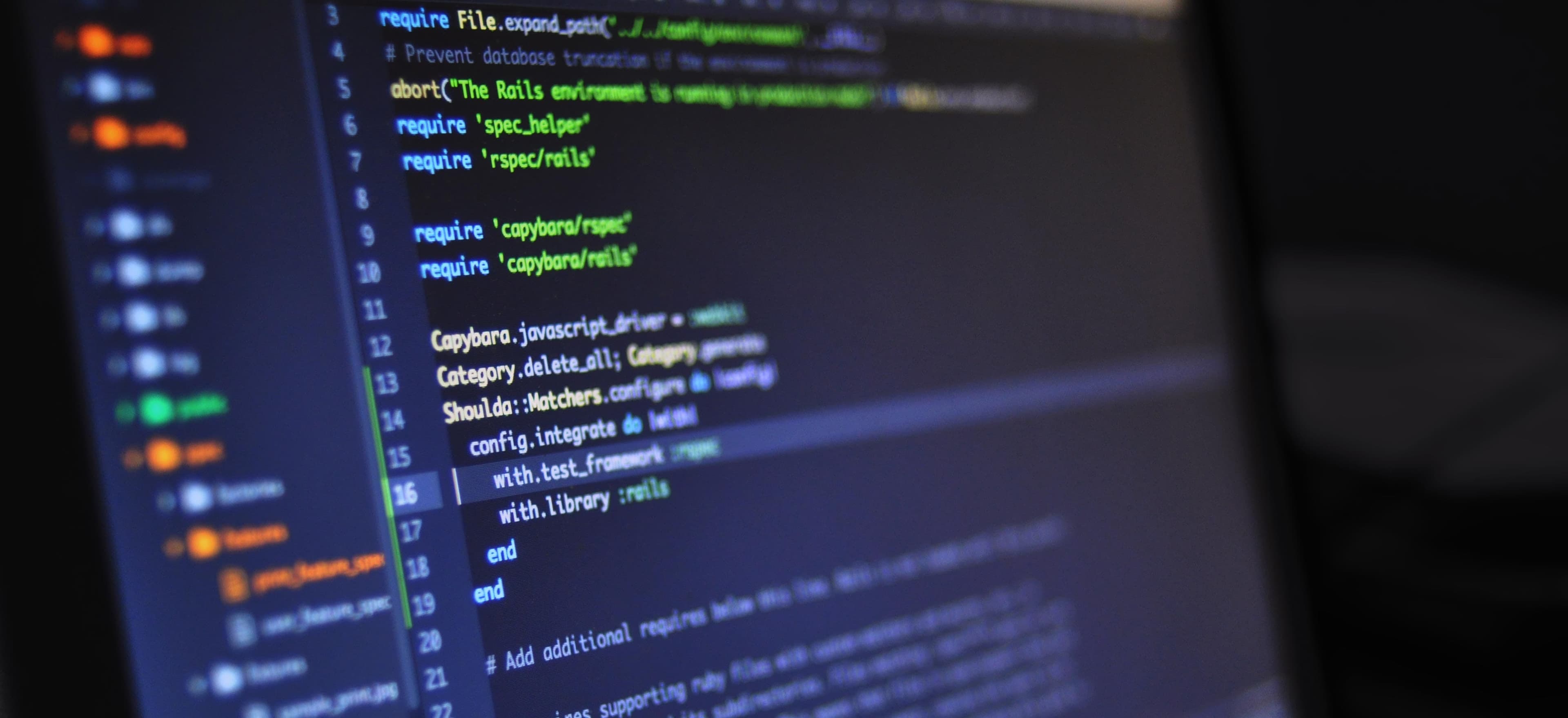
- Published on
Constructor vs Setter: Which is Best for Your Code?
When it comes to initializing objects in Java, developers often find themselves torn between two popular approaches: using constructors or employing setter methods. Each has its own merits and drawbacks. In this post, we'll delve into both options, evaluate their pros and cons, and ultimately help you decide which method suits your programming needs best.
Understanding Constructors
A constructor is a special method that gets called when creating an instance of a class. Its primary function is to initialize the object's attributes, ensuring that the object is in a valid state right from the moment it's created.
Example of a Constructor
Here is a simple class using a constructor to initialize its fields:
public class User {
private String userName;
private String email;
// Constructor
public User(String userName, String email) {
this.userName = userName;
this.email = email;
}
// Getter methods
public String getUserName() {
return userName;
}
public String getEmail() {
return email;
}
}
Why Use a Constructor?
