Seamless Integration of Kubernetes Services in CDI Beans
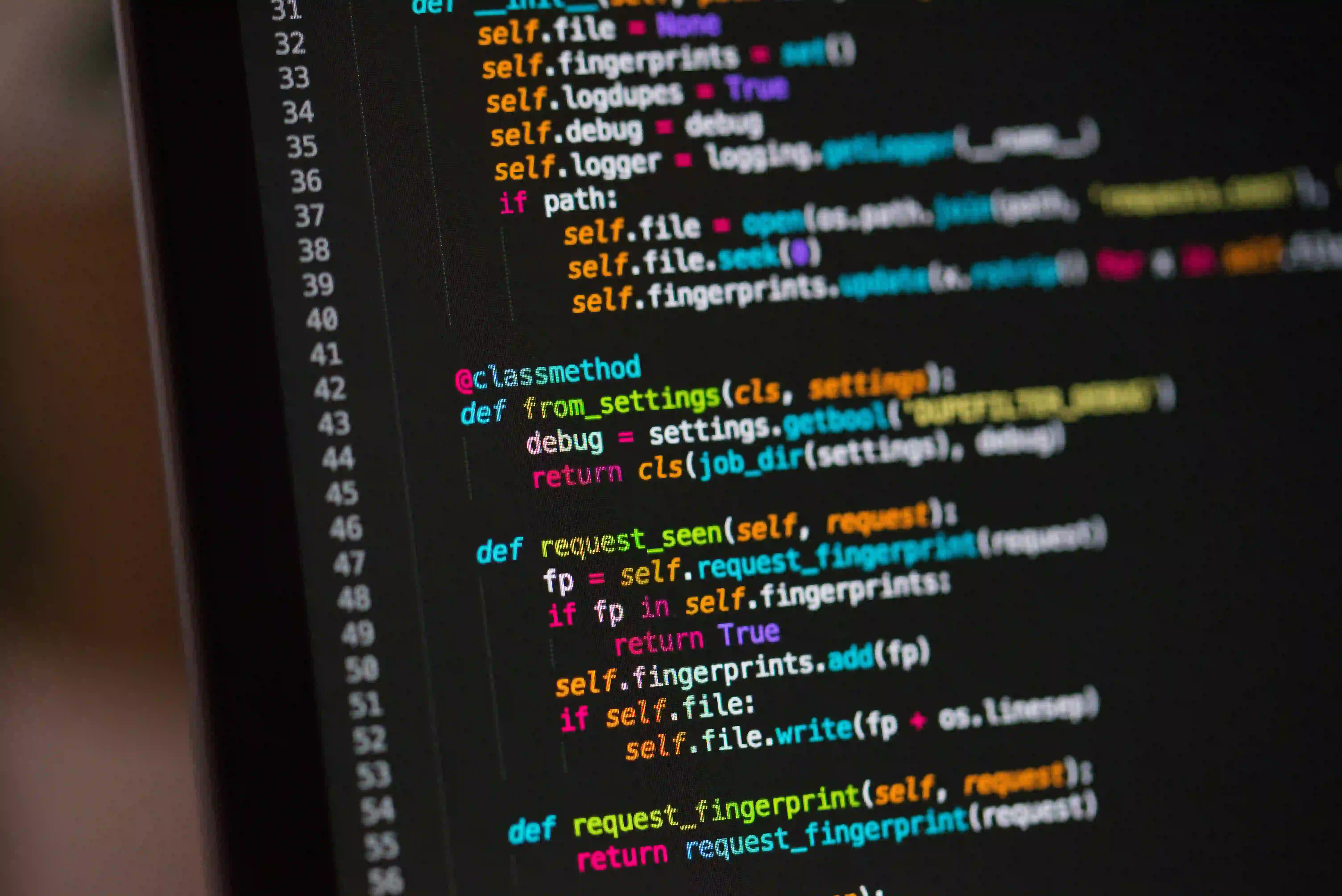
Seamless Integration of Kubernetes Services in CDI Beans
The rise of cloud-native applications has made it essential to combine the capabilities of various modern technologies. One such pair is Kubernetes and Contexts and Dependency Injection (CDI). Together, they create a powerful environment for building robust services. In this blog post, we’ll delve into how to integrate Kubernetes services into CDI beans seamlessly.
