Unlocking Java's Potential: Common Pitfalls in Real Apps
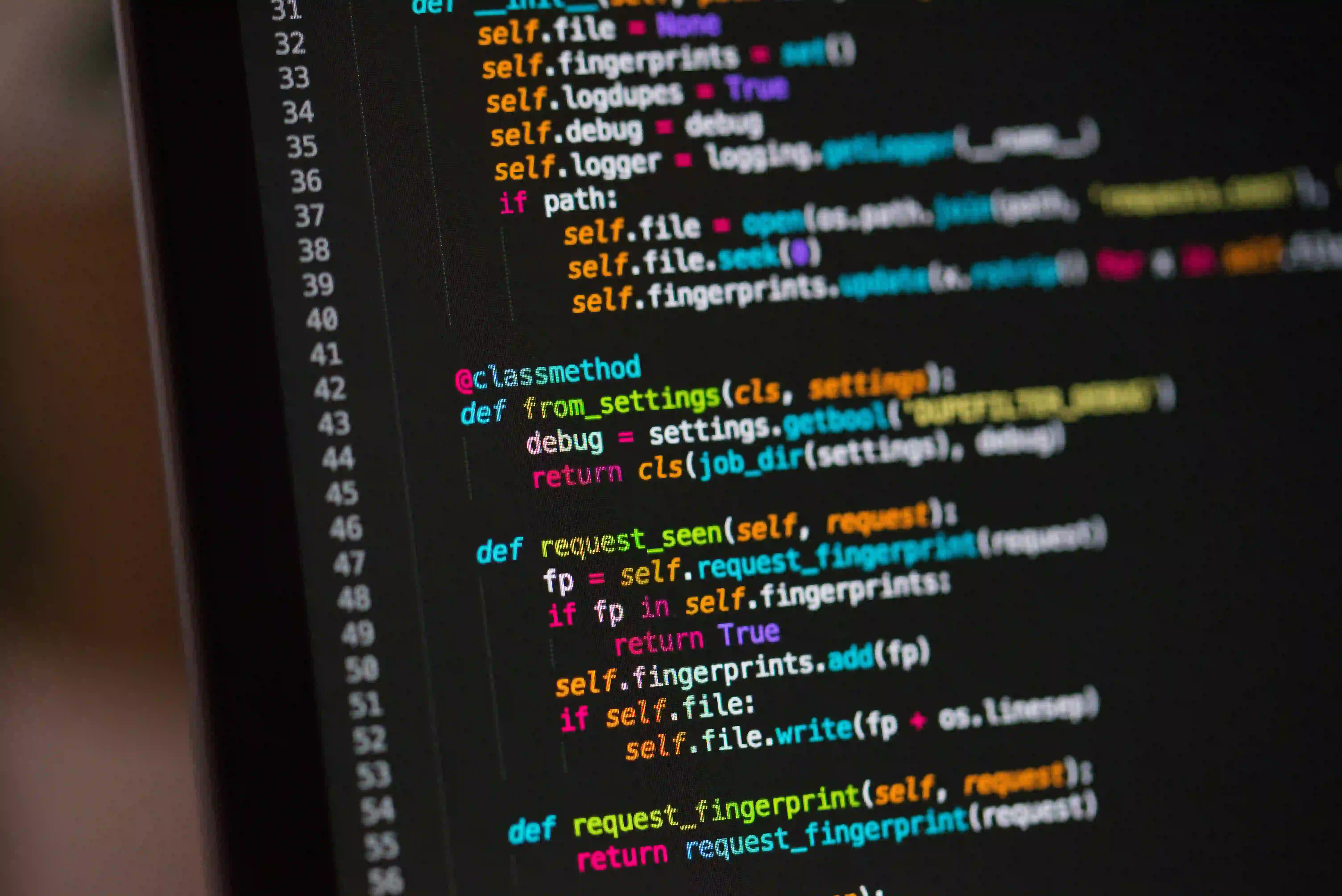
Unlocking Java's Potential: Common Pitfalls in Real Apps
Java remains one of the most popular programming languages, and for good reason. Its platform independence, robust performance, and vast ecosystem of libraries make it a preferred choice for developing everything from small-scale applications to large enterprise systems. However, even seasoned developers can encounter pitfalls in the real world that can lead to inefficient, buggy, or slow applications. In this post, we will explore some common pitfalls in Java development, how to avoid them, and best practices for ensuring high-quality applications.
