Common Mistakes When Generating Maven Source and Javadoc Artifacts
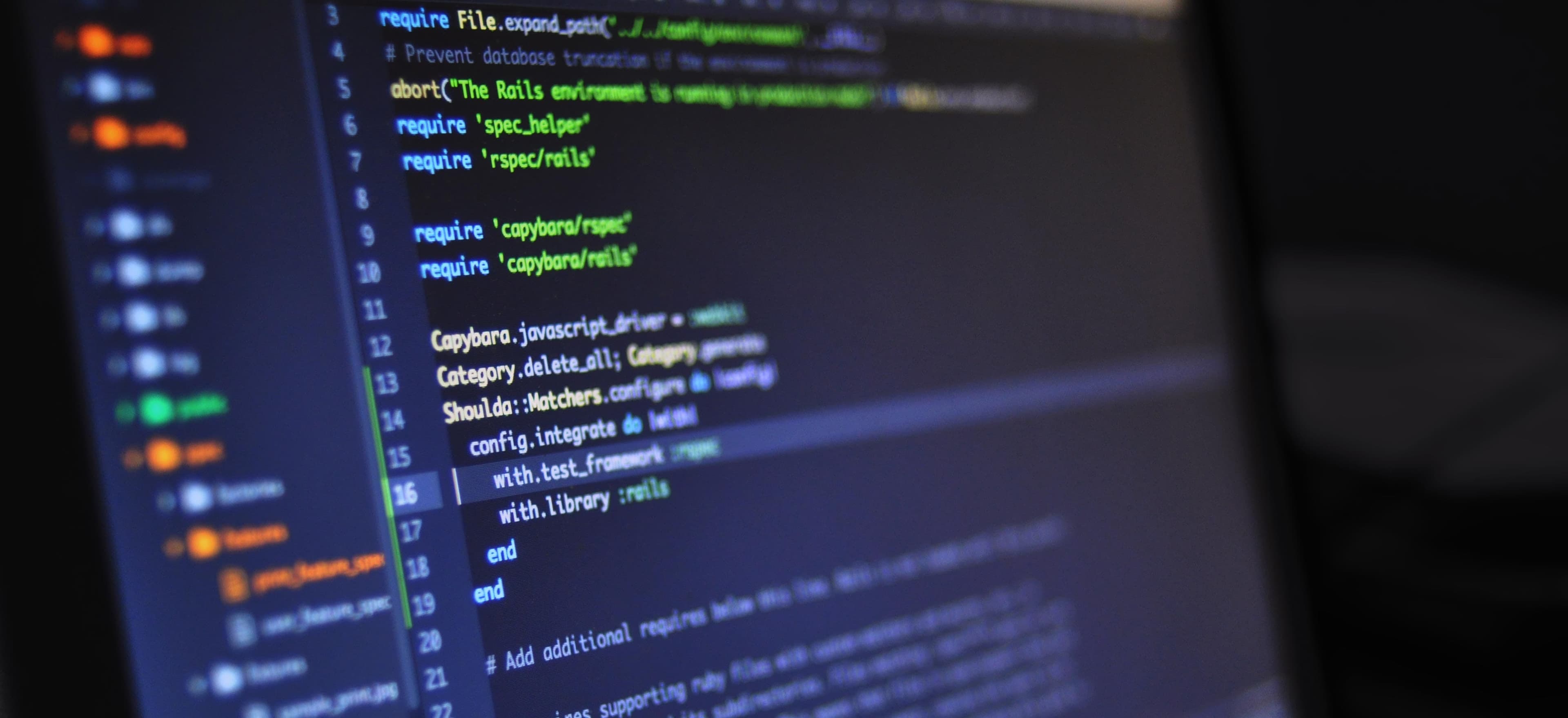
- Published on
Common Mistakes When Generating Maven Source and Javadoc Artifacts
Maven is a powerful build automation tool primarily used for Java projects. One of its many capabilities is the generation of source and Javadoc artifacts, which are crucial for providing usable documentation and source code alongside compiled JAR files. However, despite its strengths, many developers face challenges when creating these artifacts. This article will delve into common mistakes made during this process, providing best practices and code snippets to help avoid pitfalls.