Understanding Kubernetes and CDI
What is Kubernetes?
Kubernetes is an open-source platform that automates deploying, scaling, and operating application containers. With its rich ecosystem, Kubernetes allows developers to focus on writing code instead of managing the infrastructure.
What is CDI?
Contexts and Dependency Injection (CDI) is a set of APIs in Java EE that provides a powerful framework for developing Java applications. CDI offers a means to manage the lifecycle of beans, making it easier to create loosely-coupled applications. It enhances collaboration between the components of a Java application, improving modularity.
The Need for Integration
As applications become increasingly distributed, services need to communicate efficiently with one another. Integrating Kubernetes services directly into CDI beans allows for better management of these interactions. Here are key benefits:
- Streamlined Communication: Simplifies service discovery and interaction among microservices.
- Improved Scalability: Use Kubernetes features such as load balancing and auto-scaling.
- Enhanced Reliability: Leverage Kubernetes' health checks and self-healing capabilities.
Setting Up Your Environment
Before diving into the code, ensure your environment is ready. You will need:
- A Kubernetes cluster (e.g., Minikube, GKE, or AKS).
- Java 11+ with a suitable build tool like Maven or Gradle.
- A CDI-compatible framework (e.g., Payara, WildFly).
Step 1: Configure Kubernetes
Make sure you have your Kubernetes context set up. Below is a simple example of a Deployment
and a Service
configuration for a Java application.
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app
spec:
replicas: 2
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-app
image: my-app:latest
ports:
- containerPort: 8080
---
apiVersion: v1
kind: Service
metadata:
name: my-app-service
spec:
type: ClusterIP
selector:
app: my-app
ports:
- port: 80
targetPort: 8080
Step 2: Creating the CDI Bean
Next, create a CDI bean that will interact with your Kubernetes service. Below is an exemplary Java implementation.
import javax.enterprise.context.ApplicationScoped;
import javax.inject.Inject;
import javax.ws.rs.client.Client;
import javax.ws.rs.client.ClientBuilder;
import javax.ws.rs.client.WebTarget;
import javax.ws.rs.core.MediaType;
@ApplicationScoped
public class MyServiceClient {
private final String baseUrl = "http://my-app-service";
@Inject
private SomeConfiguration config;
private Client client;
public MyServiceClient() {
this.client = ClientBuilder.newClient();
}
public String fetchData() {
WebTarget target = client.target(baseUrl).path("data");
return target.request(MediaType.APPLICATION_JSON).get(String.class);
}
}
Commentary on the Code
-
Client Initialization: We use Jakarta EE's
ClientBuilder
to create a REST client. This encapsulates the complexity of creating HTTP connections. -
Base URL: The service URL is set to point to the Kubernetes service we defined earlier. This allows seamless interaction regardless of pod scaling.
-
Method for Data Fetching: The
fetchData
method illustrates a simple GET request that retrieves data from another microservice.
Using the CDI Bean in Your Application
With the CDI bean created, you can now inject and use it in other parts of your application. Consider the example below:
import javax.enterprise.context.RequestScoped;
import javax.inject.Inject;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
@Path("/consumer")
@RequestScoped
public class MyConsumerResource {
@Inject
private MyServiceClient myServiceClient;
@GET
public String getData() {
return myServiceClient.fetchData();
}
}
Explanation
- Annotation Usage: A
@Path
annotation denotes this class as a REST endpoint, allowing for a clean RESTful API design. - Dependency Injection: The
MyServiceClient
is injected, ensuring that all instances are properly managed by the CDI container.
Testing Your Integration
Testing your setup is crucial before deploying to production. Use tools like Postman or curl to hit your API endpoint. If everything is configured correctly, you should receive a response from the service running in your Kubernetes cluster:
curl http://your-domain/consumer
Ensure your network policies allow ingress to the service.
The Last Word
Integrating Kubernetes services with CDI beans can significantly enhance the modularity and scalability of your Java applications. By following the steps and code examples outlined above, you can create a robust communication layer between microservices that harnesses the strengths of both technologies.
Further Reading
- Kubernetes Documentation
- CDI Specification
- Jakarta REST Client
Seamlessly integrating Kubernetes with CDI opens up a world of possibilities for your Java applications. Embrace the power of modern architecture and deploy services that are resilient, scalable, and easy to maintain. Happy coding!