Table of Contents
- Understanding Java Memory Management
- Overusing Static Variables
- Ignoring Exception Handling
- Mismanaging Threads
- Neglecting the Power of Streams
- Using Primitive Types Inefficiently
- Conclusion
1. Understanding Java Memory Management
Java uses a process called garbage collection (GC) to manage memory. At its core, GC automatically reclaims memory that objects are no longer referencing, but it can lead to unexpected performance issues if not properly understood.
GC Pitfalls to Avoid
- Long GC Pauses: Long pauses during garbage collection can interrupt application performance. Using tools like Java VisualVM can help you analyze memory usage and GC effectiveness.
- Excessive Object Creation: Reusing objects when possible instead of creating new ones can minimize GC overhead.
public class ObjectPool {
private List<MyObject> available = new ArrayList<>();
// Fetch object from the pool instead of creating new
public MyObject getObject() {
if (available.isEmpty()) {
return new MyObject(); // Just create a new one if pool is empty
}
return available.remove(available.size() - 1);
}
public void releaseObject(MyObject obj) {
available.add(obj); // Return to pool instead of garbage collecting
}
}
In this code snippet, an object pool is created to effectively manage memory and minimize frequent instantiation of objects, which can lead to unnecessary GC.
2. Overusing Static Variables
Static variables can lead to unexpected behavior, especially in multi-threaded environments. They maintain state across instances, which can cause issues if not handled properly.
Why Avoid Static Variables?
- Memory Leaks: Static references can prevent objects from being garbage collected.
- Unexpected Behavior: Changes in one part of the application affect other parts unexpectedly.
public class Configuration {
private static Configuration instance;
private Configuration() {}
public static synchronized Configuration getInstance() {
if (instance == null) {
instance = new Configuration();
}
return instance;
}
}
While this singleton pattern prevents multiple instances from being created, static variables can lead to shared state issues across threads. Use this pattern wisely and consider alternatives like dependency injection.
3. Ignoring Exception Handling
Many developers prioritize code efficiency or readability over proper exception handling. But robust error handling is crucial to an application's resilience.
Key Practices
- Catch Specific Exceptions: Avoid catching generic exceptions; it hides bugs.
- Logging and Recovery: Always log exceptions and ensure a way to recover from them or deliver meaningful error messages.
public void readFile(String filepath) {
try (BufferedReader br = new BufferedReader(new FileReader(filepath))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (FileNotFoundException e) {
System.err.println("File not found: " + filepath);
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
}
In this example, specific exceptions are caught and logged, preventing the application from crashing while providing useful feedback.
4. Mismanaging Threads
Concurrency can be a double-edged sword. If managed incorrectly, it can lead to race conditions, deadlocks, and wasted resources.
Best Practices for Thread Management
- Use Executors: Instead of managing threads manually, employ the Executor framework.
- Avoid Shared Mutable State: Immutable objects help prevent concurrency issues since their state cannot be altered.
Executors.newFixedThreadPool(10).submit(() -> {
// Task goes here
});
This snippet shows the elegant way to create and manage threads efficiently using the Executor framework, significantly reducing the chance of concurrency issues.
5. Neglecting the Power of Streams
Java 8 introduced streams, a powerful abstraction for working with sequences of data. Neglecting this feature often leads to verbose and less efficient code.
Stream Benefits
- Conciseness: Stream operations can reduce boilerplate code.
- Parallel Operations: Streams can easily leverage multi-core architectures.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> sortedNames = names.stream()
.filter(name -> name.startsWith("A"))
.sorted()
.collect(Collectors.toList());
System.out.println(sortedNames); // Output: [Alice]
This use of streams allows for concise filtering and sorting in a single line, demonstrating the elegance and power of Java 8’s functional programming features.
6. Using Primitive Types Inefficiently
While primitives are generally more efficient than their wrapper classes, using them excessively in collections requires careful consideration regarding types.
The Primitive vs Wrapper Debate
- Memory Considerations: Each wrapper type consumes more memory than its primitive counterpart.
- Autoboxing Pitfalls: Converting between primitive types and wrappers (autoboxing) can affect performance.
List<Integer> integers = new ArrayList<>(); // Use int[] instead when possible
for (int i = 0; i < 1000; i++) {
integers.add(i); // Autoboxing can add overhead
}
In this code, using an int[]
would be more efficient than using a list of Integer
due to the overhead of autoboxing.
7. Conclusion
Java is a powerful programming language with immense potential. By being aware of common pitfalls and applying best practices, you can maximize the performance and maintainability of your applications. From understanding memory management to leveraging modern Java features like streams, enhancing your Java skills requires continuous learning and adaptation.
For more on Java best practices, consider exploring these resources:
By unlocking Java’s full potential and avoiding these common pitfalls, you are setting the stage for building robust, efficient, and scalable applications. Happy coding!