Understanding Maven Source and Javadoc Artifacts
Before diving into mistakes, let's clarify what we mean by source and Javadoc artifacts.
-
Source Artifacts: These are essentially JAR files that contain the source code of your project. They allow other developers to browse or debug the code, improving collaboration.
-
Javadoc Artifacts: These artifacts contain the generated documentation for your classes, methods, and packages. They are valuable for anyone who needs to understand how to use your library without diving deep into the code.
Now that we have a solid understanding of these terms, let’s address common pitfalls.
1. Missing Plugin Configuration
One of the most frequent mistakes is failing to configure the necessary Maven plugins for generating source and Javadoc artifacts.
Solution
Make sure to include the maven-source-plugin
and maven-javadoc-plugin
in your pom.xml
. Here's how to add them:
<project>
...
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-source-plugin</artifactId>
<version>3.2.1</version>
<executions>
<execution>
<id>attach-sources</id>
<goals>
<goal>jar</goal>
</goals>
</execution>
</executions>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-javadoc-plugin</artifactId>
<version>3.3.0</version>
<executions>
<execution>
<id>attach-javadocs</id>
<goals>
<goal>jar</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
Why: The maven-source-plugin
and maven-javadoc-plugin
must be explicitly defined to tell Maven to generate source and Javadoc JARs during the build process.
2. Ignoring Source Encoding
Another common error is neglecting to specify the source encoding. This can lead to issues if special characters are included in the source code.
Solution
Add the following property in your pom.xml
:
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
Why: Setting the source encoding ensures that your source files are interpreted correctly, preventing potential character-related errors during compilation and documentation generation.
3. Not Executing Goals Properly
Sometimes, developers may not execute the necessary goals to package the source and Javadocs. This typically leads to ".jar" files being generated without the corresponding artifacts.
Solution
To build and attach the sources and Javadocs, run:
mvn clean install
If you're generating documentation on demand, you can also run:
mvn javadoc:javadoc
Why: The install
goal is crucial as it executes the build lifecycle and ensures that all defined packaging rules, including the source and Javadoc attachments, are honored.
4. Dependency Scoping Issues
When generating Javadoc, another frequent oversight is related to dependency scope. If dependencies are marked as "provided" or "runtime," they will not be included in the Javadoc output.
Solution
Use the following example for your dependencies:
<dependency>
<groupId>org.example</groupId>
<artifactId>example-dependency</artifactId>
<version>1.0.0</version>
<scope>compile</scope>
</dependency>
Why: Using the correct scope ensures that the required dependencies are available when generating your Javadoc, resulting in more comprehensive documentation.
5. Ignoring Java Compiler Version
It's important to specify which Java version you're using for your project. Failing to do this can lead to compatibility issues and prevent proper documentation generation.
Solution
Include the following configuration in your pom.xml
:
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
Why: Specifying the Java version ensures that the generated artifacts are compatible with the intended Java runtime environment.
6. Lack of Inline Comments and Documentation
Developers often forget the importance of inline comments in Javadoc. While Maven can generate Javadoc from code annotations, lack of explanations can lead to incomplete documentation.
Solution
For effective documentation, ensure your Java classes and methods have Javadoc comments:
/**
* Adds two integers together.
*
* @param a the first integer
* @param b the second integer
* @return the sum of a and b
*/
public int add(int a, int b) {
return a + b;
}
Why: Inline comments provide clarity and context, ensuring that users understand the purpose and functionality of your code when they review the generated documentation.
Bringing It All Together
Generating Maven source and Javadoc artifacts can significantly enhance your project's usability and maintainability. By avoiding the common mistakes discussed in this article, you can ensure that your artifacts are correctly produced, well-documented, and easy to use for developers and users alike.
For further reading, consider exploring the official Maven documentation on Creating Javadoc, which delves deeper into the plugin configurations and additional parameters.
Incorporating these best practices will not only improve your development workflow but also promote collaboration within your team and the wider developer community. Happy coding!
Checkout our other articles