-
Immutable Objects: If you want your objects to be immutable (i.e., their state cannot change after creation), constructors are your best choice. Once you create an object, its state remains consistent.
-
Mandatory Fields: Constructors allow you to enforce the requirement for mandatory fields. If a necessary value isn’t provided, the compiler will throw an error.
-
Cleaner Initialization Logic: The initialization logic happens in one place, making it easier to read and maintain.
-
Encapsulation: A constructor can enforce encapsulation by preventing direct access to internal fields, keeping the object state safe and sound.
Understanding Setter Methods
Setter methods are part of the class API that allow you to set or change the value of an object's attributes after it has been created.
Example of Setter Methods
Here's how the above class might be revised to include setter methods:
public class User {
private String userName;
private String email;
// Constructor
public User() {
// Default constructor
}
// Setter methods
public void setUserName(String userName) {
this.userName = userName;
}
public void setEmail(String email) {
this.email = email;
}
// Getter methods
public String getUserName() {
return userName;
}
public String getEmail() {
return email;
}
}
Why Use Setter Methods?
-
Flexibility: With setter methods, you can change the state of your objects at any point after they've been instantiated. This is ideal for objects that need to adapt over time.
-
Optional Fields: If your object has optional attributes, setters can make initializing such attributes easier without complicating the constructor.
-
Clarity: By providing meaningful methods, you can make your code easier to understand. Reading
user.setEmail("example@example.com")
is often clearer than analyzing multiple constructor overloads. -
Complex Initialization Logic: Setters allow for setting a value at any moment, which can be useful if your initialization logic is complex or needs to react to variables at runtime.
Comparison: Constructor vs Setter
To better analyze which method is more suitable for your needs, we can categorize these two approaches based on several criteria:
1. Object State and Validation
- Constructor: Values are validated at the very moment of object creation. This means that you can enforce necessary arguments right away.
- Setter: Validation can occur each time a setter is called. This can be good for flexibility, but may introduce bugs if not carefully managed.
2. Readability and Maintenance
- Constructor: All required initialization is in one place, making the constructor the obvious choice if your object is already complicated or has many mandatory fields.
- Setter: Each setter can introduce complexity throughout the code since object state can be changed at any time and potentially from different parts of the application.
3. Immutability
- Constructor: If immutability is required, using a constructor is necessary. Once created, the state cannot be altered.
- Setter: Values can be changed freely with setters, which is unsuitable for immutable design.
4. Performance
While the performance difference between constructors and setters is usually negligible, constructors can sometimes be more efficient because they perform all object initialization at once. The hassle of repetitive calls to setter methods can slightly hinder performance.
When to Use Each Approach
Use Constructors when...
- Your object has mandatory fields.
- You want to create immutable objects.
- You want to enforce certain invariants effectively at the moment of creation.
Use Setter Methods when...
- Your object has optional fields.
- You need to modify the object's state throughout its lifecycle.
- You expect to perform complex object state adjustments.
Best Practices
-
Combine Both: For many classes, using both constructors and setters can provide the best of both worlds. Use constructors for mandatory fields while providing setters for optional fields.
-
Use Builder Pattern: If you have a class with many fields or optional parameters, consider using the Builder Pattern. This pattern provides a fluent interface for object creation without the complexity of constructors with numerous parameters.
-
Keep Methods Simple: Always avoid complex logic in setters. Their role should be purely to assign values.
-
Explicit State: Always ensure that the object state is valid after both constructor and setter usage. Sometimes additional validation logic is necessary.
-
Document Your Code: Clearly comment on your constructors and setters. Speak to the intended side effects, especially for setters.
Final Thoughts
In summary, whether to use constructors or setter methods in Java largely depends on the unique requirements of your application. Both approaches have their unique strengths and weaknesses.
- Use constructors if you prioritize immutability and immediate validation of required attributes.
- Opt for setter methods if you need flexibility and the ability to modify object state post-creation.
By understanding the scenarios, you can make more informed decisions that will lead to better code structure, enhanced maintainability, and cleaner designs.
Remember, the best practices often involve a thoughtful combination of both constructors and setters tailored to your programming problem. Happy coding!
For more insights into Java programming, consider checking out Java Design Patterns for design pattern applications in real-world projects or Effective Java by Joshua Bloch for deeper dives into best practices!